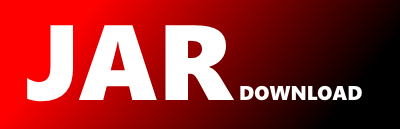
io.github.humbleui.skija.FontVariation Maven / Gradle / Ivy
// Generated by delombok at Wed Sep 14 01:50:49 UTC 2022
package io.github.humbleui.skija;
import java.util.regex.*;
import lombok.*;
import org.jetbrains.annotations.*;
public class FontVariation {
public static final FontVariation[] EMPTY = new FontVariation[0];
public final int _tag;
public final float _value;
public FontVariation(String feature, float value) {
this(FourByteTag.fromString(feature), value);
}
public String getTag() {
return FourByteTag.toString(_tag);
}
public String toString() {
return getTag() + "=" + _value;
}
@ApiStatus.Internal
public static final Pattern _splitPattern = Pattern.compile("\\s+");
@ApiStatus.Internal
public static final Pattern _variationPattern = Pattern.compile("(?[a-z0-9]{4})=(?\\d+)");
public static FontVariation parseOne(String s) {
Matcher m = _variationPattern.matcher(s);
if (!m.matches()) throw new IllegalArgumentException("Can’t parse FontVariation: " + s);
float value = Float.parseFloat(m.group("value"));
return new FontVariation(m.group("tag"), value);
}
public static FontVariation[] parse(String s) {
return _splitPattern.splitAsStream(s).map(FontVariation::parseOne).toArray(FontVariation[]::new);
}
@java.lang.SuppressWarnings("all")
public FontVariation(final int tag, final float value) {
this._tag = tag;
this._value = value;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof FontVariation)) return false;
final FontVariation other = (FontVariation) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$_tag = this.getTag();
final java.lang.Object other$_tag = other.getTag();
if (this$_tag == null ? other$_tag != null : !this$_tag.equals(other$_tag)) return false;
if (java.lang.Float.compare(this.getValue(), other.getValue()) != 0) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof FontVariation;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $_tag = this.getTag();
result = result * PRIME + ($_tag == null ? 43 : $_tag.hashCode());
result = result * PRIME + java.lang.Float.floatToIntBits(this.getValue());
return result;
}
@java.lang.SuppressWarnings("all")
public float getValue() {
return this._value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy