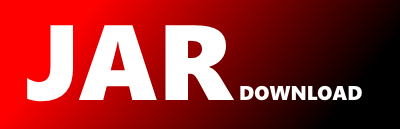
io.github.honhimw.ms.model.SearchRequest Maven / Gradle / Ivy
Show all versions of meilisearch-rest-client Show documentation
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.honhimw.ms.model;
import io.github.honhimw.ms.Experimental;
import io.swagger.v3.oas.annotations.media.Schema;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import java.util.List;
/**
* @author hon_him
* @since 2024-01-02
*/
@Data
@EqualsAndHashCode(callSuper = true)
@NoArgsConstructor
@AllArgsConstructor
public class SearchRequest extends FilterableAttributesRequest {
/**
* Query string
*/
@Schema(description = "Query string")
private String q;
/**
* Number of documents to skip", defaultValue = "0
*/
@Schema(description = "Number of documents to skip", defaultValue = "0")
private Integer offset;
/**
* Maximum number of documents returned", defaultValue = "20
*/
@Schema(description = "Maximum number of documents returned", defaultValue = "20")
private Integer limit;
/**
* Maximum number of documents returned for a page", defaultValue = "1
*/
@Schema(description = "Maximum number of documents returned for a page", defaultValue = "1")
private Integer hitsPerPage;
/**
* Request a specific page of results", defaultValue = "1
*/
@Schema(description = "Request a specific page of results", defaultValue = "1")
private Integer page;
/**
* Display the count of matches per facet
*/
@Schema(description = "Display the count of matches per facet")
private List facets;
/**
* Attributes to display in the returned documents", defaultValue = "[\"*\"]
*/
@Schema(description = "Attributes to display in the returned documents", defaultValue = "[\"*\"]")
private List attributesToRetrieve;
/**
* Attributes whose values have to be cropped
*/
@Schema(description = "Attributes whose values have to be cropped")
private List attributesToCrop;
/**
* Maximum length of cropped value in words", defaultValue = "10
*/
@Schema(description = "Maximum length of cropped value in words", defaultValue = "10")
private Integer cropLength;
/**
* String marking crop boundaries", defaultValue = "\"...\"
*/
@Schema(description = "String marking crop boundaries", defaultValue = "\"...\"")
private String cropMarker;
/**
* Highlight matching terms contained in an attribute
*/
@Schema(description = "Highlight matching terms contained in an attribute")
private List attributesToHighlight;
/**
* String inserted at the start of a highlighted term"
*/
@Schema(description = "String inserted at the start of a highlighted term", defaultValue = "\"\"")
private String highlightPreTag;
/**
* String inserted at the end of a highlighted term"
*/
@Schema(description = "String inserted at the end of a highlighted term", defaultValue = "\"\"")
private String highlightPostTag;
/**
* Return matching terms location", defaultValue = "false
*/
@Schema(description = "Return matching terms location", defaultValue = "false")
private Boolean showMatchesPosition;
/**
* Sort search results by an attribute's value
*/
@Schema(description = "Sort search results by an attribute's value", defaultValue = "null")
private List sort;
/**
* Strategy used to match query terms within documents.
*
* Expected value: last or all
*
* last: returns documents containing all the query terms first. If there are not enough results containing all query terms to meet the requested limit, Meilisearch will remove one query term at a time, starting from the end of the query.
*
* all: only returns documents that contain all query terms. Meilisearch will not match any more documents even if there aren't enough to meet the requested limit.
*/
@Schema(description = "Strategy used to match query terms within documents", defaultValue = "last")
private MatchingStrategy matchingStrategy;
/**
* Display the global ranking score of a document", defaultValue = "false
*/
@Schema(description = "Display the global ranking score of a document", defaultValue = "false")
private Boolean showRankingScore;
/**
* Display the global ranking score of a document", defaultValue = "false
*/
@Schema(description = "Display the global ranking score of a document", defaultValue = "false")
private Boolean showRankingScoreDetails;
/**
* Restrict search to the specified attributes", defaultValue = "[\"*\"]
*/
@Schema(description = "Restrict search to the specified attributes", defaultValue = "[\"*\"]")
private List attributesToSearchOn;
/**
* hybrid is an object and accepts two fields: semanticRatio and embedder
*/
@Experimental(features = Experimental.Features.VECTOR_SEARCH)
@Schema(description = "hybrid is an object and accepts two fields: semanticRatio and embedder")
private Hybrid hybrid;
/**
* must be an array of numbers indicating the search vector. You must generate these yourself when using vector search with user-provided embeddings.
*/
@Experimental(features = Experimental.Features.VECTOR_SEARCH)
@Schema(description = "must be an array of numbers indicating the search vector. You must generate these yourself when using vector search with user-provided embeddings.")
private List vector;
/**
* @since v1.9.0
*/
@Schema(description = "If a distinct attribute is already defined in the settings it'll be ignored in favor of the one defined at search time.")
private String distinct;
/**
* Meilisearch does not return any documents below the configured threshold. Excluded results do not count towards estimatedTotalHits, totalHits, and facet distribution.
*
* For performance reasons, if the number of documents above rankingScoreThreshold is higher than limit, Meilisearch does not evaluate the ranking score of the remaining documents. Results ranking below the threshold are not immediately removed from the set of candidates. In this case, Meilisearch may overestimate the count of estimatedTotalHits, totalHits and facet distribution.
* @since v1.9.0
*/
@Schema(description = "Exclude search results with low ranking scores")
private Number rankingScoreThreshold;
private SearchRequest(Builder builder) {
setFilter(builder.filter);
setQ(builder.q);
setOffset(builder.offset);
setLimit(builder.limit);
setHitsPerPage(builder.hitsPerPage);
setPage(builder.page);
setFacets(builder.facets);
setAttributesToRetrieve(builder.attributesToRetrieve);
setAttributesToCrop(builder.attributesToCrop);
setCropLength(builder.cropLength);
setCropMarker(builder.cropMarker);
setAttributesToHighlight(builder.attributesToHighlight);
setHighlightPreTag(builder.highlightPreTag);
setHighlightPostTag(builder.highlightPostTag);
setShowMatchesPosition(builder.showMatchesPosition);
setSort(builder.sort);
setMatchingStrategy(builder.matchingStrategy);
setShowRankingScore(builder.showRankingScore);
setShowRankingScoreDetails(builder.showRankingScoreDetails);
setAttributesToSearchOn(builder.attributesToSearchOn);
setHybrid(builder.hybrid);
setVector(builder.vector);
setDistinct(builder.distinct);
setRankingScoreThreshold(builder.rankingScoreThreshold);
}
public static Builder builder() {
return new Builder();
}
/**
* {@code SearchRequest} builder static inner class.
*/
public static final class Builder {
private String filter;
private String q;
private Integer offset;
private Integer limit;
private Integer hitsPerPage;
private Integer page;
private List facets;
private List attributesToRetrieve;
private List attributesToCrop;
private Integer cropLength;
private String cropMarker;
private List attributesToHighlight;
private String highlightPreTag;
private String highlightPostTag;
private Boolean showMatchesPosition;
private List sort;
private MatchingStrategy matchingStrategy;
private Boolean showRankingScore;
private Boolean showRankingScoreDetails;
private List attributesToSearchOn;
private Hybrid hybrid;
private List vector;
private String distinct;
private Number rankingScoreThreshold;
private Builder() {
}
/**
* Sets the {@code filter} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code filter} to set
* @return a reference to this Builder
*/
public Builder filter(String val) {
filter = val;
return this;
}
/**
* Sets the {@code q} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code q} to set
* @return a reference to this Builder
*/
public Builder q(String val) {
q = val;
return this;
}
/**
* Sets the {@code offset} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code offset} to set
* @return a reference to this Builder
*/
public Builder offset(Integer val) {
offset = val;
return this;
}
/**
* Sets the {@code limit} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code limit} to set
* @return a reference to this Builder
*/
public Builder limit(Integer val) {
limit = val;
return this;
}
/**
* Sets the {@code hitsPerPage} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code hitsPerPage} to set
* @return a reference to this Builder
*/
public Builder hitsPerPage(Integer val) {
hitsPerPage = val;
return this;
}
/**
* Sets the {@code page} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code page} to set
* @return a reference to this Builder
*/
public Builder page(Integer val) {
page = val;
return this;
}
/**
* Sets the {@code facets} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code facets} to set
* @return a reference to this Builder
*/
public Builder facets(List val) {
facets = val;
return this;
}
/**
* Sets the {@code attributesToRetrieve} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code attributesToRetrieve} to set
* @return a reference to this Builder
*/
public Builder attributesToRetrieve(List val) {
attributesToRetrieve = val;
return this;
}
/**
* Sets the {@code attributesToCrop} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code attributesToCrop} to set
* @return a reference to this Builder
*/
public Builder attributesToCrop(List val) {
attributesToCrop = val;
return this;
}
/**
* Sets the {@code cropLength} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code cropLength} to set
* @return a reference to this Builder
*/
public Builder cropLength(Integer val) {
cropLength = val;
return this;
}
/**
* Sets the {@code cropMarker} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code cropMarker} to set
* @return a reference to this Builder
*/
public Builder cropMarker(String val) {
cropMarker = val;
return this;
}
/**
* Sets the {@code attributesToHighlight} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code attributesToHighlight} to set
* @return a reference to this Builder
*/
public Builder attributesToHighlight(List val) {
attributesToHighlight = val;
return this;
}
/**
* Sets the {@code highlightPreTag} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code highlightPreTag} to set
* @return a reference to this Builder
*/
public Builder highlightPreTag(String val) {
highlightPreTag = val;
return this;
}
/**
* Sets the {@code highlightPostTag} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code highlightPostTag} to set
* @return a reference to this Builder
*/
public Builder highlightPostTag(String val) {
highlightPostTag = val;
return this;
}
/**
* Sets the {@code showMatchesPosition} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code showMatchesPosition} to set
* @return a reference to this Builder
*/
public Builder showMatchesPosition(Boolean val) {
showMatchesPosition = val;
return this;
}
/**
* Sets the {@code sort} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code sort} to set
* @return a reference to this Builder
*/
public Builder sort(List val) {
sort = val;
return this;
}
/**
* Sets the {@code matchingStrategy} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code matchingStrategy} to set
* @return a reference to this Builder
*/
public Builder matchingStrategy(MatchingStrategy val) {
matchingStrategy = val;
return this;
}
/**
* Sets the {@code showRankingScore} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code showRankingScore} to set
* @return a reference to this Builder
*/
public Builder showRankingScore(Boolean val) {
showRankingScore = val;
return this;
}
/**
* Sets the {@code showRankingScoreDetails} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code showRankingScoreDetails} to set
* @return a reference to this Builder
*/
public Builder showRankingScoreDetails(Boolean val) {
showRankingScoreDetails = val;
return this;
}
/**
* Sets the {@code attributesToSearchOn} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code attributesToSearchOn} to set
* @return a reference to this Builder
*/
public Builder attributesToSearchOn(List val) {
attributesToSearchOn = val;
return this;
}
/**
* Sets the {@code hybrid} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code hybrid} to set
* @return a reference to this Builder
*/
public Builder hybrid(Hybrid val) {
hybrid = val;
return this;
}
/**
* Sets the {@code vector} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code vector} to set
* @return a reference to this Builder
*/
public Builder vector(List val) {
vector = val;
return this;
}
/**
* Sets the {@code distinct} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code distinct} to set
* @return a reference to this Builder
*/
public Builder distinct(String val) {
distinct = val;
return this;
}
/**
* Sets the {@code rankingScoreThreshold} and returns a reference to this Builder enabling method chaining.
*
* @param val the {@code rankingScoreThreshold} to set
* @return a reference to this Builder
*/
public Builder rankingScoreThreshold(Number val) {
rankingScoreThreshold = val;
return this;
}
/**
* Returns a {@code SearchRequest} built from the parameters previously set.
*
* @return a {@code SearchRequest} built with parameters of this {@code SearchRequest.Builder}
*/
public SearchRequest build() {
return new SearchRequest(this);
}
}
}