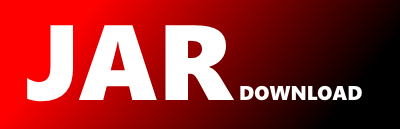
org.jgraph.utils.MathExtensions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freak-core Show documentation
Show all versions of freak-core Show documentation
Core library of the Free Evolutionary Algorithm Toolkit
package org.jgraph.utils;
import java.awt.geom.Point2D;
/**
* @author winkler
*
* To change this generated comment edit the template variable "typecomment":
* Window Preferences Java Templates.
* To enable and disable the creation of type comments go to
* Window Preferences Java Code Generation.
*/
public abstract class MathExtensions {
/******************************************************************************/
/**
* Extracts the leading sign of x.
*
* @param x Any double value.
* @return If x has a positive value -1.0
, for x = 0.0
* here comes 0.0
and if x has a negative the method returns
* -1.0
.
*/
public static double sgn(double x){
if( x < 0.0 ) {
return -1.0;
}
else if( x > 0.0 ){
return 1.0;
}
else {
return 0.0;
}
}
/******************************************************************************/
/**
* Computes the absolute value of v
. Assuming v
* is a mathematical Vector, pointing from Point Zero to the Point, represented
* by x
and y
in v, then this method returns the
* length of v.
*
* return sqrt( v.x + v.y )
*
* @param v Point the Vector is pointing at, coming from the point
* (0;0)
.
* @return Length of the mathematical Vector v, computed by Pytagoras's
* theorem.
*/
public static double abs(Point2D.Double v){
return Math.sqrt(getTransposed(v,v));
}
/******************************************************************************/
/**
* Computes the absolute value of a Vector, running from the
* Point (0;0)
to the Point (x;y)
. This is the length
* of that Vector.
*
* return sqrt( v.x� + v.y� )
*
* @param x Length of one Karthese. Between x and y is an Angle of 90�
* @param y Length of the other Karthese. Between x and y is an Angle of 90�
* @return Length of the Hypothenuse.
*/
public static double abs(double x, double y){
return (double) Math.sqrt( (x*x) + (y*y) );
}
/******************************************************************************/
/**
* Calculates the angle between v1 and v2. Assuming that v1 and v2 are
* mathematical Vectors, leading from the Point (0;0)
to
* their coordinates, the angle in (0;0)
is calculated.
*
* return arccos( ( v1.x*v2.x + v1.y*v2.y ) / ( sqrt( v1.x� + v1.y� ) *
* sqrt( v2.x� + v2.y� ) ) )
*
* @param v1 One of two Vectors leading from (0;0)
to
* (v1.x;v1.y)
* @param v2 One of two Vectors leading from (0;0)
to
* (v2.x;v2.y)
* @return The Angle between the two vectors
*/
public static double angleBetween(Point2D.Double v1, Point2D.Double v2){
double xty = getTransposed(v1,v2);
double lx = Math.sqrt(getTransposed(v1,v1));
double ly = Math.sqrt(getTransposed(v2,v2));
double result = xty/(lx*ly);
if( result > 1.0 ) result = 1.0; //gleicht rundungsfehler aus
if( result < -1.0 ) result = -1.0; //gleicht rundungsfehler aus
return Math.acos(result);
}
/******************************************************************************/
/**
* Calculates the Transposed of v1 and v2. It is assumed, that v1 and v2 are
* mathematical Vectors, leading from the Point (0;0)
to
* their coordinates.
*
* return v1.x * v2.x + v1.y * v2.y
*
* @param v1 Vector, leading from (0;0)
to the coordinates of
* the point.
* @param v2 Vector, leading from (0;0)
to the coordinates of
* the point.
* @return Transposed from v1 and v2.
*/
public static double getTransposed(Point2D.Double v1, Point2D.Double v2){
return v1.getX() * v2.getX() + v1.getY() * v2.getY();
}
/******************************************************************************/
/**
* Returns the euclidean Distance between two Points in a 2D cartesian
* coordinate system. The euclidean Distance is calculated in the following way:
*
*
* sqrt( (p1.x - p2.x)� + (p1.y - p2.y)� )
*
* @param p1 First of two Points, the Distance should be calculated between.
* @param p2 Second of two Points, the Distance should be calculated between.
* @return Distance between p1 and p2, calculated in the euclidean way.
*/
public static double getEuclideanDistance(Point2D.Double p1,
Point2D.Double p2){
return Math.sqrt(((p1.x-p2.x)*(p1.x-p2.x))+((p1.y-p2.y)*(p1.y-p2.y)));
}
/******************************************************************************/
public static Point2D.Double getNormalizedVector(Point2D.Double v){
double length = abs(v);
return new Point2D.Double(v.x / length, v.y / length );
}
/******************************************************************************/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy