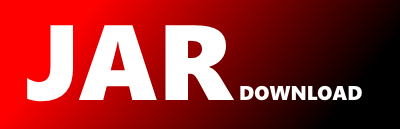
haerzig.core.utils.HttpUtil.kt Maven / Gradle / Ivy
package haerzig.core.utils
import android.content.Context
import android.content.Intent
import android.net.ConnectivityManager
import android.net.NetworkCapabilities
import android.net.Uri
import java.io.BufferedReader
import java.io.InputStreamReader
import java.net.HttpURLConnection
import java.net.URL
object HttpUtil {
private const val GET_URL = "https://www.ecb.europa.eu/stats/eurofxref/eurofxref-daily.xml"
// success
val currentCurrencyRates: String
// print result
get() = try {
val obj = URL(GET_URL)
val con = obj.openConnection() as HttpURLConnection
con.requestMethod = "GET"
con.setRequestProperty("User-Agent", "Mozilla/5.0")
val responseCode = con.responseCode
println("GET Response Code :: $responseCode")
if (responseCode == HttpURLConnection.HTTP_OK) { // success
val `in` = BufferedReader(InputStreamReader(con.inputStream))
var inputLine: String?
val response = StringBuilder()
while (`in`.readLine().also { inputLine = it } != null) {
response.append(inputLine)
}
`in`.close()
// print result
println(response.toString())
response.toString()
} else {
println("GET request not worked")
""
}
} catch (ex: Exception) {
MyLogger.logErrorMessage("HTTPRequestUtil", ex.message, ex)
""
}
fun newEmailIntent(address: String, subject: String?, body: String?, cc: String?): Intent {
val intent = Intent(Intent.ACTION_SEND)
intent.putExtra(Intent.EXTRA_EMAIL, arrayOf(address))
intent.putExtra(Intent.EXTRA_TEXT, body)
intent.putExtra(Intent.EXTRA_SUBJECT, subject)
intent.putExtra(Intent.EXTRA_CC, cc)
intent.type = "message/rfc822"
return intent
}
fun newWebsiteIntent(): Intent {
val i = Intent(Intent.ACTION_VIEW)
i.data = Uri.parse("https://haerzig-software.jimdofree.com")
return i
}
fun newFacebookIntent(page: String): Intent{
return Intent(Intent.ACTION_VIEW, Uri.parse("fb://facewebmodal/f?href=https://www.facebook.com/$page"))
}
fun isNetworkNotAvailable(context: Context): Boolean {
val connectivityManager = context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
val activeNetwork = connectivityManager.activeNetwork
var isConnected = false
if (activeNetwork != null) {
val networkCapabilities = connectivityManager.getNetworkCapabilities(activeNetwork)
if (networkCapabilities != null) {
isConnected = networkCapabilities.hasTransport(NetworkCapabilities.TRANSPORT_CELLULAR) || networkCapabilities.hasTransport(NetworkCapabilities.TRANSPORT_WIFI)
}
}
return !isConnected
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy