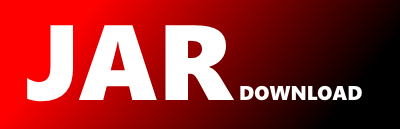
io.github.graphglue.graphql.query.TopLevelQueryProvider.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graphglue-core Show documentation
Show all versions of graphglue-core Show documentation
A library to develop annotation-based code-first GraphQL servers using GraphQL Kotlin, Spring Boot and Neo4j - excluding Spring GraphQL server dependencies
package io.github.graphglue.graphql.query
import com.expediagroup.graphql.generator.annotations.GraphQLIgnore
import com.fasterxml.jackson.databind.ObjectMapper
import graphql.execution.DataFetcherResult
import graphql.schema.DataFetchingEnvironment
import io.github.graphglue.connection.model.Connection
import io.github.graphglue.data.execution.NodeQueryEngine
import io.github.graphglue.data.execution.NodeQueryParser
import io.github.graphglue.data.execution.NodeQueryResult
import io.github.graphglue.data.execution.SearchQueryResult
import io.github.graphglue.definition.NodeDefinition
import io.github.graphglue.graphql.extensions.requiredPermission
import io.github.graphglue.model.Node
import org.springframework.beans.factory.annotation.Autowired
/**
* Provider for top level queries for specific [Node] types
* Used to generate connection like top level queries
*
* @param T the type of node for which to create the query
* @param nodeDefinition the definition of the [Node] type to query for
*/
class TopLevelQueryProvider(private val nodeDefinition: NodeDefinition) {
/**
* Handles the query for the specific [Node] type
*
* @param nodeQueryParser used to parse the query
* @param dataFetchingEnvironment necessary to generate the node query, used for caching
* @param nodeQueryEngine used to execute the query
* @param objectMapper necessary for cursor encoding and decoding
* @return the result with the correct local context
*/
@Suppress("UNCHECKED_CAST")
suspend fun getNodeQuery(
@Autowired @GraphQLIgnore
nodeQueryParser: NodeQueryParser,
dataFetchingEnvironment: DataFetchingEnvironment,
@Autowired @GraphQLIgnore
nodeQueryEngine: NodeQueryEngine,
@Autowired @GraphQLIgnore
objectMapper: ObjectMapper
): DataFetcherResult> {
val nodeQuery = nodeQueryParser.generateManyNodeQuery(
nodeDefinition,
dataFetchingEnvironment,
emptyList(),
dataFetchingEnvironment.requiredPermission
)
val queryResult = nodeQueryEngine.execute(nodeQuery) as NodeQueryResult
return DataFetcherResult.newResult>()
.data(Connection.fromQueryResult(queryResult, objectMapper))
.localContext(nodeQuery)
.build()
}
/**
* Handles the search query for the specific [Node] type
*
* @param nodeQueryParser used to parse the query
* @param dataFetchingEnvironment necessary to generate the node query, used for caching
* @param nodeQueryEngine used to execute the query
* @return the result
*/
@Suppress("UNCHECKED_CAST")
suspend fun getSearchQuery(
@Autowired @GraphQLIgnore
nodeQueryParser: NodeQueryParser,
dataFetchingEnvironment: DataFetchingEnvironment,
@Autowired @GraphQLIgnore
nodeQueryEngine: NodeQueryEngine
): List {
val searchQuery = nodeQueryParser.generateSearchQuery(
nodeDefinition,
dataFetchingEnvironment,
dataFetchingEnvironment.requiredPermission
)
val queryResult = nodeQueryEngine.execute(searchQuery) as SearchQueryResult
return queryResult.nodes
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy