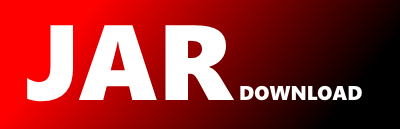
io.github.honhimw.ms.model.ExperimentalFeatures Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.model;
import io.github.honhimw.ms.api.annotation.Schema;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.io.Serializable;
/**
* Experimental features
*
* @author hon_him
* @since 2024-01-03
*/
@Data
@EqualsAndHashCode(callSuper = false)
@NoArgsConstructor
@AllArgsConstructor
public class ExperimentalFeatures implements Serializable {
/**
* true if feature is active, false otherwise
*/
@Schema(description = "true if feature is active, false otherwise")
private Boolean metrics;
/**
* documentation ISSUE #2647
*/
@Schema(description = "true if feature is active, false otherwise")
private Boolean vectorStore;
/**
* edit documents by executing a Rhai function on all the documents of your database or a subset of them that you can select by a Meilisearch filter.
*
* @since v1.10
*/
@Schema(description = "edit documents by executing a Rhai function on all the documents of your database or a subset of them that you can select by a Meilisearch filter.")
private Boolean editDocumentsByFunction;
/**
* true if feature is active, false otherwise
*/
@Schema(description = "true if feature is active, false otherwise")
private Boolean logsRoute;
/**
* Enabling the experimental feature will make a new CONTAINS operator available while filtering on strings.
* This is similar to the SQL LIKE operator used with %.
*
* @since v1.10
*/
@Schema(description = "Enabling the experimental feature will make a new CONTAINS operator available while filtering on strings.")
private Boolean containsFilter;
private ExperimentalFeatures(Builder builder) {
setMetrics(builder.metrics);
setVectorStore(builder.vectorStore);
setEditDocumentsByFunction(builder.editDocumentsByFunction);
setLogsRoute(builder.logsRoute);
setContainsFilter(builder.containsFilter);
}
/**
* Creates and returns a new instance of the Builder class.
*
* @return a new instance of the Builder class
*/
public static Builder builder() {
return new Builder();
}
/**
* {@code ExperimentalFeatures} builder static inner class.
*/
public static final class Builder {
private Boolean metrics;
private Boolean vectorStore;
private Boolean editDocumentsByFunction;
private Boolean logsRoute;
private Boolean containsFilter;
private Builder() {
}
/**
* Sets the {@code metrics} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code metrics} to set
* @return a reference to this Builder
*/
public Builder metrics(Boolean val) {
metrics = val;
return this;
}
/**
* Sets the {@code vectorStore} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code vectorStore} to set
* @return a reference to this Builder
*/
public Builder vectorStore(Boolean val) {
vectorStore = val;
return this;
}
/**
* Sets the {@code editDocumentsByFunction} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code editDocumentsByFunction} to set
* @return a reference to this Builder
*/
public Builder editDocumentsByFunction(Boolean val) {
editDocumentsByFunction = val;
return this;
}
/**
* Sets the {@code logsRoute} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code logsRoute} to set
* @return a reference to this Builder
*/
public Builder logsRoute(Boolean val) {
logsRoute = val;
return this;
}
/**
* Sets the {@code containsFilter} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code containsFilter} to set
* @return a reference to this Builder
*/
public Builder containsFilter(Boolean val) {
containsFilter = val;
return this;
}
/**
* Returns a {@code ExperimentalFeatures} built from the parameters previously set.
*
* @return a {@code ExperimentalFeatures} built with parameters of this {@code ExperimentalFeatures.Builder}
*/
public ExperimentalFeatures build() {
return new ExperimentalFeatures(this);
}
}
/*
* Stabilize since 1.7
* @Schema(description = "true if feature is active, false otherwise")
* private Boolean scoreDetails;
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy