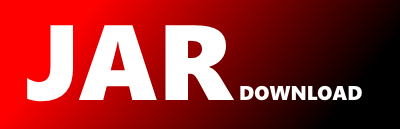
io.github.honhimw.ms.model.Key Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.model;
import io.github.honhimw.ms.api.annotation.Schema;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.util.List;
/**
* Api key
*
* @author hon_him
* @since 2024-01-02
*/
@Data
@EqualsAndHashCode(callSuper = false)
@NoArgsConstructor
@AllArgsConstructor
public class Key implements Serializable {
/**
* A human-readable name for the key
*/
@Schema(description = "A human-readable name for the key")
private String name;
/**
* A description for the key. You can add any important information about the key here
*/
@Schema(description = "A description for the key. You can add any important information about the key here")
private String description;
/**
* A uuid v4 to identify the API key. If not specified, it is automatically generated by Meilisearch
*/
@Schema(description = "A uuid v4 to identify the API key. If not specified, it is automatically generated by Meilisearch")
private String uid;
/**
* This value is also used as the {key} path variable to update, delete, or get a specific key.
* NOTE:
* Since key is a hash of the uid and master key, key values are deterministic between instances sharing the same configuration.
* This means if the master key changes, all key values are automatically changed.
*
* Since the key field depends on the master key, it is computed at runtime and therefore not propagated to dumps and snapshots.
* As a result, even if a malicious user comes into possession of your dumps or snapshots, they will not have access to your instance's API keys.
*/
@Schema(description = "An alphanumeric key value generated by Meilisearch by hashing the uid and the master key on API key creation. " +
"Used for authorization when making calls to a protected Meilisearch instance")
private String key;
/**
* You can use * as a wildcard to access all endpoints for the documents, indexes, tasks, settings, stats and dumps actions. For example, documents.* gives access to all document actions.
*
WARNING:
* For security reasons, we do not recommend creating keys that can perform all actions.
*/
@Schema(description = "An array of API actions permitted for the key, represented as strings. API actions are only possible on authorized indexes. [\"*\"] for all actions.")
private List actions;
/**
* You can also use the * character as a wildcard by adding it at the end of a string.
* This allows an API key access to all index names starting with that string.
* For example, using "indexes": ["movie*"] will give the API key access to the movies and movie_ratings indexes.
*/
@Schema(description = "An array of indexes the key is authorized to act on. Use[\"*\"] for all indexes. Only the key's permitted actions can be used on these indexes.")
private List indexes;
/**
* Date and time when the key will expire, represented in RFC 3339 format. null if the key never expires
*/
@Schema(description = "Date and time when the key will expire, represented in RFC 3339 format. null if the key never expires")
private LocalDateTime expiresAt;
/**
* Date and time when the key was created, represented in RFC 3339 format
*/
@Schema(description = "Date and time when the key was created, represented in RFC 3339 format")
private LocalDateTime createdAt;
/**
* Date and time when the key was last updated, represented in RFC 3339 format
*/
@Schema(description = "Date and time when the key was last updated, represented in RFC 3339 format")
private LocalDateTime updatedAt;
}