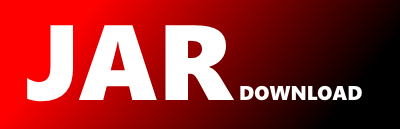
com.radiance.tonclient.Net Maven / Gradle / Ivy
Show all versions of ton-client-binding Show documentation
package com.radiance.tonclient;
import java.util.concurrent.CompletableFuture;
import java.util.stream.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Arrays;
import java.util.function.Consumer;
/**
*
*/
public class Net {
/**
*
*/
public static class OrderBy {
public OrderBy(String path, SortDirection direction) {
this.path = path;
this.direction = direction;
}
public OrderBy(String path) {
this.path = path;
}
public OrderBy() {
}
@JsonProperty("path")
private String path;
/**
*
*/
public String getPath() {
return path;
}
/**
*
*/
public void setPath(String value) {
this.path = value;
}
@JsonProperty("direction")
private SortDirection direction;
/**
*
*/
public SortDirection getDirection() {
return direction;
}
/**
*
*/
public void setDirection(SortDirection value) {
this.direction = value;
}
@Override
public String toString() {
return "{"+Stream.of((path==null?null:("\"path\":\""+path+"\"")),(direction==null?null:("\"direction\":"+direction))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public enum SortDirection {
/**
*
*/
ASC,
/**
*
*/
DESC
}
public static abstract class ParamsOfQueryOperation {
/**
*
*/
public static class QueryCollection extends ParamsOfQueryOperation {
public QueryCollection(String collection, Object filter, String result, OrderBy[] order, Number limit) {
this.collection = collection;
this.filter = filter;
this.result = result;
this.order = order;
this.limit = limit;
}
public QueryCollection(String collection, Object filter, String result, OrderBy[] order) {
this.collection = collection;
this.filter = filter;
this.result = result;
this.order = order;
}
public QueryCollection(String collection, Object filter, String result) {
this.collection = collection;
this.filter = filter;
this.result = result;
}
public QueryCollection(String collection, Object filter) {
this.collection = collection;
this.filter = filter;
}
public QueryCollection(String collection) {
this.collection = collection;
}
public QueryCollection() {
}
@JsonProperty("collection")
private String collection;
/**
*
*/
public String getCollection() {
return collection;
}
/**
*
*/
public void setCollection(String value) {
this.collection = value;
}
@JsonProperty("filter")
private Object filter;
/**
*
*/
public Object getFilter() {
return filter;
}
/**
*
*/
public void setFilter(Object value) {
this.filter = value;
}
@JsonProperty("result")
private String result;
/**
*
*/
public String getResult() {
return result;
}
/**
*
*/
public void setResult(String value) {
this.result = value;
}
@JsonProperty("order")
private OrderBy[] order;
/**
*
*/
public OrderBy[] getOrder() {
return order;
}
/**
*
*/
public void setOrder(OrderBy[] value) {
this.order = value;
}
@JsonProperty("limit")
private Number limit;
/**
*
*/
public Number getLimit() {
return limit;
}
/**
*
*/
public void setLimit(Number value) {
this.limit = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"QueryCollection\"",(collection==null?null:("\"collection\":\""+collection+"\"")),(filter==null?null:("\"filter\":"+filter)),(result==null?null:("\"result\":\""+result+"\"")),(order==null?null:("\"order\":"+Arrays.toString(order))),(limit==null?null:("\"limit\":"+limit))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class WaitForCollection extends ParamsOfQueryOperation {
public WaitForCollection(String collection, Object filter, String result, Number timeout) {
this.collection = collection;
this.filter = filter;
this.result = result;
this.timeout = timeout;
}
public WaitForCollection(String collection, Object filter, String result) {
this.collection = collection;
this.filter = filter;
this.result = result;
}
public WaitForCollection(String collection, Object filter) {
this.collection = collection;
this.filter = filter;
}
public WaitForCollection(String collection) {
this.collection = collection;
}
public WaitForCollection() {
}
@JsonProperty("collection")
private String collection;
/**
*
*/
public String getCollection() {
return collection;
}
/**
*
*/
public void setCollection(String value) {
this.collection = value;
}
@JsonProperty("filter")
private Object filter;
/**
*
*/
public Object getFilter() {
return filter;
}
/**
*
*/
public void setFilter(Object value) {
this.filter = value;
}
@JsonProperty("result")
private String result;
/**
*
*/
public String getResult() {
return result;
}
/**
*
*/
public void setResult(String value) {
this.result = value;
}
@JsonProperty("timeout")
private Number timeout;
/**
*
*/
public Number getTimeout() {
return timeout;
}
/**
*
*/
public void setTimeout(Number value) {
this.timeout = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"WaitForCollection\"",(collection==null?null:("\"collection\":\""+collection+"\"")),(filter==null?null:("\"filter\":"+filter)),(result==null?null:("\"result\":\""+result+"\"")),(timeout==null?null:("\"timeout\":"+timeout))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class AggregateCollection extends ParamsOfQueryOperation {
public AggregateCollection(String collection, Object filter, FieldAggregation[] fields) {
this.collection = collection;
this.filter = filter;
this.fields = fields;
}
public AggregateCollection(String collection, Object filter) {
this.collection = collection;
this.filter = filter;
}
public AggregateCollection(String collection) {
this.collection = collection;
}
public AggregateCollection() {
}
@JsonProperty("collection")
private String collection;
/**
*
*/
public String getCollection() {
return collection;
}
/**
*
*/
public void setCollection(String value) {
this.collection = value;
}
@JsonProperty("filter")
private Object filter;
/**
*
*/
public Object getFilter() {
return filter;
}
/**
*
*/
public void setFilter(Object value) {
this.filter = value;
}
@JsonProperty("fields")
private FieldAggregation[] fields;
/**
*
*/
public FieldAggregation[] getFields() {
return fields;
}
/**
*
*/
public void setFields(FieldAggregation[] value) {
this.fields = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"AggregateCollection\"",(collection==null?null:("\"collection\":\""+collection+"\"")),(filter==null?null:("\"filter\":"+filter)),(fields==null?null:("\"fields\":"+Arrays.toString(fields)))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class QueryCounterparties extends ParamsOfQueryOperation {
public QueryCounterparties(String account, String result, Number first, String after) {
this.account = account;
this.result = result;
this.first = first;
this.after = after;
}
public QueryCounterparties(String account, String result, Number first) {
this.account = account;
this.result = result;
this.first = first;
}
public QueryCounterparties(String account, String result) {
this.account = account;
this.result = result;
}
public QueryCounterparties(String account) {
this.account = account;
}
public QueryCounterparties() {
}
@JsonProperty("account")
private String account;
/**
*
*/
public String getAccount() {
return account;
}
/**
*
*/
public void setAccount(String value) {
this.account = value;
}
@JsonProperty("result")
private String result;
/**
*
*/
public String getResult() {
return result;
}
/**
*
*/
public void setResult(String value) {
this.result = value;
}
@JsonProperty("first")
private Number first;
/**
*
*/
public Number getFirst() {
return first;
}
/**
*
*/
public void setFirst(Number value) {
this.first = value;
}
@JsonProperty("after")
private String after;
/**
*
*/
public String getAfter() {
return after;
}
/**
*
*/
public void setAfter(String value) {
this.after = value;
}
@Override
public String toString() {
return "{"+Stream.of("\"type\":\"QueryCounterparties\"",(account==null?null:("\"account\":\""+account+"\"")),(result==null?null:("\"result\":\""+result+"\"")),(first==null?null:("\"first\":"+first)),(after==null?null:("\"after\":\""+after+"\""))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
}
/**
*
*/
public static class FieldAggregation {
public FieldAggregation(String field, AggregationFn fn) {
this.field = field;
this.fn = fn;
}
public FieldAggregation(String field) {
this.field = field;
}
public FieldAggregation() {
}
@JsonProperty("field")
private String field;
/**
*
*/
public String getField() {
return field;
}
/**
*
*/
public void setField(String value) {
this.field = value;
}
@JsonProperty("fn")
private AggregationFn fn;
/**
*
*/
public AggregationFn getFn() {
return fn;
}
/**
*
*/
public void setFn(AggregationFn value) {
this.fn = value;
}
@Override
public String toString() {
return "{"+Stream.of((field==null?null:("\"field\":\""+field+"\"")),(fn==null?null:("\"fn\":"+fn))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public enum AggregationFn {
/**
*
*/
COUNT,
/**
*
*/
MIN,
/**
*
*/
MAX,
/**
*
*/
SUM,
/**
*
*/
AVERAGE
}
/**
*
*/
public static class TransactionNode {
public TransactionNode(String id, String inMsg, String[] outMsgs, String accountAddr, String totalFees, Boolean aborted, Number exitCode) {
this.id = id;
this.inMsg = inMsg;
this.outMsgs = outMsgs;
this.accountAddr = accountAddr;
this.totalFees = totalFees;
this.aborted = aborted;
this.exitCode = exitCode;
}
public TransactionNode(String id, String inMsg, String[] outMsgs, String accountAddr, String totalFees, Boolean aborted) {
this.id = id;
this.inMsg = inMsg;
this.outMsgs = outMsgs;
this.accountAddr = accountAddr;
this.totalFees = totalFees;
this.aborted = aborted;
}
public TransactionNode(String id, String inMsg, String[] outMsgs, String accountAddr, String totalFees) {
this.id = id;
this.inMsg = inMsg;
this.outMsgs = outMsgs;
this.accountAddr = accountAddr;
this.totalFees = totalFees;
}
public TransactionNode(String id, String inMsg, String[] outMsgs, String accountAddr) {
this.id = id;
this.inMsg = inMsg;
this.outMsgs = outMsgs;
this.accountAddr = accountAddr;
}
public TransactionNode(String id, String inMsg, String[] outMsgs) {
this.id = id;
this.inMsg = inMsg;
this.outMsgs = outMsgs;
}
public TransactionNode(String id, String inMsg) {
this.id = id;
this.inMsg = inMsg;
}
public TransactionNode(String id) {
this.id = id;
}
public TransactionNode() {
}
@JsonProperty("id")
private String id;
/**
*
*/
public String getId() {
return id;
}
/**
*
*/
public void setId(String value) {
this.id = value;
}
@JsonProperty("in_msg")
private String inMsg;
/**
*
*/
public String getInMsg() {
return inMsg;
}
/**
*
*/
public void setInMsg(String value) {
this.inMsg = value;
}
@JsonProperty("out_msgs")
private String[] outMsgs;
/**
*
*/
public String[] getOutMsgs() {
return outMsgs;
}
/**
*
*/
public void setOutMsgs(String[] value) {
this.outMsgs = value;
}
@JsonProperty("account_addr")
private String accountAddr;
/**
*
*/
public String getAccountAddr() {
return accountAddr;
}
/**
*
*/
public void setAccountAddr(String value) {
this.accountAddr = value;
}
@JsonProperty("total_fees")
private String totalFees;
/**
*
*/
public String getTotalFees() {
return totalFees;
}
/**
*
*/
public void setTotalFees(String value) {
this.totalFees = value;
}
@JsonProperty("aborted")
private Boolean aborted;
/**
*
*/
public Boolean getAborted() {
return aborted;
}
/**
*
*/
public void setAborted(Boolean value) {
this.aborted = value;
}
@JsonProperty("exit_code")
private Number exitCode;
/**
*
*/
public Number getExitCode() {
return exitCode;
}
/**
*
*/
public void setExitCode(Number value) {
this.exitCode = value;
}
@Override
public String toString() {
return "{"+Stream.of((id==null?null:("\"id\":\""+id+"\"")),(inMsg==null?null:("\"in_msg\":\""+inMsg+"\"")),(outMsgs==null?null:("\"out_msgs\":\""+Arrays.toString(outMsgs)+"\"")),(accountAddr==null?null:("\"account_addr\":\""+accountAddr+"\"")),(totalFees==null?null:("\"total_fees\":\""+totalFees+"\"")),(aborted==null?null:("\"aborted\":"+aborted)),(exitCode==null?null:("\"exit_code\":"+exitCode))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class MessageNode {
public MessageNode(String id, String srcTransactionId, String dstTransactionId, String src, String dst, String value, Boolean bounce, Abi.DecodedMessageBody decodedBody) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
this.src = src;
this.dst = dst;
this.value = value;
this.bounce = bounce;
this.decodedBody = decodedBody;
}
public MessageNode(String id, String srcTransactionId, String dstTransactionId, String src, String dst, String value, Boolean bounce) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
this.src = src;
this.dst = dst;
this.value = value;
this.bounce = bounce;
}
public MessageNode(String id, String srcTransactionId, String dstTransactionId, String src, String dst, String value) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
this.src = src;
this.dst = dst;
this.value = value;
}
public MessageNode(String id, String srcTransactionId, String dstTransactionId, String src, String dst) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
this.src = src;
this.dst = dst;
}
public MessageNode(String id, String srcTransactionId, String dstTransactionId, String src) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
this.src = src;
}
public MessageNode(String id, String srcTransactionId, String dstTransactionId) {
this.id = id;
this.srcTransactionId = srcTransactionId;
this.dstTransactionId = dstTransactionId;
}
public MessageNode(String id, String srcTransactionId) {
this.id = id;
this.srcTransactionId = srcTransactionId;
}
public MessageNode(String id) {
this.id = id;
}
public MessageNode() {
}
@JsonProperty("id")
private String id;
/**
*
*/
public String getId() {
return id;
}
/**
*
*/
public void setId(String value) {
this.id = value;
}
@JsonProperty("src_transaction_id")
private String srcTransactionId;
/**
* This field is missing for an external inbound messages.
*/
public String getSrcTransactionId() {
return srcTransactionId;
}
/**
* This field is missing for an external inbound messages.
*/
public void setSrcTransactionId(String value) {
this.srcTransactionId = value;
}
@JsonProperty("dst_transaction_id")
private String dstTransactionId;
/**
* This field is missing for an external outbound messages.
*/
public String getDstTransactionId() {
return dstTransactionId;
}
/**
* This field is missing for an external outbound messages.
*/
public void setDstTransactionId(String value) {
this.dstTransactionId = value;
}
@JsonProperty("src")
private String src;
/**
*
*/
public String getSrc() {
return src;
}
/**
*
*/
public void setSrc(String value) {
this.src = value;
}
@JsonProperty("dst")
private String dst;
/**
*
*/
public String getDst() {
return dst;
}
/**
*
*/
public void setDst(String value) {
this.dst = value;
}
@JsonProperty("value")
private String value;
/**
*
*/
public String getValue() {
return value;
}
/**
*
*/
public void setValue(String value) {
this.value = value;
}
@JsonProperty("bounce")
private Boolean bounce;
/**
*
*/
public Boolean getBounce() {
return bounce;
}
/**
*
*/
public void setBounce(Boolean value) {
this.bounce = value;
}
@JsonProperty("decoded_body")
private Abi.DecodedMessageBody decodedBody;
/**
* Library tries to decode message body using provided `params.abi_registry`.This field will be missing if none of the provided abi can be used to decode.
*/
public Abi.DecodedMessageBody getDecodedBody() {
return decodedBody;
}
/**
* Library tries to decode message body using provided `params.abi_registry`.This field will be missing if none of the provided abi can be used to decode.
*/
public void setDecodedBody(Abi.DecodedMessageBody value) {
this.decodedBody = value;
}
@Override
public String toString() {
return "{"+Stream.of((id==null?null:("\"id\":\""+id+"\"")),(srcTransactionId==null?null:("\"src_transaction_id\":\""+srcTransactionId+"\"")),(dstTransactionId==null?null:("\"dst_transaction_id\":\""+dstTransactionId+"\"")),(src==null?null:("\"src\":\""+src+"\"")),(dst==null?null:("\"dst\":\""+dst+"\"")),(value==null?null:("\"value\":\""+value+"\"")),(bounce==null?null:("\"bounce\":"+bounce)),(decodedBody==null?null:("\"decoded_body\":"+decodedBody))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class ResultOfGetEndpoints {
public ResultOfGetEndpoints(String query, String[] endpoints) {
this.query = query;
this.endpoints = endpoints;
}
public ResultOfGetEndpoints(String query) {
this.query = query;
}
public ResultOfGetEndpoints() {
}
@JsonProperty("query")
private String query;
/**
*
*/
public String getQuery() {
return query;
}
/**
*
*/
public void setQuery(String value) {
this.query = value;
}
@JsonProperty("endpoints")
private String[] endpoints;
/**
*
*/
public String[] getEndpoints() {
return endpoints;
}
/**
*
*/
public void setEndpoints(String[] value) {
this.endpoints = value;
}
@Override
public String toString() {
return "{"+Stream.of((query==null?null:("\"query\":\""+query+"\"")),(endpoints==null?null:("\"endpoints\":\""+Arrays.toString(endpoints)+"\""))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class ResultOfQueryTransactionTree {
public ResultOfQueryTransactionTree(MessageNode[] messages, TransactionNode[] transactions) {
this.messages = messages;
this.transactions = transactions;
}
public ResultOfQueryTransactionTree(MessageNode[] messages) {
this.messages = messages;
}
public ResultOfQueryTransactionTree() {
}
@JsonProperty("messages")
private MessageNode[] messages;
/**
*
*/
public MessageNode[] getMessages() {
return messages;
}
/**
*
*/
public void setMessages(MessageNode[] value) {
this.messages = value;
}
@JsonProperty("transactions")
private TransactionNode[] transactions;
/**
*
*/
public TransactionNode[] getTransactions() {
return transactions;
}
/**
*
*/
public void setTransactions(TransactionNode[] value) {
this.transactions = value;
}
@Override
public String toString() {
return "{"+Stream.of((messages==null?null:("\"messages\":"+Arrays.toString(messages))),(transactions==null?null:("\"transactions\":"+Arrays.toString(transactions)))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
/**
*
*/
public static class ResultOfIteratorNext {
public ResultOfIteratorNext(Object[] items, Boolean hasMore, Object resumeState) {
this.items = items;
this.hasMore = hasMore;
this.resumeState = resumeState;
}
public ResultOfIteratorNext(Object[] items, Boolean hasMore) {
this.items = items;
this.hasMore = hasMore;
}
public ResultOfIteratorNext(Object[] items) {
this.items = items;
}
public ResultOfIteratorNext() {
}
@JsonProperty("items")
private Object[] items;
/**
* Note that `iterator_next` can return an empty items and `has_more` equals to `true`.In this case the application have to continue iteration.Such situation can take place when there is no data yet butthe requested `end_time` is not reached.
*/
public Object[] getItems() {
return items;
}
/**
* Note that `iterator_next` can return an empty items and `has_more` equals to `true`.In this case the application have to continue iteration.Such situation can take place when there is no data yet butthe requested `end_time` is not reached.
*/
public void setItems(Object[] value) {
this.items = value;
}
@JsonProperty("has_more")
private Boolean hasMore;
/**
*
*/
public Boolean getHasMore() {
return hasMore;
}
/**
*
*/
public void setHasMore(Boolean value) {
this.hasMore = value;
}
@JsonProperty("resume_state")
private Object resumeState;
/**
* This field is returned only if the `return_resume_state` parameteris specified.Note that `resume_state` corresponds to the iteration positionafter the returned items.
*/
public Object getResumeState() {
return resumeState;
}
/**
* This field is returned only if the `return_resume_state` parameteris specified.
Note that `resume_state` corresponds to the iteration positionafter the returned items.
*/
public void setResumeState(Object value) {
this.resumeState = value;
}
@Override
public String toString() {
return "{"+Stream.of((items==null?null:("\"items\":"+Arrays.toString(items))),(hasMore==null?null:("\"has_more\":"+hasMore)),(resumeState==null?null:("\"resume_state\":"+resumeState))).filter(_f -> _f != null).collect(Collectors.joining(","))+"}";
}
}
private TONContext context;
public Net(TONContext context) {
this.context = context;
}
/**
*
*
* @param query
* @param variables Must be a map with named values that can be used in query.
*/
public CompletableFuture