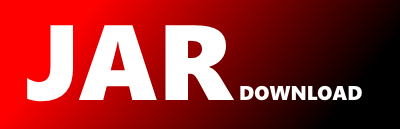
io.github.isotes.vs.model.impl.ClIncludeDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: ClInclude
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.ClIncludeDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model.impl;
/**
* A document containing one ClInclude(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public class ClIncludeDocumentImpl extends io.github.isotes.vs.model.impl.ItemDocumentImpl implements io.github.isotes.vs.model.ClIncludeDocument
{
private static final long serialVersionUID = 1L;
public ClIncludeDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName CLINCLUDE$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "ClInclude");
/**
* Gets the "ClInclude" element
*/
public io.github.isotes.vs.model.ClIncludeDocument.ClInclude getClInclude()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClIncludeDocument.ClInclude target = null;
target = (io.github.isotes.vs.model.ClIncludeDocument.ClInclude)get_store().find_element_user(CLINCLUDE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "ClInclude" element
*/
public void setClInclude(io.github.isotes.vs.model.ClIncludeDocument.ClInclude clInclude)
{
generatedSetterHelperImpl(clInclude, CLINCLUDE$0, 0, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_SINGLETON);
}
/**
* Appends and returns a new empty "ClInclude" element
*/
public io.github.isotes.vs.model.ClIncludeDocument.ClInclude addNewClInclude()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.ClIncludeDocument.ClInclude target = null;
target = (io.github.isotes.vs.model.ClIncludeDocument.ClInclude)get_store().add_element_user(CLINCLUDE$0);
return target;
}
}
/**
* An XML ClInclude(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public static class ClIncludeImpl extends io.github.isotes.vs.model.impl.SimpleItemTypeImpl implements io.github.isotes.vs.model.ClIncludeDocument.ClInclude
{
private static final long serialVersionUID = 1L;
public ClIncludeImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ADDITIONALINCLUDEDIRECTORIES$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "AdditionalIncludeDirectories");
/**
* Gets a List of "AdditionalIncludeDirectories" elements
*/
public java.util.List getAdditionalIncludeDirectoriesList()
{
final class AdditionalIncludeDirectoriesList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return ClIncludeImpl.this.getAdditionalIncludeDirectoriesArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = ClIncludeImpl.this.getAdditionalIncludeDirectoriesArray(i);
ClIncludeImpl.this.setAdditionalIncludeDirectoriesArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ ClIncludeImpl.this.insertNewAdditionalIncludeDirectories(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = ClIncludeImpl.this.getAdditionalIncludeDirectoriesArray(i);
ClIncludeImpl.this.removeAdditionalIncludeDirectories(i);
return old;
}
@Override
public int size()
{ return ClIncludeImpl.this.sizeOfAdditionalIncludeDirectoriesArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new AdditionalIncludeDirectoriesList();
}
}
/**
* Gets array of all "AdditionalIncludeDirectories" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getAdditionalIncludeDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ADDITIONALINCLUDEDIRECTORIES$0, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject getAdditionalIncludeDirectoriesArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(ADDITIONALINCLUDEDIRECTORIES$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "AdditionalIncludeDirectories" element
*/
public int sizeOfAdditionalIncludeDirectoriesArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ADDITIONALINCLUDEDIRECTORIES$0);
}
}
/**
* Sets array of all "AdditionalIncludeDirectories" element WARNING: This method is not atomicaly synchronized.
*/
public void setAdditionalIncludeDirectoriesArray(org.apache.xmlbeans.XmlObject[] additionalIncludeDirectoriesArray)
{
check_orphaned();
arraySetterHelper(additionalIncludeDirectoriesArray, ADDITIONALINCLUDEDIRECTORIES$0);
}
/**
* Sets ith "AdditionalIncludeDirectories" element
*/
public void setAdditionalIncludeDirectoriesArray(int i, org.apache.xmlbeans.XmlObject additionalIncludeDirectories)
{
generatedSetterHelperImpl(additionalIncludeDirectories, ADDITIONALINCLUDEDIRECTORIES$0, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject insertNewAdditionalIncludeDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(ADDITIONALINCLUDEDIRECTORIES$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "AdditionalIncludeDirectories" element
*/
public org.apache.xmlbeans.XmlObject addNewAdditionalIncludeDirectories()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(ADDITIONALINCLUDEDIRECTORIES$0);
return target;
}
}
/**
* Removes the ith "AdditionalIncludeDirectories" element
*/
public void removeAdditionalIncludeDirectories(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ADDITIONALINCLUDEDIRECTORIES$0, i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy