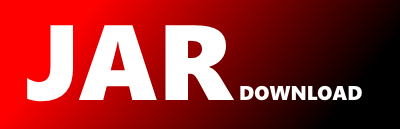
es.uam.eps.ir.relison.diffusion.metrics.IndividualSimulationMetric Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2020 Information Retrieval Group at Universidad Autónoma
* de Madrid, http://ir.ii.uam.es
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package es.uam.eps.ir.relison.diffusion.metrics;
import es.uam.eps.ir.relison.diffusion.simulation.Simulation;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Interface for the different individual metrics (can be applied over individual
* users) to apply over the simulation.
*
* @author Javier Sanz-Cruzado ([email protected])
* @author Pablo Castells ([email protected])
*
* @param type of the users.
* @param type of the information pieces.
* @param type of the features.
*/
public interface IndividualSimulationMetric extends SimulationMetric
{
/**
* Calculates the metric for each individual in the network.
* @return a map containing the values of the metric for each user.
*/
Map calculateIndividuals();
/**
* Calculates the metric for each individual user on each iteration of a simulation.
* @param simulation the whole simulation.
* @return the value of the metric for each iteration.
*/
default List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy