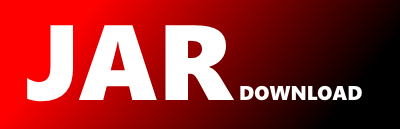
io.github.iac_m.jsonlogger.LogHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-logger-connector
Show all versions of json-logger-connector
JSON Logger is a custom-made Mule logger component which allows user to efficiently log traceable messages and Mule variables in JSON format.
The newest version!
package io.github.iac_m.jsonlogger;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import org.mule.api.MuleMessage;
import org.mule.api.transport.PropertyScope;
import io.github.iac_m.jsonlogger.dto.LogMessage;
import io.github.iac_m.jsonlogger.dto.LogVariable;
public class LogHelper {
private static final String CORRELATION_ID_INCOMING_HEADER_NAME = "x-correlation-id";
public static final String MSG_VAR_CORRELATION_ID = "correlation_id";
public static final String MSG_VAR_MESSAGE = "message";
public static LogMessage getBasicLogMessage(MuleMessage muleMessage, String message) {
LogMessage logMessage = new LogMessage();
logMessage.setCorrelationId(LogHelper.getOrGenerateCorrelationId(muleMessage));
String messageUniqueId = muleMessage.getUniqueId();
if (!messageUniqueId.equals(muleMessage.getMessageRootId())) {
// In a sub-scope such as for-each, two ids they are different
logMessage.setCorrelationIdLocal(messageUniqueId);
}
logMessage.setMessage(message);
return logMessage;
}
public static Collection extractVariables(MuleMessage message, PropertyScope scope,
Collection varNames, Boolean logType) {
Collection variables = new HashSet<>();
Set definedProperties = message.getPropertyNames(scope);
for (String varName : varNames) {
if (varName.equalsIgnoreCase("rootMessage")) {
continue;
}
if (definedProperties.contains(varName)) {
Object value = message.getProperty(varName, scope);
Map variable = new LinkedHashMap<>();
variable.put("name", varName);
variable.put("value", value);
variable.put("scope", scope.toString());
if (logType) {
variable.put("type", value != null ? value.getClass().getName() : null);
}
variables.add(new LogVariable(scope.toString(), varName, value, value.getClass().getName()));
}
}
return variables;
}
public static String variablesToString(List variables, boolean logType) {
Set variableStrings = new HashSet<>();
for (LogVariable v : variables) {
variableStrings.add(v.toString(logType));
}
return StringUtils.join(variableStrings, ", ");
}
private static String getOrGenerateCorrelationId(MuleMessage muleMessage) {
String cid = muleMessage.getInvocationProperty(MSG_VAR_CORRELATION_ID);
if (cid == null) {
// correlation id generation order:
// 1. HTTP Header x-correlation-id
// 2. Message Root Id
cid = muleMessage.getInboundProperty(CORRELATION_ID_INCOMING_HEADER_NAME) != null
? muleMessage.getInboundProperty(CORRELATION_ID_INCOMING_HEADER_NAME).toString()
: muleMessage.getMessageRootId();
// Set to flowVars
muleMessage.setInvocationProperty(MSG_VAR_CORRELATION_ID, cid);
}
return cid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy