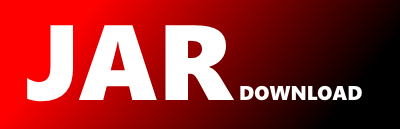
com.hundsun.lightdb.unisql.utils.Utils Maven / Gradle / Ivy
/*
* Copyright 1999-2018 Alibaba Group Holding Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hundsun.lightdb.unisql.utils;
import javax.sql.rowset.serial.SerialBlob;
import javax.sql.rowset.serial.SerialClob;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStreamWriter;
import java.io.Reader;
import java.io.StringReader;
import java.io.Writer;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.SQLException;
import java.util.Base64;
/**
* @author zhuxd
* @date 2023/1/31
*/
public class Utils {
public static Class> loadClass(String className) {
Class> clazz = null;
if (className == null) {
return null;
}
try {
return Class.forName(className);
} catch (ClassNotFoundException e) {
// skip
}
ClassLoader ctxClassLoader = Thread.currentThread().getContextClassLoader();
if (ctxClassLoader != null) {
try {
clazz = ctxClassLoader.loadClass(className);
} catch (ClassNotFoundException e) {
// skip
}
}
return clazz;
}
/**
* 将 Clob 转换为 ByteArrayOutputStream
*
* @param clob
* @return ByteArrayOutputStream
*/
public static ByteArrayOutputStream clobToByteArrayOutputStream(Clob clob) throws IOException, SQLException {
try (Reader reader = clob.getCharacterStream();
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
Writer writer = new OutputStreamWriter(byteArrayOutputStream)) {
char[] buffer = new char[1024];
int bytesRead;
while ((bytesRead = reader.read(buffer)) != -1) {
writer.write(buffer, 0, bytesRead);
}
writer.flush();
return byteArrayOutputStream;
}
}
/**
* 将 Blob 转换为 ByteArrayOutputStream
*
* @param blob
* @return ByteArrayOutputStream
*/
public static ByteArrayOutputStream blobToByteArrayOutputStream(Blob blob) throws IOException, SQLException {
try (InputStream inputStream = blob.getBinaryStream();
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream()) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
byteArrayOutputStream.write(buffer, 0, bytesRead);
}
return byteArrayOutputStream;
}
}
/**
* 将 ByteArrayOutputStream 转换为 Base64 编码
*
* @param byteArrayOutputStream
* @return String
*/
public static String byteArrayOutputStreamToBase64(ByteArrayOutputStream byteArrayOutputStream) {
byte[] byteArray = byteArrayOutputStream.toByteArray();
return Base64.getEncoder().encodeToString(byteArray);
}
/**
* 将 Base64 编码转换为字节数组
*
* @param base64String
* @return byte[]
*/
public static byte[] base64ToByteArray(String base64String) {
return Base64.getDecoder().decode(base64String);
}
/**
* 将 Base64 编码转换为 Clob
*
* @param base64String
* @return Clob
*/
public static Clob base64ToClob(String base64String) throws SQLException {
// 解码 Base64 字符串
String decodedString = new String(base64ToByteArray(base64String));
// 使用 SerialClob 创建 Clob 对象
return new SerialClob(decodedString.toCharArray());
}
/**
* 将 Base64 编码转换为 Blob
*
* @param base64String
* @return Blob
*/
public static Blob base64ToBlob(String base64String) throws SQLException {
// 解码 Base64 字符串
// 使用 SerialBlob 创建 Blob 对象
return new SerialBlob(base64ToByteArray(base64String));
}
/**
* 将 Base64 编码转换为 InputStream
*
* @param base64String
* @return InputStream
*/
public static InputStream base64ToInputStream(String base64String) {
return new ByteArrayInputStream(base64ToByteArray(base64String));
}
/**
* 将 Base64 编码转换为 Reader
*
* @param base64String
* @return Reader
*/
public static Reader base64ToReader(String base64String) {
// 将字节数组转换为字符串
String str = new String(base64ToByteArray(base64String));
// 将字符串转换为 Reader 并返回
return new StringReader(str);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy