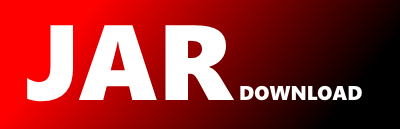
org.sam.server.util.ModelMapper Maven / Gradle / Ivy
package org.sam.server.util;
import org.sam.server.context.BeanFactory;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
/**
* 인스턴스를 다른 인스턴스로 변환할 수 있게 하는 클래스입니다.
*
* @author hypernova1
*/
public class ModelMapper {
/**
* 인스턴스를 다른 클래스 타입으로 변환합니다.
*
* @param 기존 인스턴스 타입
* @param 변경할 클래스 타입
* @param instance 기존 인스턴스
* @param clazz 변경할 클래스 타입
* @return 변경된 인스턴스
* */
public U convert(T instance, Class clazz) {
U target = null;
try {
target = clazz.getDeclaredConstructor().newInstance();
setValue(instance, target);
} catch (InstantiationException | IllegalAccessException | InvocationTargetException | NoSuchMethodException e) {
e.printStackTrace();
}
return target;
}
/**
* 기존 인스턴스의 프로퍼티를 변경할 인스턴스에 설정합니다.
*
* @param 기존 인스턴스 타입
* @param 변경할 인스턴스 타입
* @param instance 기존 인스턴스
* @param target 변경할 인스턴스
* */
private void setValue(T instance, U target) {
Method[] declaredMethods = instance.getClass().getDeclaredMethods();
for (Method declaredMethod : declaredMethods) {
String methodName = declaredMethod.getName();
if (!methodName.startsWith("get")) continue;
try {
String setterName = methodName.replace("get", "set");
Object value = declaredMethod.invoke(instance);
Method setter = target.getClass().getMethod(setterName, value.getClass());
setter.invoke(target, value);
} catch (IllegalAccessException | InvocationTargetException | NoSuchMethodException ignored) {}
}
BeanFactory beanFactory = BeanFactory.getInstance();
List> beanList = beanFactory.getBeanList(CustomModelMapper.class);
if (!beanList.isEmpty()) {
applyCustomConfig(instance, target, beanList);
}
}
private void applyCustomConfig(T instance, U target, List> beanList) {
try {
CustomModelMapper customModelMapper = (CustomModelMapper) beanList.get(0);
Method map = customModelMapper.getClass().getMethod("map", instance.getClass(), target.getClass());
map.invoke(customModelMapper, instance, target);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException ignored) {}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy