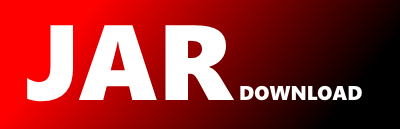
io.github.isotes.vs.model.impl.ClCompileDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: ClCompile
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.ClCompileDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model.impl;
/**
* A document containing one ClCompile(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public class ClCompileDocumentImpl extends io.github.isotes.vs.model.impl.ItemDocumentImpl implements io.github.isotes.vs.model.ClCompileDocument
{
private static final long serialVersionUID = 1L;
public ClCompileDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName CLCOMPILE$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ClCompile");
/**
* Gets the "ClCompile" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile getClCompile()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile)get_store().find_element_user(CLCOMPILE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "ClCompile" element
*/
public void setClCompile(io.github.isotes.vs.model.ClCompileDocument.ClCompile clCompile)
{
generatedSetterHelperImpl(clCompile, CLCOMPILE$0, 0, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_SINGLETON);
}
/**
* Appends and returns a new empty "ClCompile" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile addNewClCompile()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile)get_store().add_element_user(CLCOMPILE$0);
return target;
}
}
/**
* An XML ClCompile(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public static class ClCompileImpl extends io.github.isotes.vs.model.impl.SimpleItemTypeImpl implements io.github.isotes.vs.model.ClCompileDocument.ClCompile
{
private static final long serialVersionUID = 1L;
public ClCompileImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName PRECOMPILEDHEADER$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "PrecompiledHeader");
private static final javax.xml.namespace.QName ADDITIONALINCLUDEDIRECTORIES$2 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "AdditionalIncludeDirectories");
private static final javax.xml.namespace.QName ADDITIONALUSINGDIRECTORIES$4 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "AdditionalUsingDirectories");
private static final javax.xml.namespace.QName COMPILEASMANAGED$6 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "CompileAsManaged");
private static final javax.xml.namespace.QName ERRORREPORTING$8 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ErrorReporting");
private static final javax.xml.namespace.QName WARNINGLEVEL$10 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "WarningLevel");
private static final javax.xml.namespace.QName MINIMALREBUILD$12 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "MinimalRebuild");
private static final javax.xml.namespace.QName DEBUGINFORMATIONFORMAT$14 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "DebugInformationFormat");
private static final javax.xml.namespace.QName PREPROCESSORDEFINITIONS$16 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "PreprocessorDefinitions");
private static final javax.xml.namespace.QName OPTIMIZATION$18 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "Optimization");
private static final javax.xml.namespace.QName BASICRUNTIMECHECKS$20 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "BasicRuntimeChecks");
private static final javax.xml.namespace.QName RUNTIMELIBRARY$22 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "RuntimeLibrary");
private static final javax.xml.namespace.QName FUNCTIONLEVELLINKING$24 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "FunctionLevelLinking");
private static final javax.xml.namespace.QName FLOATINGPOINTMODEL$26 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "FloatingPointModel");
private static final javax.xml.namespace.QName INTRINSICFUNCTIONS$28 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "IntrinsicFunctions");
private static final javax.xml.namespace.QName PRECOMPILEDHEADERFILE$30 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "PrecompiledHeaderFile");
private static final javax.xml.namespace.QName MULTIPROCESSORCOMPILATION$32 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "MultiProcessorCompilation");
private static final javax.xml.namespace.QName USEUNICODEFORASSEMBLERLISTING$34 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "UseUnicodeForAssemblerListing");
private static final javax.xml.namespace.QName UNDEFINEPREPROCESSORDEFINITIONS$36 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "UndefinePreprocessorDefinitions");
private static final javax.xml.namespace.QName STRINGPOOLING$38 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "StringPooling");
private static final javax.xml.namespace.QName BROWSEINFORMATION$40 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "BrowseInformation");
private static final javax.xml.namespace.QName FLOATINGPOINTEXCEPTIONS$42 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "FloatingPointExceptions");
private static final javax.xml.namespace.QName CREATEHOTPATCHABLEIMAGE$44 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "CreateHotpatchableImage");
private static final javax.xml.namespace.QName RUNTIMETYPEINFO$46 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "RuntimeTypeInfo");
private static final javax.xml.namespace.QName OPENMPSUPPORT$48 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "OpenMPSupport");
private static final javax.xml.namespace.QName CALLINGCONVENTION$50 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "CallingConvention");
private static final javax.xml.namespace.QName DISABLESPECIFICWARNINGS$52 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "DisableSpecificWarnings");
private static final javax.xml.namespace.QName FORCEDINCLUDEFILES$54 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ForcedIncludeFiles");
private static final javax.xml.namespace.QName FORCEDUSINGFILES$56 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ForcedUsingFiles");
private static final javax.xml.namespace.QName SHOWINCLUDES$58 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ShowIncludes");
private static final javax.xml.namespace.QName USEFULLPATHS$60 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "UseFullPaths");
private static final javax.xml.namespace.QName OMITDEFAULTLIBNAME$62 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "OmitDefaultLibName");
private static final javax.xml.namespace.QName TREATSPECIFICWARNINGSASERRORS$64 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "TreatSpecificWarningsAsErrors");
/**
* Gets a List of "PrecompiledHeader" elements
*/
public java.util.List getPrecompiledHeaderList()
{
final class PrecompiledHeaderList extends java.util.AbstractList
{
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader get(int i)
{ return ClCompileImpl.this.getPrecompiledHeaderArray(i); }
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader set(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader o)
{
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader old = ClCompileImpl.this.getPrecompiledHeaderArray(i);
ClCompileImpl.this.setPrecompiledHeaderArray(i, o);
return old;
}
@Override
public void add(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader o)
{ ClCompileImpl.this.insertNewPrecompiledHeader(i).set(o); }
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader remove(int i)
{
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader old = ClCompileImpl.this.getPrecompiledHeaderArray(i);
ClCompileImpl.this.removePrecompiledHeader(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfPrecompiledHeaderArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new PrecompiledHeaderList();
}
}
/**
* Gets array of all "PrecompiledHeader" elements
* @deprecated
*/
@Deprecated
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[] getPrecompiledHeaderArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(PRECOMPILEDHEADER$0, targetList);
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[] result = new io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "PrecompiledHeader" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader getPrecompiledHeaderArray(int i)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader)get_store().find_element_user(PRECOMPILEDHEADER$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "PrecompiledHeader" element
*/
public int sizeOfPrecompiledHeaderArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(PRECOMPILEDHEADER$0);
}
}
/**
* Sets array of all "PrecompiledHeader" element WARNING: This method is not atomicaly synchronized.
*/
public void setPrecompiledHeaderArray(io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader[] precompiledHeaderArray)
{
check_orphaned();
arraySetterHelper(precompiledHeaderArray, PRECOMPILEDHEADER$0);
}
/**
* Sets ith "PrecompiledHeader" element
*/
public void setPrecompiledHeaderArray(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader precompiledHeader)
{
generatedSetterHelperImpl(precompiledHeader, PRECOMPILEDHEADER$0, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "PrecompiledHeader" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader insertNewPrecompiledHeader(int i)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader)get_store().insert_element_user(PRECOMPILEDHEADER$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "PrecompiledHeader" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader addNewPrecompiledHeader()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader)get_store().add_element_user(PRECOMPILEDHEADER$0);
return target;
}
}
/**
* Removes the ith "PrecompiledHeader" element
*/
public void removePrecompiledHeader(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(PRECOMPILEDHEADER$0, i);
}
}
/**
* Gets a List of "AdditionalIncludeDirectories" elements
*/
public java.util.List getAdditionalIncludeDirectoriesList()
{
final class AdditionalIncludeDirectoriesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getAdditionalIncludeDirectoriesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getAdditionalIncludeDirectoriesArray(i);
ClCompileImpl.this.setAdditionalIncludeDirectoriesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewAdditionalIncludeDirectories(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getAdditionalIncludeDirectoriesArray(i);
ClCompileImpl.this.removeAdditionalIncludeDirectories(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfAdditionalIncludeDirectoriesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new AdditionalIncludeDirectoriesList();
}
}
/**
* Gets array of all "AdditionalIncludeDirectories" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getAdditionalIncludeDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ADDITIONALINCLUDEDIRECTORIES$2, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject getAdditionalIncludeDirectoriesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(ADDITIONALINCLUDEDIRECTORIES$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "AdditionalIncludeDirectories" element
*/
public int sizeOfAdditionalIncludeDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ADDITIONALINCLUDEDIRECTORIES$2);
}
}
/**
* Sets array of all "AdditionalIncludeDirectories" element WARNING: This method is not atomicaly synchronized.
*/
public void setAdditionalIncludeDirectoriesArray(org.apache.xmlbeans.XmlObject[] additionalIncludeDirectoriesArray)
{
check_orphaned();
arraySetterHelper(additionalIncludeDirectoriesArray, ADDITIONALINCLUDEDIRECTORIES$2);
}
/**
* Sets ith "AdditionalIncludeDirectories" element
*/
public void setAdditionalIncludeDirectoriesArray(int i, org.apache.xmlbeans.XmlObject additionalIncludeDirectories)
{
generatedSetterHelperImpl(additionalIncludeDirectories, ADDITIONALINCLUDEDIRECTORIES$2, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject insertNewAdditionalIncludeDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(ADDITIONALINCLUDEDIRECTORIES$2, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject addNewAdditionalIncludeDirectories()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(ADDITIONALINCLUDEDIRECTORIES$2);
return target;
}
}
/**
* Removes the ith "AdditionalIncludeDirectories" element
*/
public void removeAdditionalIncludeDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ADDITIONALINCLUDEDIRECTORIES$2, i);
}
}
/**
* Gets a List of "AdditionalUsingDirectories" elements
*/
public java.util.List getAdditionalUsingDirectoriesList()
{
final class AdditionalUsingDirectoriesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getAdditionalUsingDirectoriesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getAdditionalUsingDirectoriesArray(i);
ClCompileImpl.this.setAdditionalUsingDirectoriesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewAdditionalUsingDirectories(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getAdditionalUsingDirectoriesArray(i);
ClCompileImpl.this.removeAdditionalUsingDirectories(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfAdditionalUsingDirectoriesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new AdditionalUsingDirectoriesList();
}
}
/**
* Gets array of all "AdditionalUsingDirectories" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getAdditionalUsingDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ADDITIONALUSINGDIRECTORIES$4, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "AdditionalUsingDirectories" element
*/
public org.apache.xmlbeans.XmlObject getAdditionalUsingDirectoriesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(ADDITIONALUSINGDIRECTORIES$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "AdditionalUsingDirectories" element
*/
public int sizeOfAdditionalUsingDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ADDITIONALUSINGDIRECTORIES$4);
}
}
/**
* Sets array of all "AdditionalUsingDirectories" element WARNING: This method is not atomicaly synchronized.
*/
public void setAdditionalUsingDirectoriesArray(org.apache.xmlbeans.XmlObject[] additionalUsingDirectoriesArray)
{
check_orphaned();
arraySetterHelper(additionalUsingDirectoriesArray, ADDITIONALUSINGDIRECTORIES$4);
}
/**
* Sets ith "AdditionalUsingDirectories" element
*/
public void setAdditionalUsingDirectoriesArray(int i, org.apache.xmlbeans.XmlObject additionalUsingDirectories)
{
generatedSetterHelperImpl(additionalUsingDirectories, ADDITIONALUSINGDIRECTORIES$4, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "AdditionalUsingDirectories" element
*/
public org.apache.xmlbeans.XmlObject insertNewAdditionalUsingDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(ADDITIONALUSINGDIRECTORIES$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "AdditionalUsingDirectories" element
*/
public org.apache.xmlbeans.XmlObject addNewAdditionalUsingDirectories()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(ADDITIONALUSINGDIRECTORIES$4);
return target;
}
}
/**
* Removes the ith "AdditionalUsingDirectories" element
*/
public void removeAdditionalUsingDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ADDITIONALUSINGDIRECTORIES$4, i);
}
}
/**
* Gets a List of "CompileAsManaged" elements
*/
public java.util.List getCompileAsManagedList()
{
final class CompileAsManagedList extends java.util.AbstractList
{
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged get(int i)
{ return ClCompileImpl.this.getCompileAsManagedArray(i); }
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged set(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged o)
{
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged old = ClCompileImpl.this.getCompileAsManagedArray(i);
ClCompileImpl.this.setCompileAsManagedArray(i, o);
return old;
}
@Override
public void add(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged o)
{ ClCompileImpl.this.insertNewCompileAsManaged(i).set(o); }
@Override
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged remove(int i)
{
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged old = ClCompileImpl.this.getCompileAsManagedArray(i);
ClCompileImpl.this.removeCompileAsManaged(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfCompileAsManagedArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new CompileAsManagedList();
}
}
/**
* Gets array of all "CompileAsManaged" elements
* @deprecated
*/
@Deprecated
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[] getCompileAsManagedArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(COMPILEASMANAGED$6, targetList);
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[] result = new io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "CompileAsManaged" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged getCompileAsManagedArray(int i)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged)get_store().find_element_user(COMPILEASMANAGED$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "CompileAsManaged" element
*/
public int sizeOfCompileAsManagedArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(COMPILEASMANAGED$6);
}
}
/**
* Sets array of all "CompileAsManaged" element WARNING: This method is not atomicaly synchronized.
*/
public void setCompileAsManagedArray(io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged[] compileAsManagedArray)
{
check_orphaned();
arraySetterHelper(compileAsManagedArray, COMPILEASMANAGED$6);
}
/**
* Sets ith "CompileAsManaged" element
*/
public void setCompileAsManagedArray(int i, io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged compileAsManaged)
{
generatedSetterHelperImpl(compileAsManaged, COMPILEASMANAGED$6, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "CompileAsManaged" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged insertNewCompileAsManaged(int i)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged)get_store().insert_element_user(COMPILEASMANAGED$6, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "CompileAsManaged" element
*/
public io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged addNewCompileAsManaged()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged target = null;
target = (io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged)get_store().add_element_user(COMPILEASMANAGED$6);
return target;
}
}
/**
* Removes the ith "CompileAsManaged" element
*/
public void removeCompileAsManaged(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(COMPILEASMANAGED$6, i);
}
}
/**
* Gets a List of "ErrorReporting" elements
*/
public java.util.List getErrorReportingList()
{
final class ErrorReportingList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getErrorReportingArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getErrorReportingArray(i);
ClCompileImpl.this.setErrorReportingArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewErrorReporting(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getErrorReportingArray(i);
ClCompileImpl.this.removeErrorReporting(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfErrorReportingArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new ErrorReportingList();
}
}
/**
* Gets array of all "ErrorReporting" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getErrorReportingArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ERRORREPORTING$8, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "ErrorReporting" element
*/
public org.apache.xmlbeans.XmlObject getErrorReportingArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(ERRORREPORTING$8, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "ErrorReporting" element
*/
public int sizeOfErrorReportingArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ERRORREPORTING$8);
}
}
/**
* Sets array of all "ErrorReporting" element WARNING: This method is not atomicaly synchronized.
*/
public void setErrorReportingArray(org.apache.xmlbeans.XmlObject[] errorReportingArray)
{
check_orphaned();
arraySetterHelper(errorReportingArray, ERRORREPORTING$8);
}
/**
* Sets ith "ErrorReporting" element
*/
public void setErrorReportingArray(int i, org.apache.xmlbeans.XmlObject errorReporting)
{
generatedSetterHelperImpl(errorReporting, ERRORREPORTING$8, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "ErrorReporting" element
*/
public org.apache.xmlbeans.XmlObject insertNewErrorReporting(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(ERRORREPORTING$8, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "ErrorReporting" element
*/
public org.apache.xmlbeans.XmlObject addNewErrorReporting()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(ERRORREPORTING$8);
return target;
}
}
/**
* Removes the ith "ErrorReporting" element
*/
public void removeErrorReporting(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ERRORREPORTING$8, i);
}
}
/**
* Gets a List of "WarningLevel" elements
*/
public java.util.List getWarningLevelList()
{
final class WarningLevelList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getWarningLevelArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getWarningLevelArray(i);
ClCompileImpl.this.setWarningLevelArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewWarningLevel(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getWarningLevelArray(i);
ClCompileImpl.this.removeWarningLevel(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfWarningLevelArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new WarningLevelList();
}
}
/**
* Gets array of all "WarningLevel" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getWarningLevelArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(WARNINGLEVEL$10, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "WarningLevel" element
*/
public org.apache.xmlbeans.XmlObject getWarningLevelArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(WARNINGLEVEL$10, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "WarningLevel" element
*/
public int sizeOfWarningLevelArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(WARNINGLEVEL$10);
}
}
/**
* Sets array of all "WarningLevel" element WARNING: This method is not atomicaly synchronized.
*/
public void setWarningLevelArray(org.apache.xmlbeans.XmlObject[] warningLevelArray)
{
check_orphaned();
arraySetterHelper(warningLevelArray, WARNINGLEVEL$10);
}
/**
* Sets ith "WarningLevel" element
*/
public void setWarningLevelArray(int i, org.apache.xmlbeans.XmlObject warningLevel)
{
generatedSetterHelperImpl(warningLevel, WARNINGLEVEL$10, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "WarningLevel" element
*/
public org.apache.xmlbeans.XmlObject insertNewWarningLevel(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(WARNINGLEVEL$10, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "WarningLevel" element
*/
public org.apache.xmlbeans.XmlObject addNewWarningLevel()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(WARNINGLEVEL$10);
return target;
}
}
/**
* Removes the ith "WarningLevel" element
*/
public void removeWarningLevel(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(WARNINGLEVEL$10, i);
}
}
/**
* Gets a List of "MinimalRebuild" elements
*/
public java.util.List getMinimalRebuildList()
{
final class MinimalRebuildList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getMinimalRebuildArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getMinimalRebuildArray(i);
ClCompileImpl.this.setMinimalRebuildArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewMinimalRebuild(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getMinimalRebuildArray(i);
ClCompileImpl.this.removeMinimalRebuild(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfMinimalRebuildArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new MinimalRebuildList();
}
}
/**
* Gets array of all "MinimalRebuild" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getMinimalRebuildArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(MINIMALREBUILD$12, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "MinimalRebuild" element
*/
public org.apache.xmlbeans.XmlObject getMinimalRebuildArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(MINIMALREBUILD$12, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "MinimalRebuild" element
*/
public int sizeOfMinimalRebuildArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(MINIMALREBUILD$12);
}
}
/**
* Sets array of all "MinimalRebuild" element WARNING: This method is not atomicaly synchronized.
*/
public void setMinimalRebuildArray(org.apache.xmlbeans.XmlObject[] minimalRebuildArray)
{
check_orphaned();
arraySetterHelper(minimalRebuildArray, MINIMALREBUILD$12);
}
/**
* Sets ith "MinimalRebuild" element
*/
public void setMinimalRebuildArray(int i, org.apache.xmlbeans.XmlObject minimalRebuild)
{
generatedSetterHelperImpl(minimalRebuild, MINIMALREBUILD$12, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "MinimalRebuild" element
*/
public org.apache.xmlbeans.XmlObject insertNewMinimalRebuild(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(MINIMALREBUILD$12, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "MinimalRebuild" element
*/
public org.apache.xmlbeans.XmlObject addNewMinimalRebuild()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(MINIMALREBUILD$12);
return target;
}
}
/**
* Removes the ith "MinimalRebuild" element
*/
public void removeMinimalRebuild(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(MINIMALREBUILD$12, i);
}
}
/**
* Gets a List of "DebugInformationFormat" elements
*/
public java.util.List getDebugInformationFormatList()
{
final class DebugInformationFormatList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getDebugInformationFormatArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getDebugInformationFormatArray(i);
ClCompileImpl.this.setDebugInformationFormatArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewDebugInformationFormat(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getDebugInformationFormatArray(i);
ClCompileImpl.this.removeDebugInformationFormat(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfDebugInformationFormatArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new DebugInformationFormatList();
}
}
/**
* Gets array of all "DebugInformationFormat" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getDebugInformationFormatArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DEBUGINFORMATIONFORMAT$14, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "DebugInformationFormat" element
*/
public org.apache.xmlbeans.XmlObject getDebugInformationFormatArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(DEBUGINFORMATIONFORMAT$14, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "DebugInformationFormat" element
*/
public int sizeOfDebugInformationFormatArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DEBUGINFORMATIONFORMAT$14);
}
}
/**
* Sets array of all "DebugInformationFormat" element WARNING: This method is not atomicaly synchronized.
*/
public void setDebugInformationFormatArray(org.apache.xmlbeans.XmlObject[] debugInformationFormatArray)
{
check_orphaned();
arraySetterHelper(debugInformationFormatArray, DEBUGINFORMATIONFORMAT$14);
}
/**
* Sets ith "DebugInformationFormat" element
*/
public void setDebugInformationFormatArray(int i, org.apache.xmlbeans.XmlObject debugInformationFormat)
{
generatedSetterHelperImpl(debugInformationFormat, DEBUGINFORMATIONFORMAT$14, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "DebugInformationFormat" element
*/
public org.apache.xmlbeans.XmlObject insertNewDebugInformationFormat(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(DEBUGINFORMATIONFORMAT$14, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "DebugInformationFormat" element
*/
public org.apache.xmlbeans.XmlObject addNewDebugInformationFormat()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(DEBUGINFORMATIONFORMAT$14);
return target;
}
}
/**
* Removes the ith "DebugInformationFormat" element
*/
public void removeDebugInformationFormat(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DEBUGINFORMATIONFORMAT$14, i);
}
}
/**
* Gets a List of "PreprocessorDefinitions" elements
*/
public java.util.List getPreprocessorDefinitionsList()
{
final class PreprocessorDefinitionsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getPreprocessorDefinitionsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getPreprocessorDefinitionsArray(i);
ClCompileImpl.this.setPreprocessorDefinitionsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewPreprocessorDefinitions(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getPreprocessorDefinitionsArray(i);
ClCompileImpl.this.removePreprocessorDefinitions(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfPreprocessorDefinitionsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new PreprocessorDefinitionsList();
}
}
/**
* Gets array of all "PreprocessorDefinitions" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getPreprocessorDefinitionsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(PREPROCESSORDEFINITIONS$16, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "PreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject getPreprocessorDefinitionsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(PREPROCESSORDEFINITIONS$16, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "PreprocessorDefinitions" element
*/
public int sizeOfPreprocessorDefinitionsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(PREPROCESSORDEFINITIONS$16);
}
}
/**
* Sets array of all "PreprocessorDefinitions" element WARNING: This method is not atomicaly synchronized.
*/
public void setPreprocessorDefinitionsArray(org.apache.xmlbeans.XmlObject[] preprocessorDefinitionsArray)
{
check_orphaned();
arraySetterHelper(preprocessorDefinitionsArray, PREPROCESSORDEFINITIONS$16);
}
/**
* Sets ith "PreprocessorDefinitions" element
*/
public void setPreprocessorDefinitionsArray(int i, org.apache.xmlbeans.XmlObject preprocessorDefinitions)
{
generatedSetterHelperImpl(preprocessorDefinitions, PREPROCESSORDEFINITIONS$16, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "PreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject insertNewPreprocessorDefinitions(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(PREPROCESSORDEFINITIONS$16, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "PreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject addNewPreprocessorDefinitions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(PREPROCESSORDEFINITIONS$16);
return target;
}
}
/**
* Removes the ith "PreprocessorDefinitions" element
*/
public void removePreprocessorDefinitions(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(PREPROCESSORDEFINITIONS$16, i);
}
}
/**
* Gets a List of "Optimization" elements
*/
public java.util.List getOptimizationList()
{
final class OptimizationList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getOptimizationArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOptimizationArray(i);
ClCompileImpl.this.setOptimizationArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewOptimization(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOptimizationArray(i);
ClCompileImpl.this.removeOptimization(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfOptimizationArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new OptimizationList();
}
}
/**
* Gets array of all "Optimization" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getOptimizationArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(OPTIMIZATION$18, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "Optimization" element
*/
public org.apache.xmlbeans.XmlObject getOptimizationArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(OPTIMIZATION$18, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "Optimization" element
*/
public int sizeOfOptimizationArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(OPTIMIZATION$18);
}
}
/**
* Sets array of all "Optimization" element WARNING: This method is not atomicaly synchronized.
*/
public void setOptimizationArray(org.apache.xmlbeans.XmlObject[] optimizationArray)
{
check_orphaned();
arraySetterHelper(optimizationArray, OPTIMIZATION$18);
}
/**
* Sets ith "Optimization" element
*/
public void setOptimizationArray(int i, org.apache.xmlbeans.XmlObject optimization)
{
generatedSetterHelperImpl(optimization, OPTIMIZATION$18, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "Optimization" element
*/
public org.apache.xmlbeans.XmlObject insertNewOptimization(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(OPTIMIZATION$18, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "Optimization" element
*/
public org.apache.xmlbeans.XmlObject addNewOptimization()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(OPTIMIZATION$18);
return target;
}
}
/**
* Removes the ith "Optimization" element
*/
public void removeOptimization(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(OPTIMIZATION$18, i);
}
}
/**
* Gets a List of "BasicRuntimeChecks" elements
*/
public java.util.List getBasicRuntimeChecksList()
{
final class BasicRuntimeChecksList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getBasicRuntimeChecksArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getBasicRuntimeChecksArray(i);
ClCompileImpl.this.setBasicRuntimeChecksArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewBasicRuntimeChecks(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getBasicRuntimeChecksArray(i);
ClCompileImpl.this.removeBasicRuntimeChecks(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfBasicRuntimeChecksArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new BasicRuntimeChecksList();
}
}
/**
* Gets array of all "BasicRuntimeChecks" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getBasicRuntimeChecksArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(BASICRUNTIMECHECKS$20, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "BasicRuntimeChecks" element
*/
public org.apache.xmlbeans.XmlObject getBasicRuntimeChecksArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(BASICRUNTIMECHECKS$20, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "BasicRuntimeChecks" element
*/
public int sizeOfBasicRuntimeChecksArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(BASICRUNTIMECHECKS$20);
}
}
/**
* Sets array of all "BasicRuntimeChecks" element WARNING: This method is not atomicaly synchronized.
*/
public void setBasicRuntimeChecksArray(org.apache.xmlbeans.XmlObject[] basicRuntimeChecksArray)
{
check_orphaned();
arraySetterHelper(basicRuntimeChecksArray, BASICRUNTIMECHECKS$20);
}
/**
* Sets ith "BasicRuntimeChecks" element
*/
public void setBasicRuntimeChecksArray(int i, org.apache.xmlbeans.XmlObject basicRuntimeChecks)
{
generatedSetterHelperImpl(basicRuntimeChecks, BASICRUNTIMECHECKS$20, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "BasicRuntimeChecks" element
*/
public org.apache.xmlbeans.XmlObject insertNewBasicRuntimeChecks(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(BASICRUNTIMECHECKS$20, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "BasicRuntimeChecks" element
*/
public org.apache.xmlbeans.XmlObject addNewBasicRuntimeChecks()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(BASICRUNTIMECHECKS$20);
return target;
}
}
/**
* Removes the ith "BasicRuntimeChecks" element
*/
public void removeBasicRuntimeChecks(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(BASICRUNTIMECHECKS$20, i);
}
}
/**
* Gets a List of "RuntimeLibrary" elements
*/
public java.util.List getRuntimeLibraryList()
{
final class RuntimeLibraryList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getRuntimeLibraryArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getRuntimeLibraryArray(i);
ClCompileImpl.this.setRuntimeLibraryArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewRuntimeLibrary(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getRuntimeLibraryArray(i);
ClCompileImpl.this.removeRuntimeLibrary(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfRuntimeLibraryArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new RuntimeLibraryList();
}
}
/**
* Gets array of all "RuntimeLibrary" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getRuntimeLibraryArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(RUNTIMELIBRARY$22, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "RuntimeLibrary" element
*/
public org.apache.xmlbeans.XmlObject getRuntimeLibraryArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(RUNTIMELIBRARY$22, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "RuntimeLibrary" element
*/
public int sizeOfRuntimeLibraryArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(RUNTIMELIBRARY$22);
}
}
/**
* Sets array of all "RuntimeLibrary" element WARNING: This method is not atomicaly synchronized.
*/
public void setRuntimeLibraryArray(org.apache.xmlbeans.XmlObject[] runtimeLibraryArray)
{
check_orphaned();
arraySetterHelper(runtimeLibraryArray, RUNTIMELIBRARY$22);
}
/**
* Sets ith "RuntimeLibrary" element
*/
public void setRuntimeLibraryArray(int i, org.apache.xmlbeans.XmlObject runtimeLibrary)
{
generatedSetterHelperImpl(runtimeLibrary, RUNTIMELIBRARY$22, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "RuntimeLibrary" element
*/
public org.apache.xmlbeans.XmlObject insertNewRuntimeLibrary(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(RUNTIMELIBRARY$22, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "RuntimeLibrary" element
*/
public org.apache.xmlbeans.XmlObject addNewRuntimeLibrary()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(RUNTIMELIBRARY$22);
return target;
}
}
/**
* Removes the ith "RuntimeLibrary" element
*/
public void removeRuntimeLibrary(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(RUNTIMELIBRARY$22, i);
}
}
/**
* Gets a List of "FunctionLevelLinking" elements
*/
public java.util.List getFunctionLevelLinkingList()
{
final class FunctionLevelLinkingList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getFunctionLevelLinkingArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFunctionLevelLinkingArray(i);
ClCompileImpl.this.setFunctionLevelLinkingArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewFunctionLevelLinking(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFunctionLevelLinkingArray(i);
ClCompileImpl.this.removeFunctionLevelLinking(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfFunctionLevelLinkingArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new FunctionLevelLinkingList();
}
}
/**
* Gets array of all "FunctionLevelLinking" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getFunctionLevelLinkingArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(FUNCTIONLEVELLINKING$24, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "FunctionLevelLinking" element
*/
public org.apache.xmlbeans.XmlObject getFunctionLevelLinkingArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(FUNCTIONLEVELLINKING$24, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "FunctionLevelLinking" element
*/
public int sizeOfFunctionLevelLinkingArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(FUNCTIONLEVELLINKING$24);
}
}
/**
* Sets array of all "FunctionLevelLinking" element WARNING: This method is not atomicaly synchronized.
*/
public void setFunctionLevelLinkingArray(org.apache.xmlbeans.XmlObject[] functionLevelLinkingArray)
{
check_orphaned();
arraySetterHelper(functionLevelLinkingArray, FUNCTIONLEVELLINKING$24);
}
/**
* Sets ith "FunctionLevelLinking" element
*/
public void setFunctionLevelLinkingArray(int i, org.apache.xmlbeans.XmlObject functionLevelLinking)
{
generatedSetterHelperImpl(functionLevelLinking, FUNCTIONLEVELLINKING$24, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "FunctionLevelLinking" element
*/
public org.apache.xmlbeans.XmlObject insertNewFunctionLevelLinking(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(FUNCTIONLEVELLINKING$24, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "FunctionLevelLinking" element
*/
public org.apache.xmlbeans.XmlObject addNewFunctionLevelLinking()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(FUNCTIONLEVELLINKING$24);
return target;
}
}
/**
* Removes the ith "FunctionLevelLinking" element
*/
public void removeFunctionLevelLinking(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(FUNCTIONLEVELLINKING$24, i);
}
}
/**
* Gets a List of "FloatingPointModel" elements
*/
public java.util.List getFloatingPointModelList()
{
final class FloatingPointModelList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getFloatingPointModelArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFloatingPointModelArray(i);
ClCompileImpl.this.setFloatingPointModelArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewFloatingPointModel(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFloatingPointModelArray(i);
ClCompileImpl.this.removeFloatingPointModel(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfFloatingPointModelArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new FloatingPointModelList();
}
}
/**
* Gets array of all "FloatingPointModel" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getFloatingPointModelArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(FLOATINGPOINTMODEL$26, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "FloatingPointModel" element
*/
public org.apache.xmlbeans.XmlObject getFloatingPointModelArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(FLOATINGPOINTMODEL$26, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "FloatingPointModel" element
*/
public int sizeOfFloatingPointModelArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(FLOATINGPOINTMODEL$26);
}
}
/**
* Sets array of all "FloatingPointModel" element WARNING: This method is not atomicaly synchronized.
*/
public void setFloatingPointModelArray(org.apache.xmlbeans.XmlObject[] floatingPointModelArray)
{
check_orphaned();
arraySetterHelper(floatingPointModelArray, FLOATINGPOINTMODEL$26);
}
/**
* Sets ith "FloatingPointModel" element
*/
public void setFloatingPointModelArray(int i, org.apache.xmlbeans.XmlObject floatingPointModel)
{
generatedSetterHelperImpl(floatingPointModel, FLOATINGPOINTMODEL$26, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "FloatingPointModel" element
*/
public org.apache.xmlbeans.XmlObject insertNewFloatingPointModel(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(FLOATINGPOINTMODEL$26, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "FloatingPointModel" element
*/
public org.apache.xmlbeans.XmlObject addNewFloatingPointModel()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(FLOATINGPOINTMODEL$26);
return target;
}
}
/**
* Removes the ith "FloatingPointModel" element
*/
public void removeFloatingPointModel(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(FLOATINGPOINTMODEL$26, i);
}
}
/**
* Gets a List of "IntrinsicFunctions" elements
*/
public java.util.List getIntrinsicFunctionsList()
{
final class IntrinsicFunctionsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getIntrinsicFunctionsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getIntrinsicFunctionsArray(i);
ClCompileImpl.this.setIntrinsicFunctionsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewIntrinsicFunctions(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getIntrinsicFunctionsArray(i);
ClCompileImpl.this.removeIntrinsicFunctions(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfIntrinsicFunctionsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new IntrinsicFunctionsList();
}
}
/**
* Gets array of all "IntrinsicFunctions" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getIntrinsicFunctionsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(INTRINSICFUNCTIONS$28, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "IntrinsicFunctions" element
*/
public org.apache.xmlbeans.XmlObject getIntrinsicFunctionsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(INTRINSICFUNCTIONS$28, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "IntrinsicFunctions" element
*/
public int sizeOfIntrinsicFunctionsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(INTRINSICFUNCTIONS$28);
}
}
/**
* Sets array of all "IntrinsicFunctions" element WARNING: This method is not atomicaly synchronized.
*/
public void setIntrinsicFunctionsArray(org.apache.xmlbeans.XmlObject[] intrinsicFunctionsArray)
{
check_orphaned();
arraySetterHelper(intrinsicFunctionsArray, INTRINSICFUNCTIONS$28);
}
/**
* Sets ith "IntrinsicFunctions" element
*/
public void setIntrinsicFunctionsArray(int i, org.apache.xmlbeans.XmlObject intrinsicFunctions)
{
generatedSetterHelperImpl(intrinsicFunctions, INTRINSICFUNCTIONS$28, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "IntrinsicFunctions" element
*/
public org.apache.xmlbeans.XmlObject insertNewIntrinsicFunctions(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(INTRINSICFUNCTIONS$28, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "IntrinsicFunctions" element
*/
public org.apache.xmlbeans.XmlObject addNewIntrinsicFunctions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(INTRINSICFUNCTIONS$28);
return target;
}
}
/**
* Removes the ith "IntrinsicFunctions" element
*/
public void removeIntrinsicFunctions(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(INTRINSICFUNCTIONS$28, i);
}
}
/**
* Gets a List of "PrecompiledHeaderFile" elements
*/
public java.util.List getPrecompiledHeaderFileList()
{
final class PrecompiledHeaderFileList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getPrecompiledHeaderFileArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getPrecompiledHeaderFileArray(i);
ClCompileImpl.this.setPrecompiledHeaderFileArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewPrecompiledHeaderFile(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getPrecompiledHeaderFileArray(i);
ClCompileImpl.this.removePrecompiledHeaderFile(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfPrecompiledHeaderFileArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new PrecompiledHeaderFileList();
}
}
/**
* Gets array of all "PrecompiledHeaderFile" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getPrecompiledHeaderFileArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(PRECOMPILEDHEADERFILE$30, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "PrecompiledHeaderFile" element
*/
public org.apache.xmlbeans.XmlObject getPrecompiledHeaderFileArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(PRECOMPILEDHEADERFILE$30, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "PrecompiledHeaderFile" element
*/
public int sizeOfPrecompiledHeaderFileArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(PRECOMPILEDHEADERFILE$30);
}
}
/**
* Sets array of all "PrecompiledHeaderFile" element WARNING: This method is not atomicaly synchronized.
*/
public void setPrecompiledHeaderFileArray(org.apache.xmlbeans.XmlObject[] precompiledHeaderFileArray)
{
check_orphaned();
arraySetterHelper(precompiledHeaderFileArray, PRECOMPILEDHEADERFILE$30);
}
/**
* Sets ith "PrecompiledHeaderFile" element
*/
public void setPrecompiledHeaderFileArray(int i, org.apache.xmlbeans.XmlObject precompiledHeaderFile)
{
generatedSetterHelperImpl(precompiledHeaderFile, PRECOMPILEDHEADERFILE$30, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "PrecompiledHeaderFile" element
*/
public org.apache.xmlbeans.XmlObject insertNewPrecompiledHeaderFile(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(PRECOMPILEDHEADERFILE$30, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "PrecompiledHeaderFile" element
*/
public org.apache.xmlbeans.XmlObject addNewPrecompiledHeaderFile()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(PRECOMPILEDHEADERFILE$30);
return target;
}
}
/**
* Removes the ith "PrecompiledHeaderFile" element
*/
public void removePrecompiledHeaderFile(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(PRECOMPILEDHEADERFILE$30, i);
}
}
/**
* Gets a List of "MultiProcessorCompilation" elements
*/
public java.util.List getMultiProcessorCompilationList()
{
final class MultiProcessorCompilationList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getMultiProcessorCompilationArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getMultiProcessorCompilationArray(i);
ClCompileImpl.this.setMultiProcessorCompilationArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewMultiProcessorCompilation(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getMultiProcessorCompilationArray(i);
ClCompileImpl.this.removeMultiProcessorCompilation(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfMultiProcessorCompilationArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new MultiProcessorCompilationList();
}
}
/**
* Gets array of all "MultiProcessorCompilation" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getMultiProcessorCompilationArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(MULTIPROCESSORCOMPILATION$32, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "MultiProcessorCompilation" element
*/
public org.apache.xmlbeans.XmlObject getMultiProcessorCompilationArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(MULTIPROCESSORCOMPILATION$32, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "MultiProcessorCompilation" element
*/
public int sizeOfMultiProcessorCompilationArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(MULTIPROCESSORCOMPILATION$32);
}
}
/**
* Sets array of all "MultiProcessorCompilation" element WARNING: This method is not atomicaly synchronized.
*/
public void setMultiProcessorCompilationArray(org.apache.xmlbeans.XmlObject[] multiProcessorCompilationArray)
{
check_orphaned();
arraySetterHelper(multiProcessorCompilationArray, MULTIPROCESSORCOMPILATION$32);
}
/**
* Sets ith "MultiProcessorCompilation" element
*/
public void setMultiProcessorCompilationArray(int i, org.apache.xmlbeans.XmlObject multiProcessorCompilation)
{
generatedSetterHelperImpl(multiProcessorCompilation, MULTIPROCESSORCOMPILATION$32, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "MultiProcessorCompilation" element
*/
public org.apache.xmlbeans.XmlObject insertNewMultiProcessorCompilation(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(MULTIPROCESSORCOMPILATION$32, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "MultiProcessorCompilation" element
*/
public org.apache.xmlbeans.XmlObject addNewMultiProcessorCompilation()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(MULTIPROCESSORCOMPILATION$32);
return target;
}
}
/**
* Removes the ith "MultiProcessorCompilation" element
*/
public void removeMultiProcessorCompilation(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(MULTIPROCESSORCOMPILATION$32, i);
}
}
/**
* Gets a List of "UseUnicodeForAssemblerListing" elements
*/
public java.util.List getUseUnicodeForAssemblerListingList()
{
final class UseUnicodeForAssemblerListingList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getUseUnicodeForAssemblerListingArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUseUnicodeForAssemblerListingArray(i);
ClCompileImpl.this.setUseUnicodeForAssemblerListingArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewUseUnicodeForAssemblerListing(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUseUnicodeForAssemblerListingArray(i);
ClCompileImpl.this.removeUseUnicodeForAssemblerListing(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfUseUnicodeForAssemblerListingArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new UseUnicodeForAssemblerListingList();
}
}
/**
* Gets array of all "UseUnicodeForAssemblerListing" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getUseUnicodeForAssemblerListingArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(USEUNICODEFORASSEMBLERLISTING$34, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "UseUnicodeForAssemblerListing" element
*/
public org.apache.xmlbeans.XmlObject getUseUnicodeForAssemblerListingArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(USEUNICODEFORASSEMBLERLISTING$34, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "UseUnicodeForAssemblerListing" element
*/
public int sizeOfUseUnicodeForAssemblerListingArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(USEUNICODEFORASSEMBLERLISTING$34);
}
}
/**
* Sets array of all "UseUnicodeForAssemblerListing" element WARNING: This method is not atomicaly synchronized.
*/
public void setUseUnicodeForAssemblerListingArray(org.apache.xmlbeans.XmlObject[] useUnicodeForAssemblerListingArray)
{
check_orphaned();
arraySetterHelper(useUnicodeForAssemblerListingArray, USEUNICODEFORASSEMBLERLISTING$34);
}
/**
* Sets ith "UseUnicodeForAssemblerListing" element
*/
public void setUseUnicodeForAssemblerListingArray(int i, org.apache.xmlbeans.XmlObject useUnicodeForAssemblerListing)
{
generatedSetterHelperImpl(useUnicodeForAssemblerListing, USEUNICODEFORASSEMBLERLISTING$34, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "UseUnicodeForAssemblerListing" element
*/
public org.apache.xmlbeans.XmlObject insertNewUseUnicodeForAssemblerListing(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(USEUNICODEFORASSEMBLERLISTING$34, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "UseUnicodeForAssemblerListing" element
*/
public org.apache.xmlbeans.XmlObject addNewUseUnicodeForAssemblerListing()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(USEUNICODEFORASSEMBLERLISTING$34);
return target;
}
}
/**
* Removes the ith "UseUnicodeForAssemblerListing" element
*/
public void removeUseUnicodeForAssemblerListing(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(USEUNICODEFORASSEMBLERLISTING$34, i);
}
}
/**
* Gets a List of "UndefinePreprocessorDefinitions" elements
*/
public java.util.List getUndefinePreprocessorDefinitionsList()
{
final class UndefinePreprocessorDefinitionsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getUndefinePreprocessorDefinitionsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUndefinePreprocessorDefinitionsArray(i);
ClCompileImpl.this.setUndefinePreprocessorDefinitionsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewUndefinePreprocessorDefinitions(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUndefinePreprocessorDefinitionsArray(i);
ClCompileImpl.this.removeUndefinePreprocessorDefinitions(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfUndefinePreprocessorDefinitionsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new UndefinePreprocessorDefinitionsList();
}
}
/**
* Gets array of all "UndefinePreprocessorDefinitions" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getUndefinePreprocessorDefinitionsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(UNDEFINEPREPROCESSORDEFINITIONS$36, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "UndefinePreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject getUndefinePreprocessorDefinitionsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(UNDEFINEPREPROCESSORDEFINITIONS$36, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "UndefinePreprocessorDefinitions" element
*/
public int sizeOfUndefinePreprocessorDefinitionsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(UNDEFINEPREPROCESSORDEFINITIONS$36);
}
}
/**
* Sets array of all "UndefinePreprocessorDefinitions" element WARNING: This method is not atomicaly synchronized.
*/
public void setUndefinePreprocessorDefinitionsArray(org.apache.xmlbeans.XmlObject[] undefinePreprocessorDefinitionsArray)
{
check_orphaned();
arraySetterHelper(undefinePreprocessorDefinitionsArray, UNDEFINEPREPROCESSORDEFINITIONS$36);
}
/**
* Sets ith "UndefinePreprocessorDefinitions" element
*/
public void setUndefinePreprocessorDefinitionsArray(int i, org.apache.xmlbeans.XmlObject undefinePreprocessorDefinitions)
{
generatedSetterHelperImpl(undefinePreprocessorDefinitions, UNDEFINEPREPROCESSORDEFINITIONS$36, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "UndefinePreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject insertNewUndefinePreprocessorDefinitions(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(UNDEFINEPREPROCESSORDEFINITIONS$36, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "UndefinePreprocessorDefinitions" element
*/
public org.apache.xmlbeans.XmlObject addNewUndefinePreprocessorDefinitions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(UNDEFINEPREPROCESSORDEFINITIONS$36);
return target;
}
}
/**
* Removes the ith "UndefinePreprocessorDefinitions" element
*/
public void removeUndefinePreprocessorDefinitions(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(UNDEFINEPREPROCESSORDEFINITIONS$36, i);
}
}
/**
* Gets a List of "StringPooling" elements
*/
public java.util.List getStringPoolingList()
{
final class StringPoolingList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getStringPoolingArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getStringPoolingArray(i);
ClCompileImpl.this.setStringPoolingArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewStringPooling(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getStringPoolingArray(i);
ClCompileImpl.this.removeStringPooling(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfStringPoolingArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new StringPoolingList();
}
}
/**
* Gets array of all "StringPooling" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getStringPoolingArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(STRINGPOOLING$38, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "StringPooling" element
*/
public org.apache.xmlbeans.XmlObject getStringPoolingArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(STRINGPOOLING$38, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "StringPooling" element
*/
public int sizeOfStringPoolingArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(STRINGPOOLING$38);
}
}
/**
* Sets array of all "StringPooling" element WARNING: This method is not atomicaly synchronized.
*/
public void setStringPoolingArray(org.apache.xmlbeans.XmlObject[] stringPoolingArray)
{
check_orphaned();
arraySetterHelper(stringPoolingArray, STRINGPOOLING$38);
}
/**
* Sets ith "StringPooling" element
*/
public void setStringPoolingArray(int i, org.apache.xmlbeans.XmlObject stringPooling)
{
generatedSetterHelperImpl(stringPooling, STRINGPOOLING$38, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "StringPooling" element
*/
public org.apache.xmlbeans.XmlObject insertNewStringPooling(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(STRINGPOOLING$38, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "StringPooling" element
*/
public org.apache.xmlbeans.XmlObject addNewStringPooling()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(STRINGPOOLING$38);
return target;
}
}
/**
* Removes the ith "StringPooling" element
*/
public void removeStringPooling(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(STRINGPOOLING$38, i);
}
}
/**
* Gets a List of "BrowseInformation" elements
*/
public java.util.List getBrowseInformationList()
{
final class BrowseInformationList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getBrowseInformationArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getBrowseInformationArray(i);
ClCompileImpl.this.setBrowseInformationArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewBrowseInformation(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getBrowseInformationArray(i);
ClCompileImpl.this.removeBrowseInformation(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfBrowseInformationArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new BrowseInformationList();
}
}
/**
* Gets array of all "BrowseInformation" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getBrowseInformationArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(BROWSEINFORMATION$40, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "BrowseInformation" element
*/
public org.apache.xmlbeans.XmlObject getBrowseInformationArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(BROWSEINFORMATION$40, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "BrowseInformation" element
*/
public int sizeOfBrowseInformationArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(BROWSEINFORMATION$40);
}
}
/**
* Sets array of all "BrowseInformation" element WARNING: This method is not atomicaly synchronized.
*/
public void setBrowseInformationArray(org.apache.xmlbeans.XmlObject[] browseInformationArray)
{
check_orphaned();
arraySetterHelper(browseInformationArray, BROWSEINFORMATION$40);
}
/**
* Sets ith "BrowseInformation" element
*/
public void setBrowseInformationArray(int i, org.apache.xmlbeans.XmlObject browseInformation)
{
generatedSetterHelperImpl(browseInformation, BROWSEINFORMATION$40, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "BrowseInformation" element
*/
public org.apache.xmlbeans.XmlObject insertNewBrowseInformation(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(BROWSEINFORMATION$40, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "BrowseInformation" element
*/
public org.apache.xmlbeans.XmlObject addNewBrowseInformation()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(BROWSEINFORMATION$40);
return target;
}
}
/**
* Removes the ith "BrowseInformation" element
*/
public void removeBrowseInformation(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(BROWSEINFORMATION$40, i);
}
}
/**
* Gets a List of "FloatingPointExceptions" elements
*/
public java.util.List getFloatingPointExceptionsList()
{
final class FloatingPointExceptionsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getFloatingPointExceptionsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFloatingPointExceptionsArray(i);
ClCompileImpl.this.setFloatingPointExceptionsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewFloatingPointExceptions(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getFloatingPointExceptionsArray(i);
ClCompileImpl.this.removeFloatingPointExceptions(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfFloatingPointExceptionsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new FloatingPointExceptionsList();
}
}
/**
* Gets array of all "FloatingPointExceptions" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getFloatingPointExceptionsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(FLOATINGPOINTEXCEPTIONS$42, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "FloatingPointExceptions" element
*/
public org.apache.xmlbeans.XmlObject getFloatingPointExceptionsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(FLOATINGPOINTEXCEPTIONS$42, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "FloatingPointExceptions" element
*/
public int sizeOfFloatingPointExceptionsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(FLOATINGPOINTEXCEPTIONS$42);
}
}
/**
* Sets array of all "FloatingPointExceptions" element WARNING: This method is not atomicaly synchronized.
*/
public void setFloatingPointExceptionsArray(org.apache.xmlbeans.XmlObject[] floatingPointExceptionsArray)
{
check_orphaned();
arraySetterHelper(floatingPointExceptionsArray, FLOATINGPOINTEXCEPTIONS$42);
}
/**
* Sets ith "FloatingPointExceptions" element
*/
public void setFloatingPointExceptionsArray(int i, org.apache.xmlbeans.XmlObject floatingPointExceptions)
{
generatedSetterHelperImpl(floatingPointExceptions, FLOATINGPOINTEXCEPTIONS$42, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "FloatingPointExceptions" element
*/
public org.apache.xmlbeans.XmlObject insertNewFloatingPointExceptions(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(FLOATINGPOINTEXCEPTIONS$42, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "FloatingPointExceptions" element
*/
public org.apache.xmlbeans.XmlObject addNewFloatingPointExceptions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(FLOATINGPOINTEXCEPTIONS$42);
return target;
}
}
/**
* Removes the ith "FloatingPointExceptions" element
*/
public void removeFloatingPointExceptions(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(FLOATINGPOINTEXCEPTIONS$42, i);
}
}
/**
* Gets a List of "CreateHotpatchableImage" elements
*/
public java.util.List getCreateHotpatchableImageList()
{
final class CreateHotpatchableImageList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getCreateHotpatchableImageArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getCreateHotpatchableImageArray(i);
ClCompileImpl.this.setCreateHotpatchableImageArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewCreateHotpatchableImage(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getCreateHotpatchableImageArray(i);
ClCompileImpl.this.removeCreateHotpatchableImage(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfCreateHotpatchableImageArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new CreateHotpatchableImageList();
}
}
/**
* Gets array of all "CreateHotpatchableImage" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getCreateHotpatchableImageArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(CREATEHOTPATCHABLEIMAGE$44, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "CreateHotpatchableImage" element
*/
public org.apache.xmlbeans.XmlObject getCreateHotpatchableImageArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(CREATEHOTPATCHABLEIMAGE$44, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "CreateHotpatchableImage" element
*/
public int sizeOfCreateHotpatchableImageArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(CREATEHOTPATCHABLEIMAGE$44);
}
}
/**
* Sets array of all "CreateHotpatchableImage" element WARNING: This method is not atomicaly synchronized.
*/
public void setCreateHotpatchableImageArray(org.apache.xmlbeans.XmlObject[] createHotpatchableImageArray)
{
check_orphaned();
arraySetterHelper(createHotpatchableImageArray, CREATEHOTPATCHABLEIMAGE$44);
}
/**
* Sets ith "CreateHotpatchableImage" element
*/
public void setCreateHotpatchableImageArray(int i, org.apache.xmlbeans.XmlObject createHotpatchableImage)
{
generatedSetterHelperImpl(createHotpatchableImage, CREATEHOTPATCHABLEIMAGE$44, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "CreateHotpatchableImage" element
*/
public org.apache.xmlbeans.XmlObject insertNewCreateHotpatchableImage(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(CREATEHOTPATCHABLEIMAGE$44, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "CreateHotpatchableImage" element
*/
public org.apache.xmlbeans.XmlObject addNewCreateHotpatchableImage()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(CREATEHOTPATCHABLEIMAGE$44);
return target;
}
}
/**
* Removes the ith "CreateHotpatchableImage" element
*/
public void removeCreateHotpatchableImage(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(CREATEHOTPATCHABLEIMAGE$44, i);
}
}
/**
* Gets a List of "RuntimeTypeInfo" elements
*/
public java.util.List getRuntimeTypeInfoList()
{
final class RuntimeTypeInfoList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getRuntimeTypeInfoArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getRuntimeTypeInfoArray(i);
ClCompileImpl.this.setRuntimeTypeInfoArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewRuntimeTypeInfo(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getRuntimeTypeInfoArray(i);
ClCompileImpl.this.removeRuntimeTypeInfo(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfRuntimeTypeInfoArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new RuntimeTypeInfoList();
}
}
/**
* Gets array of all "RuntimeTypeInfo" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getRuntimeTypeInfoArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(RUNTIMETYPEINFO$46, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "RuntimeTypeInfo" element
*/
public org.apache.xmlbeans.XmlObject getRuntimeTypeInfoArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(RUNTIMETYPEINFO$46, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "RuntimeTypeInfo" element
*/
public int sizeOfRuntimeTypeInfoArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(RUNTIMETYPEINFO$46);
}
}
/**
* Sets array of all "RuntimeTypeInfo" element WARNING: This method is not atomicaly synchronized.
*/
public void setRuntimeTypeInfoArray(org.apache.xmlbeans.XmlObject[] runtimeTypeInfoArray)
{
check_orphaned();
arraySetterHelper(runtimeTypeInfoArray, RUNTIMETYPEINFO$46);
}
/**
* Sets ith "RuntimeTypeInfo" element
*/
public void setRuntimeTypeInfoArray(int i, org.apache.xmlbeans.XmlObject runtimeTypeInfo)
{
generatedSetterHelperImpl(runtimeTypeInfo, RUNTIMETYPEINFO$46, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "RuntimeTypeInfo" element
*/
public org.apache.xmlbeans.XmlObject insertNewRuntimeTypeInfo(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(RUNTIMETYPEINFO$46, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "RuntimeTypeInfo" element
*/
public org.apache.xmlbeans.XmlObject addNewRuntimeTypeInfo()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(RUNTIMETYPEINFO$46);
return target;
}
}
/**
* Removes the ith "RuntimeTypeInfo" element
*/
public void removeRuntimeTypeInfo(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(RUNTIMETYPEINFO$46, i);
}
}
/**
* Gets a List of "OpenMPSupport" elements
*/
public java.util.List getOpenMPSupportList()
{
final class OpenMPSupportList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getOpenMPSupportArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOpenMPSupportArray(i);
ClCompileImpl.this.setOpenMPSupportArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewOpenMPSupport(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOpenMPSupportArray(i);
ClCompileImpl.this.removeOpenMPSupport(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfOpenMPSupportArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new OpenMPSupportList();
}
}
/**
* Gets array of all "OpenMPSupport" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getOpenMPSupportArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(OPENMPSUPPORT$48, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "OpenMPSupport" element
*/
public org.apache.xmlbeans.XmlObject getOpenMPSupportArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(OPENMPSUPPORT$48, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "OpenMPSupport" element
*/
public int sizeOfOpenMPSupportArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(OPENMPSUPPORT$48);
}
}
/**
* Sets array of all "OpenMPSupport" element WARNING: This method is not atomicaly synchronized.
*/
public void setOpenMPSupportArray(org.apache.xmlbeans.XmlObject[] openMPSupportArray)
{
check_orphaned();
arraySetterHelper(openMPSupportArray, OPENMPSUPPORT$48);
}
/**
* Sets ith "OpenMPSupport" element
*/
public void setOpenMPSupportArray(int i, org.apache.xmlbeans.XmlObject openMPSupport)
{
generatedSetterHelperImpl(openMPSupport, OPENMPSUPPORT$48, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "OpenMPSupport" element
*/
public org.apache.xmlbeans.XmlObject insertNewOpenMPSupport(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(OPENMPSUPPORT$48, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "OpenMPSupport" element
*/
public org.apache.xmlbeans.XmlObject addNewOpenMPSupport()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(OPENMPSUPPORT$48);
return target;
}
}
/**
* Removes the ith "OpenMPSupport" element
*/
public void removeOpenMPSupport(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(OPENMPSUPPORT$48, i);
}
}
/**
* Gets a List of "CallingConvention" elements
*/
public java.util.List getCallingConventionList()
{
final class CallingConventionList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getCallingConventionArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getCallingConventionArray(i);
ClCompileImpl.this.setCallingConventionArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewCallingConvention(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getCallingConventionArray(i);
ClCompileImpl.this.removeCallingConvention(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfCallingConventionArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new CallingConventionList();
}
}
/**
* Gets array of all "CallingConvention" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getCallingConventionArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(CALLINGCONVENTION$50, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "CallingConvention" element
*/
public org.apache.xmlbeans.XmlObject getCallingConventionArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(CALLINGCONVENTION$50, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "CallingConvention" element
*/
public int sizeOfCallingConventionArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(CALLINGCONVENTION$50);
}
}
/**
* Sets array of all "CallingConvention" element WARNING: This method is not atomicaly synchronized.
*/
public void setCallingConventionArray(org.apache.xmlbeans.XmlObject[] callingConventionArray)
{
check_orphaned();
arraySetterHelper(callingConventionArray, CALLINGCONVENTION$50);
}
/**
* Sets ith "CallingConvention" element
*/
public void setCallingConventionArray(int i, org.apache.xmlbeans.XmlObject callingConvention)
{
generatedSetterHelperImpl(callingConvention, CALLINGCONVENTION$50, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "CallingConvention" element
*/
public org.apache.xmlbeans.XmlObject insertNewCallingConvention(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(CALLINGCONVENTION$50, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "CallingConvention" element
*/
public org.apache.xmlbeans.XmlObject addNewCallingConvention()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(CALLINGCONVENTION$50);
return target;
}
}
/**
* Removes the ith "CallingConvention" element
*/
public void removeCallingConvention(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(CALLINGCONVENTION$50, i);
}
}
/**
* Gets a List of "DisableSpecificWarnings" elements
*/
public java.util.List getDisableSpecificWarningsList()
{
final class DisableSpecificWarningsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getDisableSpecificWarningsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getDisableSpecificWarningsArray(i);
ClCompileImpl.this.setDisableSpecificWarningsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewDisableSpecificWarnings(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getDisableSpecificWarningsArray(i);
ClCompileImpl.this.removeDisableSpecificWarnings(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfDisableSpecificWarningsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new DisableSpecificWarningsList();
}
}
/**
* Gets array of all "DisableSpecificWarnings" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getDisableSpecificWarningsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DISABLESPECIFICWARNINGS$52, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "DisableSpecificWarnings" element
*/
public org.apache.xmlbeans.XmlObject getDisableSpecificWarningsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(DISABLESPECIFICWARNINGS$52, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "DisableSpecificWarnings" element
*/
public int sizeOfDisableSpecificWarningsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DISABLESPECIFICWARNINGS$52);
}
}
/**
* Sets array of all "DisableSpecificWarnings" element WARNING: This method is not atomicaly synchronized.
*/
public void setDisableSpecificWarningsArray(org.apache.xmlbeans.XmlObject[] disableSpecificWarningsArray)
{
check_orphaned();
arraySetterHelper(disableSpecificWarningsArray, DISABLESPECIFICWARNINGS$52);
}
/**
* Sets ith "DisableSpecificWarnings" element
*/
public void setDisableSpecificWarningsArray(int i, org.apache.xmlbeans.XmlObject disableSpecificWarnings)
{
generatedSetterHelperImpl(disableSpecificWarnings, DISABLESPECIFICWARNINGS$52, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "DisableSpecificWarnings" element
*/
public org.apache.xmlbeans.XmlObject insertNewDisableSpecificWarnings(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(DISABLESPECIFICWARNINGS$52, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "DisableSpecificWarnings" element
*/
public org.apache.xmlbeans.XmlObject addNewDisableSpecificWarnings()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(DISABLESPECIFICWARNINGS$52);
return target;
}
}
/**
* Removes the ith "DisableSpecificWarnings" element
*/
public void removeDisableSpecificWarnings(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DISABLESPECIFICWARNINGS$52, i);
}
}
/**
* Gets a List of "ForcedIncludeFiles" elements
*/
public java.util.List getForcedIncludeFilesList()
{
final class ForcedIncludeFilesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getForcedIncludeFilesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getForcedIncludeFilesArray(i);
ClCompileImpl.this.setForcedIncludeFilesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewForcedIncludeFiles(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getForcedIncludeFilesArray(i);
ClCompileImpl.this.removeForcedIncludeFiles(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfForcedIncludeFilesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new ForcedIncludeFilesList();
}
}
/**
* Gets array of all "ForcedIncludeFiles" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getForcedIncludeFilesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(FORCEDINCLUDEFILES$54, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "ForcedIncludeFiles" element
*/
public org.apache.xmlbeans.XmlObject getForcedIncludeFilesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(FORCEDINCLUDEFILES$54, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "ForcedIncludeFiles" element
*/
public int sizeOfForcedIncludeFilesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(FORCEDINCLUDEFILES$54);
}
}
/**
* Sets array of all "ForcedIncludeFiles" element WARNING: This method is not atomicaly synchronized.
*/
public void setForcedIncludeFilesArray(org.apache.xmlbeans.XmlObject[] forcedIncludeFilesArray)
{
check_orphaned();
arraySetterHelper(forcedIncludeFilesArray, FORCEDINCLUDEFILES$54);
}
/**
* Sets ith "ForcedIncludeFiles" element
*/
public void setForcedIncludeFilesArray(int i, org.apache.xmlbeans.XmlObject forcedIncludeFiles)
{
generatedSetterHelperImpl(forcedIncludeFiles, FORCEDINCLUDEFILES$54, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "ForcedIncludeFiles" element
*/
public org.apache.xmlbeans.XmlObject insertNewForcedIncludeFiles(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(FORCEDINCLUDEFILES$54, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "ForcedIncludeFiles" element
*/
public org.apache.xmlbeans.XmlObject addNewForcedIncludeFiles()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(FORCEDINCLUDEFILES$54);
return target;
}
}
/**
* Removes the ith "ForcedIncludeFiles" element
*/
public void removeForcedIncludeFiles(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(FORCEDINCLUDEFILES$54, i);
}
}
/**
* Gets a List of "ForcedUsingFiles" elements
*/
public java.util.List getForcedUsingFilesList()
{
final class ForcedUsingFilesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getForcedUsingFilesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getForcedUsingFilesArray(i);
ClCompileImpl.this.setForcedUsingFilesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewForcedUsingFiles(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getForcedUsingFilesArray(i);
ClCompileImpl.this.removeForcedUsingFiles(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfForcedUsingFilesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new ForcedUsingFilesList();
}
}
/**
* Gets array of all "ForcedUsingFiles" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getForcedUsingFilesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(FORCEDUSINGFILES$56, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "ForcedUsingFiles" element
*/
public org.apache.xmlbeans.XmlObject getForcedUsingFilesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(FORCEDUSINGFILES$56, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "ForcedUsingFiles" element
*/
public int sizeOfForcedUsingFilesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(FORCEDUSINGFILES$56);
}
}
/**
* Sets array of all "ForcedUsingFiles" element WARNING: This method is not atomicaly synchronized.
*/
public void setForcedUsingFilesArray(org.apache.xmlbeans.XmlObject[] forcedUsingFilesArray)
{
check_orphaned();
arraySetterHelper(forcedUsingFilesArray, FORCEDUSINGFILES$56);
}
/**
* Sets ith "ForcedUsingFiles" element
*/
public void setForcedUsingFilesArray(int i, org.apache.xmlbeans.XmlObject forcedUsingFiles)
{
generatedSetterHelperImpl(forcedUsingFiles, FORCEDUSINGFILES$56, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "ForcedUsingFiles" element
*/
public org.apache.xmlbeans.XmlObject insertNewForcedUsingFiles(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(FORCEDUSINGFILES$56, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "ForcedUsingFiles" element
*/
public org.apache.xmlbeans.XmlObject addNewForcedUsingFiles()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(FORCEDUSINGFILES$56);
return target;
}
}
/**
* Removes the ith "ForcedUsingFiles" element
*/
public void removeForcedUsingFiles(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(FORCEDUSINGFILES$56, i);
}
}
/**
* Gets a List of "ShowIncludes" elements
*/
public java.util.List getShowIncludesList()
{
final class ShowIncludesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getShowIncludesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getShowIncludesArray(i);
ClCompileImpl.this.setShowIncludesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewShowIncludes(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getShowIncludesArray(i);
ClCompileImpl.this.removeShowIncludes(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfShowIncludesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new ShowIncludesList();
}
}
/**
* Gets array of all "ShowIncludes" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getShowIncludesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(SHOWINCLUDES$58, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "ShowIncludes" element
*/
public org.apache.xmlbeans.XmlObject getShowIncludesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(SHOWINCLUDES$58, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "ShowIncludes" element
*/
public int sizeOfShowIncludesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(SHOWINCLUDES$58);
}
}
/**
* Sets array of all "ShowIncludes" element WARNING: This method is not atomicaly synchronized.
*/
public void setShowIncludesArray(org.apache.xmlbeans.XmlObject[] showIncludesArray)
{
check_orphaned();
arraySetterHelper(showIncludesArray, SHOWINCLUDES$58);
}
/**
* Sets ith "ShowIncludes" element
*/
public void setShowIncludesArray(int i, org.apache.xmlbeans.XmlObject showIncludes)
{
generatedSetterHelperImpl(showIncludes, SHOWINCLUDES$58, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "ShowIncludes" element
*/
public org.apache.xmlbeans.XmlObject insertNewShowIncludes(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(SHOWINCLUDES$58, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "ShowIncludes" element
*/
public org.apache.xmlbeans.XmlObject addNewShowIncludes()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(SHOWINCLUDES$58);
return target;
}
}
/**
* Removes the ith "ShowIncludes" element
*/
public void removeShowIncludes(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(SHOWINCLUDES$58, i);
}
}
/**
* Gets a List of "UseFullPaths" elements
*/
public java.util.List getUseFullPathsList()
{
final class UseFullPathsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getUseFullPathsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUseFullPathsArray(i);
ClCompileImpl.this.setUseFullPathsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewUseFullPaths(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getUseFullPathsArray(i);
ClCompileImpl.this.removeUseFullPaths(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfUseFullPathsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new UseFullPathsList();
}
}
/**
* Gets array of all "UseFullPaths" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getUseFullPathsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(USEFULLPATHS$60, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "UseFullPaths" element
*/
public org.apache.xmlbeans.XmlObject getUseFullPathsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(USEFULLPATHS$60, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "UseFullPaths" element
*/
public int sizeOfUseFullPathsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(USEFULLPATHS$60);
}
}
/**
* Sets array of all "UseFullPaths" element WARNING: This method is not atomicaly synchronized.
*/
public void setUseFullPathsArray(org.apache.xmlbeans.XmlObject[] useFullPathsArray)
{
check_orphaned();
arraySetterHelper(useFullPathsArray, USEFULLPATHS$60);
}
/**
* Sets ith "UseFullPaths" element
*/
public void setUseFullPathsArray(int i, org.apache.xmlbeans.XmlObject useFullPaths)
{
generatedSetterHelperImpl(useFullPaths, USEFULLPATHS$60, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "UseFullPaths" element
*/
public org.apache.xmlbeans.XmlObject insertNewUseFullPaths(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(USEFULLPATHS$60, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "UseFullPaths" element
*/
public org.apache.xmlbeans.XmlObject addNewUseFullPaths()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(USEFULLPATHS$60);
return target;
}
}
/**
* Removes the ith "UseFullPaths" element
*/
public void removeUseFullPaths(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(USEFULLPATHS$60, i);
}
}
/**
* Gets a List of "OmitDefaultLibName" elements
*/
public java.util.List getOmitDefaultLibNameList()
{
final class OmitDefaultLibNameList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getOmitDefaultLibNameArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOmitDefaultLibNameArray(i);
ClCompileImpl.this.setOmitDefaultLibNameArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewOmitDefaultLibName(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getOmitDefaultLibNameArray(i);
ClCompileImpl.this.removeOmitDefaultLibName(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfOmitDefaultLibNameArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new OmitDefaultLibNameList();
}
}
/**
* Gets array of all "OmitDefaultLibName" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getOmitDefaultLibNameArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(OMITDEFAULTLIBNAME$62, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "OmitDefaultLibName" element
*/
public org.apache.xmlbeans.XmlObject getOmitDefaultLibNameArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(OMITDEFAULTLIBNAME$62, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "OmitDefaultLibName" element
*/
public int sizeOfOmitDefaultLibNameArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(OMITDEFAULTLIBNAME$62);
}
}
/**
* Sets array of all "OmitDefaultLibName" element WARNING: This method is not atomicaly synchronized.
*/
public void setOmitDefaultLibNameArray(org.apache.xmlbeans.XmlObject[] omitDefaultLibNameArray)
{
check_orphaned();
arraySetterHelper(omitDefaultLibNameArray, OMITDEFAULTLIBNAME$62);
}
/**
* Sets ith "OmitDefaultLibName" element
*/
public void setOmitDefaultLibNameArray(int i, org.apache.xmlbeans.XmlObject omitDefaultLibName)
{
generatedSetterHelperImpl(omitDefaultLibName, OMITDEFAULTLIBNAME$62, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "OmitDefaultLibName" element
*/
public org.apache.xmlbeans.XmlObject insertNewOmitDefaultLibName(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(OMITDEFAULTLIBNAME$62, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "OmitDefaultLibName" element
*/
public org.apache.xmlbeans.XmlObject addNewOmitDefaultLibName()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(OMITDEFAULTLIBNAME$62);
return target;
}
}
/**
* Removes the ith "OmitDefaultLibName" element
*/
public void removeOmitDefaultLibName(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(OMITDEFAULTLIBNAME$62, i);
}
}
/**
* Gets a List of "TreatSpecificWarningsAsErrors" elements
*/
public java.util.List getTreatSpecificWarningsAsErrorsList()
{
final class TreatSpecificWarningsAsErrorsList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClCompileImpl.this.getTreatSpecificWarningsAsErrorsArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getTreatSpecificWarningsAsErrorsArray(i);
ClCompileImpl.this.setTreatSpecificWarningsAsErrorsArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClCompileImpl.this.insertNewTreatSpecificWarningsAsErrors(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClCompileImpl.this.getTreatSpecificWarningsAsErrorsArray(i);
ClCompileImpl.this.removeTreatSpecificWarningsAsErrors(i);
return old;
}
@Override
public int size()
{ return ClCompileImpl.this.sizeOfTreatSpecificWarningsAsErrorsArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new TreatSpecificWarningsAsErrorsList();
}
}
/**
* Gets array of all "TreatSpecificWarningsAsErrors" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getTreatSpecificWarningsAsErrorsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(TREATSPECIFICWARNINGSASERRORS$64, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "TreatSpecificWarningsAsErrors" element
*/
public org.apache.xmlbeans.XmlObject getTreatSpecificWarningsAsErrorsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(TREATSPECIFICWARNINGSASERRORS$64, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "TreatSpecificWarningsAsErrors" element
*/
public int sizeOfTreatSpecificWarningsAsErrorsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(TREATSPECIFICWARNINGSASERRORS$64);
}
}
/**
* Sets array of all "TreatSpecificWarningsAsErrors" element WARNING: This method is not atomicaly synchronized.
*/
public void setTreatSpecificWarningsAsErrorsArray(org.apache.xmlbeans.XmlObject[] treatSpecificWarningsAsErrorsArray)
{
check_orphaned();
arraySetterHelper(treatSpecificWarningsAsErrorsArray, TREATSPECIFICWARNINGSASERRORS$64);
}
/**
* Sets ith "TreatSpecificWarningsAsErrors" element
*/
public void setTreatSpecificWarningsAsErrorsArray(int i, org.apache.xmlbeans.XmlObject treatSpecificWarningsAsErrors)
{
generatedSetterHelperImpl(treatSpecificWarningsAsErrors, TREATSPECIFICWARNINGSASERRORS$64, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "TreatSpecificWarningsAsErrors" element
*/
public org.apache.xmlbeans.XmlObject insertNewTreatSpecificWarningsAsErrors(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(TREATSPECIFICWARNINGSASERRORS$64, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "TreatSpecificWarningsAsErrors" element
*/
public org.apache.xmlbeans.XmlObject addNewTreatSpecificWarningsAsErrors()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(TREATSPECIFICWARNINGSASERRORS$64);
return target;
}
}
/**
* Removes the ith "TreatSpecificWarningsAsErrors" element
*/
public void removeTreatSpecificWarningsAsErrors(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(TREATSPECIFICWARNINGSASERRORS$64, i);
}
}
/**
* An XML PrecompiledHeader(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is an atomic type that is a restriction of io.github.isotes.vs.model.ClCompileDocument$ClCompile$PrecompiledHeader.
*/
public static class PrecompiledHeaderImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements io.github.isotes.vs.model.ClCompileDocument.ClCompile.PrecompiledHeader
{
private static final long serialVersionUID = 1L;
public PrecompiledHeaderImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, true);
}
protected PrecompiledHeaderImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
private static final javax.xml.namespace.QName CONDITION$0 =
new javax.xml.namespace.QName("", "Condition");
/**
* Gets the "Condition" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getCondition()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONDITION$0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Condition" attribute
*/
public boolean isSetCondition()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(CONDITION$0) != null;
}
}
/**
* Sets the "Condition" attribute
*/
public void setCondition(org.apache.xmlbeans.XmlAnySimpleType condition)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONDITION$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONDITION$0);
}
target.set(condition);
}
}
/**
* Appends and returns a new empty "Condition" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewCondition()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONDITION$0);
return target;
}
}
/**
* Unsets the "Condition" attribute
*/
public void unsetCondition()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(CONDITION$0);
}
}
}
/**
* An XML CompileAsManaged(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is an atomic type that is a restriction of io.github.isotes.vs.model.ClCompileDocument$ClCompile$CompileAsManaged.
*/
public static class CompileAsManagedImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements io.github.isotes.vs.model.ClCompileDocument.ClCompile.CompileAsManaged
{
private static final long serialVersionUID = 1L;
public CompileAsManagedImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, true);
}
protected CompileAsManagedImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
private static final javax.xml.namespace.QName CONDITION$0 =
new javax.xml.namespace.QName("", "Condition");
/**
* Gets the "Condition" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getCondition()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONDITION$0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Condition" attribute
*/
public boolean isSetCondition()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(CONDITION$0) != null;
}
}
/**
* Sets the "Condition" attribute
*/
public void setCondition(org.apache.xmlbeans.XmlAnySimpleType condition)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONDITION$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONDITION$0);
}
target.set(condition);
}
}
/**
* Appends and returns a new empty "Condition" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewCondition()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONDITION$0);
return target;
}
}
/**
* Unsets the "Condition" attribute
*/
public void unsetCondition()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(CONDITION$0);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy