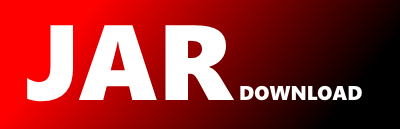
io.github.honhimw.ms.support.MapBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.support;
import java.util.HashMap;
import java.util.Map;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
* Map builder
*
* @param Key type
* @param Value type
* @author hon_him
* @since 2024-01-23
*/
@SuppressWarnings("unused")
public class MapBuilder {
private final Map _map;
private MapBuilder() {
this._map = new HashMap<>();
}
/**
* Create a new {@link MapBuilder}
*
* @param Key type
* @param Value type
* @return a new {@link MapBuilder}
*/
public static MapBuilder builder() {
return new MapBuilder<>();
}
/**
* Put a key-value pair
*
* @param k key
* @param v value
* @return this
*/
public MapBuilder put(K k, V v) {
_map.put(k, v);
return this;
}
/**
* Put all key-value pairs
*
* @param another another map
* @return this
*/
public MapBuilder putAll(Map another) {
_map.putAll(another);
return this;
}
/**
* Clear the map
*
* @return this
*/
public MapBuilder clear() {
_map.clear();
return this;
}
/**
* Build the map
*
* @return the map
*/
public Map build() {
return build(true);
}
/**
* Build the map with mutability. If false, the map will be immutable
*
* @param mut mutability
* @return the map
*/
public Map build(boolean mut) {
if (mut) {
return _map;
} else {
return new ImmutableHashMap<>(_map);
}
}
/**
* Immutable HashMap
*
* @param Key type
* @param Value type
*/
private static class ImmutableHashMap extends HashMap {
/**
* Create an immutable map
*
* @param m from
*/
public ImmutableHashMap(Map extends K, ? extends V> m) {
super(m);
}
@Override
public V put(K key, V value) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public void putAll(Map extends K, ? extends V> m) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V putIfAbsent(K key, V value) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V computeIfAbsent(K key, Function super K, ? extends V> mappingFunction) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V computeIfPresent(K key, BiFunction super K, ? super V, ? extends V> remappingFunction) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V compute(K key, BiFunction super K, ? super V, ? extends V> remappingFunction) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public void replaceAll(BiFunction super K, ? super V, ? extends V> function) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V remove(Object key) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public void clear() {
throw new UnsupportedOperationException("immutable map");
}
@Override
public boolean remove(Object key, Object value) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public boolean replace(K key, V oldValue, V newValue) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V replace(K key, V value) {
throw new UnsupportedOperationException("immutable map");
}
@Override
public V merge(K key, V value, BiFunction super V, ? super V, ? extends V> remappingFunction) {
throw new UnsupportedOperationException("immutable map");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy