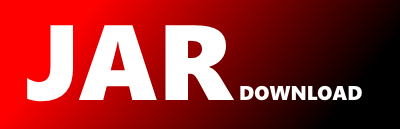
io.github.isotes.vs.model.impl.CompileDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: Compile
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.CompileDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model.impl;
/**
* A document containing one Compile(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public class CompileDocumentImpl extends io.github.isotes.vs.model.impl.ItemDocumentImpl implements io.github.isotes.vs.model.CompileDocument
{
private static final long serialVersionUID = 1L;
public CompileDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName COMPILE$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "Compile");
/**
* Gets the "Compile" element
*/
public io.github.isotes.vs.model.CompileDocument.Compile getCompile()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.CompileDocument.Compile target = null;
target = (io.github.isotes.vs.model.CompileDocument.Compile)get_store().find_element_user(COMPILE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "Compile" element
*/
public void setCompile(io.github.isotes.vs.model.CompileDocument.Compile compile)
{
generatedSetterHelperImpl(compile, COMPILE$0, 0, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_SINGLETON);
}
/**
* Appends and returns a new empty "Compile" element
*/
public io.github.isotes.vs.model.CompileDocument.Compile addNewCompile()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.CompileDocument.Compile target = null;
target = (io.github.isotes.vs.model.CompileDocument.Compile)get_store().add_element_user(COMPILE$0);
return target;
}
}
/**
* An XML Compile(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public static class CompileImpl extends io.github.isotes.vs.model.impl.SimpleItemTypeImpl implements io.github.isotes.vs.model.CompileDocument.Compile
{
private static final long serialVersionUID = 1L;
public CompileImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName SUBTYPE$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "SubType");
private static final javax.xml.namespace.QName DEPENDENTUPON$2 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "DependentUpon");
private static final javax.xml.namespace.QName AUTOGEN$4 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "AutoGen");
private static final javax.xml.namespace.QName DESIGNTIME$6 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "DesignTime");
private static final javax.xml.namespace.QName LINK$8 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "Link");
private static final javax.xml.namespace.QName DESIGNTIMESHAREDINPUT$10 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "DesignTimeSharedInput");
private static final javax.xml.namespace.QName VISIBLE$12 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "Visible");
private static final javax.xml.namespace.QName COPYTOOUTPUTDIRECTORY$14 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "CopyToOutputDirectory");
private static final javax.xml.namespace.QName VBMYEXTENSIONTEMPLATEID$16 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "VBMyExtensionTemplateID");
/**
* Gets a List of "SubType" elements
*/
public java.util.List getSubTypeList()
{
final class SubTypeList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getSubTypeArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getSubTypeArray(i);
CompileImpl.this.setSubTypeArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewSubType(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getSubTypeArray(i);
CompileImpl.this.removeSubType(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfSubTypeArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new SubTypeList();
}
}
/**
* Gets array of all "SubType" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getSubTypeArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(SUBTYPE$0, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "SubType" element
*/
public org.apache.xmlbeans.XmlObject getSubTypeArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(SUBTYPE$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "SubType" element
*/
public int sizeOfSubTypeArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(SUBTYPE$0);
}
}
/**
* Sets array of all "SubType" element WARNING: This method is not atomicaly synchronized.
*/
public void setSubTypeArray(org.apache.xmlbeans.XmlObject[] subTypeArray)
{
check_orphaned();
arraySetterHelper(subTypeArray, SUBTYPE$0);
}
/**
* Sets ith "SubType" element
*/
public void setSubTypeArray(int i, org.apache.xmlbeans.XmlObject subType)
{
generatedSetterHelperImpl(subType, SUBTYPE$0, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "SubType" element
*/
public org.apache.xmlbeans.XmlObject insertNewSubType(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(SUBTYPE$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "SubType" element
*/
public org.apache.xmlbeans.XmlObject addNewSubType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(SUBTYPE$0);
return target;
}
}
/**
* Removes the ith "SubType" element
*/
public void removeSubType(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(SUBTYPE$0, i);
}
}
/**
* Gets a List of "DependentUpon" elements
*/
public java.util.List getDependentUponList()
{
final class DependentUponList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getDependentUponArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDependentUponArray(i);
CompileImpl.this.setDependentUponArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewDependentUpon(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDependentUponArray(i);
CompileImpl.this.removeDependentUpon(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfDependentUponArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new DependentUponList();
}
}
/**
* Gets array of all "DependentUpon" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getDependentUponArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DEPENDENTUPON$2, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "DependentUpon" element
*/
public org.apache.xmlbeans.XmlObject getDependentUponArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(DEPENDENTUPON$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "DependentUpon" element
*/
public int sizeOfDependentUponArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DEPENDENTUPON$2);
}
}
/**
* Sets array of all "DependentUpon" element WARNING: This method is not atomicaly synchronized.
*/
public void setDependentUponArray(org.apache.xmlbeans.XmlObject[] dependentUponArray)
{
check_orphaned();
arraySetterHelper(dependentUponArray, DEPENDENTUPON$2);
}
/**
* Sets ith "DependentUpon" element
*/
public void setDependentUponArray(int i, org.apache.xmlbeans.XmlObject dependentUpon)
{
generatedSetterHelperImpl(dependentUpon, DEPENDENTUPON$2, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "DependentUpon" element
*/
public org.apache.xmlbeans.XmlObject insertNewDependentUpon(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(DEPENDENTUPON$2, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "DependentUpon" element
*/
public org.apache.xmlbeans.XmlObject addNewDependentUpon()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(DEPENDENTUPON$2);
return target;
}
}
/**
* Removes the ith "DependentUpon" element
*/
public void removeDependentUpon(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DEPENDENTUPON$2, i);
}
}
/**
* Gets a List of "AutoGen" elements
*/
public java.util.List getAutoGenList()
{
final class AutoGenList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getAutoGenArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getAutoGenArray(i);
CompileImpl.this.setAutoGenArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewAutoGen(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getAutoGenArray(i);
CompileImpl.this.removeAutoGen(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfAutoGenArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new AutoGenList();
}
}
/**
* Gets array of all "AutoGen" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getAutoGenArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(AUTOGEN$4, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "AutoGen" element
*/
public org.apache.xmlbeans.XmlObject getAutoGenArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(AUTOGEN$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "AutoGen" element
*/
public int sizeOfAutoGenArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(AUTOGEN$4);
}
}
/**
* Sets array of all "AutoGen" element WARNING: This method is not atomicaly synchronized.
*/
public void setAutoGenArray(org.apache.xmlbeans.XmlObject[] autoGenArray)
{
check_orphaned();
arraySetterHelper(autoGenArray, AUTOGEN$4);
}
/**
* Sets ith "AutoGen" element
*/
public void setAutoGenArray(int i, org.apache.xmlbeans.XmlObject autoGen)
{
generatedSetterHelperImpl(autoGen, AUTOGEN$4, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "AutoGen" element
*/
public org.apache.xmlbeans.XmlObject insertNewAutoGen(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(AUTOGEN$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "AutoGen" element
*/
public org.apache.xmlbeans.XmlObject addNewAutoGen()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(AUTOGEN$4);
return target;
}
}
/**
* Removes the ith "AutoGen" element
*/
public void removeAutoGen(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(AUTOGEN$4, i);
}
}
/**
* Gets a List of "DesignTime" elements
*/
public java.util.List getDesignTimeList()
{
final class DesignTimeList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getDesignTimeArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDesignTimeArray(i);
CompileImpl.this.setDesignTimeArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewDesignTime(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDesignTimeArray(i);
CompileImpl.this.removeDesignTime(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfDesignTimeArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new DesignTimeList();
}
}
/**
* Gets array of all "DesignTime" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getDesignTimeArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DESIGNTIME$6, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "DesignTime" element
*/
public org.apache.xmlbeans.XmlObject getDesignTimeArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(DESIGNTIME$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "DesignTime" element
*/
public int sizeOfDesignTimeArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DESIGNTIME$6);
}
}
/**
* Sets array of all "DesignTime" element WARNING: This method is not atomicaly synchronized.
*/
public void setDesignTimeArray(org.apache.xmlbeans.XmlObject[] designTimeArray)
{
check_orphaned();
arraySetterHelper(designTimeArray, DESIGNTIME$6);
}
/**
* Sets ith "DesignTime" element
*/
public void setDesignTimeArray(int i, org.apache.xmlbeans.XmlObject designTime)
{
generatedSetterHelperImpl(designTime, DESIGNTIME$6, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "DesignTime" element
*/
public org.apache.xmlbeans.XmlObject insertNewDesignTime(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(DESIGNTIME$6, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "DesignTime" element
*/
public org.apache.xmlbeans.XmlObject addNewDesignTime()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(DESIGNTIME$6);
return target;
}
}
/**
* Removes the ith "DesignTime" element
*/
public void removeDesignTime(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DESIGNTIME$6, i);
}
}
/**
* Gets a List of "Link" elements
*/
public java.util.List getLinkList()
{
final class LinkList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getLinkArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getLinkArray(i);
CompileImpl.this.setLinkArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewLink(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getLinkArray(i);
CompileImpl.this.removeLink(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfLinkArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new LinkList();
}
}
/**
* Gets array of all "Link" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getLinkArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(LINK$8, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "Link" element
*/
public org.apache.xmlbeans.XmlObject getLinkArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(LINK$8, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "Link" element
*/
public int sizeOfLinkArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(LINK$8);
}
}
/**
* Sets array of all "Link" element WARNING: This method is not atomicaly synchronized.
*/
public void setLinkArray(org.apache.xmlbeans.XmlObject[] linkArray)
{
check_orphaned();
arraySetterHelper(linkArray, LINK$8);
}
/**
* Sets ith "Link" element
*/
public void setLinkArray(int i, org.apache.xmlbeans.XmlObject link)
{
generatedSetterHelperImpl(link, LINK$8, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "Link" element
*/
public org.apache.xmlbeans.XmlObject insertNewLink(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(LINK$8, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "Link" element
*/
public org.apache.xmlbeans.XmlObject addNewLink()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(LINK$8);
return target;
}
}
/**
* Removes the ith "Link" element
*/
public void removeLink(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(LINK$8, i);
}
}
/**
* Gets a List of "DesignTimeSharedInput" elements
*/
public java.util.List getDesignTimeSharedInputList()
{
final class DesignTimeSharedInputList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getDesignTimeSharedInputArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDesignTimeSharedInputArray(i);
CompileImpl.this.setDesignTimeSharedInputArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewDesignTimeSharedInput(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getDesignTimeSharedInputArray(i);
CompileImpl.this.removeDesignTimeSharedInput(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfDesignTimeSharedInputArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new DesignTimeSharedInputList();
}
}
/**
* Gets array of all "DesignTimeSharedInput" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getDesignTimeSharedInputArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(DESIGNTIMESHAREDINPUT$10, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "DesignTimeSharedInput" element
*/
public org.apache.xmlbeans.XmlObject getDesignTimeSharedInputArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(DESIGNTIMESHAREDINPUT$10, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "DesignTimeSharedInput" element
*/
public int sizeOfDesignTimeSharedInputArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DESIGNTIMESHAREDINPUT$10);
}
}
/**
* Sets array of all "DesignTimeSharedInput" element WARNING: This method is not atomicaly synchronized.
*/
public void setDesignTimeSharedInputArray(org.apache.xmlbeans.XmlObject[] designTimeSharedInputArray)
{
check_orphaned();
arraySetterHelper(designTimeSharedInputArray, DESIGNTIMESHAREDINPUT$10);
}
/**
* Sets ith "DesignTimeSharedInput" element
*/
public void setDesignTimeSharedInputArray(int i, org.apache.xmlbeans.XmlObject designTimeSharedInput)
{
generatedSetterHelperImpl(designTimeSharedInput, DESIGNTIMESHAREDINPUT$10, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "DesignTimeSharedInput" element
*/
public org.apache.xmlbeans.XmlObject insertNewDesignTimeSharedInput(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(DESIGNTIMESHAREDINPUT$10, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "DesignTimeSharedInput" element
*/
public org.apache.xmlbeans.XmlObject addNewDesignTimeSharedInput()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(DESIGNTIMESHAREDINPUT$10);
return target;
}
}
/**
* Removes the ith "DesignTimeSharedInput" element
*/
public void removeDesignTimeSharedInput(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DESIGNTIMESHAREDINPUT$10, i);
}
}
/**
* Gets a List of "Visible" elements
*/
public java.util.List getVisibleList()
{
final class VisibleList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getVisibleArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getVisibleArray(i);
CompileImpl.this.setVisibleArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewVisible(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getVisibleArray(i);
CompileImpl.this.removeVisible(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfVisibleArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new VisibleList();
}
}
/**
* Gets array of all "Visible" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getVisibleArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VISIBLE$12, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "Visible" element
*/
public org.apache.xmlbeans.XmlObject getVisibleArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(VISIBLE$12, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "Visible" element
*/
public int sizeOfVisibleArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(VISIBLE$12);
}
}
/**
* Sets array of all "Visible" element WARNING: This method is not atomicaly synchronized.
*/
public void setVisibleArray(org.apache.xmlbeans.XmlObject[] visibleArray)
{
check_orphaned();
arraySetterHelper(visibleArray, VISIBLE$12);
}
/**
* Sets ith "Visible" element
*/
public void setVisibleArray(int i, org.apache.xmlbeans.XmlObject visible)
{
generatedSetterHelperImpl(visible, VISIBLE$12, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "Visible" element
*/
public org.apache.xmlbeans.XmlObject insertNewVisible(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(VISIBLE$12, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "Visible" element
*/
public org.apache.xmlbeans.XmlObject addNewVisible()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(VISIBLE$12);
return target;
}
}
/**
* Removes the ith "Visible" element
*/
public void removeVisible(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(VISIBLE$12, i);
}
}
/**
* Gets a List of "CopyToOutputDirectory" elements
*/
public java.util.List getCopyToOutputDirectoryList()
{
final class CopyToOutputDirectoryList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getCopyToOutputDirectoryArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getCopyToOutputDirectoryArray(i);
CompileImpl.this.setCopyToOutputDirectoryArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewCopyToOutputDirectory(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getCopyToOutputDirectoryArray(i);
CompileImpl.this.removeCopyToOutputDirectory(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfCopyToOutputDirectoryArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new CopyToOutputDirectoryList();
}
}
/**
* Gets array of all "CopyToOutputDirectory" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getCopyToOutputDirectoryArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(COPYTOOUTPUTDIRECTORY$14, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "CopyToOutputDirectory" element
*/
public org.apache.xmlbeans.XmlObject getCopyToOutputDirectoryArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(COPYTOOUTPUTDIRECTORY$14, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "CopyToOutputDirectory" element
*/
public int sizeOfCopyToOutputDirectoryArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(COPYTOOUTPUTDIRECTORY$14);
}
}
/**
* Sets array of all "CopyToOutputDirectory" element WARNING: This method is not atomicaly synchronized.
*/
public void setCopyToOutputDirectoryArray(org.apache.xmlbeans.XmlObject[] copyToOutputDirectoryArray)
{
check_orphaned();
arraySetterHelper(copyToOutputDirectoryArray, COPYTOOUTPUTDIRECTORY$14);
}
/**
* Sets ith "CopyToOutputDirectory" element
*/
public void setCopyToOutputDirectoryArray(int i, org.apache.xmlbeans.XmlObject copyToOutputDirectory)
{
generatedSetterHelperImpl(copyToOutputDirectory, COPYTOOUTPUTDIRECTORY$14, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "CopyToOutputDirectory" element
*/
public org.apache.xmlbeans.XmlObject insertNewCopyToOutputDirectory(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(COPYTOOUTPUTDIRECTORY$14, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "CopyToOutputDirectory" element
*/
public org.apache.xmlbeans.XmlObject addNewCopyToOutputDirectory()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(COPYTOOUTPUTDIRECTORY$14);
return target;
}
}
/**
* Removes the ith "CopyToOutputDirectory" element
*/
public void removeCopyToOutputDirectory(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(COPYTOOUTPUTDIRECTORY$14, i);
}
}
/**
* Gets a List of "VBMyExtensionTemplateID" elements
*/
public java.util.List getVBMyExtensionTemplateIDList()
{
final class VBMyExtensionTemplateIDList extends java.util.AbstractList
{
@Override
public org.apache.xmlbeans.XmlObject get(int i)
{ return CompileImpl.this.getVBMyExtensionTemplateIDArray(i); }
@Override
public org.apache.xmlbeans.XmlObject set(int i, org.apache.xmlbeans.XmlObject o)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getVBMyExtensionTemplateIDArray(i);
CompileImpl.this.setVBMyExtensionTemplateIDArray(i, o);
return old;
}
@Override
public void add(int i, org.apache.xmlbeans.XmlObject o)
{ CompileImpl.this.insertNewVBMyExtensionTemplateID(i).set(o); }
@Override
public org.apache.xmlbeans.XmlObject remove(int i)
{
org.apache.xmlbeans.XmlObject old = CompileImpl.this.getVBMyExtensionTemplateIDArray(i);
CompileImpl.this.removeVBMyExtensionTemplateID(i);
return old;
}
@Override
public int size()
{ return CompileImpl.this.sizeOfVBMyExtensionTemplateIDArray(); }
}
synchronized (monitor())
{
check_orphaned();
return new VBMyExtensionTemplateIDList();
}
}
/**
* Gets array of all "VBMyExtensionTemplateID" elements
* @deprecated
*/
@Deprecated
public org.apache.xmlbeans.XmlObject[] getVBMyExtensionTemplateIDArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VBMYEXTENSIONTEMPLATEID$16, targetList);
org.apache.xmlbeans.XmlObject[] result = new org.apache.xmlbeans.XmlObject[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "VBMyExtensionTemplateID" element
*/
public org.apache.xmlbeans.XmlObject getVBMyExtensionTemplateIDArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().find_element_user(VBMYEXTENSIONTEMPLATEID$16, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "VBMyExtensionTemplateID" element
*/
public int sizeOfVBMyExtensionTemplateIDArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(VBMYEXTENSIONTEMPLATEID$16);
}
}
/**
* Sets array of all "VBMyExtensionTemplateID" element WARNING: This method is not atomicaly synchronized.
*/
public void setVBMyExtensionTemplateIDArray(org.apache.xmlbeans.XmlObject[] vbMyExtensionTemplateIDArray)
{
check_orphaned();
arraySetterHelper(vbMyExtensionTemplateIDArray, VBMYEXTENSIONTEMPLATEID$16);
}
/**
* Sets ith "VBMyExtensionTemplateID" element
*/
public void setVBMyExtensionTemplateIDArray(int i, org.apache.xmlbeans.XmlObject vbMyExtensionTemplateID)
{
generatedSetterHelperImpl(vbMyExtensionTemplateID, VBMYEXTENSIONTEMPLATEID$16, i, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_ARRAYITEM);
}
/**
* Inserts and returns a new empty value (as xml) as the ith "VBMyExtensionTemplateID" element
*/
public org.apache.xmlbeans.XmlObject insertNewVBMyExtensionTemplateID(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().insert_element_user(VBMYEXTENSIONTEMPLATEID$16, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "VBMyExtensionTemplateID" element
*/
public org.apache.xmlbeans.XmlObject addNewVBMyExtensionTemplateID()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlObject target = null;
target = (org.apache.xmlbeans.XmlObject)get_store().add_element_user(VBMYEXTENSIONTEMPLATEID$16);
return target;
}
}
/**
* Removes the ith "VBMyExtensionTemplateID" element
*/
public void removeVBMyExtensionTemplateID(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(VBMYEXTENSIONTEMPLATEID$16, i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy