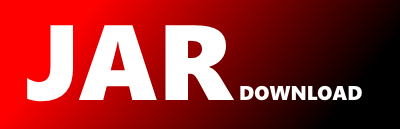
com.hundsun.lightdb.unisql.proxy.jdbc.UnisqlConnection Maven / Gradle / Ivy
package com.hundsun.lightdb.unisql.proxy.jdbc;
import com.hundsun.lightdb.unisql.golang.Transformer;
import com.hundsun.lightdb.unisql.golang.VariableParameter;
import com.hundsun.lightdb.unisql.model.UnisqlProperties;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
import java.sql.Array;
import java.sql.Blob;
import java.sql.CallableStatement;
import java.sql.Clob;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.NClob;
import java.sql.PreparedStatement;
import java.sql.SQLClientInfoException;
import java.sql.SQLException;
import java.sql.SQLWarning;
import java.sql.SQLXML;
import java.sql.Savepoint;
import java.sql.Statement;
import java.sql.Struct;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.Executor;
/**
* @author zhuxd
* @date 2023/1/30
*/
@Getter
@ToString
@EqualsAndHashCode
@Slf4j
@SuppressFBWarnings({"SQL_INJECTION_JDBC", "EXTERNAL_CONFIG_CONTROL"})
public class UnisqlConnection implements Connection {
public static final String ORACLE_JDBC_DRIVER_ORACLE_CONNECTION = "oracle.jdbc.driver.OracleConnection";
private final DbType sourceDialect;
private final DbType targetDialect;
private final String dataBase;
@Override
public Statement createStatement() throws SQLException {
return new UnisqlStatement(this, delegate.createStatement());
}
@Override
public PreparedStatement prepareStatement(String sql) throws SQLException {
return new UnisqlPreparedStatement(this, delegate.prepareStatement(transformSql(sql, true)), sql);
}
public String transformSql(String sql) {
return transformSql(sql, false);
}
private String transformSql(String sql, boolean isPrecompiled) {
long start = System.nanoTime();
String transform;
// http发送sql到`比对中心`服务
if (Transformer.IS_COMPARE) {
// 跳过`统一sql运行时sql转换`步骤,原因:内部客户uf30是多套测试环境,不需要在运行时候进行sql转换(由一种sql方言转换为另一种方言)
if (UnisqlProperties.skipUnisqlRuntimeConvertProperty) {
return sql;
}
// 比对服务开启时,预编译的SQL会调用两次;第一次预编译sql时,此参数为1 不调用比对服务,第二次获取到绑定变量值后,此参数为0 调用比对服务。
VariableParameter variableParameter = VariableParameter.builder().dbName(dataBase).build();
if (isPrecompiled) {
variableParameter.setIsPrecompiled(1);
}
transform = Transformer.parse(sql, sourceDialect.name(), targetDialect.name(), variableParameter);
} else {
transform = Transformer.parse(sql, sourceDialect.name(), targetDialect.name());
}
long cost = System.nanoTime() - start;
if (log.isDebugEnabled()) {
log.debug("[UNISQL][transformSql]cost:{} ns. Source db is {}, Target db is {}, From:\n{}\nTo:\n{}. ", cost,
sourceDialect.name(), targetDialect.name(), sql, transform);
}
return transform;
}
@Override
public CallableStatement prepareCall(String sql) throws SQLException {
throw new SQLException("not implemented");
// return delegate.prepareCall(sql);
}
@Override
public String nativeSQL(String sql) throws SQLException {
return delegate.nativeSQL(sql);
}
@Override
public void setAutoCommit(boolean autoCommit) throws SQLException {
delegate.setAutoCommit(autoCommit);
}
@Override
public boolean getAutoCommit() throws SQLException {
return delegate.getAutoCommit();
}
@Override
public void commit() throws SQLException {
delegate.commit();
}
@Override
public void rollback() throws SQLException {
delegate.rollback();
}
@Override
public void close() throws SQLException {
delegate.close();
}
@Override
public boolean isClosed() throws SQLException {
return delegate.isClosed();
}
@Override
public DatabaseMetaData getMetaData() throws SQLException {
return delegate.getMetaData();
}
@Override
public void setReadOnly(boolean readOnly) throws SQLException {
delegate.setReadOnly(readOnly);
}
@Override
public boolean isReadOnly() throws SQLException {
return delegate.isReadOnly();
}
@Override
public void setCatalog(String catalog) throws SQLException {
delegate.setCatalog(catalog);
}
@Override
public String getCatalog() throws SQLException {
return delegate.getCatalog();
}
@Override
public void setTransactionIsolation(int level) throws SQLException {
delegate.setTransactionIsolation(level);
}
@Override
public int getTransactionIsolation() throws SQLException {
return delegate.getTransactionIsolation();
}
@Override
public SQLWarning getWarnings() throws SQLException {
return delegate.getWarnings();
}
@Override
public void clearWarnings() throws SQLException {
delegate.clearWarnings();
}
@Override
public Statement createStatement(int resultSetType, int resultSetConcurrency) throws SQLException {
return new UnisqlStatement(this, delegate.createStatement(resultSetType, resultSetConcurrency));
}
@Override
public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency) throws SQLException {
return new UnisqlPreparedStatement(this,
delegate.prepareStatement(transformSql(sql, true), resultSetType, resultSetConcurrency), sql);
}
@Override
public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency) throws SQLException {
throw new SQLException("not implemented");
// return delegate.prepareCall(sql, resultSetType, resultSetConcurrency);
}
@Override
public Map> getTypeMap() throws SQLException {
return delegate.getTypeMap();
}
@Override
public void setTypeMap(Map> map) throws SQLException {
delegate.setTypeMap(map);
}
@Override
public void setHoldability(int holdability) throws SQLException {
delegate.setHoldability(holdability);
}
@Override
public int getHoldability() throws SQLException {
return delegate.getHoldability();
}
@Override
public Savepoint setSavepoint() throws SQLException {
return delegate.setSavepoint();
}
@Override
public Savepoint setSavepoint(String name) throws SQLException {
return delegate.setSavepoint(name);
}
@Override
public void rollback(Savepoint savepoint) throws SQLException {
delegate.rollback(savepoint);
}
@Override
public void releaseSavepoint(Savepoint savepoint) throws SQLException {
delegate.releaseSavepoint(savepoint);
}
@Override
public Statement createStatement(int resultSetType, int resultSetConcurrency, int resultSetHoldability) throws SQLException {
return new UnisqlStatement(this, delegate.createStatement(resultSetType, resultSetConcurrency,
resultSetHoldability));
}
@Override
public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException {
return new UnisqlPreparedStatement(this, delegate.prepareStatement(transformSql(sql, true), resultSetType,
resultSetConcurrency, resultSetHoldability), sql);
}
@Override
public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException {
throw new SQLException("not implemented");
// return delegate.prepareCall(sql, resultSetType, resultSetConcurrency, resultSetHoldability);
}
@Override
public PreparedStatement prepareStatement(String sql, int autoGeneratedKeys) throws SQLException {
return new UnisqlPreparedStatement(this, delegate.prepareStatement(transformSql(sql, true), autoGeneratedKeys), sql);
}
@Override
public PreparedStatement prepareStatement(String sql, int[] columnIndexes) throws SQLException {
return new UnisqlPreparedStatement(this, delegate.prepareStatement(transformSql(sql, true), columnIndexes), sql);
}
@Override
public PreparedStatement prepareStatement(String sql, String[] columnNames) throws SQLException {
return new UnisqlPreparedStatement(this, delegate.prepareStatement(transformSql(sql, true), columnNames), sql);
}
@Override
public Clob createClob() throws SQLException {
return delegate.createClob();
}
@Override
public Blob createBlob() throws SQLException {
return delegate.createBlob();
}
@Override
public NClob createNClob() throws SQLException {
return delegate.createNClob();
}
@Override
public SQLXML createSQLXML() throws SQLException {
return delegate.createSQLXML();
}
@Override
public boolean isValid(int timeout) throws SQLException {
return delegate.isValid(timeout);
}
@Override
public void setClientInfo(String name, String value) throws SQLClientInfoException {
delegate.setClientInfo(name, value);
}
@Override
public void setClientInfo(Properties properties) throws SQLClientInfoException {
delegate.setClientInfo(properties);
}
@Override
public String getClientInfo(String name) throws SQLException {
return delegate.getClientInfo(name);
}
@Override
public Properties getClientInfo() throws SQLException {
return delegate.getClientInfo();
}
@Override
public Array createArrayOf(String typeName, Object[] elements) throws SQLException {
return delegate.createArrayOf(typeName, elements);
}
@Override
public Struct createStruct(String typeName, Object[] attributes) throws SQLException {
return delegate.createStruct(typeName, attributes);
}
@Override
public void setSchema(String schema) throws SQLException {
delegate.setSchema(schema);
}
@Override
public String getSchema() throws SQLException {
return delegate.getSchema();
}
@Override
public void abort(Executor executor) throws SQLException {
delegate.abort(executor);
}
@Override
public void setNetworkTimeout(Executor executor, int milliseconds) throws SQLException {
delegate.setNetworkTimeout(executor, milliseconds);
}
@Override
public int getNetworkTimeout() throws SQLException {
return delegate.getNetworkTimeout();
}
@Override
public T unwrap(Class iface) throws SQLException {
return delegate.unwrap(iface);
}
@Override
public boolean isWrapperFor(Class> iface) throws SQLException {
/**
* 1 兼容研发中心本地缓存组件(localcache)版本(1.1.32.1)代码中方法java.sql.Wrapper.isWrapperFor入参类型`oracle.jdbc.driver.OracleConnection.class`(这是个抽象类)
* 2 delegate.isWrapperFor(`oracle.jdbc.driver.OracleConnection.class`)会抛出异常`java.sql.SQLException: Object does not wrap anything with requested interface(对象不使用请求的接口包装任何内容)
* at oracle.jdbc.driver.OracleConnection.isWrapperFor(OracleConnection.java:260) ~[ojdbc8-19.3.0.0.jar!/:19.3.0.0.0]`
* @Author: liangdong30629
* @Date: 2024/7/10 10:27
*/
if (ORACLE_JDBC_DRIVER_ORACLE_CONNECTION.equals(iface.getName())) {
// 参考数据源hikari判断逻辑:com.zaxxer.hikari.pool.ProxyConnection.isWrapperFor
return iface.isInstance(delegate);
}
return delegate.isWrapperFor(iface);
}
private final Connection delegate;
public UnisqlConnection(Connection delegate, DbType sourceDialect, DbType targetDialect, String dataBase) {
this.delegate = delegate;
this.sourceDialect = sourceDialect;
this.targetDialect = targetDialect;
this.dataBase = dataBase;
}
public Connection getDelegate() {
return delegate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy