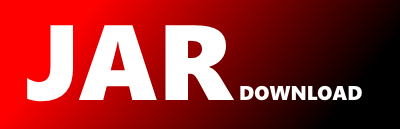
io.github.honhimw.ms.model.CreateKeyRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.model;
import io.github.honhimw.ms.api.annotation.Schema;
import jakarta.annotation.Nonnull;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.util.List;
/**
* Create key request
*
* @author hon_him
* @since 2024-01-02
*/
@Data
@EqualsAndHashCode(callSuper = false)
@NoArgsConstructor
@AllArgsConstructor
public class CreateKeyRequest implements Serializable {
/**
* A list of API actions permitted for the key. [\"*\"] for all actions
* Not null
*/
@Schema(description = "A list of API actions permitted for the key. [\"*\"] for all actions")
@Nonnull
private List actions;
/**
* An array of indexes the key is authorized to act on. [\"*\"] for all indexes
* Not null
*/
@Schema(description = "An array of indexes the key is authorized to act on. [\"*\"] for all indexes")
@Nonnull
private List indexes;
/**
* Date and time when the key will expire, represented in RFC 3339 format. null if the key never expires
*/
@Schema(description = "Date and time when the key will expire, represented in RFC 3339 format. null if the key never expires")
private LocalDateTime expiresAt;
/**
* A human-readable name for the key
*/
@Schema(description = "A human-readable name for the key")
private String name;
/**
* A uuid v4 to identify the API key. If not specified, it is generated by Meilisearch
*/
@Schema(description = "A uuid v4 to identify the API key. If not specified, it is generated by Meilisearch")
private String uid;
/**
* An optional description for the key
*/
@Schema(description = "An optional description for the key")
private String description;
private CreateKeyRequest(Builder builder) {
setActions(builder.actions);
setIndexes(builder.indexes);
setExpiresAt(builder.expiresAt);
setName(builder.name);
setUid(builder.uid);
setDescription(builder.description);
}
/**
* Creates and returns a new instance of the Builder class.
*
* @return a new instance of the Builder class
*/
public static Builder builder() {
return new Builder();
}
/**
* {@code CreateKeyRequest} builder static inner class.
*/
public static final class Builder {
private List actions;
private List indexes;
private LocalDateTime expiresAt;
private String name;
private String uid;
private String description;
private Builder() {
}
/**
* Sets the {@code actions} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code actions} to set
* @return a reference to this Builder
*/
public Builder actions(List val) {
actions = val;
return this;
}
/**
* Sets the {@code indexes} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code indexes} to set
* @return a reference to this Builder
*/
public Builder indexes(List val) {
indexes = val;
return this;
}
/**
* Sets the {@code expiresAt} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code expiresAt} to set
* @return a reference to this Builder
*/
public Builder expiresAt(LocalDateTime val) {
expiresAt = val;
return this;
}
/**
* Sets the {@code name} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code name} to set
* @return a reference to this Builder
*/
public Builder name(String val) {
name = val;
return this;
}
/**
* Sets the {@code uid} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code uid} to set
* @return a reference to this Builder
*/
public Builder uid(String val) {
uid = val;
return this;
}
/**
* Sets the {@code description} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code description} to set
* @return a reference to this Builder
*/
public Builder description(String val) {
description = val;
return this;
}
/**
* Returns a {@code CreateKeyRequest} built from the parameters previously set.
*
* @return a {@code CreateKeyRequest} built with parameters of this {@code CreateKeyRequest.Builder}
*/
public CreateKeyRequest build() {
return new CreateKeyRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy