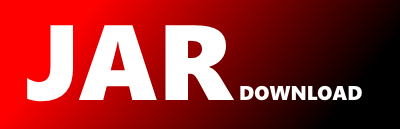
io.envoyproxy.envoy.admin.v2alpha.ClusterStatus.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.admin.v2alpha
/** Details an individual cluster's current status.
* [#next-free-field: 6]
*
* @param name
* Name of the cluster.
* @param addedViaApi
* Denotes whether this cluster was added via API or configured statically.
* @param successRateEjectionThreshold
* The success rate threshold used in the last interval.
* If
* :ref:`outlier_detection.split_external_local_origin_errors<envoy_api_field_cluster.OutlierDetection.split_external_local_origin_errors>`
* is *false*, all errors: externally and locally generated were used to calculate the threshold.
* If
* :ref:`outlier_detection.split_external_local_origin_errors<envoy_api_field_cluster.OutlierDetection.split_external_local_origin_errors>`
* is *true*, only externally generated errors were used to calculate the threshold.
* The threshold is used to eject hosts based on their success rate. See
* :ref:`Cluster outlier detection <arch_overview_outlier_detection>` documentation for details.
*
* Note: this field may be omitted in any of the three following cases:
*
* 1. There were not enough hosts with enough request volume to proceed with success rate based
* outlier ejection.
* 2. The threshold is computed to be < 0 because a negative value implies that there was no
* threshold for that interval.
* 3. Outlier detection is not enabled for this cluster.
* @param hostStatuses
* Mapping from host address to the host's current status.
* @param localOriginSuccessRateEjectionThreshold
* The success rate threshold used in the last interval when only locally originated failures were
* taken into account and externally originated errors were treated as success.
* This field should be interpreted only when
* :ref:`outlier_detection.split_external_local_origin_errors<envoy_api_field_cluster.OutlierDetection.split_external_local_origin_errors>`
* is *true*. The threshold is used to eject hosts based on their success rate.
* See :ref:`Cluster outlier detection <arch_overview_outlier_detection>` documentation for
* details.
*
* Note: this field may be omitted in any of the three following cases:
*
* 1. There were not enough hosts with enough request volume to proceed with success rate based
* outlier ejection.
* 2. The threshold is computed to be < 0 because a negative value implies that there was no
* threshold for that interval.
* 3. Outlier detection is not enabled for this cluster.
*/
@SerialVersionUID(0L)
final case class ClusterStatus(
name: _root_.scala.Predef.String = "",
addedViaApi: _root_.scala.Boolean = false,
successRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None,
hostStatuses: _root_.scala.Seq[io.envoyproxy.envoy.admin.v2alpha.HostStatus] = _root_.scala.Seq.empty,
localOriginSuccessRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[ClusterStatus] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = addedViaApi
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(2, __value)
}
};
if (successRateEjectionThreshold.isDefined) {
val __value = successRateEjectionThreshold.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
hostStatuses.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (localOriginSuccessRateEjectionThreshold.isDefined) {
val __value = localOriginSuccessRateEjectionThreshold.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = addedViaApi
if (__v != false) {
_output__.writeBool(2, __v)
}
};
successRateEjectionThreshold.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
hostStatuses.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
localOriginSuccessRateEjectionThreshold.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withName(__v: _root_.scala.Predef.String): ClusterStatus = copy(name = __v)
def withAddedViaApi(__v: _root_.scala.Boolean): ClusterStatus = copy(addedViaApi = __v)
def getSuccessRateEjectionThreshold: io.envoyproxy.envoy.`type`.Percent = successRateEjectionThreshold.getOrElse(io.envoyproxy.envoy.`type`.Percent.defaultInstance)
def clearSuccessRateEjectionThreshold: ClusterStatus = copy(successRateEjectionThreshold = _root_.scala.None)
def withSuccessRateEjectionThreshold(__v: io.envoyproxy.envoy.`type`.Percent): ClusterStatus = copy(successRateEjectionThreshold = Option(__v))
def clearHostStatuses = copy(hostStatuses = _root_.scala.Seq.empty)
def addHostStatuses(__vs: io.envoyproxy.envoy.admin.v2alpha.HostStatus *): ClusterStatus = addAllHostStatuses(__vs)
def addAllHostStatuses(__vs: Iterable[io.envoyproxy.envoy.admin.v2alpha.HostStatus]): ClusterStatus = copy(hostStatuses = hostStatuses ++ __vs)
def withHostStatuses(__v: _root_.scala.Seq[io.envoyproxy.envoy.admin.v2alpha.HostStatus]): ClusterStatus = copy(hostStatuses = __v)
def getLocalOriginSuccessRateEjectionThreshold: io.envoyproxy.envoy.`type`.Percent = localOriginSuccessRateEjectionThreshold.getOrElse(io.envoyproxy.envoy.`type`.Percent.defaultInstance)
def clearLocalOriginSuccessRateEjectionThreshold: ClusterStatus = copy(localOriginSuccessRateEjectionThreshold = _root_.scala.None)
def withLocalOriginSuccessRateEjectionThreshold(__v: io.envoyproxy.envoy.`type`.Percent): ClusterStatus = copy(localOriginSuccessRateEjectionThreshold = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = name
if (__t != "") __t else null
}
case 2 => {
val __t = addedViaApi
if (__t != false) __t else null
}
case 3 => successRateEjectionThreshold.orNull
case 4 => hostStatuses
case 5 => localOriginSuccessRateEjectionThreshold.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(name)
case 2 => _root_.scalapb.descriptors.PBoolean(addedViaApi)
case 3 => successRateEjectionThreshold.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => _root_.scalapb.descriptors.PRepeated(hostStatuses.iterator.map(_.toPMessage).toVector)
case 5 => localOriginSuccessRateEjectionThreshold.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.admin.v2alpha.ClusterStatus.type = io.envoyproxy.envoy.admin.v2alpha.ClusterStatus
// @@protoc_insertion_point(GeneratedMessage[envoy.admin.v2alpha.ClusterStatus])
}
object ClusterStatus extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.admin.v2alpha.ClusterStatus] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.admin.v2alpha.ClusterStatus] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.admin.v2alpha.ClusterStatus = {
var __name: _root_.scala.Predef.String = ""
var __addedViaApi: _root_.scala.Boolean = false
var __successRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None
val __hostStatuses: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.admin.v2alpha.HostStatus] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.admin.v2alpha.HostStatus]
var __localOriginSuccessRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__name = _input__.readStringRequireUtf8()
case 16 =>
__addedViaApi = _input__.readBool()
case 26 =>
__successRateEjectionThreshold = Option(__successRateEjectionThreshold.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.`type`.Percent](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 34 =>
__hostStatuses += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.admin.v2alpha.HostStatus](_input__)
case 42 =>
__localOriginSuccessRateEjectionThreshold = Option(__localOriginSuccessRateEjectionThreshold.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.`type`.Percent](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.admin.v2alpha.ClusterStatus(
name = __name,
addedViaApi = __addedViaApi,
successRateEjectionThreshold = __successRateEjectionThreshold,
hostStatuses = __hostStatuses.result(),
localOriginSuccessRateEjectionThreshold = __localOriginSuccessRateEjectionThreshold,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.admin.v2alpha.ClusterStatus] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.admin.v2alpha.ClusterStatus(
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
addedViaApi = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
successRateEjectionThreshold = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]]),
hostStatuses = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.admin.v2alpha.HostStatus]]).getOrElse(_root_.scala.Seq.empty),
localOriginSuccessRateEjectionThreshold = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = ClustersProto.javaDescriptor.getMessageTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = ClustersProto.scalaDescriptor.messages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 3 => __out = io.envoyproxy.envoy.`type`.Percent
case 4 => __out = io.envoyproxy.envoy.admin.v2alpha.HostStatus
case 5 => __out = io.envoyproxy.envoy.`type`.Percent
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.admin.v2alpha.ClusterStatus(
name = "",
addedViaApi = false,
successRateEjectionThreshold = _root_.scala.None,
hostStatuses = _root_.scala.Seq.empty,
localOriginSuccessRateEjectionThreshold = _root_.scala.None
)
implicit class ClusterStatusLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.admin.v2alpha.ClusterStatus]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.admin.v2alpha.ClusterStatus](_l) {
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def addedViaApi: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.addedViaApi)((c_, f_) => c_.copy(addedViaApi = f_))
def successRateEjectionThreshold: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.`type`.Percent] = field(_.getSuccessRateEjectionThreshold)((c_, f_) => c_.copy(successRateEjectionThreshold = Option(f_)))
def optionalSuccessRateEjectionThreshold: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]] = field(_.successRateEjectionThreshold)((c_, f_) => c_.copy(successRateEjectionThreshold = f_))
def hostStatuses: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.admin.v2alpha.HostStatus]] = field(_.hostStatuses)((c_, f_) => c_.copy(hostStatuses = f_))
def localOriginSuccessRateEjectionThreshold: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.`type`.Percent] = field(_.getLocalOriginSuccessRateEjectionThreshold)((c_, f_) => c_.copy(localOriginSuccessRateEjectionThreshold = Option(f_)))
def optionalLocalOriginSuccessRateEjectionThreshold: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]] = field(_.localOriginSuccessRateEjectionThreshold)((c_, f_) => c_.copy(localOriginSuccessRateEjectionThreshold = f_))
}
final val NAME_FIELD_NUMBER = 1
final val ADDED_VIA_API_FIELD_NUMBER = 2
final val SUCCESS_RATE_EJECTION_THRESHOLD_FIELD_NUMBER = 3
final val HOST_STATUSES_FIELD_NUMBER = 4
final val LOCAL_ORIGIN_SUCCESS_RATE_EJECTION_THRESHOLD_FIELD_NUMBER = 5
def of(
name: _root_.scala.Predef.String,
addedViaApi: _root_.scala.Boolean,
successRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent],
hostStatuses: _root_.scala.Seq[io.envoyproxy.envoy.admin.v2alpha.HostStatus],
localOriginSuccessRateEjectionThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]
): _root_.io.envoyproxy.envoy.admin.v2alpha.ClusterStatus = _root_.io.envoyproxy.envoy.admin.v2alpha.ClusterStatus(
name,
addedViaApi,
successRateEjectionThreshold,
hostStatuses,
localOriginSuccessRateEjectionThreshold
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.admin.v2alpha.ClusterStatus])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy