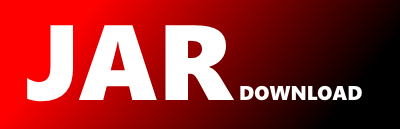
io.github.heykb.sqlhelper.spring.PropertyLogicDeleteInfoHandler Maven / Gradle / Ivy
package io.github.heykb.sqlhelper.spring;
import io.github.heykb.sqlhelper.handler.abstractor.LogicDeleteInfoHandler;
import io.github.heykb.sqlhelper.utils.CommonUtils;
import org.springframework.util.AntPathMatcher;
import java.util.List;
public class PropertyLogicDeleteInfoHandler extends LogicDeleteInfoHandler {
static final AntPathMatcher antPathMatcher = new AntPathMatcher(".");
private String deleteSqlDemo = "UPDATE a SET is_deleted = 'Y'";
private String columnName = "is_deleted";
private String notDeletedValue = "'N'";
private List mapperIds;
private List tables;
private List ignoreMapperIds;
private List ignoreTables;
/**
* Gets ignore mapper ids.
*
* @return the ignore mapper ids
*/
public List getIgnoreMapperIds() {
return ignoreMapperIds;
}
/**
* Sets ignore mapper ids.
*
* @param ignoreMapperIds the ignore mapper ids
*/
public void setIgnoreMapperIds(List ignoreMapperIds) {
this.ignoreMapperIds = ignoreMapperIds;
}
/**
* Gets ignore tables.
*
* @return the ignore tables
*/
public List getIgnoreTables() {
return ignoreTables;
}
/**
* Sets ignore tables.
*
* @param ignoreTables the ignore tables
*/
public void setIgnoreTables(List ignoreTables) {
this.ignoreTables = ignoreTables;
}
public List getMapperIds() {
return mapperIds;
}
public void setMapperIds(List mapperIds) {
this.mapperIds = mapperIds;
}
public List getTables() {
return tables;
}
public void setTables(List tables) {
this.tables = tables;
}
@Override
public String getDeleteSqlDemo() {
return this.deleteSqlDemo;
}
@Override
public String getNotDeletedValue() {
return notDeletedValue;
}
/**
* Sets not deleted value.
*
* @param notDeletedValue the not deleted value
*/
public void setNotDeletedValue(String notDeletedValue) {
this.notDeletedValue = notDeletedValue;
}
@Override
public String getColumnName() {
return this.columnName;
}
public void setDeleteSqlDemo(String deleteSqlDemo) {
this.deleteSqlDemo = deleteSqlDemo;
}
/**
* Sets column name.
*
* @param columnName the column name
*/
public void setColumnName(String columnName) {
this.columnName = columnName;
}
@Override
public boolean checkTableName(String tableName) {
if(tables!=null && !wildcardMatchAny(this.tables,tableName)){
return false;
}
if(ignoreTables!=null && wildcardMatchAny(ignoreTables,tableName)){
return false;
}
return true;
}
@Override
public boolean checkMapperId(String mapperId) {
if(mapperIds!=null && !pathMatchAny(this.mapperIds,mapperId)){
return false;
}
if(ignoreMapperIds!=null && pathMatchAny(ignoreMapperIds,mapperId)){
return false;
}
return true;
}
boolean wildcardMatchAny(List patterns,String target){
for(String pattern:patterns){
if(CommonUtils.wildcardMatch(pattern,target)){
return true;
}
}
return false;
}
boolean pathMatchAny(List patterns,String target){
for(String pattern:patterns){
if(antPathMatcher.match(pattern,target)){
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy