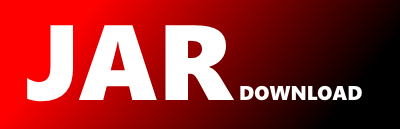
fuookami.ospf.kotlin.utils.physics.quantity.Quantity.kt Maven / Gradle / Ivy
The newest version!
package fuookami.ospf.kotlin.utils.physics.quantity
import java.math.BigDecimal
import java.math.BigInteger
import fuookami.ospf.kotlin.utils.math.*
import fuookami.ospf.kotlin.utils.operator.*
import fuookami.ospf.kotlin.utils.physics.unit.*
data class Quantity(
val value: V,
val unit: PhysicalUnit
)
infix fun Quantity.eq(other: Quantity): Boolean where V : Arithmetic, V : Eq {
return if (this.unit == other.unit) {
this.value eq other.value
} else if (this.unit.quantity == other.unit.quantity) {
TODO("not implemented yet")
} else {
false
}
}
infix fun Quantity.partialOrd(other: Quantity): Order? where V : Arithmetic, V : PartialOrd {
return if (this.unit == other.unit) {
this.value partialOrd other.value
} else if (this.unit.quantity == other.unit.quantity) {
TODO("not implemented yet")
} else {
null
}
}
fun > Quantity.toInt64(): Quantity {
return Quantity(this.value.toInt64(), this.unit)
}
fun > Quantity.toUInt64(): Quantity {
return Quantity(this.value.toUInt64(), this.unit)
}
fun > Quantity.toIntX(): Quantity {
return Quantity(this.value.toIntX(), this.unit)
}
fun > Quantity.toFlt64(): Quantity {
return Quantity(this.value.toFlt64(), this.unit)
}
fun > Quantity.toFltX(): Quantity {
return Quantity(this.value.toFltX(), this.unit)
}
@JvmName("convertQuantityInt64")
fun Quantity.to(unit: PhysicalUnit): Quantity? {
return if (this.unit == unit) {
Quantity(this.value, unit)
} else {
this.unit.to(unit)?.value?.let {
Quantity(it.toInt64() * this.value, unit)
}
}
}
@JvmName("convertQuantityUInt64")
fun Quantity.to(unit: PhysicalUnit): Quantity? {
return if (this.unit == unit) {
Quantity(this.value, unit)
} else {
this.unit.to(unit)?.value?.let {
Quantity(it.toUInt64() * this.value, unit)
}
}
}
fun Quantity.to(unit: PhysicalUnit): Quantity? {
return if (this.unit == unit) {
Quantity(this.value, unit)
} else {
this.unit.to(unit)?.value?.let {
Quantity(it.toIntX() * this.value, unit)
}
}
}
@JvmName("convertQuantityFlt64")
fun Quantity.to(unit: PhysicalUnit): Quantity? {
return if (this.unit == unit) {
Quantity(this.value, unit)
} else {
this.unit.to(unit)?.value?.let {
Quantity(it * this.value, unit)
}
}
}
@JvmName("convertQuantityFltX")
fun Quantity.to(unit: PhysicalUnit): Quantity? {
return if (this.unit == unit) {
Quantity(this.value, unit)
} else {
this.unit.to(unit)?.value?.let {
Quantity(it.toFltX() * this.value, unit)
}
}
}
operator fun > V.times(unit: PhysicalUnit): Quantity {
return Quantity(this, unit)
}
operator fun Int.times(unit: PhysicalUnit): Quantity {
return Quantity(Int64(this.toLong()), unit)
}
operator fun UInt.times(unit: PhysicalUnit): Quantity {
return Quantity(UInt64(this.toULong()), unit)
}
operator fun BigInteger.times(unit: PhysicalUnit): Quantity {
return Quantity(IntX(this), unit)
}
operator fun Double.times(unit: PhysicalUnit): Quantity {
return Quantity(Flt64(this), unit)
}
operator fun BigDecimal.times(unit: PhysicalUnit): Quantity {
return Quantity(FltX(this), unit)
}
@JvmName("plusQuantityInt64")
operator fun Quantity.plus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value + other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value + other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value + other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("plusQuantityUInt64")
operator fun Quantity.plus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value + other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value + other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value + other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("plusQuantityIntX")
operator fun Quantity.plus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value + other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value + other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value + other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("plusQuantityFlt64")
operator fun Quantity.plus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value + other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value + other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value + other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("plusQuantityFltX")
operator fun Quantity.plus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value + other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value + other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value + other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("minusQuantityInt64")
operator fun Quantity.minus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value - other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value - other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value - other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("minusQuantityUInt64")
operator fun Quantity.minus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value - other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value - other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value - other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("minusQuantityIntX")
operator fun Quantity.minus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value - other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value - other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value - other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("minusQuantityFlt64")
operator fun Quantity.minus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value - other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value - other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value - other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
@JvmName("minusQuantityFltX")
operator fun Quantity.minus(other: Quantity): Quantity {
return if (this.unit == other.unit) {
Quantity(this.value - other.value, this.unit)
} else if (this.unit.quantity == other.unit.quantity) {
if (this.unit.scale.value leq other.unit.scale.value) {
Quantity(this.value - other.to(this.unit)!!.value, this.unit)
} else {
Quantity(this.to(other.unit)!!.value - other.value, other.unit)
}
} else {
TODO("not implemented yet")
}
}
operator fun Quantity.times(other: Quantity): Quantity where V : Arithmetic, V : Times {
return if (this.unit.quantity == other.unit.quantity) {
Quantity(this.value * other.value, this.unit * other.unit)
} else {
TODO("not implemented yet")
}
}
operator fun Quantity.div(other: Quantity): Quantity where V : Arithmetic, V : Div {
return if (this.unit.quantity == other.unit.quantity) {
Quantity(this.value / other.value, this.unit / other.unit)
} else {
TODO("not implemented yet")
}
}
operator fun Quantity.unaryMinus(): Quantity where V : Arithmetic, V : Neg {
return Quantity(-this.value, this.unit)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy