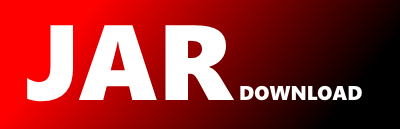
com.baidu.haotianjing.core.utils.JacksonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of haotianjing-java-sdk Show documentation
Show all versions of haotianjing-java-sdk Show documentation
Haotianjing SaaS SDK for Java
The newest version!
package com.baidu.haotianjing.core.utils;
import com.baidu.haotianjing.core.exception.RiskSdkException;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import java.io.IOException;
import java.io.StringWriter;
import java.text.SimpleDateFormat;
public class JacksonUtils {
private static JsonFactory jsonFactory = new JsonFactory();
private static ObjectMapper objectMapper = createObjectMapper();
private static final String DATE_PATTERN = "yyyyMMddHHmmss";
/**
* 创建一个自定义的JSON ObjectMapper
*/
private static ObjectMapper createObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setDateFormat(new SimpleDateFormat(DATE_PATTERN));
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(SerializationFeature.INDENT_OUTPUT, false);
objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_CONTROL_CHARS, true);
objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
objectMapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
objectMapper.configure(JsonParser.Feature.ALLOW_BACKSLASH_ESCAPING_ANY_CHARACTER, true);
return objectMapper;
}
/**
* 将对象转换为JSON字符串
*/
public static String toJson(T value) throws RiskSdkException {
if (value == null) {
return null;
}
StringWriter sw = new StringWriter();
JsonGenerator gen = null;
try {
gen = jsonFactory.createGenerator(sw);
objectMapper.writeValue(gen, value);
return sw.toString();
} catch (IOException e) {
throw new RiskSdkException("object to json exp. object: " + value, e);
} finally {
if (gen != null) {
try {
gen.close();
} catch (IOException e) {
/** ignore */
}
}
}
}
/**
* 将JSON字符串转换为指定对象
*/
@SuppressWarnings("unchecked")
public static T toObject(String jsonString, Class valueType, Class>... itemTypes) throws Exception {
if (null == jsonString || jsonString.length() < 1) {
return null;
}
// 返回值加强制转换是因为scmpf编译机JDK版本jdk1.6.0_20的BUG,编译时会出错,在jdk1.6.0_25之后已修复
try {
if (itemTypes.length == 0) {
// 非集合类型
return (T) objectMapper.readValue(jsonString, valueType);
} else {
// 集合类型, 如List,Map
JavaType javaType = objectMapper.getTypeFactory()
.constructParametrizedType(valueType, valueType, itemTypes);
return (T) objectMapper.readValue(jsonString, javaType);
}
} catch (Exception e) {
throw new RiskSdkException("json to object exp. json: " + jsonString, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy