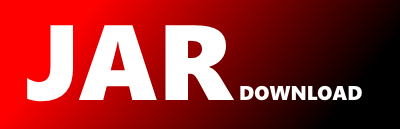
gate.lang.contentDisposition.ContentDisposition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gate Show documentation
Show all versions of gate Show documentation
A multipurpose java library
package gate.lang.contentDisposition;
import gate.error.AppError;
import gate.error.ConversionException;
import java.io.IOException;
import java.io.StringReader;
import java.io.UncheckedIOException;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.text.ParseException;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
public class ContentDisposition
{
private final String value;
private final Map parameters;
public ContentDisposition(String value,
Map parameters)
{
Objects.requireNonNull(parameters);
this.value = value;
this.parameters = parameters;
}
public String getValue()
{
return value;
}
public Map getParameters()
{
return Collections.unmodifiableMap(parameters);
}
@Override
public String toString()
{
StringBuilder string = new StringBuilder();
if (value != null)
string.append(value);
parameters
.entrySet().forEach(e ->
{
try
{
string.append(';').append(e.getKey()).append('=').append(URLEncoder.encode(e.getValue(), "UTF-8"));
} catch (UnsupportedEncodingException ex)
{
throw new UncheckedIOException(ex);
}
});
return string.toString();
}
public static ContentDisposition parse(String string) throws ParseException
{
try ( ContentDispositionParser parser = new ContentDispositionParser(new ContentDispositionScanner(new StringReader(string))))
{
try
{
return parser.parse();
} catch (IOException ex)
{
throw new AppError(ex);
}
}
}
public static ContentDisposition valueOf(String string) throws ConversionException
{
try
{
return parse(string);
} catch (ParseException ex)
{
throw new ConversionException("Invalid content disposition header");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy