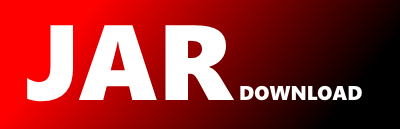
io.github.honhimw.ms.api.reactive.ReactiveTasks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.api.reactive;
import io.github.honhimw.ms.model.*;
import io.github.honhimw.ms.api.annotation.Operation;
import reactor.core.publisher.Mono;
import java.time.Duration;
import java.util.function.Consumer;
/**
* The tasks route gives information about the progress of the asynchronous operations.
*
* @author hon_him
* @since 2024-01-02
*/
public interface ReactiveTasks {
/**
* Get all tasks
*
* @param request GetTasksRequest
* @return paginated result
*/
@Operation(method = "GET", paths = "/tasks")
Mono> list(GetTasksRequest request);
/**
* Get all tasks
*
* @param builder request builder
* @return paginated result
*/
@Operation(method = "GET", paths = "/tasks")
default Mono> list(Consumer builder) {
GetTasksRequest.Builder _builder = GetTasksRequest.builder();
builder.accept(_builder);
return list(_builder.build());
}
/**
* Delete finished tasks
*
* @param request GetTasksRequest
* @return delete task
*/
@Operation(method = "DELETE", paths = "/tasks")
Mono delete(GetTasksRequest request);
/**
* Delete finished tasks
*
* @param builder request builder
* @return delete task
*/
@Operation(method = "DELETE", paths = "/tasks")
default Mono delete(Consumer builder) {
GetTasksRequest.Builder _builder = GetTasksRequest.builder();
builder.accept(_builder);
return delete(_builder.build());
}
/**
* Get a single task.
*
* @param uid uid of the requested task
* @return the requested task
*/
@Operation(method = "GET", paths = "/tasks/{taskUid}")
Mono get(Integer uid);
/**
* Cancel any number of enqueued or processing tasks based on their uid, status, type, indexUid,
* or the date at which they were enqueued, processed, or completed.
* Task cancelation is an atomic transaction: either all tasks are successfully canceled or none are.
*
* @param request A valid uids, statuses, types, indexUids, or date(beforeXAt or afterXAt) parameter is required.
* @return cancel task
*/
@Operation(method = "POST", paths = "/tasks/cancel")
Mono cancel(CancelTasksRequest request);
/**
* Cancel any number of enqueued or processing tasks based on their uid, status, type, indexUid,
* or the date at which they were enqueued, processed, or completed.
* Task cancelation is an atomic transaction: either all tasks are successfully canceled or none are.
*
* @param builder request builder
* @return cancel task
*/
@Operation(method = "POST", paths = "/tasks/cancel")
default Mono cancel(Consumer builder) {
CancelTasksRequest.Builder _builder = CancelTasksRequest.builder();
builder.accept(_builder);
return cancel(_builder.build());
}
/**
* Wait for task to complete, default 100 attempts with 50ms delay.
*
* @param uid task uid
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
Mono await(int uid);
/**
* Wait for task to complete
* @param uid task uid
* @param maxAttempts max attempts
* @param fixedDelay fixed delay
* @param maxDuration max duration
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
Mono await(int uid, int maxAttempts, Duration fixedDelay, Duration maxDuration);
/**
* Wait for task to complete
*
* @param uid task uid
* @param maxAttempts max attempts
* @param fixedDelay fixed delay
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
default Mono await(int uid, int maxAttempts, Duration fixedDelay) {
return await(uid, maxAttempts, fixedDelay, Duration.ofSeconds(10));
}
/**
* Wait for task to complete, default 100 attempts with 50ms delay.
*
* @param taskInfo task info
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
default Mono await(TaskInfo taskInfo) {
return await(taskInfo.getTaskUid());
}
/**
* Wait for task to complete
*
* @param taskInfo task info
* @param maxAttempts max attempts
* @param fixedDelay fixed delay
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
default Mono await(TaskInfo taskInfo, int maxAttempts, Duration fixedDelay) {
return await(taskInfo.getTaskUid(), maxAttempts, fixedDelay);
}
/**
* Wait for task to complete
*
* @param taskInfo task info
* @param maxAttempts max attempts
* @param fixedDelay fixed delay
* @param maxDuration max duration
* @return task info if MSearchConfig#isAwaitExhaustedError() is false, Mono.error() if true.
*/
default Mono await(TaskInfo taskInfo, int maxAttempts, Duration fixedDelay, Duration maxDuration) {
return await(taskInfo.getTaskUid(), maxAttempts, fixedDelay, maxDuration);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy