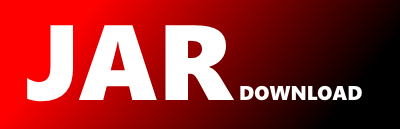
io.github.humbleui.skija.paragraph.LineMetrics Maven / Gradle / Ivy
// Generated by delombok at Wed Sep 14 01:50:49 UTC 2022
package io.github.humbleui.skija.paragraph;
public class LineMetrics {
/**
* The index in the text buffer the line begins.
*/
public final long _startIndex;
/**
* The index in the text buffer the line ends.
*/
public final long _endIndex;
public final long _endExcludingWhitespaces;
public final long _endIncludingNewline;
public final boolean _hardBreak;
/**
* The final computed ascent for the line. This can be impacted by the strut, height,
* scaling, as well as outlying runs that are very tall. The top edge is
* {@code getBaseline() - getAscent()} and the bottom edge is {@code getBaseline() + getDescent()}.
* Ascent and descent are provided as positive numbers. These values are the cumulative metrics for the entire line.
*/
public final double _ascent;
/**
* The final computed descent for the line. This can be impacted by the strut, height,
* scaling, as well as outlying runs that are very tall. The top edge is
* {@code getBaseline() - getAscent()} and the bottom edge is {@code getBaseline() + getDescent()}.
* Ascent and descent are provided as positive numbers. These values are the cumulative metrics for the entire line.
*/
public final double _descent;
public final double _unscaledAscent;
/**
* Total height of the paragraph including the current line.
*/
public final double _height;
/**
* Width of the line.
*/
public final double _width;
/**
* The left edge of the line.
*/
public final double _left;
/**
* The y position of the baseline for this line from the top of the paragraph.
*/
public final double _baseline;
/**
* Zero indexed line number
*/
public final long _lineNumber;
/**
* The height of the current line, equals to {@code Math.round(getAscent() + getDescent())}.
*/
public double getLineHeight() {
return _ascent + _descent;
}
/**
* The right edge of the line, equals to {@code getLeft() + getWidth()}
*/
public double getRight() {
return _left + _width;
}
@java.lang.SuppressWarnings("all")
public LineMetrics(final long startIndex, final long endIndex, final long endExcludingWhitespaces, final long endIncludingNewline, final boolean hardBreak, final double ascent, final double descent, final double unscaledAscent, final double height, final double width, final double left, final double baseline, final long lineNumber) {
this._startIndex = startIndex;
this._endIndex = endIndex;
this._endExcludingWhitespaces = endExcludingWhitespaces;
this._endIncludingNewline = endIncludingNewline;
this._hardBreak = hardBreak;
this._ascent = ascent;
this._descent = descent;
this._unscaledAscent = unscaledAscent;
this._height = height;
this._width = width;
this._left = left;
this._baseline = baseline;
this._lineNumber = lineNumber;
}
/**
* The index in the text buffer the line begins.
*/
@java.lang.SuppressWarnings("all")
public long getStartIndex() {
return this._startIndex;
}
/**
* The index in the text buffer the line ends.
*/
@java.lang.SuppressWarnings("all")
public long getEndIndex() {
return this._endIndex;
}
@java.lang.SuppressWarnings("all")
public long getEndExcludingWhitespaces() {
return this._endExcludingWhitespaces;
}
@java.lang.SuppressWarnings("all")
public long getEndIncludingNewline() {
return this._endIncludingNewline;
}
@java.lang.SuppressWarnings("all")
public boolean isHardBreak() {
return this._hardBreak;
}
/**
* The final computed ascent for the line. This can be impacted by the strut, height,
* scaling, as well as outlying runs that are very tall. The top edge is
* {@code getBaseline() - getAscent()} and the bottom edge is {@code getBaseline() + getDescent()}.
* Ascent and descent are provided as positive numbers. These values are the cumulative metrics for the entire line.
*/
@java.lang.SuppressWarnings("all")
public double getAscent() {
return this._ascent;
}
/**
* The final computed descent for the line. This can be impacted by the strut, height,
* scaling, as well as outlying runs that are very tall. The top edge is
* {@code getBaseline() - getAscent()} and the bottom edge is {@code getBaseline() + getDescent()}.
* Ascent and descent are provided as positive numbers. These values are the cumulative metrics for the entire line.
*/
@java.lang.SuppressWarnings("all")
public double getDescent() {
return this._descent;
}
@java.lang.SuppressWarnings("all")
public double getUnscaledAscent() {
return this._unscaledAscent;
}
/**
* Total height of the paragraph including the current line.
*/
@java.lang.SuppressWarnings("all")
public double getHeight() {
return this._height;
}
/**
* Width of the line.
*/
@java.lang.SuppressWarnings("all")
public double getWidth() {
return this._width;
}
/**
* The left edge of the line.
*/
@java.lang.SuppressWarnings("all")
public double getLeft() {
return this._left;
}
/**
* The y position of the baseline for this line from the top of the paragraph.
*/
@java.lang.SuppressWarnings("all")
public double getBaseline() {
return this._baseline;
}
/**
* Zero indexed line number
*/
@java.lang.SuppressWarnings("all")
public long getLineNumber() {
return this._lineNumber;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof LineMetrics)) return false;
final LineMetrics other = (LineMetrics) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (this.getStartIndex() != other.getStartIndex()) return false;
if (this.getEndIndex() != other.getEndIndex()) return false;
if (this.getEndExcludingWhitespaces() != other.getEndExcludingWhitespaces()) return false;
if (this.getEndIncludingNewline() != other.getEndIncludingNewline()) return false;
if (this.isHardBreak() != other.isHardBreak()) return false;
if (java.lang.Double.compare(this.getAscent(), other.getAscent()) != 0) return false;
if (java.lang.Double.compare(this.getDescent(), other.getDescent()) != 0) return false;
if (java.lang.Double.compare(this.getUnscaledAscent(), other.getUnscaledAscent()) != 0) return false;
if (java.lang.Double.compare(this.getHeight(), other.getHeight()) != 0) return false;
if (java.lang.Double.compare(this.getWidth(), other.getWidth()) != 0) return false;
if (java.lang.Double.compare(this.getLeft(), other.getLeft()) != 0) return false;
if (java.lang.Double.compare(this.getBaseline(), other.getBaseline()) != 0) return false;
if (this.getLineNumber() != other.getLineNumber()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof LineMetrics;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final long $_startIndex = this.getStartIndex();
result = result * PRIME + (int) ($_startIndex >>> 32 ^ $_startIndex);
final long $_endIndex = this.getEndIndex();
result = result * PRIME + (int) ($_endIndex >>> 32 ^ $_endIndex);
final long $_endExcludingWhitespaces = this.getEndExcludingWhitespaces();
result = result * PRIME + (int) ($_endExcludingWhitespaces >>> 32 ^ $_endExcludingWhitespaces);
final long $_endIncludingNewline = this.getEndIncludingNewline();
result = result * PRIME + (int) ($_endIncludingNewline >>> 32 ^ $_endIncludingNewline);
result = result * PRIME + (this.isHardBreak() ? 79 : 97);
final long $_ascent = java.lang.Double.doubleToLongBits(this.getAscent());
result = result * PRIME + (int) ($_ascent >>> 32 ^ $_ascent);
final long $_descent = java.lang.Double.doubleToLongBits(this.getDescent());
result = result * PRIME + (int) ($_descent >>> 32 ^ $_descent);
final long $_unscaledAscent = java.lang.Double.doubleToLongBits(this.getUnscaledAscent());
result = result * PRIME + (int) ($_unscaledAscent >>> 32 ^ $_unscaledAscent);
final long $_height = java.lang.Double.doubleToLongBits(this.getHeight());
result = result * PRIME + (int) ($_height >>> 32 ^ $_height);
final long $_width = java.lang.Double.doubleToLongBits(this.getWidth());
result = result * PRIME + (int) ($_width >>> 32 ^ $_width);
final long $_left = java.lang.Double.doubleToLongBits(this.getLeft());
result = result * PRIME + (int) ($_left >>> 32 ^ $_left);
final long $_baseline = java.lang.Double.doubleToLongBits(this.getBaseline());
result = result * PRIME + (int) ($_baseline >>> 32 ^ $_baseline);
final long $_lineNumber = this.getLineNumber();
result = result * PRIME + (int) ($_lineNumber >>> 32 ^ $_lineNumber);
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "LineMetrics(_startIndex=" + this.getStartIndex() + ", _endIndex=" + this.getEndIndex() + ", _endExcludingWhitespaces=" + this.getEndExcludingWhitespaces() + ", _endIncludingNewline=" + this.getEndIncludingNewline() + ", _hardBreak=" + this.isHardBreak() + ", _ascent=" + this.getAscent() + ", _descent=" + this.getDescent() + ", _unscaledAscent=" + this.getUnscaledAscent() + ", _height=" + this.getHeight() + ", _width=" + this.getWidth() + ", _left=" + this.getLeft() + ", _baseline=" + this.getBaseline() + ", _lineNumber=" + this.getLineNumber() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy