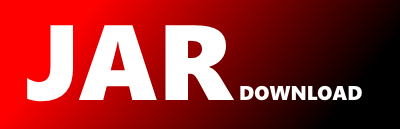
io.github.humbleui.skija.FontFeature Maven / Gradle / Ivy
// Generated by delombok at Wed Sep 14 01:50:49 UTC 2022
package io.github.humbleui.skija;
import lombok.experimental.Tolerate;
import java.util.Objects;
import java.util.regex.*;
public class FontFeature {
public final int _tag;
public final int _value;
public final long _start;
public final long _end;
public static final long GLOBAL_START = 0;
public static final long GLOBAL_END = Long.MAX_VALUE;
public static final FontFeature[] EMPTY = new FontFeature[0];
public FontFeature(String feature, int value, long start, long end) {
this(FourByteTag.fromString(feature), value, start, end);
}
public FontFeature(String feature, int value) {
this(FourByteTag.fromString(feature), value, GLOBAL_START, GLOBAL_END);
}
public FontFeature(String feature, boolean value) {
this(FourByteTag.fromString(feature), value ? 1 : 0, GLOBAL_START, GLOBAL_END);
}
public FontFeature(String feature) {
this(FourByteTag.fromString(feature), 1, GLOBAL_START, GLOBAL_END);
}
public String getTag() {
return FourByteTag.toString(_tag);
}
@Override
public String toString() {
String range = "";
if (_start > 0 || _end < Long.MAX_VALUE) {
range = "[" + (_start > 0 ? _start : "") + ":" + (_end < Long.MAX_VALUE ? _end : "") + "]";
}
String valuePrefix = "";
String valueSuffix = "";
if (_value == 0) valuePrefix = "-";
else if (_value == 1) valuePrefix = "+";
else valueSuffix = "=" + _value;
return "FontFeature(" + valuePrefix + getTag() + range + valueSuffix + ")";
}
public static final Pattern _splitPattern = Pattern.compile("\\s+");
public static final Pattern _featurePattern = Pattern.compile("(?[-+])?(?[a-z0-9]{4})(?:\\[(?\\d+)?:(?\\d+)?\\])?(?:=(?\\d+))?");
public static FontFeature parseOne(String s) {
Matcher m = _featurePattern.matcher(s);
if (!m.matches()) throw new IllegalArgumentException("Can’t parse FontFeature: " + s);
int value = m.group("value") != null ? Integer.parseInt(m.group("value")) : m.group("sign") == null ? 1 : "-".equals(m.group("sign")) ? 0 : 1;
long start = m.group("start") == null ? 0 : Long.parseLong(m.group("start"));
long end = m.group("end") == null ? Long.MAX_VALUE : Long.parseLong(m.group("end"));
return new FontFeature(m.group("tag"), value, start, end);
}
public static FontFeature[] parse(String s) {
return _splitPattern.splitAsStream(s).map(FontFeature::parseOne).toArray(FontFeature[]::new);
}
@java.lang.SuppressWarnings("all")
public FontFeature(final int tag, final int value, final long start, final long end) {
this._tag = tag;
this._value = value;
this._start = start;
this._end = end;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof FontFeature)) return false;
final FontFeature other = (FontFeature) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$_tag = this.getTag();
final java.lang.Object other$_tag = other.getTag();
if (this$_tag == null ? other$_tag != null : !this$_tag.equals(other$_tag)) return false;
if (this.getValue() != other.getValue()) return false;
if (this.getStart() != other.getStart()) return false;
if (this.getEnd() != other.getEnd()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof FontFeature;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $_tag = this.getTag();
result = result * PRIME + ($_tag == null ? 43 : $_tag.hashCode());
result = result * PRIME + this.getValue();
final long $_start = this.getStart();
result = result * PRIME + (int) ($_start >>> 32 ^ $_start);
final long $_end = this.getEnd();
result = result * PRIME + (int) ($_end >>> 32 ^ $_end);
return result;
}
@java.lang.SuppressWarnings("all")
public int getValue() {
return this._value;
}
@java.lang.SuppressWarnings("all")
public long getStart() {
return this._start;
}
@java.lang.SuppressWarnings("all")
public long getEnd() {
return this._end;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy