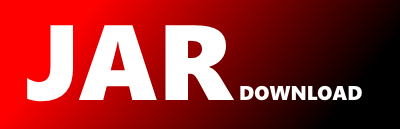
com.baidu.haotianjing.core.endpoint.EndpointConfigLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of haotianjing-java-sdk Show documentation
Show all versions of haotianjing-java-sdk Show documentation
Haotianjing SaaS SDK for Java
The newest version!
package com.baidu.haotianjing.core.endpoint;
import com.baidu.haotianjing.core.exception.RiskSdkException;
import com.baidu.haotianjing.core.utils.JacksonUtils;
import org.apache.commons.lang3.StringUtils;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class EndpointConfigLoader {
public static final String ENDPOINT_CONFIG_FILE = "haotianjing_endpoints.json";
private static final Map>> ENDPOINT_MAP;
static {
ENDPOINT_MAP = loadEndpoints();
}
public static Map>> loadEndpoints() throws RiskSdkException {
InputStream inputStream;
try {
inputStream = EndpointConfigLoader.class.getClassLoader().getResourceAsStream(ENDPOINT_CONFIG_FILE);
} catch (Exception e) {
throw new RiskSdkException("get endpoint config file exp. file: " + ENDPOINT_CONFIG_FILE, e);
}
return load(inputStream);
}
public static Map>> load(InputStream inputStream) throws RiskSdkException {
String content = readAsString(inputStream);
return parseContent(content);
}
public static Map>> parseContent(String content) throws RiskSdkException {
if (StringUtils.isBlank(content)) {
throw new RiskSdkException("endpoint config file content is empty. file: " + ENDPOINT_CONFIG_FILE);
}
Map>> endpointMap = new HashMap<>();
Map configMap;
try {
// configMap = JacksonUtils.toObject(content, Map.class, Map.class, List.class);
configMap = JacksonUtils.toObject(content, Map.class);
} catch (Exception e) {
throw new RiskSdkException("endpoint config file not json. file: " + ENDPOINT_CONFIG_FILE);
}
if (null == configMap || configMap.size() < 1) {
throw new RiskSdkException("endpoint config file is empty. file: " + ENDPOINT_CONFIG_FILE);
}
for (Map.Entry serviceEntry : configMap.entrySet()) {
String serviceCode = serviceEntry.getKey();
if (!endpointMap.containsKey(serviceCode)) {
endpointMap.put(serviceCode, new HashMap>());
}
Map regionConfigMap = (HashMap) serviceEntry.getValue();
if (null == regionConfigMap || regionConfigMap.size() < 1) {
continue;
}
for (Map.Entry regionEntry : regionConfigMap.entrySet()) {
String regionCode = regionEntry.getKey();
List domainList = (ArrayList) regionEntry.getValue();
if (null == domainList || domainList.size() < 1) {
continue;
}
List endpointList = new ArrayList<>();
for (String domain : domainList) {
Endpoint endpoint = new Endpoint();
endpoint.setServiceCode(serviceCode);
endpoint.setRegionCode(regionCode);
endpoint.setDomain(domain);
endpointList.add(endpoint);
}
endpointMap.get(serviceCode).put(regionCode, endpointList);
}
}
if (endpointMap.size() < 1) {
throw new RiskSdkException("endpoint config file not correct. file: " + ENDPOINT_CONFIG_FILE);
}
return endpointMap;
}
private static String readAsString(InputStream inputStream) throws RiskSdkException {
try {
try (InputStreamReader reader = new InputStreamReader(inputStream, StandardCharsets.UTF_8)) {
StringBuilder sb = new StringBuilder();
char[] buffer = new char[1024 * 4];
int n;
while ((n = reader.read(buffer)) != -1) {
sb.append(buffer, 0, n);
}
return sb.toString();
}
} catch (IOException e) {
throw new RiskSdkException("read endpoint config file exp. file: " + ENDPOINT_CONFIG_FILE, e);
}
}
public static Map>> getEndpointMap() {
return ENDPOINT_MAP;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy