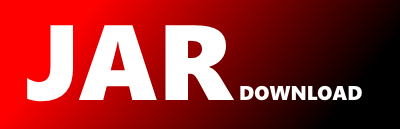
org.eolang.parser.ProgramParser Maven / Gradle / Ivy
The newest version!
// Generated from org/eolang/parser/Program.g4 by ANTLR 4.8
package org.eolang.parser;
import java.util.LinkedList;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class ProgramParser extends Parser {
static { RuntimeMetaData.checkVersion("4.8", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
COMMENT=1, META=2, REGEX=3, STAR=4, DOTS=5, CONST=6, SLASH=7, COLON=8,
ARROW=9, SELF=10, PLUS=11, MINUS=12, SPACE=13, DOT=14, LSQ=15, RSQ=16,
LB=17, RB=18, AT=19, PARENT=20, HASH=21, EOL=22, BYTES=23, BOOL=24, CHAR=25,
STRING=26, INT=27, FLOAT=28, HEX=29, NAME=30, TAB=31, UNTAB=32;
public static final int
RULE_program = 0, RULE_license = 1, RULE_metas = 2, RULE_objects = 3,
RULE_object = 4, RULE_anonymous = 5, RULE_abstraction = 6, RULE_attributes = 7,
RULE_attribute = 8, RULE_label = 9, RULE_tail = 10, RULE_suffix = 11,
RULE_method = 12, RULE_application = 13, RULE_htail = 14, RULE_head = 15,
RULE_has = 16, RULE_data = 17;
private static String[] makeRuleNames() {
return new String[] {
"program", "license", "metas", "objects", "object", "anonymous", "abstraction",
"attributes", "attribute", "label", "tail", "suffix", "method", "application",
"htail", "head", "has", "data"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, "'*'", "'...'", "'!'", "'/'", "':'", "'>'", "'$'",
"'+'", "'-'", "' '", "'.'", "'['", "']'", "'('", "')'", "'@'", "'^'",
"'#'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "COMMENT", "META", "REGEX", "STAR", "DOTS", "CONST", "SLASH", "COLON",
"ARROW", "SELF", "PLUS", "MINUS", "SPACE", "DOT", "LSQ", "RSQ", "LB",
"RB", "AT", "PARENT", "HASH", "EOL", "BYTES", "BOOL", "CHAR", "STRING",
"INT", "FLOAT", "HEX", "NAME", "TAB", "UNTAB"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "Program.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public ProgramParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class ProgramContext extends ParserRuleContext {
public ObjectsContext objects() {
return getRuleContext(ObjectsContext.class,0);
}
public TerminalNode EOF() { return getToken(ProgramParser.EOF, 0); }
public LicenseContext license() {
return getRuleContext(LicenseContext.class,0);
}
public MetasContext metas() {
return getRuleContext(MetasContext.class,0);
}
public ProgramContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_program; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterProgram(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitProgram(this);
}
}
public final ProgramContext program() throws RecognitionException {
ProgramContext _localctx = new ProgramContext(_ctx, getState());
enterRule(_localctx, 0, RULE_program);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(37);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
{
setState(36);
license();
}
break;
}
setState(40);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==META) {
{
setState(39);
metas();
}
}
setState(42);
objects();
setState(43);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LicenseContext extends ParserRuleContext {
public List COMMENT() { return getTokens(ProgramParser.COMMENT); }
public TerminalNode COMMENT(int i) {
return getToken(ProgramParser.COMMENT, i);
}
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public LicenseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_license; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterLicense(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitLicense(this);
}
}
public final LicenseContext license() throws RecognitionException {
LicenseContext _localctx = new LicenseContext(_ctx, getState());
enterRule(_localctx, 2, RULE_license);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(47);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(45);
match(COMMENT);
setState(46);
match(EOL);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(49);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MetasContext extends ParserRuleContext {
public List META() { return getTokens(ProgramParser.META); }
public TerminalNode META(int i) {
return getToken(ProgramParser.META, i);
}
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public MetasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_metas; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterMetas(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitMetas(this);
}
}
public final MetasContext metas() throws RecognitionException {
MetasContext _localctx = new MetasContext(_ctx, getState());
enterRule(_localctx, 4, RULE_metas);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(53);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(51);
match(META);
setState(52);
match(EOL);
}
}
setState(55);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==META );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ObjectsContext extends ParserRuleContext {
public List object() {
return getRuleContexts(ObjectContext.class);
}
public ObjectContext object(int i) {
return getRuleContext(ObjectContext.class,i);
}
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public List COMMENT() { return getTokens(ProgramParser.COMMENT); }
public TerminalNode COMMENT(int i) {
return getToken(ProgramParser.COMMENT, i);
}
public ObjectsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_objects; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterObjects(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitObjects(this);
}
}
public final ObjectsContext objects() throws RecognitionException {
ObjectsContext _localctx = new ObjectsContext(_ctx, getState());
enterRule(_localctx, 6, RULE_objects);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(67);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(61);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(57);
match(COMMENT);
setState(58);
match(EOL);
}
}
}
setState(63);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
}
setState(64);
object();
setState(65);
match(EOL);
}
}
setState(69);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( (((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMENT) | (1L << REGEX) | (1L << STAR) | (1L << SELF) | (1L << LSQ) | (1L << LB) | (1L << AT) | (1L << PARENT) | (1L << BYTES) | (1L << BOOL) | (1L << CHAR) | (1L << STRING) | (1L << INT) | (1L << FLOAT) | (1L << HEX) | (1L << NAME))) != 0) );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ObjectContext extends ParserRuleContext {
public AnonymousContext anonymous() {
return getRuleContext(AnonymousContext.class,0);
}
public AbstractionContext abstraction() {
return getRuleContext(AbstractionContext.class,0);
}
public ApplicationContext application() {
return getRuleContext(ApplicationContext.class,0);
}
public List tail() {
return getRuleContexts(TailContext.class);
}
public TailContext tail(int i) {
return getRuleContext(TailContext.class,i);
}
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public List method() {
return getRuleContexts(MethodContext.class);
}
public MethodContext method(int i) {
return getRuleContext(MethodContext.class,i);
}
public List htail() {
return getRuleContexts(HtailContext.class);
}
public HtailContext htail(int i) {
return getRuleContext(HtailContext.class,i);
}
public List suffix() {
return getRuleContexts(SuffixContext.class);
}
public SuffixContext suffix(int i) {
return getRuleContext(SuffixContext.class,i);
}
public ObjectContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_object; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterObject(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitObject(this);
}
}
public final ObjectContext object() throws RecognitionException {
ObjectContext _localctx = new ObjectContext(_ctx, getState());
enterRule(_localctx, 8, RULE_object);
int _la;
try {
int _alt;
setState(95);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,12,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(71);
anonymous();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(74);
_errHandler.sync(this);
switch (_input.LA(1)) {
case COMMENT:
case LSQ:
{
setState(72);
abstraction();
}
break;
case REGEX:
case STAR:
case SELF:
case LB:
case AT:
case PARENT:
case BYTES:
case BOOL:
case CHAR:
case STRING:
case INT:
case FLOAT:
case HEX:
case NAME:
{
setState(73);
application(0);
}
break;
default:
throw new NoViableAltException(this);
}
setState(77);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,7,_ctx) ) {
case 1:
{
setState(76);
tail();
}
break;
}
setState(92);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(79);
match(EOL);
setState(80);
method();
setState(82);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,8,_ctx) ) {
case 1:
{
setState(81);
htail();
}
break;
}
setState(85);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SPACE) {
{
setState(84);
suffix();
}
}
setState(88);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,10,_ctx) ) {
case 1:
{
setState(87);
tail();
}
break;
}
}
}
}
setState(94);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AnonymousContext extends ParserRuleContext {
public AttributesContext attributes() {
return getRuleContext(AttributesContext.class,0);
}
public HtailContext htail() {
return getRuleContext(HtailContext.class,0);
}
public AnonymousContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_anonymous; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterAnonymous(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitAnonymous(this);
}
}
public final AnonymousContext anonymous() throws RecognitionException {
AnonymousContext _localctx = new AnonymousContext(_ctx, getState());
enterRule(_localctx, 10, RULE_anonymous);
try {
enterOuterAlt(_localctx, 1);
{
setState(97);
attributes();
setState(98);
htail();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AbstractionContext extends ParserRuleContext {
public AttributesContext attributes() {
return getRuleContext(AttributesContext.class,0);
}
public List COMMENT() { return getTokens(ProgramParser.COMMENT); }
public TerminalNode COMMENT(int i) {
return getToken(ProgramParser.COMMENT, i);
}
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public SuffixContext suffix() {
return getRuleContext(SuffixContext.class,0);
}
public TerminalNode SPACE() { return getToken(ProgramParser.SPACE, 0); }
public TerminalNode SLASH() { return getToken(ProgramParser.SLASH, 0); }
public TerminalNode NAME() { return getToken(ProgramParser.NAME, 0); }
public AbstractionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_abstraction; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterAbstraction(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitAbstraction(this);
}
}
public final AbstractionContext abstraction() throws RecognitionException {
AbstractionContext _localctx = new AbstractionContext(_ctx, getState());
enterRule(_localctx, 12, RULE_abstraction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(104);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMENT) {
{
{
setState(100);
match(COMMENT);
setState(101);
match(EOL);
}
}
setState(106);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(107);
attributes();
setState(114);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SPACE) {
{
setState(108);
suffix();
setState(112);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SPACE) {
{
setState(109);
match(SPACE);
setState(110);
match(SLASH);
setState(111);
match(NAME);
}
}
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AttributesContext extends ParserRuleContext {
public TerminalNode LSQ() { return getToken(ProgramParser.LSQ, 0); }
public TerminalNode RSQ() { return getToken(ProgramParser.RSQ, 0); }
public List attribute() {
return getRuleContexts(AttributeContext.class);
}
public AttributeContext attribute(int i) {
return getRuleContext(AttributeContext.class,i);
}
public List SPACE() { return getTokens(ProgramParser.SPACE); }
public TerminalNode SPACE(int i) {
return getToken(ProgramParser.SPACE, i);
}
public AttributesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_attributes; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterAttributes(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitAttributes(this);
}
}
public final AttributesContext attributes() throws RecognitionException {
AttributesContext _localctx = new AttributesContext(_ctx, getState());
enterRule(_localctx, 14, RULE_attributes);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(116);
match(LSQ);
setState(125);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT || _la==NAME) {
{
setState(117);
attribute();
setState(122);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SPACE) {
{
{
setState(118);
match(SPACE);
setState(119);
attribute();
}
}
setState(124);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(127);
match(RSQ);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AttributeContext extends ParserRuleContext {
public LabelContext label() {
return getRuleContext(LabelContext.class,0);
}
public AttributeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_attribute; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterAttribute(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitAttribute(this);
}
}
public final AttributeContext attribute() throws RecognitionException {
AttributeContext _localctx = new AttributeContext(_ctx, getState());
enterRule(_localctx, 16, RULE_attribute);
try {
enterOuterAlt(_localctx, 1);
{
setState(129);
label();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LabelContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(ProgramParser.AT, 0); }
public TerminalNode NAME() { return getToken(ProgramParser.NAME, 0); }
public TerminalNode DOTS() { return getToken(ProgramParser.DOTS, 0); }
public LabelContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_label; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterLabel(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitLabel(this);
}
}
public final LabelContext label() throws RecognitionException {
LabelContext _localctx = new LabelContext(_ctx, getState());
enterRule(_localctx, 18, RULE_label);
try {
setState(136);
_errHandler.sync(this);
switch (_input.LA(1)) {
case AT:
enterOuterAlt(_localctx, 1);
{
setState(131);
match(AT);
}
break;
case NAME:
enterOuterAlt(_localctx, 2);
{
setState(132);
match(NAME);
setState(134);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,18,_ctx) ) {
case 1:
{
setState(133);
match(DOTS);
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TailContext extends ParserRuleContext {
public List EOL() { return getTokens(ProgramParser.EOL); }
public TerminalNode EOL(int i) {
return getToken(ProgramParser.EOL, i);
}
public TerminalNode TAB() { return getToken(ProgramParser.TAB, 0); }
public TerminalNode UNTAB() { return getToken(ProgramParser.UNTAB, 0); }
public List object() {
return getRuleContexts(ObjectContext.class);
}
public ObjectContext object(int i) {
return getRuleContext(ObjectContext.class,i);
}
public TailContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_tail; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterTail(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitTail(this);
}
}
public final TailContext tail() throws RecognitionException {
TailContext _localctx = new TailContext(_ctx, getState());
enterRule(_localctx, 20, RULE_tail);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(138);
match(EOL);
setState(139);
match(TAB);
setState(143);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(140);
object();
setState(141);
match(EOL);
}
}
setState(145);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( (((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMENT) | (1L << REGEX) | (1L << STAR) | (1L << SELF) | (1L << LSQ) | (1L << LB) | (1L << AT) | (1L << PARENT) | (1L << BYTES) | (1L << BOOL) | (1L << CHAR) | (1L << STRING) | (1L << INT) | (1L << FLOAT) | (1L << HEX) | (1L << NAME))) != 0) );
setState(147);
match(UNTAB);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SuffixContext extends ParserRuleContext {
public List SPACE() { return getTokens(ProgramParser.SPACE); }
public TerminalNode SPACE(int i) {
return getToken(ProgramParser.SPACE, i);
}
public TerminalNode ARROW() { return getToken(ProgramParser.ARROW, 0); }
public LabelContext label() {
return getRuleContext(LabelContext.class,0);
}
public TerminalNode CONST() { return getToken(ProgramParser.CONST, 0); }
public SuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_suffix; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterSuffix(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitSuffix(this);
}
}
public final SuffixContext suffix() throws RecognitionException {
SuffixContext _localctx = new SuffixContext(_ctx, getState());
enterRule(_localctx, 22, RULE_suffix);
try {
enterOuterAlt(_localctx, 1);
{
setState(149);
match(SPACE);
setState(150);
match(ARROW);
setState(151);
match(SPACE);
setState(152);
label();
setState(154);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,21,_ctx) ) {
case 1:
{
setState(153);
match(CONST);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MethodContext extends ParserRuleContext {
public TerminalNode DOT() { return getToken(ProgramParser.DOT, 0); }
public TerminalNode NAME() { return getToken(ProgramParser.NAME, 0); }
public TerminalNode PARENT() { return getToken(ProgramParser.PARENT, 0); }
public MethodContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_method; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterMethod(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitMethod(this);
}
}
public final MethodContext method() throws RecognitionException {
MethodContext _localctx = new MethodContext(_ctx, getState());
enterRule(_localctx, 24, RULE_method);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(156);
match(DOT);
setState(157);
_la = _input.LA(1);
if ( !(_la==PARENT || _la==NAME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ApplicationContext extends ParserRuleContext {
public HeadContext head() {
return getRuleContext(HeadContext.class,0);
}
public HtailContext htail() {
return getRuleContext(HtailContext.class,0);
}
public TerminalNode LB() { return getToken(ProgramParser.LB, 0); }
public ApplicationContext application() {
return getRuleContext(ApplicationContext.class,0);
}
public TerminalNode RB() { return getToken(ProgramParser.RB, 0); }
public MethodContext method() {
return getRuleContext(MethodContext.class,0);
}
public HasContext has() {
return getRuleContext(HasContext.class,0);
}
public SuffixContext suffix() {
return getRuleContext(SuffixContext.class,0);
}
public ApplicationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_application; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterApplication(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitApplication(this);
}
}
public final ApplicationContext application() throws RecognitionException {
return application(0);
}
private ApplicationContext application(int _p) throws RecognitionException {
ParserRuleContext _parentctx = _ctx;
int _parentState = getState();
ApplicationContext _localctx = new ApplicationContext(_ctx, _parentState);
ApplicationContext _prevctx = _localctx;
int _startState = 26;
enterRecursionRule(_localctx, 26, RULE_application, _p);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(170);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REGEX:
case STAR:
case SELF:
case AT:
case PARENT:
case BYTES:
case BOOL:
case CHAR:
case STRING:
case INT:
case FLOAT:
case HEX:
case NAME:
{
setState(160);
head();
setState(162);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,22,_ctx) ) {
case 1:
{
setState(161);
htail();
}
break;
}
}
break;
case LB:
{
setState(164);
match(LB);
setState(165);
application(0);
setState(166);
match(RB);
setState(168);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,23,_ctx) ) {
case 1:
{
setState(167);
htail();
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
_ctx.stop = _input.LT(-1);
setState(189);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,29,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
if ( _parseListeners!=null ) triggerExitRuleEvent();
_prevctx = _localctx;
{
setState(187);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,28,_ctx) ) {
case 1:
{
_localctx = new ApplicationContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_application);
setState(172);
if (!(precpred(_ctx, 4))) throw new FailedPredicateException(this, "precpred(_ctx, 4)");
setState(173);
method();
setState(175);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,25,_ctx) ) {
case 1:
{
setState(174);
htail();
}
break;
}
}
break;
case 2:
{
_localctx = new ApplicationContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_application);
setState(177);
if (!(precpred(_ctx, 2))) throw new FailedPredicateException(this, "precpred(_ctx, 2)");
setState(178);
has();
setState(180);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,26,_ctx) ) {
case 1:
{
setState(179);
htail();
}
break;
}
}
break;
case 3:
{
_localctx = new ApplicationContext(_parentctx, _parentState);
pushNewRecursionContext(_localctx, _startState, RULE_application);
setState(182);
if (!(precpred(_ctx, 1))) throw new FailedPredicateException(this, "precpred(_ctx, 1)");
setState(183);
suffix();
setState(185);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,27,_ctx) ) {
case 1:
{
setState(184);
htail();
}
break;
}
}
break;
}
}
}
setState(191);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,29,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
unrollRecursionContexts(_parentctx);
}
return _localctx;
}
public static class HtailContext extends ParserRuleContext {
public List SPACE() { return getTokens(ProgramParser.SPACE); }
public TerminalNode SPACE(int i) {
return getToken(ProgramParser.SPACE, i);
}
public List head() {
return getRuleContexts(HeadContext.class);
}
public HeadContext head(int i) {
return getRuleContext(HeadContext.class,i);
}
public List application() {
return getRuleContexts(ApplicationContext.class);
}
public ApplicationContext application(int i) {
return getRuleContext(ApplicationContext.class,i);
}
public List method() {
return getRuleContexts(MethodContext.class);
}
public MethodContext method(int i) {
return getRuleContext(MethodContext.class,i);
}
public List LB() { return getTokens(ProgramParser.LB); }
public TerminalNode LB(int i) {
return getToken(ProgramParser.LB, i);
}
public List RB() { return getTokens(ProgramParser.RB); }
public TerminalNode RB(int i) {
return getToken(ProgramParser.RB, i);
}
public List has() {
return getRuleContexts(HasContext.class);
}
public HasContext has(int i) {
return getRuleContext(HasContext.class,i);
}
public List suffix() {
return getRuleContexts(SuffixContext.class);
}
public SuffixContext suffix(int i) {
return getRuleContext(SuffixContext.class,i);
}
public List anonymous() {
return getRuleContexts(AnonymousContext.class);
}
public AnonymousContext anonymous(int i) {
return getRuleContext(AnonymousContext.class,i);
}
public HtailContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_htail; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterHtail(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitHtail(this);
}
}
public final HtailContext htail() throws RecognitionException {
HtailContext _localctx = new HtailContext(_ctx, getState());
enterRule(_localctx, 28, RULE_htail);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(213);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
setState(213);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,30,_ctx) ) {
case 1:
{
setState(192);
match(SPACE);
setState(193);
head();
}
break;
case 2:
{
setState(194);
match(SPACE);
setState(195);
application(0);
setState(196);
method();
}
break;
case 3:
{
setState(198);
match(SPACE);
setState(199);
match(LB);
setState(200);
application(0);
setState(201);
match(RB);
}
break;
case 4:
{
setState(203);
match(SPACE);
setState(204);
application(0);
setState(205);
has();
}
break;
case 5:
{
setState(207);
match(SPACE);
setState(208);
application(0);
setState(209);
suffix();
}
break;
case 6:
{
setState(211);
match(SPACE);
setState(212);
anonymous();
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(215);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,31,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class HeadContext extends ParserRuleContext {
public TerminalNode AT() { return getToken(ProgramParser.AT, 0); }
public TerminalNode PARENT() { return getToken(ProgramParser.PARENT, 0); }
public TerminalNode SELF() { return getToken(ProgramParser.SELF, 0); }
public TerminalNode STAR() { return getToken(ProgramParser.STAR, 0); }
public TerminalNode NAME() { return getToken(ProgramParser.NAME, 0); }
public TerminalNode DOT() { return getToken(ProgramParser.DOT, 0); }
public DataContext data() {
return getRuleContext(DataContext.class,0);
}
public HeadContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_head; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterHead(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitHead(this);
}
}
public final HeadContext head() throws RecognitionException {
HeadContext _localctx = new HeadContext(_ctx, getState());
enterRule(_localctx, 30, RULE_head);
try {
setState(225);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,32,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(217);
match(AT);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(218);
match(PARENT);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(219);
match(SELF);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(220);
match(STAR);
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(221);
match(NAME);
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(222);
match(NAME);
setState(223);
match(DOT);
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(224);
data();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class HasContext extends ParserRuleContext {
public TerminalNode COLON() { return getToken(ProgramParser.COLON, 0); }
public TerminalNode NAME() { return getToken(ProgramParser.NAME, 0); }
public HasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_has; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterHas(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitHas(this);
}
}
public final HasContext has() throws RecognitionException {
HasContext _localctx = new HasContext(_ctx, getState());
enterRule(_localctx, 32, RULE_has);
try {
enterOuterAlt(_localctx, 1);
{
setState(227);
match(COLON);
setState(228);
match(NAME);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DataContext extends ParserRuleContext {
public TerminalNode BYTES() { return getToken(ProgramParser.BYTES, 0); }
public TerminalNode BOOL() { return getToken(ProgramParser.BOOL, 0); }
public TerminalNode STRING() { return getToken(ProgramParser.STRING, 0); }
public TerminalNode INT() { return getToken(ProgramParser.INT, 0); }
public TerminalNode FLOAT() { return getToken(ProgramParser.FLOAT, 0); }
public TerminalNode HEX() { return getToken(ProgramParser.HEX, 0); }
public TerminalNode CHAR() { return getToken(ProgramParser.CHAR, 0); }
public TerminalNode REGEX() { return getToken(ProgramParser.REGEX, 0); }
public DataContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_data; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).enterData(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ProgramListener ) ((ProgramListener)listener).exitData(this);
}
}
public final DataContext data() throws RecognitionException {
DataContext _localctx = new DataContext(_ctx, getState());
enterRule(_localctx, 34, RULE_data);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(230);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << REGEX) | (1L << BYTES) | (1L << BOOL) | (1L << CHAR) | (1L << STRING) | (1L << INT) | (1L << FLOAT) | (1L << HEX))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 13:
return application_sempred((ApplicationContext)_localctx, predIndex);
}
return true;
}
private boolean application_sempred(ApplicationContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return precpred(_ctx, 4);
case 1:
return precpred(_ctx, 2);
case 2:
return precpred(_ctx, 1);
}
return true;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\"\u00eb\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\3\2\5\2(\n\2\3\2\5\2+\n\2\3\2\3\2\3\2\3\3\3\3\6\3\62\n\3\r"+
"\3\16\3\63\3\4\3\4\6\48\n\4\r\4\16\49\3\5\3\5\7\5>\n\5\f\5\16\5A\13\5"+
"\3\5\3\5\3\5\6\5F\n\5\r\5\16\5G\3\6\3\6\3\6\5\6M\n\6\3\6\5\6P\n\6\3\6"+
"\3\6\3\6\5\6U\n\6\3\6\5\6X\n\6\3\6\5\6[\n\6\7\6]\n\6\f\6\16\6`\13\6\5"+
"\6b\n\6\3\7\3\7\3\7\3\b\3\b\7\bi\n\b\f\b\16\bl\13\b\3\b\3\b\3\b\3\b\3"+
"\b\5\bs\n\b\5\bu\n\b\3\t\3\t\3\t\3\t\7\t{\n\t\f\t\16\t~\13\t\5\t\u0080"+
"\n\t\3\t\3\t\3\n\3\n\3\13\3\13\3\13\5\13\u0089\n\13\5\13\u008b\n\13\3"+
"\f\3\f\3\f\3\f\3\f\6\f\u0092\n\f\r\f\16\f\u0093\3\f\3\f\3\r\3\r\3\r\3"+
"\r\3\r\5\r\u009d\n\r\3\16\3\16\3\16\3\17\3\17\3\17\5\17\u00a5\n\17\3\17"+
"\3\17\3\17\3\17\5\17\u00ab\n\17\5\17\u00ad\n\17\3\17\3\17\3\17\5\17\u00b2"+
"\n\17\3\17\3\17\3\17\5\17\u00b7\n\17\3\17\3\17\3\17\5\17\u00bc\n\17\7"+
"\17\u00be\n\17\f\17\16\17\u00c1\13\17\3\20\3\20\3\20\3\20\3\20\3\20\3"+
"\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3"+
"\20\6\20\u00d8\n\20\r\20\16\20\u00d9\3\21\3\21\3\21\3\21\3\21\3\21\3\21"+
"\3\21\5\21\u00e4\n\21\3\22\3\22\3\22\3\23\3\23\3\23\2\3\34\24\2\4\6\b"+
"\n\f\16\20\22\24\26\30\32\34\36 \"$\2\4\4\2\26\26 \4\2\5\5\31\37\2\u0103"+
"\2\'\3\2\2\2\4\61\3\2\2\2\6\67\3\2\2\2\bE\3\2\2\2\na\3\2\2\2\fc\3\2\2"+
"\2\16j\3\2\2\2\20v\3\2\2\2\22\u0083\3\2\2\2\24\u008a\3\2\2\2\26\u008c"+
"\3\2\2\2\30\u0097\3\2\2\2\32\u009e\3\2\2\2\34\u00ac\3\2\2\2\36\u00d7\3"+
"\2\2\2 \u00e3\3\2\2\2\"\u00e5\3\2\2\2$\u00e8\3\2\2\2&(\5\4\3\2\'&\3\2"+
"\2\2\'(\3\2\2\2(*\3\2\2\2)+\5\6\4\2*)\3\2\2\2*+\3\2\2\2+,\3\2\2\2,-\5"+
"\b\5\2-.\7\2\2\3.\3\3\2\2\2/\60\7\3\2\2\60\62\7\30\2\2\61/\3\2\2\2\62"+
"\63\3\2\2\2\63\61\3\2\2\2\63\64\3\2\2\2\64\5\3\2\2\2\65\66\7\4\2\2\66"+
"8\7\30\2\2\67\65\3\2\2\289\3\2\2\29\67\3\2\2\29:\3\2\2\2:\7\3\2\2\2;<"+
"\7\3\2\2<>\7\30\2\2=;\3\2\2\2>A\3\2\2\2?=\3\2\2\2?@\3\2\2\2@B\3\2\2\2"+
"A?\3\2\2\2BC\5\n\6\2CD\7\30\2\2DF\3\2\2\2E?\3\2\2\2FG\3\2\2\2GE\3\2\2"+
"\2GH\3\2\2\2H\t\3\2\2\2Ib\5\f\7\2JM\5\16\b\2KM\5\34\17\2LJ\3\2\2\2LK\3"+
"\2\2\2MO\3\2\2\2NP\5\26\f\2ON\3\2\2\2OP\3\2\2\2P^\3\2\2\2QR\7\30\2\2R"+
"T\5\32\16\2SU\5\36\20\2TS\3\2\2\2TU\3\2\2\2UW\3\2\2\2VX\5\30\r\2WV\3\2"+
"\2\2WX\3\2\2\2XZ\3\2\2\2Y[\5\26\f\2ZY\3\2\2\2Z[\3\2\2\2[]\3\2\2\2\\Q\3"+
"\2\2\2]`\3\2\2\2^\\\3\2\2\2^_\3\2\2\2_b\3\2\2\2`^\3\2\2\2aI\3\2\2\2aL"+
"\3\2\2\2b\13\3\2\2\2cd\5\20\t\2de\5\36\20\2e\r\3\2\2\2fg\7\3\2\2gi\7\30"+
"\2\2hf\3\2\2\2il\3\2\2\2jh\3\2\2\2jk\3\2\2\2km\3\2\2\2lj\3\2\2\2mt\5\20"+
"\t\2nr\5\30\r\2op\7\17\2\2pq\7\t\2\2qs\7 \2\2ro\3\2\2\2rs\3\2\2\2su\3"+
"\2\2\2tn\3\2\2\2tu\3\2\2\2u\17\3\2\2\2v\177\7\21\2\2w|\5\22\n\2xy\7\17"+
"\2\2y{\5\22\n\2zx\3\2\2\2{~\3\2\2\2|z\3\2\2\2|}\3\2\2\2}\u0080\3\2\2\2"+
"~|\3\2\2\2\177w\3\2\2\2\177\u0080\3\2\2\2\u0080\u0081\3\2\2\2\u0081\u0082"+
"\7\22\2\2\u0082\21\3\2\2\2\u0083\u0084\5\24\13\2\u0084\23\3\2\2\2\u0085"+
"\u008b\7\25\2\2\u0086\u0088\7 \2\2\u0087\u0089\7\7\2\2\u0088\u0087\3\2"+
"\2\2\u0088\u0089\3\2\2\2\u0089\u008b\3\2\2\2\u008a\u0085\3\2\2\2\u008a"+
"\u0086\3\2\2\2\u008b\25\3\2\2\2\u008c\u008d\7\30\2\2\u008d\u0091\7!\2"+
"\2\u008e\u008f\5\n\6\2\u008f\u0090\7\30\2\2\u0090\u0092\3\2\2\2\u0091"+
"\u008e\3\2\2\2\u0092\u0093\3\2\2\2\u0093\u0091\3\2\2\2\u0093\u0094\3\2"+
"\2\2\u0094\u0095\3\2\2\2\u0095\u0096\7\"\2\2\u0096\27\3\2\2\2\u0097\u0098"+
"\7\17\2\2\u0098\u0099\7\13\2\2\u0099\u009a\7\17\2\2\u009a\u009c\5\24\13"+
"\2\u009b\u009d\7\b\2\2\u009c\u009b\3\2\2\2\u009c\u009d\3\2\2\2\u009d\31"+
"\3\2\2\2\u009e\u009f\7\20\2\2\u009f\u00a0\t\2\2\2\u00a0\33\3\2\2\2\u00a1"+
"\u00a2\b\17\1\2\u00a2\u00a4\5 \21\2\u00a3\u00a5\5\36\20\2\u00a4\u00a3"+
"\3\2\2\2\u00a4\u00a5\3\2\2\2\u00a5\u00ad\3\2\2\2\u00a6\u00a7\7\23\2\2"+
"\u00a7\u00a8\5\34\17\2\u00a8\u00aa\7\24\2\2\u00a9\u00ab\5\36\20\2\u00aa"+
"\u00a9\3\2\2\2\u00aa\u00ab\3\2\2\2\u00ab\u00ad\3\2\2\2\u00ac\u00a1\3\2"+
"\2\2\u00ac\u00a6\3\2\2\2\u00ad\u00bf\3\2\2\2\u00ae\u00af\f\6\2\2\u00af"+
"\u00b1\5\32\16\2\u00b0\u00b2\5\36\20\2\u00b1\u00b0\3\2\2\2\u00b1\u00b2"+
"\3\2\2\2\u00b2\u00be\3\2\2\2\u00b3\u00b4\f\4\2\2\u00b4\u00b6\5\"\22\2"+
"\u00b5\u00b7\5\36\20\2\u00b6\u00b5\3\2\2\2\u00b6\u00b7\3\2\2\2\u00b7\u00be"+
"\3\2\2\2\u00b8\u00b9\f\3\2\2\u00b9\u00bb\5\30\r\2\u00ba\u00bc\5\36\20"+
"\2\u00bb\u00ba\3\2\2\2\u00bb\u00bc\3\2\2\2\u00bc\u00be\3\2\2\2\u00bd\u00ae"+
"\3\2\2\2\u00bd\u00b3\3\2\2\2\u00bd\u00b8\3\2\2\2\u00be\u00c1\3\2\2\2\u00bf"+
"\u00bd\3\2\2\2\u00bf\u00c0\3\2\2\2\u00c0\35\3\2\2\2\u00c1\u00bf\3\2\2"+
"\2\u00c2\u00c3\7\17\2\2\u00c3\u00d8\5 \21\2\u00c4\u00c5\7\17\2\2\u00c5"+
"\u00c6\5\34\17\2\u00c6\u00c7\5\32\16\2\u00c7\u00d8\3\2\2\2\u00c8\u00c9"+
"\7\17\2\2\u00c9\u00ca\7\23\2\2\u00ca\u00cb\5\34\17\2\u00cb\u00cc\7\24"+
"\2\2\u00cc\u00d8\3\2\2\2\u00cd\u00ce\7\17\2\2\u00ce\u00cf\5\34\17\2\u00cf"+
"\u00d0\5\"\22\2\u00d0\u00d8\3\2\2\2\u00d1\u00d2\7\17\2\2\u00d2\u00d3\5"+
"\34\17\2\u00d3\u00d4\5\30\r\2\u00d4\u00d8\3\2\2\2\u00d5\u00d6\7\17\2\2"+
"\u00d6\u00d8\5\f\7\2\u00d7\u00c2\3\2\2\2\u00d7\u00c4\3\2\2\2\u00d7\u00c8"+
"\3\2\2\2\u00d7\u00cd\3\2\2\2\u00d7\u00d1\3\2\2\2\u00d7\u00d5\3\2\2\2\u00d8"+
"\u00d9\3\2\2\2\u00d9\u00d7\3\2\2\2\u00d9\u00da\3\2\2\2\u00da\37\3\2\2"+
"\2\u00db\u00e4\7\25\2\2\u00dc\u00e4\7\26\2\2\u00dd\u00e4\7\f\2\2\u00de"+
"\u00e4\7\6\2\2\u00df\u00e4\7 \2\2\u00e0\u00e1\7 \2\2\u00e1\u00e4\7\20"+
"\2\2\u00e2\u00e4\5$\23\2\u00e3\u00db\3\2\2\2\u00e3\u00dc\3\2\2\2\u00e3"+
"\u00dd\3\2\2\2\u00e3\u00de\3\2\2\2\u00e3\u00df\3\2\2\2\u00e3\u00e0\3\2"+
"\2\2\u00e3\u00e2\3\2\2\2\u00e4!\3\2\2\2\u00e5\u00e6\7\n\2\2\u00e6\u00e7"+
"\7 \2\2\u00e7#\3\2\2\2\u00e8\u00e9\t\3\2\2\u00e9%\3\2\2\2#\'*\639?GLO"+
"TWZ^ajrt|\177\u0088\u008a\u0093\u009c\u00a4\u00aa\u00ac\u00b1\u00b6\u00bb"+
"\u00bd\u00bf\u00d7\u00d9\u00e3";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}