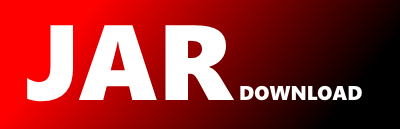
io.github.isotes.vs.model.ClCompileDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: ClCompile
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.ClCompileDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model;
/**
* A document containing one ClCompile(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public interface ClCompileDocument extends io.github.isotes.vs.model.ItemDocument
{
public static final org.apache.xmlbeans.SchemaType type = (org.apache.xmlbeans.SchemaType)
org.apache.xmlbeans.XmlBeans.typeSystemForClassLoader(ClCompileDocument.class.getClassLoader(), "schemaorg_apache_xmlbeans.system.io.github.isotes.vs.model.typesystemholder").resolveHandle("clcompile603adoctype");
/**
* Gets the "ClCompile" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile getClCompile();
/**
* Sets the "ClCompile" element
*/
void setClCompile(io.github.isotes.vs.model.ClCompileDocument.ClCompile clCompile);
/**
* Appends and returns a new empty "ClCompile" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile addNewClCompile();
/**
* An XML ClCompile(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public interface ClCompile extends io.github.isotes.vs.model.SimpleItemType
{
public static final org.apache.xmlbeans.SchemaType type = (org.apache.xmlbeans.SchemaType)
org.apache.xmlbeans.XmlBeans.typeSystemForClassLoader(ClCompile.class.getClassLoader(), "schemaorg_apache_xmlbeans.system.io.github.isotes.vs.model.typesystemholder").resolveHandle("clcompilec010elemtype");
/**
* Gets a List of "PrecompiledHeader" elements
*/
java.util.List getPrecompiledHeaderList();
/**
* Gets array of all "PrecompiledHeader" elements
* @deprecated
*/
@Deprecated
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[] getPrecompiledHeaderArray();
/**
* Gets ith "PrecompiledHeader" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader getPrecompiledHeaderArray(int i);
/**
* Returns number of "PrecompiledHeader" element
*/
int sizeOfPrecompiledHeaderArray();
/**
* Sets array of all "PrecompiledHeader" element
*/
void setPrecompiledHeaderArray(io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[] precompiledHeaderArray);
/**
* Sets ith "PrecompiledHeader" element
*/
void setPrecompiledHeaderArray(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader precompiledHeader);
/**
* Inserts and returns a new empty value (as xml) as the ith "PrecompiledHeader" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader insertNewPrecompiledHeader(int i);
/**
* Appends and returns a new empty value (as xml) as the last "PrecompiledHeader" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader addNewPrecompiledHeader();
/**
* Removes the ith "PrecompiledHeader" element
*/
void removePrecompiledHeader(int i);
/**
* Gets a List of "AdditionalIncludeDirectories" elements
*/
java.util.List getAdditionalIncludeDirectoriesList();
/**
* Gets array of all "AdditionalIncludeDirectories" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getAdditionalIncludeDirectoriesArray();
/**
* Gets ith "AdditionalIncludeDirectories" element
*/
org.apache.xmlbeans.XmlObject getAdditionalIncludeDirectoriesArray(int i);
/**
* Returns number of "AdditionalIncludeDirectories" element
*/
int sizeOfAdditionalIncludeDirectoriesArray();
/**
* Sets array of all "AdditionalIncludeDirectories" element
*/
void setAdditionalIncludeDirectoriesArray(org.apache.xmlbeans.XmlObject[] additionalIncludeDirectoriesArray);
/**
* Sets ith "AdditionalIncludeDirectories" element
*/
void setAdditionalIncludeDirectoriesArray(int i, org.apache.xmlbeans.XmlObject additionalIncludeDirectories);
/**
* Inserts and returns a new empty value (as xml) as the ith "AdditionalIncludeDirectories" element
*/
org.apache.xmlbeans.XmlObject insertNewAdditionalIncludeDirectories(int i);
/**
* Appends and returns a new empty value (as xml) as the last "AdditionalIncludeDirectories" element
*/
org.apache.xmlbeans.XmlObject addNewAdditionalIncludeDirectories();
/**
* Removes the ith "AdditionalIncludeDirectories" element
*/
void removeAdditionalIncludeDirectories(int i);
/**
* Gets a List of "AdditionalUsingDirectories" elements
*/
java.util.List getAdditionalUsingDirectoriesList();
/**
* Gets array of all "AdditionalUsingDirectories" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getAdditionalUsingDirectoriesArray();
/**
* Gets ith "AdditionalUsingDirectories" element
*/
org.apache.xmlbeans.XmlObject getAdditionalUsingDirectoriesArray(int i);
/**
* Returns number of "AdditionalUsingDirectories" element
*/
int sizeOfAdditionalUsingDirectoriesArray();
/**
* Sets array of all "AdditionalUsingDirectories" element
*/
void setAdditionalUsingDirectoriesArray(org.apache.xmlbeans.XmlObject[] additionalUsingDirectoriesArray);
/**
* Sets ith "AdditionalUsingDirectories" element
*/
void setAdditionalUsingDirectoriesArray(int i, org.apache.xmlbeans.XmlObject additionalUsingDirectories);
/**
* Inserts and returns a new empty value (as xml) as the ith "AdditionalUsingDirectories" element
*/
org.apache.xmlbeans.XmlObject insertNewAdditionalUsingDirectories(int i);
/**
* Appends and returns a new empty value (as xml) as the last "AdditionalUsingDirectories" element
*/
org.apache.xmlbeans.XmlObject addNewAdditionalUsingDirectories();
/**
* Removes the ith "AdditionalUsingDirectories" element
*/
void removeAdditionalUsingDirectories(int i);
/**
* Gets a List of "CompileAsManaged" elements
*/
java.util.List getCompileAsManagedList();
/**
* Gets array of all "CompileAsManaged" elements
* @deprecated
*/
@Deprecated
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[] getCompileAsManagedArray();
/**
* Gets ith "CompileAsManaged" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged getCompileAsManagedArray(int i);
/**
* Returns number of "CompileAsManaged" element
*/
int sizeOfCompileAsManagedArray();
/**
* Sets array of all "CompileAsManaged" element
*/
void setCompileAsManagedArray(io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[] compileAsManagedArray);
/**
* Sets ith "CompileAsManaged" element
*/
void setCompileAsManagedArray(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged compileAsManaged);
/**
* Inserts and returns a new empty value (as xml) as the ith "CompileAsManaged" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged insertNewCompileAsManaged(int i);
/**
* Appends and returns a new empty value (as xml) as the last "CompileAsManaged" element
*/
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged addNewCompileAsManaged();
/**
* Removes the ith "CompileAsManaged" element
*/
void removeCompileAsManaged(int i);
/**
* Gets a List of "ErrorReporting" elements
*/
java.util.List getErrorReportingList();
/**
* Gets array of all "ErrorReporting" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getErrorReportingArray();
/**
* Gets ith "ErrorReporting" element
*/
org.apache.xmlbeans.XmlObject getErrorReportingArray(int i);
/**
* Returns number of "ErrorReporting" element
*/
int sizeOfErrorReportingArray();
/**
* Sets array of all "ErrorReporting" element
*/
void setErrorReportingArray(org.apache.xmlbeans.XmlObject[] errorReportingArray);
/**
* Sets ith "ErrorReporting" element
*/
void setErrorReportingArray(int i, org.apache.xmlbeans.XmlObject errorReporting);
/**
* Inserts and returns a new empty value (as xml) as the ith "ErrorReporting" element
*/
org.apache.xmlbeans.XmlObject insertNewErrorReporting(int i);
/**
* Appends and returns a new empty value (as xml) as the last "ErrorReporting" element
*/
org.apache.xmlbeans.XmlObject addNewErrorReporting();
/**
* Removes the ith "ErrorReporting" element
*/
void removeErrorReporting(int i);
/**
* Gets a List of "WarningLevel" elements
*/
java.util.List getWarningLevelList();
/**
* Gets array of all "WarningLevel" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getWarningLevelArray();
/**
* Gets ith "WarningLevel" element
*/
org.apache.xmlbeans.XmlObject getWarningLevelArray(int i);
/**
* Returns number of "WarningLevel" element
*/
int sizeOfWarningLevelArray();
/**
* Sets array of all "WarningLevel" element
*/
void setWarningLevelArray(org.apache.xmlbeans.XmlObject[] warningLevelArray);
/**
* Sets ith "WarningLevel" element
*/
void setWarningLevelArray(int i, org.apache.xmlbeans.XmlObject warningLevel);
/**
* Inserts and returns a new empty value (as xml) as the ith "WarningLevel" element
*/
org.apache.xmlbeans.XmlObject insertNewWarningLevel(int i);
/**
* Appends and returns a new empty value (as xml) as the last "WarningLevel" element
*/
org.apache.xmlbeans.XmlObject addNewWarningLevel();
/**
* Removes the ith "WarningLevel" element
*/
void removeWarningLevel(int i);
/**
* Gets a List of "MinimalRebuild" elements
*/
java.util.List getMinimalRebuildList();
/**
* Gets array of all "MinimalRebuild" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getMinimalRebuildArray();
/**
* Gets ith "MinimalRebuild" element
*/
org.apache.xmlbeans.XmlObject getMinimalRebuildArray(int i);
/**
* Returns number of "MinimalRebuild" element
*/
int sizeOfMinimalRebuildArray();
/**
* Sets array of all "MinimalRebuild" element
*/
void setMinimalRebuildArray(org.apache.xmlbeans.XmlObject[] minimalRebuildArray);
/**
* Sets ith "MinimalRebuild" element
*/
void setMinimalRebuildArray(int i, org.apache.xmlbeans.XmlObject minimalRebuild);
/**
* Inserts and returns a new empty value (as xml) as the ith "MinimalRebuild" element
*/
org.apache.xmlbeans.XmlObject insertNewMinimalRebuild(int i);
/**
* Appends and returns a new empty value (as xml) as the last "MinimalRebuild" element
*/
org.apache.xmlbeans.XmlObject addNewMinimalRebuild();
/**
* Removes the ith "MinimalRebuild" element
*/
void removeMinimalRebuild(int i);
/**
* Gets a List of "DebugInformationFormat" elements
*/
java.util.List getDebugInformationFormatList();
/**
* Gets array of all "DebugInformationFormat" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getDebugInformationFormatArray();
/**
* Gets ith "DebugInformationFormat" element
*/
org.apache.xmlbeans.XmlObject getDebugInformationFormatArray(int i);
/**
* Returns number of "DebugInformationFormat" element
*/
int sizeOfDebugInformationFormatArray();
/**
* Sets array of all "DebugInformationFormat" element
*/
void setDebugInformationFormatArray(org.apache.xmlbeans.XmlObject[] debugInformationFormatArray);
/**
* Sets ith "DebugInformationFormat" element
*/
void setDebugInformationFormatArray(int i, org.apache.xmlbeans.XmlObject debugInformationFormat);
/**
* Inserts and returns a new empty value (as xml) as the ith "DebugInformationFormat" element
*/
org.apache.xmlbeans.XmlObject insertNewDebugInformationFormat(int i);
/**
* Appends and returns a new empty value (as xml) as the last "DebugInformationFormat" element
*/
org.apache.xmlbeans.XmlObject addNewDebugInformationFormat();
/**
* Removes the ith "DebugInformationFormat" element
*/
void removeDebugInformationFormat(int i);
/**
* Gets a List of "PreprocessorDefinitions" elements
*/
java.util.List getPreprocessorDefinitionsList();
/**
* Gets array of all "PreprocessorDefinitions" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getPreprocessorDefinitionsArray();
/**
* Gets ith "PreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject getPreprocessorDefinitionsArray(int i);
/**
* Returns number of "PreprocessorDefinitions" element
*/
int sizeOfPreprocessorDefinitionsArray();
/**
* Sets array of all "PreprocessorDefinitions" element
*/
void setPreprocessorDefinitionsArray(org.apache.xmlbeans.XmlObject[] preprocessorDefinitionsArray);
/**
* Sets ith "PreprocessorDefinitions" element
*/
void setPreprocessorDefinitionsArray(int i, org.apache.xmlbeans.XmlObject preprocessorDefinitions);
/**
* Inserts and returns a new empty value (as xml) as the ith "PreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject insertNewPreprocessorDefinitions(int i);
/**
* Appends and returns a new empty value (as xml) as the last "PreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject addNewPreprocessorDefinitions();
/**
* Removes the ith "PreprocessorDefinitions" element
*/
void removePreprocessorDefinitions(int i);
/**
* Gets a List of "Optimization" elements
*/
java.util.List getOptimizationList();
/**
* Gets array of all "Optimization" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getOptimizationArray();
/**
* Gets ith "Optimization" element
*/
org.apache.xmlbeans.XmlObject getOptimizationArray(int i);
/**
* Returns number of "Optimization" element
*/
int sizeOfOptimizationArray();
/**
* Sets array of all "Optimization" element
*/
void setOptimizationArray(org.apache.xmlbeans.XmlObject[] optimizationArray);
/**
* Sets ith "Optimization" element
*/
void setOptimizationArray(int i, org.apache.xmlbeans.XmlObject optimization);
/**
* Inserts and returns a new empty value (as xml) as the ith "Optimization" element
*/
org.apache.xmlbeans.XmlObject insertNewOptimization(int i);
/**
* Appends and returns a new empty value (as xml) as the last "Optimization" element
*/
org.apache.xmlbeans.XmlObject addNewOptimization();
/**
* Removes the ith "Optimization" element
*/
void removeOptimization(int i);
/**
* Gets a List of "BasicRuntimeChecks" elements
*/
java.util.List getBasicRuntimeChecksList();
/**
* Gets array of all "BasicRuntimeChecks" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getBasicRuntimeChecksArray();
/**
* Gets ith "BasicRuntimeChecks" element
*/
org.apache.xmlbeans.XmlObject getBasicRuntimeChecksArray(int i);
/**
* Returns number of "BasicRuntimeChecks" element
*/
int sizeOfBasicRuntimeChecksArray();
/**
* Sets array of all "BasicRuntimeChecks" element
*/
void setBasicRuntimeChecksArray(org.apache.xmlbeans.XmlObject[] basicRuntimeChecksArray);
/**
* Sets ith "BasicRuntimeChecks" element
*/
void setBasicRuntimeChecksArray(int i, org.apache.xmlbeans.XmlObject basicRuntimeChecks);
/**
* Inserts and returns a new empty value (as xml) as the ith "BasicRuntimeChecks" element
*/
org.apache.xmlbeans.XmlObject insertNewBasicRuntimeChecks(int i);
/**
* Appends and returns a new empty value (as xml) as the last "BasicRuntimeChecks" element
*/
org.apache.xmlbeans.XmlObject addNewBasicRuntimeChecks();
/**
* Removes the ith "BasicRuntimeChecks" element
*/
void removeBasicRuntimeChecks(int i);
/**
* Gets a List of "RuntimeLibrary" elements
*/
java.util.List getRuntimeLibraryList();
/**
* Gets array of all "RuntimeLibrary" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getRuntimeLibraryArray();
/**
* Gets ith "RuntimeLibrary" element
*/
org.apache.xmlbeans.XmlObject getRuntimeLibraryArray(int i);
/**
* Returns number of "RuntimeLibrary" element
*/
int sizeOfRuntimeLibraryArray();
/**
* Sets array of all "RuntimeLibrary" element
*/
void setRuntimeLibraryArray(org.apache.xmlbeans.XmlObject[] runtimeLibraryArray);
/**
* Sets ith "RuntimeLibrary" element
*/
void setRuntimeLibraryArray(int i, org.apache.xmlbeans.XmlObject runtimeLibrary);
/**
* Inserts and returns a new empty value (as xml) as the ith "RuntimeLibrary" element
*/
org.apache.xmlbeans.XmlObject insertNewRuntimeLibrary(int i);
/**
* Appends and returns a new empty value (as xml) as the last "RuntimeLibrary" element
*/
org.apache.xmlbeans.XmlObject addNewRuntimeLibrary();
/**
* Removes the ith "RuntimeLibrary" element
*/
void removeRuntimeLibrary(int i);
/**
* Gets a List of "FunctionLevelLinking" elements
*/
java.util.List getFunctionLevelLinkingList();
/**
* Gets array of all "FunctionLevelLinking" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getFunctionLevelLinkingArray();
/**
* Gets ith "FunctionLevelLinking" element
*/
org.apache.xmlbeans.XmlObject getFunctionLevelLinkingArray(int i);
/**
* Returns number of "FunctionLevelLinking" element
*/
int sizeOfFunctionLevelLinkingArray();
/**
* Sets array of all "FunctionLevelLinking" element
*/
void setFunctionLevelLinkingArray(org.apache.xmlbeans.XmlObject[] functionLevelLinkingArray);
/**
* Sets ith "FunctionLevelLinking" element
*/
void setFunctionLevelLinkingArray(int i, org.apache.xmlbeans.XmlObject functionLevelLinking);
/**
* Inserts and returns a new empty value (as xml) as the ith "FunctionLevelLinking" element
*/
org.apache.xmlbeans.XmlObject insertNewFunctionLevelLinking(int i);
/**
* Appends and returns a new empty value (as xml) as the last "FunctionLevelLinking" element
*/
org.apache.xmlbeans.XmlObject addNewFunctionLevelLinking();
/**
* Removes the ith "FunctionLevelLinking" element
*/
void removeFunctionLevelLinking(int i);
/**
* Gets a List of "FloatingPointModel" elements
*/
java.util.List getFloatingPointModelList();
/**
* Gets array of all "FloatingPointModel" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getFloatingPointModelArray();
/**
* Gets ith "FloatingPointModel" element
*/
org.apache.xmlbeans.XmlObject getFloatingPointModelArray(int i);
/**
* Returns number of "FloatingPointModel" element
*/
int sizeOfFloatingPointModelArray();
/**
* Sets array of all "FloatingPointModel" element
*/
void setFloatingPointModelArray(org.apache.xmlbeans.XmlObject[] floatingPointModelArray);
/**
* Sets ith "FloatingPointModel" element
*/
void setFloatingPointModelArray(int i, org.apache.xmlbeans.XmlObject floatingPointModel);
/**
* Inserts and returns a new empty value (as xml) as the ith "FloatingPointModel" element
*/
org.apache.xmlbeans.XmlObject insertNewFloatingPointModel(int i);
/**
* Appends and returns a new empty value (as xml) as the last "FloatingPointModel" element
*/
org.apache.xmlbeans.XmlObject addNewFloatingPointModel();
/**
* Removes the ith "FloatingPointModel" element
*/
void removeFloatingPointModel(int i);
/**
* Gets a List of "IntrinsicFunctions" elements
*/
java.util.List getIntrinsicFunctionsList();
/**
* Gets array of all "IntrinsicFunctions" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getIntrinsicFunctionsArray();
/**
* Gets ith "IntrinsicFunctions" element
*/
org.apache.xmlbeans.XmlObject getIntrinsicFunctionsArray(int i);
/**
* Returns number of "IntrinsicFunctions" element
*/
int sizeOfIntrinsicFunctionsArray();
/**
* Sets array of all "IntrinsicFunctions" element
*/
void setIntrinsicFunctionsArray(org.apache.xmlbeans.XmlObject[] intrinsicFunctionsArray);
/**
* Sets ith "IntrinsicFunctions" element
*/
void setIntrinsicFunctionsArray(int i, org.apache.xmlbeans.XmlObject intrinsicFunctions);
/**
* Inserts and returns a new empty value (as xml) as the ith "IntrinsicFunctions" element
*/
org.apache.xmlbeans.XmlObject insertNewIntrinsicFunctions(int i);
/**
* Appends and returns a new empty value (as xml) as the last "IntrinsicFunctions" element
*/
org.apache.xmlbeans.XmlObject addNewIntrinsicFunctions();
/**
* Removes the ith "IntrinsicFunctions" element
*/
void removeIntrinsicFunctions(int i);
/**
* Gets a List of "PrecompiledHeaderFile" elements
*/
java.util.List getPrecompiledHeaderFileList();
/**
* Gets array of all "PrecompiledHeaderFile" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getPrecompiledHeaderFileArray();
/**
* Gets ith "PrecompiledHeaderFile" element
*/
org.apache.xmlbeans.XmlObject getPrecompiledHeaderFileArray(int i);
/**
* Returns number of "PrecompiledHeaderFile" element
*/
int sizeOfPrecompiledHeaderFileArray();
/**
* Sets array of all "PrecompiledHeaderFile" element
*/
void setPrecompiledHeaderFileArray(org.apache.xmlbeans.XmlObject[] precompiledHeaderFileArray);
/**
* Sets ith "PrecompiledHeaderFile" element
*/
void setPrecompiledHeaderFileArray(int i, org.apache.xmlbeans.XmlObject precompiledHeaderFile);
/**
* Inserts and returns a new empty value (as xml) as the ith "PrecompiledHeaderFile" element
*/
org.apache.xmlbeans.XmlObject insertNewPrecompiledHeaderFile(int i);
/**
* Appends and returns a new empty value (as xml) as the last "PrecompiledHeaderFile" element
*/
org.apache.xmlbeans.XmlObject addNewPrecompiledHeaderFile();
/**
* Removes the ith "PrecompiledHeaderFile" element
*/
void removePrecompiledHeaderFile(int i);
/**
* Gets a List of "MultiProcessorCompilation" elements
*/
java.util.List getMultiProcessorCompilationList();
/**
* Gets array of all "MultiProcessorCompilation" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getMultiProcessorCompilationArray();
/**
* Gets ith "MultiProcessorCompilation" element
*/
org.apache.xmlbeans.XmlObject getMultiProcessorCompilationArray(int i);
/**
* Returns number of "MultiProcessorCompilation" element
*/
int sizeOfMultiProcessorCompilationArray();
/**
* Sets array of all "MultiProcessorCompilation" element
*/
void setMultiProcessorCompilationArray(org.apache.xmlbeans.XmlObject[] multiProcessorCompilationArray);
/**
* Sets ith "MultiProcessorCompilation" element
*/
void setMultiProcessorCompilationArray(int i, org.apache.xmlbeans.XmlObject multiProcessorCompilation);
/**
* Inserts and returns a new empty value (as xml) as the ith "MultiProcessorCompilation" element
*/
org.apache.xmlbeans.XmlObject insertNewMultiProcessorCompilation(int i);
/**
* Appends and returns a new empty value (as xml) as the last "MultiProcessorCompilation" element
*/
org.apache.xmlbeans.XmlObject addNewMultiProcessorCompilation();
/**
* Removes the ith "MultiProcessorCompilation" element
*/
void removeMultiProcessorCompilation(int i);
/**
* Gets a List of "UseUnicodeForAssemblerListing" elements
*/
java.util.List getUseUnicodeForAssemblerListingList();
/**
* Gets array of all "UseUnicodeForAssemblerListing" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getUseUnicodeForAssemblerListingArray();
/**
* Gets ith "UseUnicodeForAssemblerListing" element
*/
org.apache.xmlbeans.XmlObject getUseUnicodeForAssemblerListingArray(int i);
/**
* Returns number of "UseUnicodeForAssemblerListing" element
*/
int sizeOfUseUnicodeForAssemblerListingArray();
/**
* Sets array of all "UseUnicodeForAssemblerListing" element
*/
void setUseUnicodeForAssemblerListingArray(org.apache.xmlbeans.XmlObject[] useUnicodeForAssemblerListingArray);
/**
* Sets ith "UseUnicodeForAssemblerListing" element
*/
void setUseUnicodeForAssemblerListingArray(int i, org.apache.xmlbeans.XmlObject useUnicodeForAssemblerListing);
/**
* Inserts and returns a new empty value (as xml) as the ith "UseUnicodeForAssemblerListing" element
*/
org.apache.xmlbeans.XmlObject insertNewUseUnicodeForAssemblerListing(int i);
/**
* Appends and returns a new empty value (as xml) as the last "UseUnicodeForAssemblerListing" element
*/
org.apache.xmlbeans.XmlObject addNewUseUnicodeForAssemblerListing();
/**
* Removes the ith "UseUnicodeForAssemblerListing" element
*/
void removeUseUnicodeForAssemblerListing(int i);
/**
* Gets a List of "UndefinePreprocessorDefinitions" elements
*/
java.util.List getUndefinePreprocessorDefinitionsList();
/**
* Gets array of all "UndefinePreprocessorDefinitions" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getUndefinePreprocessorDefinitionsArray();
/**
* Gets ith "UndefinePreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject getUndefinePreprocessorDefinitionsArray(int i);
/**
* Returns number of "UndefinePreprocessorDefinitions" element
*/
int sizeOfUndefinePreprocessorDefinitionsArray();
/**
* Sets array of all "UndefinePreprocessorDefinitions" element
*/
void setUndefinePreprocessorDefinitionsArray(org.apache.xmlbeans.XmlObject[] undefinePreprocessorDefinitionsArray);
/**
* Sets ith "UndefinePreprocessorDefinitions" element
*/
void setUndefinePreprocessorDefinitionsArray(int i, org.apache.xmlbeans.XmlObject undefinePreprocessorDefinitions);
/**
* Inserts and returns a new empty value (as xml) as the ith "UndefinePreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject insertNewUndefinePreprocessorDefinitions(int i);
/**
* Appends and returns a new empty value (as xml) as the last "UndefinePreprocessorDefinitions" element
*/
org.apache.xmlbeans.XmlObject addNewUndefinePreprocessorDefinitions();
/**
* Removes the ith "UndefinePreprocessorDefinitions" element
*/
void removeUndefinePreprocessorDefinitions(int i);
/**
* Gets a List of "StringPooling" elements
*/
java.util.List getStringPoolingList();
/**
* Gets array of all "StringPooling" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getStringPoolingArray();
/**
* Gets ith "StringPooling" element
*/
org.apache.xmlbeans.XmlObject getStringPoolingArray(int i);
/**
* Returns number of "StringPooling" element
*/
int sizeOfStringPoolingArray();
/**
* Sets array of all "StringPooling" element
*/
void setStringPoolingArray(org.apache.xmlbeans.XmlObject[] stringPoolingArray);
/**
* Sets ith "StringPooling" element
*/
void setStringPoolingArray(int i, org.apache.xmlbeans.XmlObject stringPooling);
/**
* Inserts and returns a new empty value (as xml) as the ith "StringPooling" element
*/
org.apache.xmlbeans.XmlObject insertNewStringPooling(int i);
/**
* Appends and returns a new empty value (as xml) as the last "StringPooling" element
*/
org.apache.xmlbeans.XmlObject addNewStringPooling();
/**
* Removes the ith "StringPooling" element
*/
void removeStringPooling(int i);
/**
* Gets a List of "BrowseInformation" elements
*/
java.util.List getBrowseInformationList();
/**
* Gets array of all "BrowseInformation" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getBrowseInformationArray();
/**
* Gets ith "BrowseInformation" element
*/
org.apache.xmlbeans.XmlObject getBrowseInformationArray(int i);
/**
* Returns number of "BrowseInformation" element
*/
int sizeOfBrowseInformationArray();
/**
* Sets array of all "BrowseInformation" element
*/
void setBrowseInformationArray(org.apache.xmlbeans.XmlObject[] browseInformationArray);
/**
* Sets ith "BrowseInformation" element
*/
void setBrowseInformationArray(int i, org.apache.xmlbeans.XmlObject browseInformation);
/**
* Inserts and returns a new empty value (as xml) as the ith "BrowseInformation" element
*/
org.apache.xmlbeans.XmlObject insertNewBrowseInformation(int i);
/**
* Appends and returns a new empty value (as xml) as the last "BrowseInformation" element
*/
org.apache.xmlbeans.XmlObject addNewBrowseInformation();
/**
* Removes the ith "BrowseInformation" element
*/
void removeBrowseInformation(int i);
/**
* Gets a List of "FloatingPointExceptions" elements
*/
java.util.List getFloatingPointExceptionsList();
/**
* Gets array of all "FloatingPointExceptions" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getFloatingPointExceptionsArray();
/**
* Gets ith "FloatingPointExceptions" element
*/
org.apache.xmlbeans.XmlObject getFloatingPointExceptionsArray(int i);
/**
* Returns number of "FloatingPointExceptions" element
*/
int sizeOfFloatingPointExceptionsArray();
/**
* Sets array of all "FloatingPointExceptions" element
*/
void setFloatingPointExceptionsArray(org.apache.xmlbeans.XmlObject[] floatingPointExceptionsArray);
/**
* Sets ith "FloatingPointExceptions" element
*/
void setFloatingPointExceptionsArray(int i, org.apache.xmlbeans.XmlObject floatingPointExceptions);
/**
* Inserts and returns a new empty value (as xml) as the ith "FloatingPointExceptions" element
*/
org.apache.xmlbeans.XmlObject insertNewFloatingPointExceptions(int i);
/**
* Appends and returns a new empty value (as xml) as the last "FloatingPointExceptions" element
*/
org.apache.xmlbeans.XmlObject addNewFloatingPointExceptions();
/**
* Removes the ith "FloatingPointExceptions" element
*/
void removeFloatingPointExceptions(int i);
/**
* Gets a List of "CreateHotpatchableImage" elements
*/
java.util.List getCreateHotpatchableImageList();
/**
* Gets array of all "CreateHotpatchableImage" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getCreateHotpatchableImageArray();
/**
* Gets ith "CreateHotpatchableImage" element
*/
org.apache.xmlbeans.XmlObject getCreateHotpatchableImageArray(int i);
/**
* Returns number of "CreateHotpatchableImage" element
*/
int sizeOfCreateHotpatchableImageArray();
/**
* Sets array of all "CreateHotpatchableImage" element
*/
void setCreateHotpatchableImageArray(org.apache.xmlbeans.XmlObject[] createHotpatchableImageArray);
/**
* Sets ith "CreateHotpatchableImage" element
*/
void setCreateHotpatchableImageArray(int i, org.apache.xmlbeans.XmlObject createHotpatchableImage);
/**
* Inserts and returns a new empty value (as xml) as the ith "CreateHotpatchableImage" element
*/
org.apache.xmlbeans.XmlObject insertNewCreateHotpatchableImage(int i);
/**
* Appends and returns a new empty value (as xml) as the last "CreateHotpatchableImage" element
*/
org.apache.xmlbeans.XmlObject addNewCreateHotpatchableImage();
/**
* Removes the ith "CreateHotpatchableImage" element
*/
void removeCreateHotpatchableImage(int i);
/**
* Gets a List of "RuntimeTypeInfo" elements
*/
java.util.List getRuntimeTypeInfoList();
/**
* Gets array of all "RuntimeTypeInfo" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getRuntimeTypeInfoArray();
/**
* Gets ith "RuntimeTypeInfo" element
*/
org.apache.xmlbeans.XmlObject getRuntimeTypeInfoArray(int i);
/**
* Returns number of "RuntimeTypeInfo" element
*/
int sizeOfRuntimeTypeInfoArray();
/**
* Sets array of all "RuntimeTypeInfo" element
*/
void setRuntimeTypeInfoArray(org.apache.xmlbeans.XmlObject[] runtimeTypeInfoArray);
/**
* Sets ith "RuntimeTypeInfo" element
*/
void setRuntimeTypeInfoArray(int i, org.apache.xmlbeans.XmlObject runtimeTypeInfo);
/**
* Inserts and returns a new empty value (as xml) as the ith "RuntimeTypeInfo" element
*/
org.apache.xmlbeans.XmlObject insertNewRuntimeTypeInfo(int i);
/**
* Appends and returns a new empty value (as xml) as the last "RuntimeTypeInfo" element
*/
org.apache.xmlbeans.XmlObject addNewRuntimeTypeInfo();
/**
* Removes the ith "RuntimeTypeInfo" element
*/
void removeRuntimeTypeInfo(int i);
/**
* Gets a List of "OpenMPSupport" elements
*/
java.util.List getOpenMPSupportList();
/**
* Gets array of all "OpenMPSupport" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getOpenMPSupportArray();
/**
* Gets ith "OpenMPSupport" element
*/
org.apache.xmlbeans.XmlObject getOpenMPSupportArray(int i);
/**
* Returns number of "OpenMPSupport" element
*/
int sizeOfOpenMPSupportArray();
/**
* Sets array of all "OpenMPSupport" element
*/
void setOpenMPSupportArray(org.apache.xmlbeans.XmlObject[] openMPSupportArray);
/**
* Sets ith "OpenMPSupport" element
*/
void setOpenMPSupportArray(int i, org.apache.xmlbeans.XmlObject openMPSupport);
/**
* Inserts and returns a new empty value (as xml) as the ith "OpenMPSupport" element
*/
org.apache.xmlbeans.XmlObject insertNewOpenMPSupport(int i);
/**
* Appends and returns a new empty value (as xml) as the last "OpenMPSupport" element
*/
org.apache.xmlbeans.XmlObject addNewOpenMPSupport();
/**
* Removes the ith "OpenMPSupport" element
*/
void removeOpenMPSupport(int i);
/**
* Gets a List of "CallingConvention" elements
*/
java.util.List getCallingConventionList();
/**
* Gets array of all "CallingConvention" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getCallingConventionArray();
/**
* Gets ith "CallingConvention" element
*/
org.apache.xmlbeans.XmlObject getCallingConventionArray(int i);
/**
* Returns number of "CallingConvention" element
*/
int sizeOfCallingConventionArray();
/**
* Sets array of all "CallingConvention" element
*/
void setCallingConventionArray(org.apache.xmlbeans.XmlObject[] callingConventionArray);
/**
* Sets ith "CallingConvention" element
*/
void setCallingConventionArray(int i, org.apache.xmlbeans.XmlObject callingConvention);
/**
* Inserts and returns a new empty value (as xml) as the ith "CallingConvention" element
*/
org.apache.xmlbeans.XmlObject insertNewCallingConvention(int i);
/**
* Appends and returns a new empty value (as xml) as the last "CallingConvention" element
*/
org.apache.xmlbeans.XmlObject addNewCallingConvention();
/**
* Removes the ith "CallingConvention" element
*/
void removeCallingConvention(int i);
/**
* Gets a List of "DisableSpecificWarnings" elements
*/
java.util.List getDisableSpecificWarningsList();
/**
* Gets array of all "DisableSpecificWarnings" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getDisableSpecificWarningsArray();
/**
* Gets ith "DisableSpecificWarnings" element
*/
org.apache.xmlbeans.XmlObject getDisableSpecificWarningsArray(int i);
/**
* Returns number of "DisableSpecificWarnings" element
*/
int sizeOfDisableSpecificWarningsArray();
/**
* Sets array of all "DisableSpecificWarnings" element
*/
void setDisableSpecificWarningsArray(org.apache.xmlbeans.XmlObject[] disableSpecificWarningsArray);
/**
* Sets ith "DisableSpecificWarnings" element
*/
void setDisableSpecificWarningsArray(int i, org.apache.xmlbeans.XmlObject disableSpecificWarnings);
/**
* Inserts and returns a new empty value (as xml) as the ith "DisableSpecificWarnings" element
*/
org.apache.xmlbeans.XmlObject insertNewDisableSpecificWarnings(int i);
/**
* Appends and returns a new empty value (as xml) as the last "DisableSpecificWarnings" element
*/
org.apache.xmlbeans.XmlObject addNewDisableSpecificWarnings();
/**
* Removes the ith "DisableSpecificWarnings" element
*/
void removeDisableSpecificWarnings(int i);
/**
* Gets a List of "ForcedIncludeFiles" elements
*/
java.util.List getForcedIncludeFilesList();
/**
* Gets array of all "ForcedIncludeFiles" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getForcedIncludeFilesArray();
/**
* Gets ith "ForcedIncludeFiles" element
*/
org.apache.xmlbeans.XmlObject getForcedIncludeFilesArray(int i);
/**
* Returns number of "ForcedIncludeFiles" element
*/
int sizeOfForcedIncludeFilesArray();
/**
* Sets array of all "ForcedIncludeFiles" element
*/
void setForcedIncludeFilesArray(org.apache.xmlbeans.XmlObject[] forcedIncludeFilesArray);
/**
* Sets ith "ForcedIncludeFiles" element
*/
void setForcedIncludeFilesArray(int i, org.apache.xmlbeans.XmlObject forcedIncludeFiles);
/**
* Inserts and returns a new empty value (as xml) as the ith "ForcedIncludeFiles" element
*/
org.apache.xmlbeans.XmlObject insertNewForcedIncludeFiles(int i);
/**
* Appends and returns a new empty value (as xml) as the last "ForcedIncludeFiles" element
*/
org.apache.xmlbeans.XmlObject addNewForcedIncludeFiles();
/**
* Removes the ith "ForcedIncludeFiles" element
*/
void removeForcedIncludeFiles(int i);
/**
* Gets a List of "ForcedUsingFiles" elements
*/
java.util.List getForcedUsingFilesList();
/**
* Gets array of all "ForcedUsingFiles" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getForcedUsingFilesArray();
/**
* Gets ith "ForcedUsingFiles" element
*/
org.apache.xmlbeans.XmlObject getForcedUsingFilesArray(int i);
/**
* Returns number of "ForcedUsingFiles" element
*/
int sizeOfForcedUsingFilesArray();
/**
* Sets array of all "ForcedUsingFiles" element
*/
void setForcedUsingFilesArray(org.apache.xmlbeans.XmlObject[] forcedUsingFilesArray);
/**
* Sets ith "ForcedUsingFiles" element
*/
void setForcedUsingFilesArray(int i, org.apache.xmlbeans.XmlObject forcedUsingFiles);
/**
* Inserts and returns a new empty value (as xml) as the ith "ForcedUsingFiles" element
*/
org.apache.xmlbeans.XmlObject insertNewForcedUsingFiles(int i);
/**
* Appends and returns a new empty value (as xml) as the last "ForcedUsingFiles" element
*/
org.apache.xmlbeans.XmlObject addNewForcedUsingFiles();
/**
* Removes the ith "ForcedUsingFiles" element
*/
void removeForcedUsingFiles(int i);
/**
* Gets a List of "ShowIncludes" elements
*/
java.util.List getShowIncludesList();
/**
* Gets array of all "ShowIncludes" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getShowIncludesArray();
/**
* Gets ith "ShowIncludes" element
*/
org.apache.xmlbeans.XmlObject getShowIncludesArray(int i);
/**
* Returns number of "ShowIncludes" element
*/
int sizeOfShowIncludesArray();
/**
* Sets array of all "ShowIncludes" element
*/
void setShowIncludesArray(org.apache.xmlbeans.XmlObject[] showIncludesArray);
/**
* Sets ith "ShowIncludes" element
*/
void setShowIncludesArray(int i, org.apache.xmlbeans.XmlObject showIncludes);
/**
* Inserts and returns a new empty value (as xml) as the ith "ShowIncludes" element
*/
org.apache.xmlbeans.XmlObject insertNewShowIncludes(int i);
/**
* Appends and returns a new empty value (as xml) as the last "ShowIncludes" element
*/
org.apache.xmlbeans.XmlObject addNewShowIncludes();
/**
* Removes the ith "ShowIncludes" element
*/
void removeShowIncludes(int i);
/**
* Gets a List of "UseFullPaths" elements
*/
java.util.List getUseFullPathsList();
/**
* Gets array of all "UseFullPaths" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getUseFullPathsArray();
/**
* Gets ith "UseFullPaths" element
*/
org.apache.xmlbeans.XmlObject getUseFullPathsArray(int i);
/**
* Returns number of "UseFullPaths" element
*/
int sizeOfUseFullPathsArray();
/**
* Sets array of all "UseFullPaths" element
*/
void setUseFullPathsArray(org.apache.xmlbeans.XmlObject[] useFullPathsArray);
/**
* Sets ith "UseFullPaths" element
*/
void setUseFullPathsArray(int i, org.apache.xmlbeans.XmlObject useFullPaths);
/**
* Inserts and returns a new empty value (as xml) as the ith "UseFullPaths" element
*/
org.apache.xmlbeans.XmlObject insertNewUseFullPaths(int i);
/**
* Appends and returns a new empty value (as xml) as the last "UseFullPaths" element
*/
org.apache.xmlbeans.XmlObject addNewUseFullPaths();
/**
* Removes the ith "UseFullPaths" element
*/
void removeUseFullPaths(int i);
/**
* Gets a List of "OmitDefaultLibName" elements
*/
java.util.List getOmitDefaultLibNameList();
/**
* Gets array of all "OmitDefaultLibName" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getOmitDefaultLibNameArray();
/**
* Gets ith "OmitDefaultLibName" element
*/
org.apache.xmlbeans.XmlObject getOmitDefaultLibNameArray(int i);
/**
* Returns number of "OmitDefaultLibName" element
*/
int sizeOfOmitDefaultLibNameArray();
/**
* Sets array of all "OmitDefaultLibName" element
*/
void setOmitDefaultLibNameArray(org.apache.xmlbeans.XmlObject[] omitDefaultLibNameArray);
/**
* Sets ith "OmitDefaultLibName" element
*/
void setOmitDefaultLibNameArray(int i, org.apache.xmlbeans.XmlObject omitDefaultLibName);
/**
* Inserts and returns a new empty value (as xml) as the ith "OmitDefaultLibName" element
*/
org.apache.xmlbeans.XmlObject insertNewOmitDefaultLibName(int i);
/**
* Appends and returns a new empty value (as xml) as the last "OmitDefaultLibName" element
*/
org.apache.xmlbeans.XmlObject addNewOmitDefaultLibName();
/**
* Removes the ith "OmitDefaultLibName" element
*/
void removeOmitDefaultLibName(int i);
/**
* Gets a List of "TreatSpecificWarningsAsErrors" elements
*/
java.util.List getTreatSpecificWarningsAsErrorsList();
/**
* Gets array of all "TreatSpecificWarningsAsErrors" elements
* @deprecated
*/
@Deprecated
org.apache.xmlbeans.XmlObject[] getTreatSpecificWarningsAsErrorsArray();
/**
* Gets ith "TreatSpecificWarningsAsErrors" element
*/
org.apache.xmlbeans.XmlObject getTreatSpecificWarningsAsErrorsArray(int i);
/**
* Returns number of "TreatSpecificWarningsAsErrors" element
*/
int sizeOfTreatSpecificWarningsAsErrorsArray();
/**
* Sets array of all "TreatSpecificWarningsAsErrors" element
*/
void setTreatSpecificWarningsAsErrorsArray(org.apache.xmlbeans.XmlObject[] treatSpecificWarningsAsErrorsArray);
/**
* Sets ith "TreatSpecificWarningsAsErrors" element
*/
void setTreatSpecificWarningsAsErrorsArray(int i, org.apache.xmlbeans.XmlObject treatSpecificWarningsAsErrors);
/**
* Inserts and returns a new empty value (as xml) as the ith "TreatSpecificWarningsAsErrors" element
*/
org.apache.xmlbeans.XmlObject insertNewTreatSpecificWarningsAsErrors(int i);
/**
* Appends and returns a new empty value (as xml) as the last "TreatSpecificWarningsAsErrors" element
*/
org.apache.xmlbeans.XmlObject addNewTreatSpecificWarningsAsErrors();
/**
* Removes the ith "TreatSpecificWarningsAsErrors" element
*/
void removeTreatSpecificWarningsAsErrors(int i);
/**
* An XML PrecompiledHeader(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is an atomic type that is a restriction of io.github.isotes.vs.model.ClCompileDocument$ClCompile$PrecompiledHeader.
*/
public interface PrecompiledHeader extends org.apache.xmlbeans.XmlString
{
public static final org.apache.xmlbeans.SchemaType type = (org.apache.xmlbeans.SchemaType)
org.apache.xmlbeans.XmlBeans.typeSystemForClassLoader(PrecompiledHeader.class.getClassLoader(), "schemaorg_apache_xmlbeans.system.io.github.isotes.vs.model.typesystemholder").resolveHandle("precompiledheader9abdelemtype");
/**
* Gets the "Condition" attribute
*/
org.apache.xmlbeans.XmlAnySimpleType getCondition();
/**
* True if has "Condition" attribute
*/
boolean isSetCondition();
/**
* Sets the "Condition" attribute
*/
void setCondition(org.apache.xmlbeans.XmlAnySimpleType condition);
/**
* Appends and returns a new empty "Condition" attribute
*/
org.apache.xmlbeans.XmlAnySimpleType addNewCondition();
/**
* Unsets the "Condition" attribute
*/
void unsetCondition();
/**
* A factory class with static methods for creating instances
* of this type.
*/
public static final class Factory
{
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader newInstance() {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader newInstance(org.apache.xmlbeans.XmlOptions options) {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, options ); }
private Factory() { } // No instance of this class allowed
}
}
/**
* An XML CompileAsManaged(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is an atomic type that is a restriction of io.github.isotes.vs.model.ClCompileDocument$ClCompile$CompileAsManaged.
*/
public interface CompileAsManaged extends org.apache.xmlbeans.XmlString
{
public static final org.apache.xmlbeans.SchemaType type = (org.apache.xmlbeans.SchemaType)
org.apache.xmlbeans.XmlBeans.typeSystemForClassLoader(CompileAsManaged.class.getClassLoader(), "schemaorg_apache_xmlbeans.system.io.github.isotes.vs.model.typesystemholder").resolveHandle("compileasmanaged09e6elemtype");
/**
* Gets the "Condition" attribute
*/
org.apache.xmlbeans.XmlAnySimpleType getCondition();
/**
* True if has "Condition" attribute
*/
boolean isSetCondition();
/**
* Sets the "Condition" attribute
*/
void setCondition(org.apache.xmlbeans.XmlAnySimpleType condition);
/**
* Appends and returns a new empty "Condition" attribute
*/
org.apache.xmlbeans.XmlAnySimpleType addNewCondition();
/**
* Unsets the "Condition" attribute
*/
void unsetCondition();
/**
* A factory class with static methods for creating instances
* of this type.
*/
public static final class Factory
{
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged newInstance() {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged newInstance(org.apache.xmlbeans.XmlOptions options) {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, options ); }
private Factory() { } // No instance of this class allowed
}
}
/**
* A factory class with static methods for creating instances
* of this type.
*/
public static final class Factory
{
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile newInstance() {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument.ClCompile newInstance(org.apache.xmlbeans.XmlOptions options) {
return (io.github.isotes.vs.model.ClCompileDocument.ClCompile) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, options ); }
private Factory() { } // No instance of this class allowed
}
}
/**
* A factory class with static methods for creating instances
* of this type.
*/
public static final class Factory
{
public static io.github.isotes.vs.model.ClCompileDocument newInstance() {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument newInstance(org.apache.xmlbeans.XmlOptions options) {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newInstance( type, options ); }
/** @param xmlAsString the string value to parse */
public static io.github.isotes.vs.model.ClCompileDocument parse(java.lang.String xmlAsString) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( xmlAsString, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.lang.String xmlAsString, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( xmlAsString, type, options ); }
/** @param file the file from which to load an xml document */
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.File file) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( file, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.File file, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( file, type, options ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.net.URL u) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( u, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.net.URL u, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( u, type, options ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.InputStream is) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( is, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.InputStream is, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( is, type, options ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.Reader r) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( r, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(java.io.Reader r, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, java.io.IOException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( r, type, options ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(javax.xml.stream.XMLStreamReader sr) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( sr, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(javax.xml.stream.XMLStreamReader sr, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( sr, type, options ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(org.w3c.dom.Node node) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( node, type, null ); }
public static io.github.isotes.vs.model.ClCompileDocument parse(org.w3c.dom.Node node, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( node, type, options ); }
/** @deprecated {@link org.apache.xmlbeans.xml.stream.XMLInputStream} */
@Deprecated
public static io.github.isotes.vs.model.ClCompileDocument parse(org.apache.xmlbeans.xml.stream.XMLInputStream xis) throws org.apache.xmlbeans.XmlException, org.apache.xmlbeans.xml.stream.XMLStreamException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( xis, type, null ); }
/** @deprecated {@link org.apache.xmlbeans.xml.stream.XMLInputStream} */
@Deprecated
public static io.github.isotes.vs.model.ClCompileDocument parse(org.apache.xmlbeans.xml.stream.XMLInputStream xis, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, org.apache.xmlbeans.xml.stream.XMLStreamException {
return (io.github.isotes.vs.model.ClCompileDocument) org.apache.xmlbeans.XmlBeans.getContextTypeLoader().parse( xis, type, options ); }
/** @deprecated {@link org.apache.xmlbeans.xml.stream.XMLInputStream} */
@Deprecated
public static org.apache.xmlbeans.xml.stream.XMLInputStream newValidatingXMLInputStream(org.apache.xmlbeans.xml.stream.XMLInputStream xis) throws org.apache.xmlbeans.XmlException, org.apache.xmlbeans.xml.stream.XMLStreamException {
return org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newValidatingXMLInputStream( xis, type, null ); }
/** @deprecated {@link org.apache.xmlbeans.xml.stream.XMLInputStream} */
@Deprecated
public static org.apache.xmlbeans.xml.stream.XMLInputStream newValidatingXMLInputStream(org.apache.xmlbeans.xml.stream.XMLInputStream xis, org.apache.xmlbeans.XmlOptions options) throws org.apache.xmlbeans.XmlException, org.apache.xmlbeans.xml.stream.XMLStreamException {
return org.apache.xmlbeans.XmlBeans.getContextTypeLoader().newValidatingXMLInputStream( xis, type, options ); }
private Factory() { } // No instance of this class allowed
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy