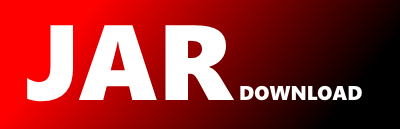
io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudio Maven / Gradle / Ivy
Show all versions of cdk-emrserverless-quickdemo-with-delta-lake Show documentation
package io.github.hsiehshujeng.cdk.emrserverless.quickdemo;
/**
* Creates an EMR Studio for EMR Serverless applications.
*
* The Studio is not only for EMR Serverless applications but also for launching an EMR cluster via a cluster template created in this constrcut to check out results transformed by EMR serverless applications.
*
* For what Studio can do further, please refer to Amazon EMR Studio.
*
*
* const workspaceBucket = new WorkSpaceBucket(this, 'EmrStudio');
* const emrStudio = new EmrStudio(this, '', {
* workSpaceBucket: workspaceBucket,
* subnetIds: ['subnet1', 'subnet2', 'subnet3']
* });
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.105.0 (build 0a2adcb)", date = "2024-12-02T01:11:38.399Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = io.github.hsiehshujeng.cdk.emrserverless.quickdemo.$Module.class, fqn = "cdk-emrserverless-with-delta-lake.EmrStudio")
public class EmrStudio extends software.constructs.Construct {
protected EmrStudio(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected EmrStudio(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param name This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public EmrStudio(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String name, final @org.jetbrains.annotations.NotNull io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudioProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(name, "name is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.emr.CfnStudio getEntity() {
return software.amazon.jsii.Kernel.get(this, "entity", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.emr.CfnStudio.class));
}
/**
* A fluent builder for {@link io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudio}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param name This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String name) {
return new Builder(scope, name);
}
private final software.constructs.Construct scope;
private final java.lang.String name;
private final io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudioProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String name) {
this.scope = scope;
this.name = name;
this.props = new io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudioProps.Builder();
}
/**
* The custom construct as the workspace S3 bucket.
*
* @return {@code this}
* @param workSpaceBucket The custom construct as the workspace S3 bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workSpaceBucket(final io.github.hsiehshujeng.cdk.emrserverless.quickdemo.WorkSpaceBucket workSpaceBucket) {
this.props.workSpaceBucket(workSpaceBucket);
return this;
}
/**
* Specifies whether the Studio authenticates users using AWS SSO or IAM.
*
* Default: - StudioAuthMode.AWS_IAM.
*
* @return {@code this}
* @param authMode Specifies whether the Studio authenticates users using AWS SSO or IAM. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authMode(final io.github.hsiehshujeng.cdk.emrserverless.quickdemo.StudioAuthMode authMode) {
this.props.authMode(authMode);
return this;
}
/**
* A detailed description of the Amazon EMR Studio.
*
* Default: - 'EMR Studio Quick Launch - by scott.hsieh'
*
* @return {@code this}
* @param description A detailed description of the Amazon EMR Studio. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The ID of the Amazon EMR Studio Engine security group.
*
* The Engine security group allows inbound network traffic from the Workspace security group, and it must be in the same VPC specified by VpcId.
*
* Default: - a security group created by `EmrStudioEngineSecurityGroup`.
*
* @return {@code this}
* @param engineSecurityGroupId The ID of the Amazon EMR Studio Engine security group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder engineSecurityGroupId(final java.lang.String engineSecurityGroupId) {
this.props.engineSecurityGroupId(engineSecurityGroupId);
return this;
}
/**
* Options for which kind of identity will be associated with the Product of the Porfolio in AWS Service Catalog for EMR cluster templates.
*
* You can choose either an IAM group, IAM role, or IAM user. If you leave it empty, an IAM user named Administrator
with the AdministratorAccess
power needs to be created first.
*
* @return {@code this}
* @param serviceCatalogProps Options for which kind of identity will be associated with the Product of the Porfolio in AWS Service Catalog for EMR cluster templates. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceCatalogProps(final io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudioDeveloperStackProps serviceCatalogProps) {
this.props.serviceCatalogProps(serviceCatalogProps);
return this;
}
/**
* @return {@code this}
* @param serviceRoleArn This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceRoleArn(final java.lang.String serviceRoleArn) {
this.props.serviceRoleArn(serviceRoleArn);
return this;
}
/**
* A name for the service role of an EMR Studio.
*
* For valid values, see the RoleName parameter for the CreateRole action in the IAM API Reference.
*
* IMPORTANT: If you specify a name, you cannot perform updates that require replacement of this resource. You can perform updates that require no or some interruption. If you must replace the resource, specify a new name.
*
* If you specify a name, you must specify the CAPABILITY_NAMED_IAM value to acknowledge your template's capabilities. For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
* Default: - 'emr-studio-service-role'
*
* @return {@code this}
* @param serviceRoleName A name for the service role of an EMR Studio. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceRoleName(final java.lang.String serviceRoleName) {
this.props.serviceRoleName(serviceRoleName);
return this;
}
/**
* A descriptive name for the Amazon EMR Studio.
*
* Default: - 'emr-sutdio-quicklaunch'
*
* @return {@code this}
* @param studioName A descriptive name for the Amazon EMR Studio. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder studioName(final java.lang.String studioName) {
this.props.studioName(studioName);
return this;
}
/**
* The subnet IDs for the EMR studio.
*
* You can select the subnets from the default VPC in your AWS account.
*
* @return {@code this}
* @param subnetIds The subnet IDs for the EMR studio. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnetIds(final java.util.List subnetIds) {
this.props.subnetIds(subnetIds);
return this;
}
/**
* The custom user role for the EMR Studio when authentication is AWS SSO.
*
* Currently, if you choose to establish an EMR serverless application where the authentication mechanism used by the EMR Studio is AWS SSO, you need to create a user role by yourself and assign the role arn to this argument if AWS SSO is chosen as authentication for the EMR Studio;
*
* @return {@code this}
* @param userRoleArn The custom user role for the EMR Studio when authentication is AWS SSO. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userRoleArn(final java.lang.String userRoleArn) {
this.props.userRoleArn(userRoleArn);
return this;
}
/**
* Used by the EMR Studio.
*
* Default: - 'The default VPC will be used.'
*
* @return {@code this}
* @param vpcId Used by the EMR Studio. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcId(final java.lang.String vpcId) {
this.props.vpcId(vpcId);
return this;
}
/**
* The ID of the security group used by the workspace.
*
* Default: - a security group created by `EmrStudioWorkspaceSecurityGroup`.
*
* @return {@code this}
* @param workSpaceSecurityGroupId The ID of the security group used by the workspace. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workSpaceSecurityGroupId(final java.lang.String workSpaceSecurityGroupId) {
this.props.workSpaceSecurityGroupId(workSpaceSecurityGroupId);
return this;
}
/**
* @return a newly built instance of {@link io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudio}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudio build() {
return new io.github.hsiehshujeng.cdk.emrserverless.quickdemo.EmrStudio(
this.scope,
this.name,
this.props.build()
);
}
}
}