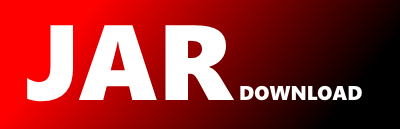
io.github.honhimw.ms.internal.reactive.ReactiveIndexesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.internal.reactive;
import io.github.honhimw.ms.api.reactive.*;
import io.github.honhimw.ms.json.TypeRef;
import io.github.honhimw.ms.model.*;
import io.github.honhimw.ms.support.TypeRefs;
import jakarta.annotation.Nullable;
import reactor.core.publisher.Mono;
import java.util.*;
/**
* @author hon_him
* @since 2024-01-03
*/
class ReactiveIndexesImpl extends AbstractReactiveImpl implements ReactiveIndexes {
protected ReactiveIndexesImpl(ReactiveMSearchClientImpl client) {
super(client);
}
@Override
public Mono> list(@Nullable Integer offset, @Nullable Integer limit) {
String _offset = Optional.ofNullable(offset).map(String::valueOf).orElse("0");
String _limit = Optional.ofNullable(limit).map(String::valueOf).orElse("20");
return get("/indexes", configurer -> configurer
.param("offset", _offset)
.param("limit", _limit),
TypeRefs.PageIndexRef.INSTANCE);
}
@Override
public Mono get(String uid) {
return get(String.format("/indexes/%s", uid), configurer -> {
}, TypeRefs.of(Index.class));
}
@Override
public Mono create(String uid, @Nullable String primaryKey) {
return post("/indexes", configurer -> configurer
.body(payload -> payload
.raw(raw -> {
Map obj = new HashMap<>();
obj.put("uid", uid);
Optional.ofNullable(primaryKey)
.ifPresent(pk -> obj.put("primaryKey", pk));
raw.json(jsonHandler.toJson(obj));
})), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono update(String uid, String primaryKey) {
return patch(String.format("/indexes/%s", uid), configurer -> configurer
.body(payload -> payload
.raw(raw -> {
Map obj = new HashMap<>();
obj.put("primaryKey", primaryKey);
raw.json(jsonHandler.toJson(obj));
})), TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono delete(String uid) {
return delete(String.format("/indexes/%s", uid), configurer -> {
}, TypeRefs.TaskInfoRef.INSTANCE);
}
@Override
public Mono swap(List> uids) {
return post("/swap-indexes", configurer -> configurer
.body(payload -> payload.raw(raw -> {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy