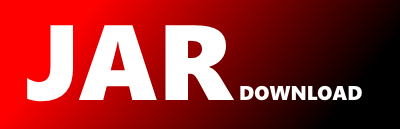
io.github.honhimw.ms.internal.reactive.ReactiveDocumentsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.internal.reactive;
import io.github.honhimw.ms.api.reactive.ReactiveDocuments;
import io.github.honhimw.ms.json.ComplexTypeRef;
import io.github.honhimw.ms.json.TypeRef;
import io.github.honhimw.ms.model.*;
import io.github.honhimw.ms.support.CollectionUtils;
import io.github.honhimw.ms.support.StringUtils;
import io.github.honhimw.ms.support.TypeRefs;
import jakarta.annotation.Nullable;
import reactor.core.publisher.Mono;
import java.util.*;
/**
* @author hon_him
* @since 2024-01-04
*/
class ReactiveDocumentsImpl extends AbstractReactiveImpl implements ReactiveDocuments {
private final ReactiveIndexesImpl indexes;
private final String indexUid;
private final String _path;
protected ReactiveDocumentsImpl(ReactiveIndexesImpl indexes, String indexUid) {
super(indexes._client);
this.indexes = indexes;
this.indexUid = indexUid;
this._path = String.format("/indexes/%s/documents", indexUid);
}
@Override
public Mono>> list(GetDocumentRequest page) {
return list(page, TypeRefs.StringObjectMapRef.INSTANCE);
}
@Override
public Mono> list(@Nullable Integer offset, @Nullable Integer limit, TypeRef typeRef) {
String _offset = Optional.ofNullable(offset).map(String::valueOf).orElse("0");
String _limit = Optional.ofNullable(limit).map(String::valueOf).orElse("20");
return get(_path, configurer -> configurer
.param("offset", _offset)
.param("limit", _limit),
new ComplexTypeRef>(typeRef) {
});
}
@Override
public Mono> list(GetDocumentRequest page, TypeRef typeRef) {
return get(_path, configurer -> {
List fields = page.getFields();
if (CollectionUtils.isNotEmpty(fields)) {
configurer.param("fields", String.join(",", fields));
}
String filter = page.getFilter();
if (StringUtils.isNotEmpty(filter)) {
configurer.param("filter", filter);
}
configurer
.param("offset", String.valueOf(page.toOffset()))
.param("limit", String.valueOf(page.toLimit()));
}, new ComplexTypeRef>(typeRef) {
});
}
@Override
public Mono save(String json) {
return post(_path, configurer -> json(configurer, json), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono save(Collection> collection) {
return post(_path, configurer -> json(configurer, collection), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono saveVectorized(Collection collection) {
return post(_path, configurer -> json(configurer, collection), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono update(String json) {
return put(_path, configurer -> json(configurer, json), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono update(Collection> collection) {
return put(_path, configurer -> json(configurer, collection), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono edit(EditRequest edit) {
return post(String.format("%s/edit", _path), configurer -> json(configurer, edit), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono deleteAll() {
return delete(_path, TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono>> batchGet(BatchGetDocumentsRequest fetch) {
return post(String.format("%s/fetch", _path), configurer -> json(configurer, fetch), TypeRefs.PageStringObjectMapRef.INSTANCE);
}
@Override
public Mono> batchGet(BatchGetDocumentsRequest fetch, TypeRef typeRef) {
return post(String.format("%s/fetch", _path), configurer -> json(configurer, fetch),
new ComplexTypeRef>(typeRef) {
});
}
@Override
public Mono batchDelete(List ids) {
return post(String.format("%s/delete-batch", _path), configurer -> json(configurer, ids), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono delete(FilterableAttributesRequest filter) {
return post(String.format("%s/delete", _path), configurer -> json(configurer, filter), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono
© 2015 - 2025 Weber Informatics LLC | Privacy Policy