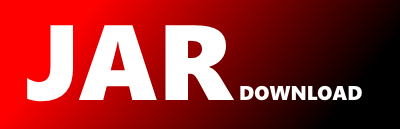
io.github.honhimw.ms.api.reactive.ReactiveSingleIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meilisearch-rest-client Show documentation
Show all versions of meilisearch-rest-client Show documentation
Reactive meilisearch rest client powered by reactor-netty-http.
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.api.reactive;
import io.github.honhimw.ms.json.TypeRef;
import io.github.honhimw.ms.model.Index;
import io.github.honhimw.ms.model.IndexStats;
import io.github.honhimw.ms.model.TaskInfo;
import io.swagger.v3.oas.annotations.Operation;
import jakarta.annotation.Nullable;
import reactor.core.publisher.Mono;
import java.util.function.Function;
/**
* Single Index operator.
*
* @author hon_him
* @since 2024-03-08
*/
public interface ReactiveSingleIndex {
/**
* Get information about current index.
*
* @return current index
*/
@Operation(method = "GET", tags = "/indexes/{index_uid}")
Mono get();
/**
* Create an index.
*
* @param primaryKey Primary key of current index
* @return create task
*/
@Operation(method = "POST", tags = "/indexes")
Mono create(@Nullable String primaryKey);
/**
* Update current index. Specify a primaryKey if it doesn't already exists yet.
*
* @param primaryKey update primary key of current index
* @return update task
*/
@Operation(method = "PATCH", tags = "/indexes/{index_uid}")
Mono update(String primaryKey);
/**
* Delete current index.
*
* @return delete task
*/
@Operation(method = "DELETE", tags = "/indexes/{index_uid}")
Mono delete();
/**
* Documents are objects composed of fields that can store any type of data.
* Each field contains an attribute and its associated value.
* Documents are stored inside indexes.
* Learn more about documents.
*
* @return {@link ReactiveDocuments} operator
*/
@Operation(tags = "/indexes/{index_uid}/documents")
ReactiveDocuments documents();
/**
* Applies the given operation to the documents of the index.
*
* @param operation operation
* @param return type
* @return the result of the operation
*/
default R documents(Function operation) {
return operation.apply(documents());
}
/**
* Get the typed documents of current index.
*
* @param typeRef type reference
* @param document type
* @return {@link ReactiveTypedDocuments} operator
*/
ReactiveTypedDocuments documents(TypeRef typeRef);
/**
* Get the typed documents of current index.
*
* @param type type
* @param document type
* @return {@link ReactiveTypedDocuments} operator
*/
default ReactiveTypedDocuments documents(Class type) {
return documents(TypeRef.of(type));
}
/**
* Applies the given operation to the typed documents of the index.
*
* @param typeRef type reference
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R documents(TypeRef typeRef, Function, R> operation) {
return operation.apply(documents(typeRef));
}
/**
* Applies the given operation to the typed documents of the index.
*
* @param type type
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R documents(Class type, Function, R> operation) {
return operation.apply(documents(type));
}
/**
* Meilisearch exposes 3 routes to perform document searches:
*
* - A POST route: this is the preferred route when using API authentication, as it allows preflight request caching and better performances.
* - A GET route: the usage of this route is discouraged, unless you have good reason to do otherwise (specific caching abilities for example). Other than the differences mentioned above, the two routes are strictly equivalent.
* - A POST multi-search route allowing to perform multiple search queries in a single HTTP request. Meilisearch exposes 1 route to perform facet searches:
* - A POST facet-search route allowing to perform a facet search query on a facet in a single HTTP request.
*
*
* @return {@link ReactiveSearch} operator
*/
@Operation(tags = "/indexes/{index_uid}/search")
ReactiveSearch search();
/**
* Applies the given operation to the search of the index.
*
* @param operation operation
* @param return type
* @return the result of the operation
*/
default R search(Function operation) {
return operation.apply(search());
}
/**
* Get the typed search of current index.
*
* @param typeRef type reference
* @param document type
* @return {@link ReactiveTypedSearch} operator
*/
ReactiveTypedSearch search(TypeRef typeRef);
/**
* Get the typed search of current index.
*
* @param type type
* @param document type
* @return {@link ReactiveTypedSearch} operator
*/
default ReactiveTypedSearch search(Class type) {
return search(TypeRef.of(type));
}
/**
* Applies the given operation to the typed search of the index.
*
* @param typeRef type reference
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R search(TypeRef typeRef, Function, R> operation) {
return operation.apply(search(typeRef));
}
/**
* Applies the given operation to the typed search of the index.
*
* @param type type
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R search(Class type, Function, R> operation) {
return operation.apply(search(type));
}
/**
* Get the typed search with details of current index.
*
* @param typeRef type reference
* @param document type
* @return {@link ReactiveTypedDetailsSearch} operator
*/
ReactiveTypedDetailsSearch searchWithDetails(TypeRef typeRef);
/**
* Get the typed search with details of current index.
*
* @param type type
* @param document type
* @return {@link ReactiveTypedDetailsSearch} operator
*/
default ReactiveTypedDetailsSearch searchWithDetails(Class type) {
return searchWithDetails(TypeRef.of(type));
}
/**
* Applies the given operation to the typed search with details of the index.
*
* @param typeRef type reference
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R searchWithDetails(TypeRef typeRef, Function, R> operation) {
return operation.apply(searchWithDetails(typeRef));
}
/**
* Applies the given operation to the typed search with details of the index.
*
* @param type type
* @param operation operation
* @param document type
* @param return type
* @return the result of the operation
*/
default R searchWithDetails(Class type, Function, R> operation) {
return operation.apply(searchWithDetails(type));
}
/**
* Get the settings of current index.
*
* @return {@link ReactiveSettings} operator
*/
@Operation(tags = "/indexes/{indexUid}/settings")
ReactiveSettings settings();
/**
* Applies the given operation to the settings of the index.
*
* @param operation operation
* @param return type
* @return the result of the operation
*/
default R settings(Function operation) {
return operation.apply(settings());
}
/**
* Get stats of current index.
*
* @return stats of current index.
*/
@Operation(method = "GET", tags = "/indexes/{index_uid}/stats")
Mono stats();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy