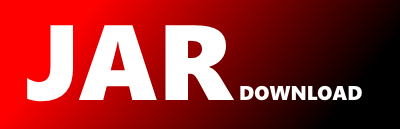
com.radiance.tonclient.Boc Maven / Gradle / Ivy
Show all versions of ton-client-binding Show documentation
package com.radiance.tonclient;
import java.util.concurrent.CompletableFuture;
import java.util.stream.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Arrays;
/**
*
*/
public class Boc {
public static abstract class BocCacheType {
/**
* Such BOC will not be removed from cache until it is unpinned
*/
public static class Pinned extends BocCacheType {
public Pinned(String pin) {
this.pin = pin;
}
public Pinned() {
}
@JsonProperty("pin")
private String pin;
/**
*
*/
public String getPin() {
return pin;
}
/**
*
*/
public void setPin(String value) {
this.pin = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"Pinned\"",(pin==null?null:("\"pin\":\""+pin+"\""))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
public static final Unpinned Unpinned = new Unpinned();
/**
*
*/
public static class Unpinned extends BocCacheType {
public Unpinned() {
}
@Override
public String toString() {
return "{"+"\"type\":\"Unpinned\""+"}";
}
}
}
public static abstract class BuilderOp {
/**
*
*/
public static class Integer extends BuilderOp {
public Integer(Number size, Object value) {
this.size = size;
this.value = value;
}
public Integer(Number size) {
this.size = size;
}
public Integer() {
}
@JsonProperty("size")
private Number size;
/**
*
*/
public Number getSize() {
return size;
}
/**
*
*/
public void setSize(Number value) {
this.size = value;
}
@JsonProperty("value")
private Object value;
/**
* e.g. `123`, `-123`. - Decimal string. e.g. `"123"`, `"-123"`.- `0x` prefixed hexadecimal string. e.g `0x123`, `0X123`, `-0x123`.
*/
public Object getValue() {
return value;
}
/**
* e.g. `123`, `-123`. - Decimal string. e.g. `"123"`, `"-123"`.- `0x` prefixed hexadecimal string. e.g `0x123`, `0X123`, `-0x123`.
*/
public void setValue(Object value) {
this.value = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"Integer\"",(size==null?null:("\"size\":"+size)),(value==null?null:("\"value\":"+value))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class BitString extends BuilderOp {
public BitString(String value) {
this.value = value;
}
public BitString() {
}
@JsonProperty("value")
private String value;
/**
* Contains hexadecimal string representation:- Can end with `_` tag.- Can be prefixed with `x` or `X`.- Can be prefixed with `x{` or `X{` and ended with `}`.Contains binary string represented as a sequenceof `0` and `1` prefixed with `n` or `N`.
Examples:`1AB`, `x1ab`, `X1AB`, `x{1abc}`, `X{1ABC}``2D9_`, `x2D9_`, `X2D9_`, `x{2D9_}`, `X{2D9_}``n00101101100`, `N00101101100`
*/
public String getValue() {
return value;
}
/**
* Contains hexadecimal string representation:- Can end with `_` tag.- Can be prefixed with `x` or `X`.- Can be prefixed with `x{` or `X{` and ended with `}`.
Contains binary string represented as a sequenceof `0` and `1` prefixed with `n` or `N`.
Examples:`1AB`, `x1ab`, `X1AB`, `x{1abc}`, `X{1ABC}``2D9_`, `x2D9_`, `X2D9_`, `x{2D9_}`, `X{2D9_}``n00101101100`, `N00101101100`
*/
public void setValue(String value) {
this.value = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"BitString\"",(value==null?null:("\"value\":\""+value+"\""))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class Cell extends BuilderOp {
public Cell(BuilderOp[] builder) {
this.builder = builder;
}
public Cell() {
}
@JsonProperty("builder")
private BuilderOp[] builder;
/**
*
*/
public BuilderOp[] getBuilder() {
return builder;
}
/**
*
*/
public void setBuilder(BuilderOp[] value) {
this.builder = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"Cell\"",(builder==null?null:("\"builder\":"+Arrays.toString(builder)))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class CellBoc extends BuilderOp {
public CellBoc(String boc) {
this.boc = boc;
}
public CellBoc() {
}
@JsonProperty("boc")
private String boc;
/**
*
*/
public String getBoc() {
return boc;
}
/**
*
*/
public void setBoc(String value) {
this.boc = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"CellBoc\"",(boc==null?null:("\"boc\":\""+boc+"\""))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
}
/**
*
*/
public static class ResultOfDecodeTvc {
public ResultOfDecodeTvc(String code, String data, String library, Boolean tick, Boolean tock, Number splitDepth) {
this.code = code;
this.data = data;
this.library = library;
this.tick = tick;
this.tock = tock;
this.splitDepth = splitDepth;
}
public ResultOfDecodeTvc(String code, String data, String library, Boolean tick, Boolean tock) {
this.code = code;
this.data = data;
this.library = library;
this.tick = tick;
this.tock = tock;
}
public ResultOfDecodeTvc(String code, String data, String library, Boolean tick) {
this.code = code;
this.data = data;
this.library = library;
this.tick = tick;
}
public ResultOfDecodeTvc(String code, String data, String library) {
this.code = code;
this.data = data;
this.library = library;
}
public ResultOfDecodeTvc(String code, String data) {
this.code = code;
this.data = data;
}
public ResultOfDecodeTvc(String code) {
this.code = code;
}
public ResultOfDecodeTvc() {
}
@JsonProperty("code")
private String code;
/**
*
*/
public String getCode() {
return code;
}
/**
*
*/
public void setCode(String value) {
this.code = value;
}
@JsonProperty("data")
private String data;
/**
*
*/
public String getData() {
return data;
}
/**
*
*/
public void setData(String value) {
this.data = value;
}
@JsonProperty("library")
private String library;
/**
*
*/
public String getLibrary() {
return library;
}
/**
*
*/
public void setLibrary(String value) {
this.library = value;
}
@JsonProperty("tick")
private Boolean tick;
/**
* Specifies the contract ability to handle tick transactions
*/
public Boolean getTick() {
return tick;
}
/**
* Specifies the contract ability to handle tick transactions
*/
public void setTick(Boolean value) {
this.tick = value;
}
@JsonProperty("tock")
private Boolean tock;
/**
* Specifies the contract ability to handle tock transactions
*/
public Boolean getTock() {
return tock;
}
/**
* Specifies the contract ability to handle tock transactions
*/
public void setTock(Boolean value) {
this.tock = value;
}
@JsonProperty("split_depth")
private Number splitDepth;
/**
*
*/
public Number getSplitDepth() {
return splitDepth;
}
/**
*
*/
public void setSplitDepth(Number value) {
this.splitDepth = value;
}
@Override
public String toString() {
return "{"+Stream.of((code==null?null:("\"code\":\""+code+"\"")),(data==null?null:("\"data\":\""+data+"\"")),(library==null?null:("\"library\":\""+library+"\"")),(tick==null?null:("\"tick\":"+tick)),(tock==null?null:("\"tock\":"+tock)),(splitDepth==null?null:("\"split_depth\":"+splitDepth))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
private TONContext context;
public Boc(TONContext context) {
this.context = context;
}
/**
* JSON structure is compatible with GraphQL API message object
*
* @param boc
*/
public CompletableFuture