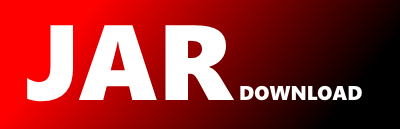
fuookami.ospf.kotlin.framework.solver.SerialCombinatorialLinearSolver.kt Maven / Gradle / Ivy
package fuookami.ospf.kotlin.framework.solver
import org.apache.logging.log4j.kotlin.*
import fuookami.ospf.kotlin.utils.math.*
import fuookami.ospf.kotlin.utils.error.*
import fuookami.ospf.kotlin.utils.functional.*
import fuookami.ospf.kotlin.core.frontend.model.*
import fuookami.ospf.kotlin.core.backend.intermediate_model.*
import fuookami.ospf.kotlin.core.backend.solver.*
import fuookami.ospf.kotlin.core.backend.solver.output.*
class SerialCombinatorialLinearSolver(
private val solvers: List>,
private val stopErrorCode: Set = setOf(ErrorCode.ORModelNoSolution, ErrorCode.ORModelUnbounded)
): LinearSolver {
private val logger = logger()
companion object {
@JvmName("constructBySolvers")
operator fun invoke(
solvers: List,
stopErrorCode: Set = setOf(ErrorCode.ORModelNoSolution, ErrorCode.ORModelUnbounded)
): SerialCombinatorialLinearSolver {
return SerialCombinatorialLinearSolver(solvers.map { lazy { it } }, stopErrorCode)
}
@JvmName("constructBySolverExtractors")
operator fun invoke(
solvers: List<() -> LinearSolver>,
stopErrorCode: Set = setOf(ErrorCode.ORModelNoSolution, ErrorCode.ORModelUnbounded)
): SerialCombinatorialLinearSolver {
return SerialCombinatorialLinearSolver(solvers.map { lazy { it() } }, stopErrorCode)
}
}
override val name: String by lazy { "SerialCombinatorial(${solvers.joinToString(",") { it.value.name }})" }
override suspend operator fun invoke(model: LinearTriadModelView): Ret {
for (solver in solvers) {
when (val result = solver.value.invoke(model)) {
is Ok -> {
return Ok(result.value)
}
is Failed -> {
if (stopErrorCode.contains(result.error.code)) {
return Failed(result.error.code, result.error.message)
} else {
logger.warn { "Solver ${solver.value.name} failed with error ${result.error.code}: ${result.error.message}" }
}
}
}
}
return Failed(ErrorCode.SolverNotFound, "No solver valid.")
}
override suspend operator fun invoke(model: LinearTriadModelView, solutionAmount: UInt64): Ret>> {
for (solver in solvers) {
when (val result = solver.value.invoke(model, solutionAmount)) {
is Ok -> {
return Ok(result.value)
}
is Failed -> {
if (stopErrorCode.contains(result.error.code)) {
return Failed(result.error.code, result.error.message)
} else {
logger.warn { "Solver ${solver.value.name} failed with error ${result.error.code}: ${result.error.message}" }
}
}
}
}
return Failed(ErrorCode.SolverNotFound, "No solver valid.")
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy