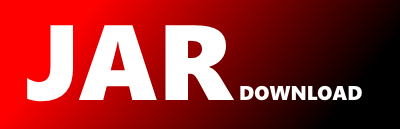
es.uam.eps.ir.relison.diffusion.sight.CountSightMechanism Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Information Retrieval Group at Universidad Autónoma
* de Madrid, http://ir.ii.uam.es
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package es.uam.eps.ir.relison.diffusion.sight;
import es.uam.eps.ir.relison.diffusion.data.Data;
import es.uam.eps.ir.relison.diffusion.data.PropagatedInformation;
import es.uam.eps.ir.relison.diffusion.simulation.UserState;
import java.io.Serializable;
import java.util.*;
/**
* The user sees (at most) a fixed number of (different) information pieces each iteration. The pieces are chosen
* randomly among all the received ones. If two instances of the same piece are received and one of them is seen,
* we assume that both have been.
*
* @author Javier Sanz-Cruzado ([email protected])
* @author Pablo Castells ([email protected])
*
* @param type of the users.
* @param type of the information pieces.
* @param type of the parameters.
*/
public class CountSightMechanism implements SightMechanism
{
/**
* Number of pieces of information that a user sees in a single iteration.
*/
private final int numSight;
/**
* Constructor.
* @param numSight number of pieces of information that a user sees (at most) in a single iteration.
*/
public CountSightMechanism(int numSight)
{
this.numSight = numSight;
}
@Override
public List seesInformation(UserState user, Data data, List prop)
{
Map> info = new HashMap<>();
List pieces = new ArrayList<>();
prop.forEach(piece ->
{
int id = piece.getInfoId();
if(!info.containsKey(id))
{
info.put(id, new ArrayList<>());
pieces.add(id);
}
info.get(id).add(piece);
});
List defList = new ArrayList<>();
if(pieces.size() <= this.numSight)
{
defList.addAll(prop);
}
else
{
Collections.shuffle(pieces);
pieces.subList(0, this.numSight).forEach(id -> defList.addAll(info.get(id)));
}
return defList;
}
@Override
public void resetSelections(Data data)
{
}
}