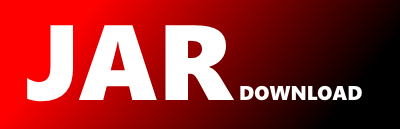
io.envoyproxy.envoy.api.v2.EndpointDiscoveryServiceGrpc.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
package io.envoyproxy.envoy.api.v2
object EndpointDiscoveryServiceGrpc {
val METHOD_STREAM_ENDPOINTS: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.api.v2.EndpointDiscoveryService", "StreamEndpoints"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor.getServices().get(0).getMethods().get(0)))
.build()
val METHOD_DELTA_ENDPOINTS: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest, io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.api.v2.EndpointDiscoveryService", "DeltaEndpoints"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor.getServices().get(0).getMethods().get(1)))
.build()
val METHOD_FETCH_ENDPOINTS: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.api.v2.EndpointDiscoveryService", "FetchEndpoints"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor.getServices().get(0).getMethods().get(2)))
.build()
val SERVICE: _root_.io.grpc.ServiceDescriptor =
_root_.io.grpc.ServiceDescriptor.newBuilder("envoy.api.v2.EndpointDiscoveryService")
.setSchemaDescriptor(new _root_.scalapb.grpc.ConcreteProtoFileDescriptorSupplier(io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor))
.addMethod(METHOD_STREAM_ENDPOINTS)
.addMethod(METHOD_DELTA_ENDPOINTS)
.addMethod(METHOD_FETCH_ENDPOINTS)
.build()
trait EndpointDiscoveryService extends _root_.scalapb.grpc.AbstractService {
override def serviceCompanion = EndpointDiscoveryService
/** The resource_names field in DiscoveryRequest specifies a list of clusters
* to subscribe to updates for.
*/
def streamEndpoints(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest]
def deltaEndpoints(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest]
def fetchEndpoints(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): scala.concurrent.Future[io.envoyproxy.envoy.api.v2.DiscoveryResponse]
}
object EndpointDiscoveryService extends _root_.scalapb.grpc.ServiceCompanion[EndpointDiscoveryService] {
implicit def serviceCompanion: _root_.scalapb.grpc.ServiceCompanion[EndpointDiscoveryService] = this
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor.getServices().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.ServiceDescriptor = io.envoyproxy.envoy.api.v2.EdsProto.scalaDescriptor.services(0)
def bindService(serviceImpl: EndpointDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition =
_root_.io.grpc.ServerServiceDefinition.builder(SERVICE)
.addMethod(
METHOD_STREAM_ENDPOINTS,
_root_.io.grpc.stub.ServerCalls.asyncBidiStreamingCall(new _root_.io.grpc.stub.ServerCalls.BidiStreamingMethod[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] {
override def invoke(observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest] =
serviceImpl.streamEndpoints(observer)
}))
.addMethod(
METHOD_DELTA_ENDPOINTS,
_root_.io.grpc.stub.ServerCalls.asyncBidiStreamingCall(new _root_.io.grpc.stub.ServerCalls.BidiStreamingMethod[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest, io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse] {
override def invoke(observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest] =
serviceImpl.deltaEndpoints(observer)
}))
.addMethod(
METHOD_FETCH_ENDPOINTS,
_root_.io.grpc.stub.ServerCalls.asyncUnaryCall(new _root_.io.grpc.stub.ServerCalls.UnaryMethod[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] {
override def invoke(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest, observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.scala.Unit =
serviceImpl.fetchEndpoints(request).onComplete(scalapb.grpc.Grpc.completeObserver(observer))(
executionContext)
}))
.build()
}
trait EndpointDiscoveryServiceBlockingClient {
def serviceCompanion = EndpointDiscoveryService
def fetchEndpoints(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): io.envoyproxy.envoy.api.v2.DiscoveryResponse
}
class EndpointDiscoveryServiceBlockingStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[EndpointDiscoveryServiceBlockingStub](channel, options) with EndpointDiscoveryServiceBlockingClient {
override def fetchEndpoints(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): io.envoyproxy.envoy.api.v2.DiscoveryResponse = {
_root_.scalapb.grpc.ClientCalls.blockingUnaryCall(channel, METHOD_FETCH_ENDPOINTS, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): EndpointDiscoveryServiceBlockingStub = new EndpointDiscoveryServiceBlockingStub(channel, options)
}
class EndpointDiscoveryServiceStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[EndpointDiscoveryServiceStub](channel, options) with EndpointDiscoveryService {
/** The resource_names field in DiscoveryRequest specifies a list of clusters
* to subscribe to updates for.
*/
override def streamEndpoints(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest] = {
_root_.scalapb.grpc.ClientCalls.asyncBidiStreamingCall(channel, METHOD_STREAM_ENDPOINTS, options, responseObserver)
}
override def deltaEndpoints(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest] = {
_root_.scalapb.grpc.ClientCalls.asyncBidiStreamingCall(channel, METHOD_DELTA_ENDPOINTS, options, responseObserver)
}
override def fetchEndpoints(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): scala.concurrent.Future[io.envoyproxy.envoy.api.v2.DiscoveryResponse] = {
_root_.scalapb.grpc.ClientCalls.asyncUnaryCall(channel, METHOD_FETCH_ENDPOINTS, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): EndpointDiscoveryServiceStub = new EndpointDiscoveryServiceStub(channel, options)
}
def bindService(serviceImpl: EndpointDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition = EndpointDiscoveryService.bindService(serviceImpl, executionContext)
def blockingStub(channel: _root_.io.grpc.Channel): EndpointDiscoveryServiceBlockingStub = new EndpointDiscoveryServiceBlockingStub(channel)
def stub(channel: _root_.io.grpc.Channel): EndpointDiscoveryServiceStub = new EndpointDiscoveryServiceStub(channel)
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.api.v2.EdsProto.javaDescriptor.getServices().get(0)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy