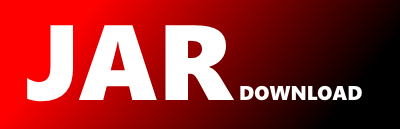
io.github.isotes.vs.model.impl.VCBuildDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vs-model Show documentation
Show all versions of vs-model Show documentation
Generated XMLBeans Java classes for the XML schema of MSBuild project files
The newest version!
/*
* An XML document type.
* Localname: VCBuild
* Namespace: http://schemas.microsoft.com/developer/msbuild/2003
* Java type: io.github.isotes.vs.model.VCBuildDocument
*
* Automatically generated - do not modify.
*/
package io.github.isotes.vs.model.impl;
/**
* A document containing one VCBuild(@http://schemas.microsoft.com/developer/msbuild/2003) element.
*
* This is a complex type.
*/
public class VCBuildDocumentImpl extends io.github.isotes.vs.model.impl.TaskDocumentImpl implements io.github.isotes.vs.model.VCBuildDocument
{
private static final long serialVersionUID = 1L;
public VCBuildDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName VCBUILD$0 =
new javax.xml.namespace.QName("http://schemas.microsoft.com/developer/msbuild/2003", "VCBuild");
/**
* Gets the "VCBuild" element
*/
public io.github.isotes.vs.model.VCBuildDocument.VCBuild getVCBuild()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.VCBuildDocument.VCBuild target = null;
target = (io.github.isotes.vs.model.VCBuildDocument.VCBuild)get_store().find_element_user(VCBUILD$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "VCBuild" element
*/
public void setVCBuild(io.github.isotes.vs.model.VCBuildDocument.VCBuild vcBuild)
{
generatedSetterHelperImpl(vcBuild, VCBUILD$0, 0, org.apache.xmlbeans.impl.values.XmlObjectBase.KIND_SETTERHELPER_SINGLETON);
}
/**
* Appends and returns a new empty "VCBuild" element
*/
public io.github.isotes.vs.model.VCBuildDocument.VCBuild addNewVCBuild()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.VCBuildDocument.VCBuild target = null;
target = (io.github.isotes.vs.model.VCBuildDocument.VCBuild)get_store().add_element_user(VCBUILD$0);
return target;
}
}
/**
* An XML VCBuild(@http://schemas.microsoft.com/developer/msbuild/2003).
*
* This is a complex type.
*/
public static class VCBuildImpl extends io.github.isotes.vs.model.impl.TaskTypeImpl implements io.github.isotes.vs.model.VCBuildDocument.VCBuild
{
private static final long serialVersionUID = 1L;
public VCBuildImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ADDITIONALLIBPATHS$0 =
new javax.xml.namespace.QName("", "AdditionalLibPaths");
private static final javax.xml.namespace.QName ADDITIONALLINKLIBRARYPATHS$2 =
new javax.xml.namespace.QName("", "AdditionalLinkLibraryPaths");
private static final javax.xml.namespace.QName ADDITIONALOPTIONS$4 =
new javax.xml.namespace.QName("", "AdditionalOptions");
private static final javax.xml.namespace.QName CLEAN$6 =
new javax.xml.namespace.QName("", "Clean");
private static final javax.xml.namespace.QName CONFIGURATION$8 =
new javax.xml.namespace.QName("", "Configuration");
private static final javax.xml.namespace.QName OVERRIDE$10 =
new javax.xml.namespace.QName("", "Override");
private static final javax.xml.namespace.QName PLATFORM$12 =
new javax.xml.namespace.QName("", "Platform");
private static final javax.xml.namespace.QName PROJECTS$14 =
new javax.xml.namespace.QName("", "Projects");
private static final javax.xml.namespace.QName REBUILD$16 =
new javax.xml.namespace.QName("", "Rebuild");
private static final javax.xml.namespace.QName SOLUTIONFILE$18 =
new javax.xml.namespace.QName("", "SolutionFile");
private static final javax.xml.namespace.QName TIMEOUT$20 =
new javax.xml.namespace.QName("", "Timeout");
private static final javax.xml.namespace.QName TOOLPATH$22 =
new javax.xml.namespace.QName("", "ToolPath");
private static final javax.xml.namespace.QName USEENVIRONMENT$24 =
new javax.xml.namespace.QName("", "UseEnvironment");
private static final javax.xml.namespace.QName USERENVIRONMENT$26 =
new javax.xml.namespace.QName("", "UserEnvironment");
/**
* Gets the "AdditionalLibPaths" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getAdditionalLibPaths()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALLIBPATHS$0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "AdditionalLibPaths" attribute
*/
public boolean isSetAdditionalLibPaths()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ADDITIONALLIBPATHS$0) != null;
}
}
/**
* Sets the "AdditionalLibPaths" attribute
*/
public void setAdditionalLibPaths(org.apache.xmlbeans.XmlAnySimpleType additionalLibPaths)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALLIBPATHS$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALLIBPATHS$0);
}
target.set(additionalLibPaths);
}
}
/**
* Appends and returns a new empty "AdditionalLibPaths" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewAdditionalLibPaths()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALLIBPATHS$0);
return target;
}
}
/**
* Unsets the "AdditionalLibPaths" attribute
*/
public void unsetAdditionalLibPaths()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ADDITIONALLIBPATHS$0);
}
}
/**
* Gets the "AdditionalLinkLibraryPaths" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getAdditionalLinkLibraryPaths()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALLINKLIBRARYPATHS$2);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "AdditionalLinkLibraryPaths" attribute
*/
public boolean isSetAdditionalLinkLibraryPaths()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ADDITIONALLINKLIBRARYPATHS$2) != null;
}
}
/**
* Sets the "AdditionalLinkLibraryPaths" attribute
*/
public void setAdditionalLinkLibraryPaths(org.apache.xmlbeans.XmlAnySimpleType additionalLinkLibraryPaths)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALLINKLIBRARYPATHS$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALLINKLIBRARYPATHS$2);
}
target.set(additionalLinkLibraryPaths);
}
}
/**
* Appends and returns a new empty "AdditionalLinkLibraryPaths" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewAdditionalLinkLibraryPaths()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALLINKLIBRARYPATHS$2);
return target;
}
}
/**
* Unsets the "AdditionalLinkLibraryPaths" attribute
*/
public void unsetAdditionalLinkLibraryPaths()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ADDITIONALLINKLIBRARYPATHS$2);
}
}
/**
* Gets the "AdditionalOptions" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getAdditionalOptions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALOPTIONS$4);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "AdditionalOptions" attribute
*/
public boolean isSetAdditionalOptions()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ADDITIONALOPTIONS$4) != null;
}
}
/**
* Sets the "AdditionalOptions" attribute
*/
public void setAdditionalOptions(org.apache.xmlbeans.XmlAnySimpleType additionalOptions)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(ADDITIONALOPTIONS$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALOPTIONS$4);
}
target.set(additionalOptions);
}
}
/**
* Appends and returns a new empty "AdditionalOptions" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewAdditionalOptions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(ADDITIONALOPTIONS$4);
return target;
}
}
/**
* Unsets the "AdditionalOptions" attribute
*/
public void unsetAdditionalOptions()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ADDITIONALOPTIONS$4);
}
}
/**
* Gets the "Clean" attribute
*/
public java.lang.String getClean()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(CLEAN$6);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "Clean" attribute
*/
public io.github.isotes.vs.model.Boolean xgetClean()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(CLEAN$6);
return target;
}
}
/**
* True if has "Clean" attribute
*/
public boolean isSetClean()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(CLEAN$6) != null;
}
}
/**
* Sets the "Clean" attribute
*/
public void setClean(java.lang.String clean)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(CLEAN$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(CLEAN$6);
}
target.setStringValue(clean);
}
}
/**
* Sets (as xml) the "Clean" attribute
*/
public void xsetClean(io.github.isotes.vs.model.Boolean clean)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(CLEAN$6);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(CLEAN$6);
}
target.set(clean);
}
}
/**
* Unsets the "Clean" attribute
*/
public void unsetClean()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(CLEAN$6);
}
}
/**
* Gets the "Configuration" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getConfiguration()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONFIGURATION$8);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Configuration" attribute
*/
public boolean isSetConfiguration()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(CONFIGURATION$8) != null;
}
}
/**
* Sets the "Configuration" attribute
*/
public void setConfiguration(org.apache.xmlbeans.XmlAnySimpleType configuration)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(CONFIGURATION$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONFIGURATION$8);
}
target.set(configuration);
}
}
/**
* Appends and returns a new empty "Configuration" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewConfiguration()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(CONFIGURATION$8);
return target;
}
}
/**
* Unsets the "Configuration" attribute
*/
public void unsetConfiguration()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(CONFIGURATION$8);
}
}
/**
* Gets the "Override" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getOverride()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(OVERRIDE$10);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Override" attribute
*/
public boolean isSetOverride()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(OVERRIDE$10) != null;
}
}
/**
* Sets the "Override" attribute
*/
public void setOverride(org.apache.xmlbeans.XmlAnySimpleType override)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(OVERRIDE$10);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(OVERRIDE$10);
}
target.set(override);
}
}
/**
* Appends and returns a new empty "Override" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewOverride()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(OVERRIDE$10);
return target;
}
}
/**
* Unsets the "Override" attribute
*/
public void unsetOverride()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(OVERRIDE$10);
}
}
/**
* Gets the "Platform" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getPlatform()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PLATFORM$12);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Platform" attribute
*/
public boolean isSetPlatform()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PLATFORM$12) != null;
}
}
/**
* Sets the "Platform" attribute
*/
public void setPlatform(org.apache.xmlbeans.XmlAnySimpleType platform)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PLATFORM$12);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PLATFORM$12);
}
target.set(platform);
}
}
/**
* Appends and returns a new empty "Platform" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewPlatform()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PLATFORM$12);
return target;
}
}
/**
* Unsets the "Platform" attribute
*/
public void unsetPlatform()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PLATFORM$12);
}
}
/**
* Gets the "Projects" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getProjects()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROJECTS$14);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "Projects" attribute
*/
public void setProjects(org.apache.xmlbeans.XmlAnySimpleType projects)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(PROJECTS$14);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROJECTS$14);
}
target.set(projects);
}
}
/**
* Appends and returns a new empty "Projects" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewProjects()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(PROJECTS$14);
return target;
}
}
/**
* Gets the "Rebuild" attribute
*/
public java.lang.String getRebuild()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REBUILD$16);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "Rebuild" attribute
*/
public io.github.isotes.vs.model.Boolean xgetRebuild()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(REBUILD$16);
return target;
}
}
/**
* True if has "Rebuild" attribute
*/
public boolean isSetRebuild()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(REBUILD$16) != null;
}
}
/**
* Sets the "Rebuild" attribute
*/
public void setRebuild(java.lang.String rebuild)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REBUILD$16);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REBUILD$16);
}
target.setStringValue(rebuild);
}
}
/**
* Sets (as xml) the "Rebuild" attribute
*/
public void xsetRebuild(io.github.isotes.vs.model.Boolean rebuild)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(REBUILD$16);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(REBUILD$16);
}
target.set(rebuild);
}
}
/**
* Unsets the "Rebuild" attribute
*/
public void unsetRebuild()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(REBUILD$16);
}
}
/**
* Gets the "SolutionFile" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getSolutionFile()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(SOLUTIONFILE$18);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "SolutionFile" attribute
*/
public boolean isSetSolutionFile()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(SOLUTIONFILE$18) != null;
}
}
/**
* Sets the "SolutionFile" attribute
*/
public void setSolutionFile(org.apache.xmlbeans.XmlAnySimpleType solutionFile)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(SOLUTIONFILE$18);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(SOLUTIONFILE$18);
}
target.set(solutionFile);
}
}
/**
* Appends and returns a new empty "SolutionFile" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewSolutionFile()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(SOLUTIONFILE$18);
return target;
}
}
/**
* Unsets the "SolutionFile" attribute
*/
public void unsetSolutionFile()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(SOLUTIONFILE$18);
}
}
/**
* Gets the "Timeout" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getTimeout()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TIMEOUT$20);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Timeout" attribute
*/
public boolean isSetTimeout()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TIMEOUT$20) != null;
}
}
/**
* Sets the "Timeout" attribute
*/
public void setTimeout(org.apache.xmlbeans.XmlAnySimpleType timeout)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TIMEOUT$20);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TIMEOUT$20);
}
target.set(timeout);
}
}
/**
* Appends and returns a new empty "Timeout" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewTimeout()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TIMEOUT$20);
return target;
}
}
/**
* Unsets the "Timeout" attribute
*/
public void unsetTimeout()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TIMEOUT$20);
}
}
/**
* Gets the "ToolPath" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType getToolPath()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TOOLPATH$22);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "ToolPath" attribute
*/
public boolean isSetToolPath()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TOOLPATH$22) != null;
}
}
/**
* Sets the "ToolPath" attribute
*/
public void setToolPath(org.apache.xmlbeans.XmlAnySimpleType toolPath)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().find_attribute_user(TOOLPATH$22);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TOOLPATH$22);
}
target.set(toolPath);
}
}
/**
* Appends and returns a new empty "ToolPath" attribute
*/
public org.apache.xmlbeans.XmlAnySimpleType addNewToolPath()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnySimpleType target = null;
target = (org.apache.xmlbeans.XmlAnySimpleType)get_store().add_attribute_user(TOOLPATH$22);
return target;
}
}
/**
* Unsets the "ToolPath" attribute
*/
public void unsetToolPath()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TOOLPATH$22);
}
}
/**
* Gets the "UseEnvironment" attribute
*/
public java.lang.String getUseEnvironment()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USEENVIRONMENT$24);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "UseEnvironment" attribute
*/
public io.github.isotes.vs.model.Boolean xgetUseEnvironment()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USEENVIRONMENT$24);
return target;
}
}
/**
* True if has "UseEnvironment" attribute
*/
public boolean isSetUseEnvironment()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(USEENVIRONMENT$24) != null;
}
}
/**
* Sets the "UseEnvironment" attribute
*/
public void setUseEnvironment(java.lang.String useEnvironment)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USEENVIRONMENT$24);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(USEENVIRONMENT$24);
}
target.setStringValue(useEnvironment);
}
}
/**
* Sets (as xml) the "UseEnvironment" attribute
*/
public void xsetUseEnvironment(io.github.isotes.vs.model.Boolean useEnvironment)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USEENVIRONMENT$24);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(USEENVIRONMENT$24);
}
target.set(useEnvironment);
}
}
/**
* Unsets the "UseEnvironment" attribute
*/
public void unsetUseEnvironment()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(USEENVIRONMENT$24);
}
}
/**
* Gets the "UserEnvironment" attribute
*/
public java.lang.String getUserEnvironment()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USERENVIRONMENT$26);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "UserEnvironment" attribute
*/
public io.github.isotes.vs.model.Boolean xgetUserEnvironment()
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USERENVIRONMENT$26);
return target;
}
}
/**
* True if has "UserEnvironment" attribute
*/
public boolean isSetUserEnvironment()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(USERENVIRONMENT$26) != null;
}
}
/**
* Sets the "UserEnvironment" attribute
*/
public void setUserEnvironment(java.lang.String userEnvironment)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(USERENVIRONMENT$26);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(USERENVIRONMENT$26);
}
target.setStringValue(userEnvironment);
}
}
/**
* Sets (as xml) the "UserEnvironment" attribute
*/
public void xsetUserEnvironment(io.github.isotes.vs.model.Boolean userEnvironment)
{
synchronized (monitor())
{
check_orphaned();
io.github.isotes.vs.model.Boolean target = null;
target = (io.github.isotes.vs.model.Boolean)get_store().find_attribute_user(USERENVIRONMENT$26);
if (target == null)
{
target = (io.github.isotes.vs.model.Boolean)get_store().add_attribute_user(USERENVIRONMENT$26);
}
target.set(userEnvironment);
}
}
/**
* Unsets the "UserEnvironment" attribute
*/
public void unsetUserEnvironment()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(USERENVIRONMENT$26);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy