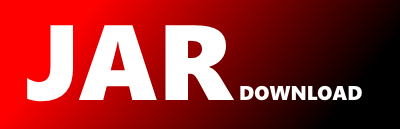
io.github.gnuf0rce.github.model.ReposMapper.kt Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2022 dsstudio Technologies and contributors.
*
* 此源代码的使用受 GNU AFFERO GENERAL PUBLIC LICENSE version 3 许可证的约束, 可以在以下链接找到该许可证.
* Use of this source code is governed by the GNU AGPLv3 license that can be found through the following link.
*
* https://github.com/gnuf0rce/github-helper/blob/master/LICENSE
*/
package io.github.gnuf0rce.github.model
import io.github.gnuf0rce.github.*
import io.github.gnuf0rce.github.entry.*
import io.ktor.http.*
import java.util.*
/**
* [Repositories](https://docs.github.com/en/rest/repos)
*/
public open class ReposMapper(parent: Url, public val owner: String, public val repo: String) :
GitHubMapper(parent = parent, path = "$owner/$repo") {
override val github: GitHubClient = GitHubClient()
// region Repositories
public open suspend fun load(): Repo = get()
public open suspend fun update(context: Temp): Temp = patch(context = context)
public open suspend fun delete(): Unit = delete()
public open suspend fun fixes(open: Boolean): Temp = open(open = open, path = "automated-security-fixes")
public open suspend fun contributors(page: Int, per: Int = 30, anon: Boolean = false): List =
page(page = page, per = per, context = mapOf("anon" to anon), path = "contributors")
public open suspend fun dispatches(context: Temp): Unit = post(context = context, path = "dispatches")
/**
* [list-repository-languages](https://docs.github.com/en/rest/repos/repos#list-repository-languages)
*/
public open suspend fun languages(): Map = get(path = "languages")
public open suspend fun tags(page: Int, per: Int = 30): List = page(page = page, per = per, path = "tags")
public open suspend fun teams(page: Int, per: Int = 30): List = page(page = page, per = per, path = "teams")
public open suspend fun topics(page: Int, per: Int = 30): List? =
get
© 2015 - 2024 Weber Informatics LLC | Privacy Policy