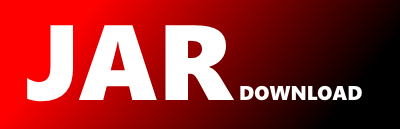
com.hundsun.lightdb.unisql.utils.ExecuteUtils Maven / Gradle / Ivy
package com.hundsun.lightdb.unisql.utils;
import com.hundsun.lightdb.unisql.model.SshResponse;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
@Slf4j
public class ExecuteUtils {
public static final int STATUS_SUCCESS = 0;
public static final int STATUS_ERROR = 1;
public static SshResponse execResult(String cmd) {
BufferedReader inputBufferedReader = null;
BufferedReader errorBufferedReader = null;
String outputTrue = "";
String outputFalse = "";
int waitFor = STATUS_SUCCESS;
try {
/* 调用、输出 */
Process process = Runtime.getRuntime().exec(cmd);
inputBufferedReader = new BufferedReader(new InputStreamReader(process.getInputStream()));
errorBufferedReader = new BufferedReader(new InputStreamReader(process.getErrorStream()));
String line = null;
// 正常信息
while ((line = inputBufferedReader.readLine()) != null) {
outputTrue = outputTrue + line + System.lineSeparator();
}
if(outputTrue!= null && !outputTrue.isEmpty()){
outputTrue = StringUtils.substringBeforeLast(outputTrue,"\n");
}
// 错误信息
while ((line = errorBufferedReader.readLine()) != null) {
outputFalse = outputFalse + line + System.lineSeparator();
}
/* 判断是否正常 */
waitFor = process.waitFor();
} catch (Exception e) {
if (log.isErrorEnabled()) {
log.error(e.getMessage(), e);
}
return new SshResponse(outputTrue, e.getMessage(), STATUS_ERROR);
} finally {
if (inputBufferedReader != null) {
try {
inputBufferedReader.close();
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error(e.getMessage(), e);
}
}
}
}
if (waitFor != STATUS_SUCCESS && StringUtils.isBlank(outputFalse)) {
outputFalse = outputTrue;
}
return new SshResponse(outputTrue, outputFalse, waitFor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy