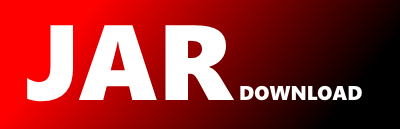
com.hundsun.lightdb.unisql.utils.MicroServiceParameterUtils Maven / Gradle / Ivy
package com.hundsun.lightdb.unisql.utils;
import com.hundsun.jrescloud.common.t2.event.IEvent;
import com.hundsun.jrescloud.common.util.ConfigUtils;
import com.hundsun.jrescloud.common.util.SpringUtils;
import com.hundsun.jrescloud.rpc.api.IRpcContext;
import com.hundsun.jrescloud.trace.util.TraceAdapterUtil;
import com.hundsun.lightdb.unisql.golang.Transformer;
import com.hundsun.lightdb.unisql.golang.VariableParameter;
import com.hundsun.lightdb.unisql.model.UnisqlProperties;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import java.util.Optional;
/**
* @program: sql-convert-runtime
* @description: 采集微服务运行时参数的工具类
* @author: liangdong30629
* @date: 2024-06-12 14:28
**/
@Slf4j
public class MicroServiceParameterUtils {
/**
* 类数组,需要检测这些类存在或者不存在
*
* @Author: liangdong30629
* @Date: 2024/6/12 14:40
*/
private static final Class>[] CLASS_TO_CHECK_ARRAY = new Class>[]{TraceAdapterUtil.class, ConfigUtils.class,
IRpcContext.class};
/**
* 表示类数组({@link MicroServiceParameterUtils#CLASS_TO_CHECK_ARRAY})中的类是否存在,true:存在,false:不存在
*/
private static boolean classPreCheckPassed;
static {
/**
* 类数组({@link MicroServiceParameterUtils#CLASS_TO_CHECK_ARRAY})中类是否均存在
* @Author: liangdong30629
* @Date: 2024/6/12 15:04
*/
classPreCheckPassed = checkClassExists();
}
/**
* `采集微服务`参数开关{@link UnisqlProperties#collectMicroServiceParametersEnabled}打开的情况下,采集`trace_id`、`微服务名称`、`方法名`和`sql
* 执行顺序`(sql执行顺序参考{@link SqlSequenceUtils#nextSequenceId(String)})
*
* @param: variableParameter 该对象用于暂存`trace_id`、`微服务名称`、`方法名`和`sql执行顺序`
* @return: void
* @Author: liangdong30629
* @Date: 2024/6/12 13:04
*/
public static void collectMicroServiceParameters(VariableParameter variableParameter) {
// 获取微服务信息所需的类已存在
if (classPreCheckPassed) {
VariableParameter vp = Transformer.VARIABLE_PARAMETER_THREAD_LOCAL.get();
String currentTraceId = StringUtils.isNotEmpty(vp.getCurrentTraceId()) ? vp.getCurrentTraceId() : TraceAdapterUtil.getCurrentTraceId();
// trace_id
variableParameter.setCurrentTraceId(currentTraceId);
// 微服务名称
variableParameter.setAppName(StringUtils.isNotEmpty(vp.getAppName()) ? vp.getAppName() : ConfigUtils.getAppName());
// 微服务功能号(对应微服务内接口方法)
variableParameter.setFunctionId(StringUtils.isNotEmpty(vp.getFunctionId()) ? vp.getFunctionId() : getFunctionID());
// sql执行顺序
variableParameter.setSqlSequence(SqlSequenceUtils.nextSequenceId(StringUtils.isBlank(currentTraceId) ? "" : currentTraceId));
}
}
/**
* 检测类数组({@link MicroServiceParameterUtils#CLASS_TO_CHECK_ARRAY})中的这些类是否存在
*
* @param: []
* @return: boolean
* @Author: liangdong30629
* @Date: 2024/6/12 14:55
*/
private static boolean checkClassExists() {
for (Class> aClass : CLASS_TO_CHECK_ARRAY) {
// 类不存在
if (!isClassExists(aClass.getName())) {
return false;
}
}
return true;
}
/**
* 判断一个类存在不存在
*
* @param className 类名称
* @return true:类存在,false:类不存在
*/
public static boolean isClassExists(String className) {
try {
Class.forName(className);
return true;
} catch (ClassNotFoundException e) {
if (log.isErrorEnabled()) {
log.error("采集微服务信息所需类{}不存在", className, e);
}
return false;
}
}
/**
* 返回微服务功能号,对应t3协议5号域{@linkplain https://iknow.hs.net/portal/docView/home/4423}
*
* @param: []
* @return: java.lang.String
* @Author: liangdong30629
* @Date: 2024/6/12 14:30
*/
private static String getFunctionID() {
IEvent event = SpringUtils.getBean(IRpcContext.class).getEvent();
// event为null
if (!Optional.ofNullable(event).isPresent()) {
return null;
}
String functionID = event.getStringAttributeValue("5");
if (StringUtils.isBlank(functionID)) {
return null;
}
return functionID;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy