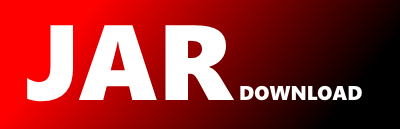
org.jdesktop.swingx.demos.table.CustomColumnFactory Maven / Gradle / Ivy
Show all versions of swingset3-demos Show documentation
/*
* Created on 29.02.2008
*
*/
package org.jdesktop.swingx.demos.table;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import javax.swing.table.TableModel;
import org.jdesktop.swingx.JXTable;
import org.jdesktop.swingx.decorator.Highlighter;
import org.jdesktop.swingx.renderer.ComponentProvider;
import org.jdesktop.swingx.renderer.DefaultTableRenderer;
import org.jdesktop.swingx.table.ColumnFactory;
import org.jdesktop.swingx.table.TableColumnExt;
// JXTable column properties
/**
* A ColumnFactory is used by JXTable to create and configure all columns.
* It can be set per-application (before creating an JXTable) or per-table
* (before setting the model).
*
* Additional features:
*
* - reads column titles from Application context provided ResourceManager
*
- supports list of identifiers to exclude from column creation
*
- supports list of identifiers to hide on column configuration
*
- supports pluggable maximal rowCount to measure
*
- supports per-column componentProviders
*
- supports per-column highlighters
*
- supports per-column sizing hints by prototypes
*
*/
//
public class CustomColumnFactory extends ColumnFactory {
@SuppressWarnings("unused")
private static final Logger LOG = Logger.getLogger(CustomColumnFactory.class.getName());
/** base class for resource lookup. */
private Class> baseClass;
/**
* {@inheritDoc}
* Overridden to not create columns for the model columns which are
* listed in the excludes.
*/
@Override
public TableColumnExt createAndConfigureTableColumn(TableModel model, int modelIndex) {
if (getExcludeNames().contains(model.getColumnName(modelIndex))) {
return null;
}
return super.createAndConfigureTableColumn(model, modelIndex);
}
// JXTable column properties
/**
* {@inheritDoc}
*
* Overridden to set the column's identifier, lookup the title
*/
@Override
public void configureTableColumn(TableModel model, TableColumnExt columnExt) {
super.configureTableColumn(model, columnExt);
columnExt.setIdentifier(model.getColumnName(columnExt.getModelIndex()));
configureTitle(columnExt);
ComponentProvider> provider = getComponentProvider(columnExt.getIdentifier());
if (provider != null) {
columnExt.setCellRenderer(new DefaultTableRenderer(provider));
}
Highlighter highlighter = getHighlighter(columnExt.getIdentifier());
if (highlighter != null) {
columnExt.setHighlighters(highlighter);
}
columnExt.setComparator(getComparator(columnExt.getIdentifier()));
columnExt.setPrototypeValue(getPrototypeValue(columnExt.getIdentifier()));
if (getHiddenNames().contains(columnExt.getIdentifier())) {
columnExt.setVisible(false);
}
}
//
/**
* Sets the title of view column
* @param columnExt TableColumn extension for enhanced view column configuration
*/
protected void configureTitle(TableColumnExt columnExt) {
columnExt.setTitle(OscarTableModel.columnIds[columnExt.getModelIndex()]);
}
/**
* {@inheritDoc}
* Overridden to check if the wants to limit the count of rows when
* measuring.
*/
@Override
protected int getRowCount(JXTable table) {
if (table.getClientProperty("ColumnFactory.maxRowCount") instanceof Integer) {
return Math.min(table.getRowCount(), (Integer) table.getClientProperty("ColumnFactory.maxRowCount"));
}
return super.getRowCount(table);
}
//------------------------ manage the column properties (by identifier)
/**
*
* @param names the identifiers to exclude.
*/
public void addExcludeNames(Object... names) {
for (Object object : names) {
getExcludeNames().add(object);
}
}
/**
*
* @param names the identifiers to exclude.
*/
public void addHiddenNames(Object... names) {
for (Object object : names) {
getHiddenNames().add(object);
}
}
/**
* set Base Class
* @param baseClass the Base Class
*/
public void setBaseClass(Class> baseClass) {
this.baseClass = baseClass;
}
/**
* add Component Provider
* @param id with which the provider is to be associated
* @param provider ComponentProvider to add
*/
public void addComponentProvider(Object id, ComponentProvider> provider) {
getComponentProviders().put(id, provider);
}
/**
* add Prototype Value
* @param id with which the prototype is to be associated
* @param prototype value Object to add
*/
public void addPrototypeValue(Object id, Object prototype) {
getPrototypeValues().put(id, prototype);
}
/**
* add Highlighter Object
* @param id with which the highlighter is to be associated
* @param hl Highlighter to add
*/
public void addHighlighter(Object id, Highlighter hl) {
getHighlighters().put(id, hl);
}
/**
* add Comparator Object
* @param id with which the comparator is to be associated
* @param comparator Comparator to add
*/
public void addComparator(Object id, Comparator> comparator) {
getComparators().put(id, comparator);
}
private List