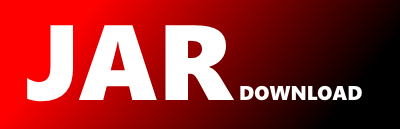
io.github.humbleui.skija.ImageInfo Maven / Gradle / Ivy
// Generated by delombok at Wed Sep 14 01:50:49 UTC 2022
package io.github.humbleui.skija;
import lombok.*;
import org.jetbrains.annotations.*;
import io.github.humbleui.skija.impl.*;
import io.github.humbleui.types.*;
/**
* Describes pixel dimensions and encoding. Bitmap, Image, Pixmap, and Surface
* can be created from ImageInfo. ImageInfo can be retrieved from Bitmap and
* Pixmap, but not from Image and Surface. For example, Image and Surface
* implementations may defer pixel depth, so may not completely specify ImageInfo.
*
* ImageInfo contains dimensions, the pixel integral width and height. It encodes
* how pixel bits describe alpha, transparency; color components red, blue,
* and green; and ColorSpace, the range and linearity of colors.
*/
public class ImageInfo {
public final ColorInfo _colorInfo;
public final int _width;
public final int _height;
public static final ImageInfo DEFAULT = new ImageInfo(ColorInfo.DEFAULT, 0, 0);
public ImageInfo(int width, int height, @NotNull ColorType colorType, @NotNull ColorAlphaType alphaType) {
this(new ColorInfo(colorType, alphaType, null), width, height);
}
public ImageInfo(int width, int height, @NotNull ColorType colorType, @NotNull ColorAlphaType alphaType, @Nullable ColorSpace colorSpace) {
this(new ColorInfo(colorType, alphaType, colorSpace), width, height);
}
@ApiStatus.Internal
public ImageInfo(int width, int height, int colorType, int alphaType, long colorSpace) {
this(width, height, ColorType._values[colorType], ColorAlphaType._values[alphaType], colorSpace == 0 ? null : new ColorSpace(colorSpace));
}
/**
* @return ImageInfo with {@link ColorType#N32}
*/
@NotNull
@Contract("_, _, _ -> new")
public static ImageInfo makeN32(int width, int height, @NotNull ColorAlphaType alphaType) {
return new ImageInfo(new ColorInfo(ColorType.N32, alphaType, null), width, height);
}
/**
* @return ImageInfo with {@link ColorType#N32}
*/
@NotNull
@Contract("_, _, _, _ -> new")
public static ImageInfo makeN32(int width, int height, @NotNull ColorAlphaType alphaType, @Nullable ColorSpace colorSpace) {
return new ImageInfo(new ColorInfo(ColorType.N32, alphaType, colorSpace), width, height);
}
/**
* @return ImageInfo with {@link ColorType#N32} and {@link ColorSpace#getSRGB()}
*
* @see https://fiddle.skia.org/c/@ImageInfo_MakeS32
*/
@NotNull
@Contract("_, _, _ -> new")
public static ImageInfo makeS32(int width, int height, @NotNull ColorAlphaType alphaType) {
return new ImageInfo(new ColorInfo(ColorType.N32, alphaType, ColorSpace.getSRGB()), width, height);
}
/**
* @return ImageInfo with {@link ColorType#N32} and {@link ColorAlphaType#PREMUL}
*/
@NotNull
@Contract("_, _ -> new")
public static ImageInfo makeN32Premul(int width, int height) {
return new ImageInfo(new ColorInfo(ColorType.N32, ColorAlphaType.PREMUL, null), width, height);
}
/**
* @return ImageInfo with {@link ColorType#N32} and {@link ColorAlphaType#PREMUL}
*/
@NotNull
@Contract("_, _, _ -> new")
public static ImageInfo makeN32Premul(int width, int height, @Nullable ColorSpace colorSpace) {
return new ImageInfo(new ColorInfo(ColorType.N32, ColorAlphaType.PREMUL, colorSpace), width, height);
}
/**
* @return ImageInfo with {@link ColorType#ALPHA_8} and {@link ColorAlphaType#PREMUL}
*/
@NotNull
@Contract("_, _ -> new")
public static ImageInfo makeA8(int width, int height) {
return new ImageInfo(new ColorInfo(ColorType.ALPHA_8, ColorAlphaType.PREMUL, null), width, height);
}
/**
* @return ImageInfo with {@link ColorType#UNKNOWN} and {@link ColorAlphaType#UNKNOWN}
*/
@NotNull
@Contract("_, _ -> new")
public static ImageInfo makeUnknown(int width, int height) {
return new ImageInfo(new ColorInfo(ColorType.UNKNOWN, ColorAlphaType.UNKNOWN, null), width, height);
}
@NotNull
public ColorType getColorType() {
return _colorInfo.getColorType();
}
@NotNull
public ImageInfo withColorType(@NotNull ColorType colorType) {
return withColorInfo(_colorInfo.withColorType(colorType));
}
@NotNull
public ColorAlphaType getColorAlphaType() {
return _colorInfo.getAlphaType();
}
@NotNull
public ImageInfo withColorAlphaType(@NotNull ColorAlphaType alphaType) {
return withColorInfo(_colorInfo.withAlphaType(alphaType));
}
@Nullable
public ColorSpace getColorSpace() {
return _colorInfo.getColorSpace();
}
@NotNull
public ImageInfo withColorSpace(@NotNull ColorSpace colorSpace) {
return withColorInfo(_colorInfo.withColorSpace(colorSpace));
}
/**
* @return true if either dimension is zero or smaller
*/
public boolean isEmpty() {
return _width <= 0 || _height <= 0;
}
/**
* Returns true if ColorAlphaType is set to hint that all pixels are opaque; their
* alpha value is implicitly or explicitly 1.0. If true, and all pixels are
* not opaque, Skia may draw incorrectly.
*
* Does not check if ColorType allows alpha, or if any pixel value has
* transparency.
*
* @return true if alphaType is {@link ColorAlphaType#OPAQUE}
*/
public boolean isOpaque() {
return _colorInfo.isOpaque();
}
/**
* @return integral rectangle from (0, 0) to (getWidth(), getHeight())
*/
@NotNull
public IRect getBounds() {
return IRect.makeXYWH(0, 0, _width, _height);
}
/**
* @return true if associated ColorSpace is not null, and ColorSpace gamma
* is approximately the same as sRGB.
*/
public boolean isGammaCloseToSRGB() {
return _colorInfo.isGammaCloseToSRGB();
}
@NotNull
public ImageInfo withWidthHeight(int width, int height) {
return new ImageInfo(_colorInfo, width, height);
}
/**
* Returns number of bytes per pixel required by ColorType.
* Returns zero if {@link #getColorType()} is {@link ColorType#UNKNOWN}.
*
* @return bytes in pixel
*/
public int getBytesPerPixel() {
return _colorInfo.getBytesPerPixel();
}
/**
* Returns bit shift converting row bytes to row pixels.
* Returns zero for {@link ColorType#UNKNOWN}.
*
* @return one of: 0, 1, 2, 3, 4; left shift to convert pixels to bytes
*/
public int getShiftPerPixel() {
return _colorInfo.getShiftPerPixel();
}
/**
* Returns minimum bytes per row, computed from pixel getWidth() and ColorType, which
* specifies getBytesPerPixel(). Bitmap maximum value for row bytes must fit
* in 31 bits.
*/
public long getMinRowBytes() {
return _width * getBytesPerPixel();
}
/**
* Returns byte offset of pixel from pixel base address.
*
* Asserts in debug build if x or y is outside of bounds. Does not assert if
* rowBytes is smaller than {@link #getMinRowBytes()}, even though result may be incorrect.
*
* @param x column index, zero or greater, and less than getWidth()
* @param y row index, zero or greater, and less than getHeight()
* @param rowBytes size of pixel row or larger
* @return offset within pixel array
*
* @see https://fiddle.skia.org/c/@ImageInfo_computeOffset
*/
public long computeOffset(int x, int y, long rowBytes) {
return _colorInfo._colorType.computeOffset(x, y, rowBytes);
}
/**
* Returns storage required by pixel array, given ImageInfo dimensions, ColorType,
* and rowBytes. rowBytes is assumed to be at least as large as {@link #getMinRowBytes()}.
*
* Returns zero if height is zero.
*
* @param rowBytes size of pixel row or larger
* @return memory required by pixel buffer
*
* @see https://fiddle.skia.org/c/@ImageInfo_computeByteSize
*/
public long computeByteSize(long rowBytes) {
if (0 == _height) return 0;
return (_height - 1) * rowBytes + _width * getBytesPerPixel();
}
/**
* Returns storage required by pixel array, given ImageInfo dimensions, and
* ColorType. Uses {@link #getMinRowBytes()} to compute bytes for pixel row.
*
* Returns zero if height is zero.
*
* @return least memory required by pixel buffer
*/
public long computeMinByteSize() {
return computeByteSize(getMinRowBytes());
}
/**
* Returns true if rowBytes is valid for this ImageInfo.
*
* @param rowBytes size of pixel row including padding
* @return true if rowBytes is large enough to contain pixel row and is properly aligned
*/
public boolean isRowBytesValid(long rowBytes) {
if (rowBytes < getMinRowBytes()) return false;
int shift = getShiftPerPixel();
return rowBytes >> shift << shift == rowBytes;
}
@java.lang.SuppressWarnings("all")
public ImageInfo(final ColorInfo colorInfo, final int width, final int height) {
this._colorInfo = colorInfo;
this._width = width;
this._height = height;
}
@java.lang.SuppressWarnings("all")
public ColorInfo getColorInfo() {
return this._colorInfo;
}
@java.lang.SuppressWarnings("all")
public int getWidth() {
return this._width;
}
@java.lang.SuppressWarnings("all")
public int getHeight() {
return this._height;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ImageInfo)) return false;
final ImageInfo other = (ImageInfo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (this.getWidth() != other.getWidth()) return false;
if (this.getHeight() != other.getHeight()) return false;
final java.lang.Object this$_colorInfo = this.getColorInfo();
final java.lang.Object other$_colorInfo = other.getColorInfo();
if (this$_colorInfo == null ? other$_colorInfo != null : !this$_colorInfo.equals(other$_colorInfo)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ImageInfo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getWidth();
result = result * PRIME + this.getHeight();
final java.lang.Object $_colorInfo = this.getColorInfo();
result = result * PRIME + ($_colorInfo == null ? 43 : $_colorInfo.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ImageInfo(_colorInfo=" + this.getColorInfo() + ", _width=" + this.getWidth() + ", _height=" + this.getHeight() + ")";
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ImageInfo withColorInfo(final ColorInfo _colorInfo) {
return this._colorInfo == _colorInfo ? this : new ImageInfo(_colorInfo, this._width, this._height);
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ImageInfo withWidth(final int _width) {
return this._width == _width ? this : new ImageInfo(this._colorInfo, _width, this._height);
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ImageInfo withHeight(final int _height) {
return this._height == _height ? this : new ImageInfo(this._colorInfo, this._width, _height);
}
}