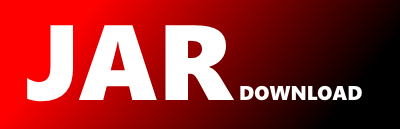
io.github.honhimw.ms.api.reactive.ReactiveDocuments Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.api.reactive;
import io.github.honhimw.ms.Experimental;
import io.github.honhimw.ms.json.TypeRef;
import io.github.honhimw.ms.model.*;
import io.github.honhimw.ms.api.annotation.Operation;
import jakarta.annotation.Nullable;
import reactor.core.publisher.Mono;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
/**
* Documents are objects composed of fields that can store any type of data.
* Each field contains an attribute and its associated value.
* Documents are stored inside indexes.
* Learn more about documents.
*
* @author hon_him
* @since 2023-12-31
*/
public interface ReactiveDocuments {
/**
* Get documents by batch.
*
* @param offset default 0
* @param limit default 20
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
default Mono>> list(@Nullable Integer offset, @Nullable Integer limit) {
return list(request -> {
request.setOffset(offset);
request.setLimit(limit);
});
}
/**
* Get documents by batch.
*
* @param page page request builder
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
default Mono>> list(Consumer page) {
GetDocumentRequest pageRequest = new GetDocumentRequest();
page.accept(pageRequest);
return list(pageRequest);
}
/**
* Get documents by batch.
*
* @param page page request
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
Mono>> list(GetDocumentRequest page);
/**
* Get documents by batch with type.
*
* @param offset default 0
* @param limit default 20
* @param typeRef type
* @param document type
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
Mono> list(@Nullable Integer offset, @Nullable Integer limit, TypeRef typeRef);
/**
* Get documents by batch with type.
*
* @param offset default 0
* @param limit default 20
* @param type type
* @param document type
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
default Mono> list(@Nullable Integer offset, @Nullable Integer limit, Class type) {
return list(offset, limit, TypeRef.of(type));
}
/**
* Get documents by batch with type.
*
* @param page page request
* @param typeRef type
* @param document type
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
Mono> list(GetDocumentRequest page, TypeRef typeRef);
/**
* Get documents by batch with type.
*
* @param page page request builder
* @param typeRef type
* @param document type
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
default Mono> list(Consumer page, TypeRef typeRef) {
GetDocumentRequest pageRequest = new GetDocumentRequest();
page.accept(pageRequest);
return list(pageRequest, typeRef);
}
/**
* Get documents by batch with type.
*
* @param page page request builder
* @param type type
* @param document type
* @return a list of documents
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents")
default Mono> list(Consumer page, Class type) {
return list(page, TypeRef.of(type));
}
/**
* Add a list of documents or replace them if they already exist.
*
* If you send an already existing document (same id)
* the whole existing document will be overwritten by the new document.
* Fields previously in the document not present in the new document are removed.
*
* For a partial update of the document see Add or update documents route.
*
* - info If the provided index does not exist, it will be created.
* - _geo is an object made of lat and lng field.
* - Use the reserved _vectors arrays of floats to add embeddings to a document. _vectors is an array of floats or multiple arrays of floats in an outer array.
*
*
* @param json json formatted array
* @return save task
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents")
Mono save(String json);
/**
* Save one document
*
* @param one document
* @return save task
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents")
default Mono save(Object one) {
return save(Collections.singleton(one));
}
/**
* Save a list of documents
*
* @param collection documents
* @return save task
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents")
Mono save(Collection> collection);
/**
* Save one vectorized document
*
* @param one vectorized document
* @return save task
*/
@Experimental(features = Experimental.Features.VECTOR_SEARCH)
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents")
default Mono saveVectorized(VectorizedDocument one) {
return save(Collections.singleton(one));
}
/**
* Save a list of vectorized documents
*
* @param collection vectorized documents
* @return save task
*/
@Experimental(features = Experimental.Features.VECTOR_SEARCH)
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents")
Mono saveVectorized(Collection collection);
/**
* Add a list of documents or update them if they already exist.
*
* If you send an already existing document (same id)
* the old document will be only partially updated according to the fields of the new document.
* Thus, any fields not present in the new document are kept and remained unchanged.
*
* To completely overwrite a document, see Add or replace documents route.
*
* - info If the provided index does not exist, it will be created.
* - info Use the reserved _geo object to add geo coordinates to a document. _geo is an object made of lat and lng field.
* - Use the reserved _vectors arrays of floats to add embeddings to a document. _vectors is an array of floats or multiple arrays of floats in an outer array.
*
*
* @param json json formatted array
* @return update task
*/
@Operation(method = "PUT", paths = "/indexes/{indexUid}/documents")
Mono update(String json);
/**
* Add one document or update it if it already exists.
*
* @param one document
* @return update task
*/
@Operation(method = "PUT", paths = "/indexes/{indexUid}/documents")
default Mono update(Object one) {
return update(Collections.singleton(one));
}
/**
* Add a list of documents or update them if they already exist.
*
* @param collection documents
* @return update task
*/
@Operation(method = "PUT", paths = "/indexes/{indexUid}/documents")
Mono update(Collection> collection);
/**
* You can edit documents by executing a Rhai function on all the documents of your database or a subset of them that you can select by a Meilisearch filter.
*
* @param edit edit request
* @return edit task
*/
@Experimental(features = Experimental.Features.EDIT_DOCUMENTS_BY_FUNCTION)
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/edit")
Mono edit(EditRequest edit);
/**
* Delete all documents in the specified index.
*
* @return delete task
*/
@Operation(method = "DELETE", paths = "/indexes/{indexUid}/documents")
Mono deleteAll();
/**
* Get documents by batch.
*
* @param fetch fetch request
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
Mono>> batchGet(BatchGetDocumentsRequest fetch);
/**
* Get documents by batch.
*
* @param builder fetch request builder
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
default Mono>> batchGet(Consumer builder) {
BatchGetDocumentsRequest.Builder _builder = BatchGetDocumentsRequest.builder();
builder.accept(_builder);
return batchGet(_builder.build());
}
/**
* Get documents by batch.
*
* @param fetch fetch request
* @param typeRef type reference
* @param document type
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
Mono> batchGet(BatchGetDocumentsRequest fetch, TypeRef typeRef);
/**
* Get documents by batch.
*
* @param builder fetch request builder
* @param typeRef type reference
* @param document type
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
default Mono> batchGet(Consumer builder, TypeRef typeRef) {
BatchGetDocumentsRequest.Builder _builder = BatchGetDocumentsRequest.builder();
builder.accept(_builder);
return batchGet(_builder.build(), typeRef);
}
/**
* Get documents by batch.
*
* @param fetch fetch request
* @param type type
* @param document type
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
default Mono> batchGet(BatchGetDocumentsRequest fetch, Class type) {
return batchGet(fetch, TypeRef.of(type));
}
/**
* Get documents by batch.
*
* @param builder fetch request builder
* @param type type
* @param document type
* @return a list of documents
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/fetch")
default Mono> batchGet(Consumer builder, Class type) {
BatchGetDocumentsRequest.Builder _builder = BatchGetDocumentsRequest.builder();
builder.accept(_builder);
return batchGet(_builder.build(), TypeRef.of(type));
}
/**
* Delete a set of documents based on an array of document ids.
*
* @param ids An array of numbers containing the unique ids of the documents to be deleted.
* @return delete task
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/delete-batch")
Mono batchDelete(List ids);
/**
* Delete a set of documents based on a filter.
*
* @param filter A filter expression written as a string or array of array of strings for the documents to be deleted.
* @return delete task
*/
@Operation(method = "POST", paths = "/indexes/{indexUid}/documents/delete")
Mono delete(FilterableAttributesRequest filter);
/**
* Get one document using its unique id.
*
* @param id Document id of the requested document
* @param fields Comma-separated list of fields to display for an API resource.
* By default it contains all fields of an API resource.
* Default *.
* @return one document
*/
@Operation(method = "GET", paths = "/indexes/{indexUid}/documents/{documentId}")
Mono
© 2015 - 2025 Weber Informatics LLC | Privacy Policy