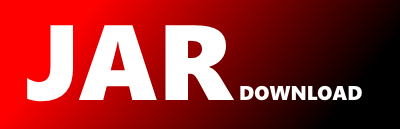
com.baidu.haotianjing.core.client.ClientProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of haotianjing-java-sdk Show documentation
Show all versions of haotianjing-java-sdk Show documentation
Haotianjing SaaS SDK for Java
The newest version!
package com.baidu.haotianjing.core.client;
import com.baidu.haotianjing.core.auth.Credentials;
import com.baidu.haotianjing.core.auth.MD5SignerImpl;
import com.baidu.haotianjing.core.auth.Signer;
import com.baidu.haotianjing.core.http.HttpClientConfig;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
public class ClientProfile {
/**
* 指定要使用的服务名
* risk - 业务风控服务
* device - 设备风控服务
* knowledge - 风控知识库服务
*/
private String serviceCode;
/**
* 指定要使用的服务所在就近区域
* 例如:
* guangzhou - 只调用广州集群服务
* guangzhou,suzhou - 优先调用广州集群服务,广州不可用时,自动切换到苏州集群
* guangzhou,suzhou,beijing - 优先广州集群,其次苏州,再次北京
*/
private String regionCodes;
/**
* 是否开启服务故障后自动切换到其他地域,默认开启
*/
private Boolean failOverOn = Boolean.TRUE;
/**
* 默认使用MD5签名器
*/
private Signer signer = MD5SignerImpl.INSTANCE;
private Credentials credentials;
private HttpClientConfig httpClientConfig;
private int maxRetryCount = 1;
/**
* 昊天镜服务编码,标识访问哪一个服务产品
* 示例:
* risk - 使用昊天镜业务风控服务
* device - 使用昊天镜设备风控服务
* knowledge - 使用昊天镜知识库服务
*/
public ClientProfile serviceCode(String serviceCode) {
this.serviceCode = serviceCode;
return this;
}
/**
* 昊天镜服务所在地域,可填写多个,英文逗号分割,优先采用第一个地域集群
* 示例:
* guangzhou - 使用昊天镜服务广州集群
* guangzhou,suzhou - 优先使用昊天镜服务广州集群,若故障,自动切到苏州集群
* guangzhou,suzhou,bejing - 优先使用昊天镜服务广州集群,其次使用苏州集群,兜底使用北京集群
*/
public ClientProfile regionCodes(String regionCodes) {
this.regionCodes = regionCodes;
return this;
}
/**
* 访问昊天镜服务的凭证信息
* accountId:可登录百度云->鼠标移到右上角头像->用户中心->点击进入,可找到账户ID。
* appkey/seckey:可登录百度云->服务接入->应用管理->点击管理,可找到自己的认证信息。
*/
public ClientProfile credentials(Credentials credentials) {
this.credentials = credentials;
return this;
}
/**
* 访问昊天镜服务的凭证信息
* accountId:可登录百度云->鼠标移到右上角头像->用户中心->点击进入,可找到账户ID。
* appkey/seckey:可登录百度云->服务接入->应用管理->点击管理,可找到自己的认证信息。
*/
public ClientProfile credentials(String accountId, String appkey, String seckey) {
credentials(new Credentials(accountId, appkey, seckey));
return this;
}
/**
* HTTP客户端相关配置
*/
public ClientProfile httpClientConfig(HttpClientConfig httpClientConfig) {
this.httpClientConfig = httpClientConfig;
return this;
}
/**
* 最大重试次数,默认重试1次,如果小于等于0,则不重试
*/
public ClientProfile maxRetryCount(int maxRetryCount) {
this.maxRetryCount = maxRetryCount;
return this;
}
public int maxRetryCount() {
return maxRetryCount;
}
/**
* 设置不重试
*/
public ClientProfile noRetry() {
return maxRetryCount(0);
}
/**
* 是否开启故障服务集群自动切换,默认开启
*/
public ClientProfile failOverOn(Boolean failOverOn) {
this.failOverOn = failOverOn;
return this;
}
/**
* 所有参数使用默认配置
*/
public static ClientProfile defaultProfile(Credentials credentials) {
return new ClientProfile()
.credentials(credentials)
.httpClientConfig(HttpClientConfig.defaultConfig());
}
/**
* 所有参数使用默认配置
*/
public static ClientProfile defaultProfile(String accountId, String appkey, String seckey) {
return defaultProfile(new Credentials(accountId, appkey, seckey));
}
public String getRegionCodes() {
return regionCodes;
}
public void setRegionCodes(String regionCodes) {
this.regionCodes = regionCodes;
}
public Credentials getCredentials() {
return credentials;
}
public void setCredentials(Credentials credentials) {
this.credentials = credentials;
}
public HttpClientConfig getHttpClientConfig() {
return httpClientConfig;
}
public void setHttpClientConfig(HttpClientConfig httpClientConfig) {
this.httpClientConfig = httpClientConfig;
}
public int getMaxRetryCount() {
return maxRetryCount;
}
public void setMaxRetryCount(int maxRetryCount) {
this.maxRetryCount = maxRetryCount;
}
public Boolean getFailOverOn() {
return failOverOn;
}
public String getServiceCode() {
return serviceCode;
}
public Signer getSigner() {
return signer;
}
@Override
public String toString() {
return ReflectionToStringBuilder.
toString(this, ToStringStyle.MULTI_LINE_STYLE, true, true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy