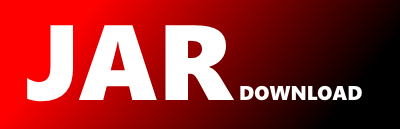
com.baidu.haotianjing.core.endpoint.EndpointResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of haotianjing-java-sdk Show documentation
Show all versions of haotianjing-java-sdk Show documentation
Haotianjing SaaS SDK for Java
The newest version!
package com.baidu.haotianjing.core.endpoint;
import com.baidu.haotianjing.core.client.Client;
import com.baidu.haotianjing.core.client.ClientProfile;
import com.baidu.haotianjing.core.exception.RiskSdkException;
import com.baidu.haotianjing.core.request.BaseRequest;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import static java.lang.Boolean.FALSE;
import static java.lang.Boolean.TRUE;
public abstract class EndpointResolver {
private String serviceCode;
private String regionCodes;
private String[] regionArr;
private ScheduledExecutorService scheduledExecutorService;
private final Client client;
public EndpointResolver(ClientProfile clientProfile, Client client) {
this.serviceCode = clientProfile.getServiceCode();
this.regionCodes = clientProfile.getRegionCodes();
this.regionArr = regionCodes.split(",");
this.scheduledExecutorService = new ScheduledThreadPoolExecutor(1);
this.client = client;
if (this.client.endpointRunningContext().getFailOverOn()) {
scheduledExecutorService.scheduleAtFixedRate(
new EndpointDetector(), 0, 10, TimeUnit.SECONDS);
}
}
/**
* 探测服务集群可用性,探测规则:
* 连续二次(每次间隔1s)均探测成功时,认为该域名可用;
*/
public Boolean detect(String domain) throws RiskSdkException {
T t = buildDetectRequest(domain);
int succCnt = 0;
for (int cnt = 1; cnt <= 2; cnt++) {
try {
getClient().execute(t);
succCnt++;
} catch (RiskSdkException e) {
break;
}
try {
Thread.sleep(1000L);
} catch (InterruptedException e) {
// just do nothing
}
}
if (succCnt == 2) {
return Boolean.TRUE;
} else {
return Boolean.FALSE;
}
}
public abstract T buildDetectRequest(String domain) throws RiskSdkException;
public String getServiceCode() {
return serviceCode;
}
public String getRegionCodes() {
return regionCodes;
}
/**
* 探测服务集群可用性,探测规则:
* 连续二次(每次间隔1s)探测成功时,认为该域名可用;
* 探测失败时,切到下一个备选域名,继续上述探测;
* 所有域名均探测失败时,采用第一个域名
*/
private class EndpointDetector extends Thread {
@Override
public void run() {
EndpointRunningContext endpointRunningContext = client.endpointRunningContext();
if (!endpointRunningContext.detectStatus()) {
return;
}
Map> regionMap = EndpointConfigLoader.getEndpointMap().get(serviceCode);
int seq = 0;
Boolean detectSucc = FALSE;
for (String region : regionArr) {
List endpointList = regionMap.get(region);
if (null != endpointList && endpointList.size() > 0) {
for (Endpoint endpoint : endpointList) {
String reginCode = endpoint.getRegionCode();
String domain = endpoint.getDomain();
if (detect(domain)) {
detectSucc = TRUE;
endpointRunningContext.freshPrimaryDomain(domain);
endpointRunningContext.setPrimaryRegionCode(reginCode);
endpointRunningContext.setLastDetectTime(new Date());
endpointRunningContext.getTotalDetectCount().incrementAndGet();
if (seq == 0) {
endpointRunningContext.noDetect();
} else {
endpointRunningContext.mustDetect("1");
}
break;
}
}
}
if (detectSucc) {
break;
}
seq++;
}
if (!detectSucc) {
List firstEndpointList = regionMap.get(regionArr[0]);
if (null != firstEndpointList && firstEndpointList.size() > 0) {
endpointRunningContext.freshPrimaryDomain(firstEndpointList.get(0).getDomain());
endpointRunningContext.setPrimaryRegionCode(firstEndpointList.get(0).getRegionCode());
}
}
}
}
public Client getClient() {
return client;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy