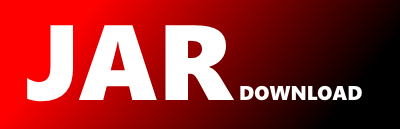
io.github.isotes.vs.model.impl.MSBuildDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: MSBuild
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.MSBuildDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model.impl;
/**
* A document containing one MSBuild(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public class MSBuildDocumentImpl extends io.github.isotes.vs.model.impl.TaskDocumentImpl implements io.github.isotes.vs.model.MSBuildDocument
{
private static final long serialVersionUID = 1L;
public MSBuildDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName MSBUILD$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "MSBuild");
/**
* Gets the "MSBuild" element
*/
public io.github.isotes.vs.model.MSBuildDocument.MSBuild getMSBuild()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.MSBuildDocument.MSBuild target = null;
target = (io.github.isotes.vs.model.MSBuildDocument.MSBuild)get_store().find_element_user(MSBUILD$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "MSBuild" element
*/
public void setMSBuild(io.github.isotes.vs.model.MSBuildDocument.MSBuild msBuild)
{
generatedSetterHelperImpl(msBuild, MSBUILD$0, 0, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_SINGLETON);
}
/**
* Appends and returns a new empty "MSBuild" element
*/
public io.github.isotes.vs.model.MSBuildDocument.MSBuild addNewMSBuild()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.MSBuildDocument.MSBuild target = null;
target = (io.github.isotes.vs.model.MSBuildDocument.MSBuild)get_store().add_element_user(MSBUILD$0);
return target;
}
}
/**
* An XML MSBuild(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public static class MSBuildImpl extends io.github.isotes.vs.model.impl.TaskTypeImpl implements io.github.isotes.vs.model.MSBuildDocument.MSBuild
{
private static final long serialVersionUID = 1L;
public MSBuildImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName BUILDINPARALLEL$0 =
new javax.xml.namespace.QName("", "BuildInParallel");
private static final javax.xml.namespace.QName PROJECTS$2 =
new javax.xml.namespace.QName("", "Projects");
private static final javax.xml.namespace.QName PROPERTIES$4 =
new javax.xml.namespace.QName("", "Properties");
private static final javax.xml.namespace.QName REBASEOUTPUTS$6 =
new javax.xml.namespace.QName("", "RebaseOutputs");
private static final javax.xml.namespace.QName RUNEACHTARGETSEPARATELY$8 =
new javax.xml.namespace.QName("", "RunEachTargetSeparately");
private static final javax.xml.namespace.QName SKIPNONEXISTENTPROJECTS$10 =
new javax.xml.namespace.QName("", "SkipNonexistentProjects");
private static final javax.xml.namespace.QName SKIPNONEXISTENTTARGETS$12 =
new javax.xml.namespace.QName("", "SkipNonexistentTargets");
private static final javax.xml.namespace.QName STOPONFIRSTFAILURE$14 =
new javax.xml.namespace.QName("", "StopOnFirstFailure");
private static final javax.xml.namespace.QName TARGETANDPROPERTYLISTSEPARATORS$16 =
new javax.xml.namespace.QName("", "TargetAndPropertyListSeparators");
private static final javax.xml.namespace.QName TARGETS$18 =
new javax.xml.namespace.QName("", "Targets");
private static final javax.xml.namespace.QName TOOLSVERSION$20 =
new javax.xml.namespace.QName("", "ToolsVersion");
private static final javax.xml.namespace.QName UNLOADPROJECTSONCOMPLETION$22 =
new javax.xml.namespace.QName("", "UnloadProjectsOnCompletion");
private static final javax.xml.namespace.QName USERESULTSCACHE$24 =
new javax.xml.namespace.QName("", "UseResultsCache");
/**
* Gets the "BuildInParallel" attribute
*/
public java.lang.String getBuildInParallel()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BUILDINPARALLEL$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "BuildInParallel" attribute
*/
public io.github.isotes.vs.model.Boolean xgetBuildInParallel()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(BUILDINPARALLEL$0);
return target;
}
}
/**
* True if has "BuildInParallel" attribute
*/
public boolean isSetBuildInParallel()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(BUILDINPARALLEL$0) != null;
}
}
/**
* Sets the "BuildInParallel" attribute
*/
public void setBuildInParallel(java.lang.String buildInParallel)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BUILDINPARALLEL$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BUILDINPARALLEL$0);
}
target.setStringValue(buildInParallel);
}
}
/**
* Sets (as xml) the "BuildInParallel" attribute
*/
public void xsetBuildInParallel(io.github.isotes.vs.model.Boolean buildInParallel)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(BUILDINPARALLEL$0);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(BUILDINPARALLEL$0);
}
target.set(buildInParallel);
}
}
/**
* Unsets the "BuildInParallel" attribute
*/
public void unsetBuildInParallel()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(BUILDINPARALLEL$0);
}
}
/**
* Gets the "Projects" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getProjects()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROJECTS$2);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "Projects" attribute
*/
public void setProjects(org.apache.xmlbeans.XmlAnySimpleType projects)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROJECTS$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROJECTS$2);
}
target.set(projects);
}
}
/**
* Appends and returns a new empty "Projects" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewProjects()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROJECTS$2);
return target;
}
}
/**
* Gets the "Properties" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getProperties()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROPERTIES$4);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Properties" attribute
*/
public boolean isSetProperties()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PROPERTIES$4) != null;
}
}
/**
* Sets the "Properties" attribute
*/
public void setProperties(org.apache.xmlbeans.XmlAnySimpleType properties)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROPERTIES$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROPERTIES$4);
}
target.set(properties);
}
}
/**
* Appends and returns a new empty "Properties" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewProperties()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROPERTIES$4);
return target;
}
}
/**
* Unsets the "Properties" attribute
*/
public void unsetProperties()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PROPERTIES$4);
}
}
/**
* Gets the "RebaseOutputs" attribute
*/
public java.lang.String getRebaseOutputs()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REBASEOUTPUTS$6);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "RebaseOutputs" attribute
*/
public io.github.isotes.vs.model.Boolean xgetRebaseOutputs()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(REBASEOUTPUTS$6);
return target;
}
}
/**
* True if has "RebaseOutputs" attribute
*/
public boolean isSetRebaseOutputs()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(REBASEOUTPUTS$6) != null;
}
}
/**
* Sets the "RebaseOutputs" attribute
*/
public void setRebaseOutputs(java.lang.String rebaseOutputs)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REBASEOUTPUTS$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REBASEOUTPUTS$6);
}
target.setStringValue(rebaseOutputs);
}
}
/**
* Sets (as xml) the "RebaseOutputs" attribute
*/
public void xsetRebaseOutputs(io.github.isotes.vs.model.Boolean rebaseOutputs)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(REBASEOUTPUTS$6);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(REBASEOUTPUTS$6);
}
target.set(rebaseOutputs);
}
}
/**
* Unsets the "RebaseOutputs" attribute
*/
public void unsetRebaseOutputs()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(REBASEOUTPUTS$6);
}
}
/**
* Gets the "RunEachTargetSeparately" attribute
*/
public java.lang.String getRunEachTargetSeparately()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(RUNEACHTARGETSEPARATELY$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "RunEachTargetSeparately" attribute
*/
public io.github.isotes.vs.model.Boolean xgetRunEachTargetSeparately()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(RUNEACHTARGETSEPARATELY$8);
return target;
}
}
/**
* True if has "RunEachTargetSeparately" attribute
*/
public boolean isSetRunEachTargetSeparately()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(RUNEACHTARGETSEPARATELY$8) != null;
}
}
/**
* Sets the "RunEachTargetSeparately" attribute
*/
public void setRunEachTargetSeparately(java.lang.String runEachTargetSeparately)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(RUNEACHTARGETSEPARATELY$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(RUNEACHTARGETSEPARATELY$8);
}
target.setStringValue(runEachTargetSeparately);
}
}
/**
* Sets (as xml) the "RunEachTargetSeparately" attribute
*/
public void xsetRunEachTargetSeparately(io.github.isotes.vs.model.Boolean runEachTargetSeparately)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(RUNEACHTARGETSEPARATELY$8);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(RUNEACHTARGETSEPARATELY$8);
}
target.set(runEachTargetSeparately);
}
}
/**
* Unsets the "RunEachTargetSeparately" attribute
*/
public void unsetRunEachTargetSeparately()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(RUNEACHTARGETSEPARATELY$8);
}
}
/**
* Gets the "SkipNonexistentProjects" attribute
*/
public java.lang.String getSkipNonexistentProjects()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SKIPNONEXISTENTPROJECTS$10);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "SkipNonexistentProjects" attribute
*/
public io.github.isotes.vs.model.Boolean xgetSkipNonexistentProjects()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(SKIPNONEXISTENTPROJECTS$10);
return target;
}
}
/**
* True if has "SkipNonexistentProjects" attribute
*/
public boolean isSetSkipNonexistentProjects()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(SKIPNONEXISTENTPROJECTS$10) != null;
}
}
/**
* Sets the "SkipNonexistentProjects" attribute
*/
public void setSkipNonexistentProjects(java.lang.String skipNonexistentProjects)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SKIPNONEXISTENTPROJECTS$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(SKIPNONEXISTENTPROJECTS$10);
}
target.setStringValue(skipNonexistentProjects);
}
}
/**
* Sets (as xml) the "SkipNonexistentProjects" attribute
*/
public void xsetSkipNonexistentProjects(io.github.isotes.vs.model.Boolean skipNonexistentProjects)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(SKIPNONEXISTENTPROJECTS$10);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(SKIPNONEXISTENTPROJECTS$10);
}
target.set(skipNonexistentProjects);
}
}
/**
* Unsets the "SkipNonexistentProjects" attribute
*/
public void unsetSkipNonexistentProjects()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(SKIPNONEXISTENTPROJECTS$10);
}
}
/**
* Gets the "SkipNonexistentTargets" attribute
*/
public java.lang.String getSkipNonexistentTargets()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SKIPNONEXISTENTTARGETS$12);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "SkipNonexistentTargets" attribute
*/
public io.github.isotes.vs.model.Boolean xgetSkipNonexistentTargets()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(SKIPNONEXISTENTTARGETS$12);
return target;
}
}
/**
* True if has "SkipNonexistentTargets" attribute
*/
public boolean isSetSkipNonexistentTargets()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(SKIPNONEXISTENTTARGETS$12) != null;
}
}
/**
* Sets the "SkipNonexistentTargets" attribute
*/
public void setSkipNonexistentTargets(java.lang.String skipNonexistentTargets)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SKIPNONEXISTENTTARGETS$12);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(SKIPNONEXISTENTTARGETS$12);
}
target.setStringValue(skipNonexistentTargets);
}
}
/**
* Sets (as xml) the "SkipNonexistentTargets" attribute
*/
public void xsetSkipNonexistentTargets(io.github.isotes.vs.model.Boolean skipNonexistentTargets)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(SKIPNONEXISTENTTARGETS$12);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(SKIPNONEXISTENTTARGETS$12);
}
target.set(skipNonexistentTargets);
}
}
/**
* Unsets the "SkipNonexistentTargets" attribute
*/
public void unsetSkipNonexistentTargets()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(SKIPNONEXISTENTTARGETS$12);
}
}
/**
* Gets the "StopOnFirstFailure" attribute
*/
public java.lang.String getStopOnFirstFailure()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(STOPONFIRSTFAILURE$14);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "StopOnFirstFailure" attribute
*/
public io.github.isotes.vs.model.Boolean xgetStopOnFirstFailure()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(STOPONFIRSTFAILURE$14);
return target;
}
}
/**
* True if has "StopOnFirstFailure" attribute
*/
public boolean isSetStopOnFirstFailure()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(STOPONFIRSTFAILURE$14) != null;
}
}
/**
* Sets the "StopOnFirstFailure" attribute
*/
public void setStopOnFirstFailure(java.lang.String stopOnFirstFailure)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(STOPONFIRSTFAILURE$14);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(STOPONFIRSTFAILURE$14);
}
target.setStringValue(stopOnFirstFailure);
}
}
/**
* Sets (as xml) the "StopOnFirstFailure" attribute
*/
public void xsetStopOnFirstFailure(io.github.isotes.vs.model.Boolean stopOnFirstFailure)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(STOPONFIRSTFAILURE$14);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(STOPONFIRSTFAILURE$14);
}
target.set(stopOnFirstFailure);
}
}
/**
* Unsets the "StopOnFirstFailure" attribute
*/
public void unsetStopOnFirstFailure()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(STOPONFIRSTFAILURE$14);
}
}
/**
* Gets the "TargetAndPropertyListSeparators" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getTargetAndPropertyListSeparators()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TARGETANDPROPERTYLISTSEPARATORS$16);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "TargetAndPropertyListSeparators" attribute
*/
public boolean isSetTargetAndPropertyListSeparators()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TARGETANDPROPERTYLISTSEPARATORS$16) != null;
}
}
/**
* Sets the "TargetAndPropertyListSeparators" attribute
*/
public void setTargetAndPropertyListSeparators(org.apache.xmlbeans.XmlAnySimpleType targetAndPropertyListSeparators)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TARGETANDPROPERTYLISTSEPARATORS$16);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TARGETANDPROPERTYLISTSEPARATORS$16);
}
target.set(targetAndPropertyListSeparators);
}
}
/**
* Appends and returns a new empty "TargetAndPropertyListSeparators" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewTargetAndPropertyListSeparators()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TARGETANDPROPERTYLISTSEPARATORS$16);
return target;
}
}
/**
* Unsets the "TargetAndPropertyListSeparators" attribute
*/
public void unsetTargetAndPropertyListSeparators()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TARGETANDPROPERTYLISTSEPARATORS$16);
}
}
/**
* Gets the "Targets" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getTargets()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TARGETS$18);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Targets" attribute
*/
public boolean isSetTargets()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TARGETS$18) != null;
}
}
/**
* Sets the "Targets" attribute
*/
public void setTargets(org.apache.xmlbeans.XmlAnySimpleType targets)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TARGETS$18);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TARGETS$18);
}
target.set(targets);
}
}
/**
* Appends and returns a new empty "Targets" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewTargets()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TARGETS$18);
return target;
}
}
/**
* Unsets the "Targets" attribute
*/
public void unsetTargets()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TARGETS$18);
}
}
/**
* Gets the "ToolsVersion" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getToolsVersion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TOOLSVERSION$20);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "ToolsVersion" attribute
*/
public boolean isSetToolsVersion()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TOOLSVERSION$20) != null;
}
}
/**
* Sets the "ToolsVersion" attribute
*/
public void setToolsVersion(org.apache.xmlbeans.XmlAnySimpleType toolsVersion)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TOOLSVERSION$20);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TOOLSVERSION$20);
}
target.set(toolsVersion);
}
}
/**
* Appends and returns a new empty "ToolsVersion" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewToolsVersion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TOOLSVERSION$20);
return target;
}
}
/**
* Unsets the "ToolsVersion" attribute
*/
public void unsetToolsVersion()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TOOLSVERSION$20);
}
}
/**
* Gets the "UnloadProjectsOnCompletion" attribute
*/
public java.lang.String getUnloadProjectsOnCompletion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "UnloadProjectsOnCompletion" attribute
*/
public io.github.isotes.vs.model.Boolean xgetUnloadProjectsOnCompletion()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
return target;
}
}
/**
* True if has "UnloadProjectsOnCompletion" attribute
*/
public boolean isSetUnloadProjectsOnCompletion()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(UNLOADPROJECTSONCOMPLETION$22) != null;
}
}
/**
* Sets the "UnloadProjectsOnCompletion" attribute
*/
public void setUnloadProjectsOnCompletion(java.lang.String unloadProjectsOnCompletion)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
}
target.setStringValue(unloadProjectsOnCompletion);
}
}
/**
* Sets (as xml) the "UnloadProjectsOnCompletion" attribute
*/
public void xsetUnloadProjectsOnCompletion(io.github.isotes.vs.model.Boolean unloadProjectsOnCompletion)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(UNLOADPROJECTSONCOMPLETION$22);
}
target.set(unloadProjectsOnCompletion);
}
}
/**
* Unsets the "UnloadProjectsOnCompletion" attribute
*/
public void unsetUnloadProjectsOnCompletion()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(UNLOADPROJECTSONCOMPLETION$22);
}
}
/**
* Gets the "UseResultsCache" attribute
*/
public java.lang.String getUseResultsCache()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USERESULTSCACHE$24);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "UseResultsCache" attribute
*/
public io.github.isotes.vs.model.Boolean xgetUseResultsCache()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USERESULTSCACHE$24);
return target;
}
}
/**
* True if has "UseResultsCache" attribute
*/
public boolean isSetUseResultsCache()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(USERESULTSCACHE$24) != null;
}
}
/**
* Sets the "UseResultsCache" attribute
*/
public void setUseResultsCache(java.lang.String useResultsCache)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USERESULTSCACHE$24);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(USERESULTSCACHE$24);
}
target.setStringValue(useResultsCache);
}
}
/**
* Sets (as xml) the "UseResultsCache" attribute
*/
public void xsetUseResultsCache(io.github.isotes.vs.model.Boolean useResultsCache)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USERESULTSCACHE$24);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(USERESULTSCACHE$24);
}
target.set(useResultsCache);
}
}
/**
* Unsets the "UseResultsCache" attribute
*/
public void unsetUseResultsCache()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(USERESULTSCACHE$24);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy