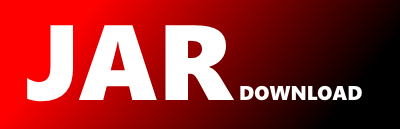
commonMain.com.hoc081098.channeleventbus.ChannelEvent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of channel-event-bus Show documentation
Show all versions of channel-event-bus Show documentation
Multi-keys, multi-producers, single-consumer event bus backed by Kotlinx Coroutines Channels
The newest version!
package com.hoc081098.channeleventbus
import kotlin.jvm.JvmField
import kotlin.jvm.JvmSynthetic
import kotlin.reflect.KClass
import kotlin.reflect.cast
import kotlinx.coroutines.channels.Channel
/**
* Represents an event that can be sent to a [ChannelEventBus].
*/
public interface ChannelEvent> {
/**
* The key to identify a bus for this type of events.
*/
public val key: Key
/**
* The [ChannelEvent.Key] to identify a bus for this type of events.
*
* @param T the type of events.
* @param eventClass the [KClass] of events.
* @param capacity the [ChannelEventBusCapacity] of the [Channel] associated with this key.
* Default is [ChannelEventBusCapacity.UNLIMITED].
*
* @see [ChannelEventBusCapacity]
*/
public open class Key>(
@JvmField
internal val eventClass: KClass,
@JvmField
internal val capacity: ChannelEventBusCapacity = ChannelEventBusCapacity.UNLIMITED,
) {
final override fun equals(other: Any?): Boolean {
if (this === other) return true
if (other !is Key<*>) return false
return eventClass == other.eventClass && capacity == other.capacity
}
final override fun hashCode(): Int = 31 * eventClass.hashCode() + capacity.hashCode()
final override fun toString(): String = "ChannelEvent.Key(${eventClass.simpleName}, $capacity)"
@JvmSynthetic
internal inline fun cast(it: Any): T = eventClass.cast(it)
@JvmSynthetic
internal inline fun createChannel(): Channel = Channel(capacity.asInt())
}
}
/**
* Alias for [ChannelEvent.Key].
* @see [ChannelEvent.Key]
*/
public typealias ChannelEventKey = ChannelEvent.Key
/**
* Retrieve the [ChannelEvent.Key] for [T].
*
* You should cache the result of this function to avoid unnecessary object creation, for example:
*
* ```kotlin
* @JvmField
* val awesomeEventKey = channelEventKeyOf()
* bus.receiveAsFlow(awesomeEventKey).collect { e: AwesomeEvent -> println(e) }
* ```
*/
public inline fun > channelEventKeyOf(
capacity: ChannelEventBusCapacity = ChannelEventBusCapacity.UNLIMITED,
): ChannelEventKey = ChannelEventKey(T::class, capacity)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy