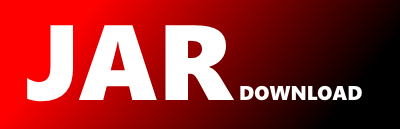
io.github.icodegarden.commons.lang.serialization.KryoDeserializer Maven / Gradle / Ivy
package io.github.icodegarden.commons.lang.serialization;
import java.io.ByteArrayInputStream;
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.KryoException;
import com.esotericsoftware.kryo.io.Input;
import io.github.icodegarden.commons.lang.serialization.KryoSerializer.AbstractKryoFactory;
import io.github.icodegarden.commons.lang.serialization.KryoSerializer.ThreadLocalKryoFactory;
/**
*
* @author Fangfang.Xu
*
*/
public class KryoDeserializer implements Deserializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy