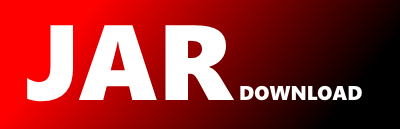
io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.api.v2.auth
/** TLS context shared by both client and server TLS contexts.
* [#next-free-field: 9]
*
* @param tlsParams
* TLS protocol versions, cipher suites etc.
* @param tlsCertificates
* :ref:`Multiple TLS certificates <arch_overview_ssl_cert_select>` can be associated with the
* same context to allow both RSA and ECDSA certificates.
*
* Only a single TLS certificate is supported in client contexts. In server contexts, the first
* RSA certificate is used for clients that only support RSA and the first ECDSA certificate is
* used for clients that support ECDSA.
* @param tlsCertificateSdsSecretConfigs
* Configs for fetching TLS certificates via SDS API.
* @param alpnProtocols
* Supplies the list of ALPN protocols that the listener should expose. In
* practice this is likely to be set to one of two values (see the
* :ref:`codec_type
* <envoy_api_field_config.filter.network.http_connection_manager.v2.HttpConnectionManager.codec_type>`
* parameter in the HTTP connection manager for more information):
*
* * "h2,http/1.1" If the listener is going to support both HTTP/2 and HTTP/1.1.
* * "http/1.1" If the listener is only going to support HTTP/1.1.
*
* There is no default for this parameter. If empty, Envoy will not expose ALPN.
*/
@SerialVersionUID(0L)
final case class CommonTlsContext(
tlsParams: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.TlsParameters] = _root_.scala.None,
tlsCertificates: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.TlsCertificate] = _root_.scala.Seq.empty,
tlsCertificateSdsSecretConfigs: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = _root_.scala.Seq.empty,
validationContextType: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.Empty,
alpnProtocols: _root_.scala.Seq[_root_.scala.Predef.String] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[CommonTlsContext] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (tlsParams.isDefined) {
val __value = tlsParams.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
tlsCertificates.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
tlsCertificateSdsSecretConfigs.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (validationContextType.validationContext.isDefined) {
val __value = validationContextType.validationContext.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (validationContextType.validationContextSdsSecretConfig.isDefined) {
val __value = validationContextType.validationContextSdsSecretConfig.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (validationContextType.combinedValidationContext.isDefined) {
val __value = validationContextType.combinedValidationContext.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
alpnProtocols.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(4, __value)
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
tlsParams.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
tlsCertificates.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
validationContextType.validationContext.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
alpnProtocols.foreach { __v =>
val __m = __v
_output__.writeString(4, __m)
};
tlsCertificateSdsSecretConfigs.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
validationContextType.validationContextSdsSecretConfig.foreach { __v =>
val __m = __v
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
validationContextType.combinedValidationContext.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getTlsParams: io.envoyproxy.envoy.api.v2.auth.TlsParameters = tlsParams.getOrElse(io.envoyproxy.envoy.api.v2.auth.TlsParameters.defaultInstance)
def clearTlsParams: CommonTlsContext = copy(tlsParams = _root_.scala.None)
def withTlsParams(__v: io.envoyproxy.envoy.api.v2.auth.TlsParameters): CommonTlsContext = copy(tlsParams = Option(__v))
def clearTlsCertificates = copy(tlsCertificates = _root_.scala.Seq.empty)
def addTlsCertificates(__vs: io.envoyproxy.envoy.api.v2.auth.TlsCertificate *): CommonTlsContext = addAllTlsCertificates(__vs)
def addAllTlsCertificates(__vs: Iterable[io.envoyproxy.envoy.api.v2.auth.TlsCertificate]): CommonTlsContext = copy(tlsCertificates = tlsCertificates ++ __vs)
def withTlsCertificates(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.TlsCertificate]): CommonTlsContext = copy(tlsCertificates = __v)
def clearTlsCertificateSdsSecretConfigs = copy(tlsCertificateSdsSecretConfigs = _root_.scala.Seq.empty)
def addTlsCertificateSdsSecretConfigs(__vs: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig *): CommonTlsContext = addAllTlsCertificateSdsSecretConfigs(__vs)
def addAllTlsCertificateSdsSecretConfigs(__vs: Iterable[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]): CommonTlsContext = copy(tlsCertificateSdsSecretConfigs = tlsCertificateSdsSecretConfigs ++ __vs)
def withTlsCertificateSdsSecretConfigs(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]): CommonTlsContext = copy(tlsCertificateSdsSecretConfigs = __v)
def getValidationContext: io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext = validationContextType.validationContext.getOrElse(io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext.defaultInstance)
def withValidationContext(__v: io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext): CommonTlsContext = copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContext(__v))
def getValidationContextSdsSecretConfig: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig = validationContextType.validationContextSdsSecretConfig.getOrElse(io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig.defaultInstance)
def withValidationContextSdsSecretConfig(__v: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig): CommonTlsContext = copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContextSdsSecretConfig(__v))
def getCombinedValidationContext: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext = validationContextType.combinedValidationContext.getOrElse(io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext.defaultInstance)
def withCombinedValidationContext(__v: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext): CommonTlsContext = copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.CombinedValidationContext(__v))
def clearAlpnProtocols = copy(alpnProtocols = _root_.scala.Seq.empty)
def addAlpnProtocols(__vs: _root_.scala.Predef.String *): CommonTlsContext = addAllAlpnProtocols(__vs)
def addAllAlpnProtocols(__vs: Iterable[_root_.scala.Predef.String]): CommonTlsContext = copy(alpnProtocols = alpnProtocols ++ __vs)
def withAlpnProtocols(__v: _root_.scala.Seq[_root_.scala.Predef.String]): CommonTlsContext = copy(alpnProtocols = __v)
def clearValidationContextType: CommonTlsContext = copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.Empty)
def withValidationContextType(__v: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType): CommonTlsContext = copy(validationContextType = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => tlsParams.orNull
case 2 => tlsCertificates
case 6 => tlsCertificateSdsSecretConfigs
case 3 => validationContextType.validationContext.orNull
case 7 => validationContextType.validationContextSdsSecretConfig.orNull
case 8 => validationContextType.combinedValidationContext.orNull
case 4 => alpnProtocols
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => tlsParams.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PRepeated(tlsCertificates.iterator.map(_.toPMessage).toVector)
case 6 => _root_.scalapb.descriptors.PRepeated(tlsCertificateSdsSecretConfigs.iterator.map(_.toPMessage).toVector)
case 3 => validationContextType.validationContext.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 7 => validationContextType.validationContextSdsSecretConfig.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 8 => validationContextType.combinedValidationContext.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => _root_.scalapb.descriptors.PRepeated(alpnProtocols.iterator.map(_root_.scalapb.descriptors.PString(_)).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.type = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.auth.CommonTlsContext])
}
object CommonTlsContext extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.auth.CommonTlsContext = {
var __tlsParams: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.TlsParameters] = _root_.scala.None
val __tlsCertificates: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.auth.TlsCertificate] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.auth.TlsCertificate]
val __tlsCertificateSdsSecretConfigs: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]
val __alpnProtocols: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String]
var __validationContextType: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.Empty
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__tlsParams = Option(__tlsParams.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.TlsParameters](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__tlsCertificates += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.TlsCertificate](_input__)
case 50 =>
__tlsCertificateSdsSecretConfigs += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig](_input__)
case 26 =>
__validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContext(__validationContextType.validationContext.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 58 =>
__validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContextSdsSecretConfig(__validationContextType.validationContextSdsSecretConfig.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 66 =>
__validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.CombinedValidationContext(__validationContextType.combinedValidationContext.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 34 =>
__alpnProtocols += _input__.readStringRequireUtf8()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.auth.CommonTlsContext(
tlsParams = __tlsParams,
tlsCertificates = __tlsCertificates.result(),
tlsCertificateSdsSecretConfigs = __tlsCertificateSdsSecretConfigs.result(),
alpnProtocols = __alpnProtocols.result(),
validationContextType = __validationContextType,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.auth.CommonTlsContext(
tlsParams = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.TlsParameters]]),
tlsCertificates = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.TlsCertificate]]).getOrElse(_root_.scala.Seq.empty),
tlsCertificateSdsSecretConfigs = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]]).getOrElse(_root_.scala.Seq.empty),
alpnProtocols = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[_root_.scala.Predef.String]]).getOrElse(_root_.scala.Seq.empty),
validationContextType = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext]]).map(io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContext(_))
.orElse[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType](__fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]]).map(io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContextSdsSecretConfig(_)))
.orElse[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType](__fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext]]).map(io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.CombinedValidationContext(_)))
.getOrElse(io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.Empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = TlsProto.javaDescriptor.getMessageTypes().get(2)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = TlsProto.scalaDescriptor.messages(2)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.api.v2.auth.TlsParameters
case 2 => __out = io.envoyproxy.envoy.api.v2.auth.TlsCertificate
case 6 => __out = io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig
case 3 => __out = io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext
case 7 => __out = io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig
case 8 => __out = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext(
tlsParams = _root_.scala.None,
tlsCertificates = _root_.scala.Seq.empty,
tlsCertificateSdsSecretConfigs = _root_.scala.Seq.empty,
alpnProtocols = _root_.scala.Seq.empty,
validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.Empty
)
sealed trait ValidationContextType extends _root_.scalapb.GeneratedOneof {
def isEmpty: _root_.scala.Boolean = false
def isDefined: _root_.scala.Boolean = true
def isValidationContext: _root_.scala.Boolean = false
def isValidationContextSdsSecretConfig: _root_.scala.Boolean = false
def isCombinedValidationContext: _root_.scala.Boolean = false
def validationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = _root_.scala.None
def validationContextSdsSecretConfig: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = _root_.scala.None
def combinedValidationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] = _root_.scala.None
}
object ValidationContextType {
@SerialVersionUID(0L)
case object Empty extends io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType {
type ValueType = _root_.scala.Nothing
override def isEmpty: _root_.scala.Boolean = true
override def isDefined: _root_.scala.Boolean = false
override def number: _root_.scala.Int = 0
override def value: _root_.scala.Nothing = throw new java.util.NoSuchElementException("Empty.value")
}
@SerialVersionUID(0L)
final case class ValidationContext(value: io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext) extends io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType {
type ValueType = io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext
override def isValidationContext: _root_.scala.Boolean = true
override def validationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = Some(value)
override def number: _root_.scala.Int = 3
}
@SerialVersionUID(0L)
final case class ValidationContextSdsSecretConfig(value: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig) extends io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType {
type ValueType = io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig
override def isValidationContextSdsSecretConfig: _root_.scala.Boolean = true
override def validationContextSdsSecretConfig: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = Some(value)
override def number: _root_.scala.Int = 7
}
@SerialVersionUID(0L)
final case class CombinedValidationContext(value: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext) extends io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType {
type ValueType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext
override def isCombinedValidationContext: _root_.scala.Boolean = true
override def combinedValidationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] = Some(value)
override def number: _root_.scala.Int = 8
}
}
/** @param defaultValidationContext
* How to validate peer certificates.
* @param validationContextSdsSecretConfig
* Config for fetching validation context via SDS API.
*/
@SerialVersionUID(0L)
final case class CombinedCertificateValidationContext(
defaultValidationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = _root_.scala.None,
validationContextSdsSecretConfig: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[CombinedCertificateValidationContext] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (defaultValidationContext.isDefined) {
val __value = defaultValidationContext.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (validationContextSdsSecretConfig.isDefined) {
val __value = validationContextSdsSecretConfig.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
defaultValidationContext.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
validationContextSdsSecretConfig.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getDefaultValidationContext: io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext = defaultValidationContext.getOrElse(io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext.defaultInstance)
def clearDefaultValidationContext: CombinedCertificateValidationContext = copy(defaultValidationContext = _root_.scala.None)
def withDefaultValidationContext(__v: io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext): CombinedCertificateValidationContext = copy(defaultValidationContext = Option(__v))
def getValidationContextSdsSecretConfig: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig = validationContextSdsSecretConfig.getOrElse(io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig.defaultInstance)
def clearValidationContextSdsSecretConfig: CombinedCertificateValidationContext = copy(validationContextSdsSecretConfig = _root_.scala.None)
def withValidationContextSdsSecretConfig(__v: io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig): CombinedCertificateValidationContext = copy(validationContextSdsSecretConfig = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => defaultValidationContext.orNull
case 2 => validationContextSdsSecretConfig.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => defaultValidationContext.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => validationContextSdsSecretConfig.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext.type = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext])
}
object CombinedCertificateValidationContext extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext = {
var __defaultValidationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = _root_.scala.None
var __validationContextSdsSecretConfig: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__defaultValidationContext = Option(__defaultValidationContext.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__validationContextSdsSecretConfig = Option(__validationContextSdsSecretConfig.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext(
defaultValidationContext = __defaultValidationContext,
validationContextSdsSecretConfig = __validationContextSdsSecretConfig,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext(
defaultValidationContext = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext]]),
validationContextSdsSecretConfig = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext
case 2 => __out = io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext(
defaultValidationContext = _root_.scala.None,
validationContextSdsSecretConfig = _root_.scala.None
)
implicit class CombinedCertificateValidationContextLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext](_l) {
def defaultValidationContext: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = field(_.getDefaultValidationContext)((c_, f_) => c_.copy(defaultValidationContext = Option(f_)))
def optionalDefaultValidationContext: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext]] = field(_.defaultValidationContext)((c_, f_) => c_.copy(defaultValidationContext = f_))
def validationContextSdsSecretConfig: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = field(_.getValidationContextSdsSecretConfig)((c_, f_) => c_.copy(validationContextSdsSecretConfig = Option(f_)))
def optionalValidationContextSdsSecretConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]] = field(_.validationContextSdsSecretConfig)((c_, f_) => c_.copy(validationContextSdsSecretConfig = f_))
}
final val DEFAULT_VALIDATION_CONTEXT_FIELD_NUMBER = 1
final val VALIDATION_CONTEXT_SDS_SECRET_CONFIG_FIELD_NUMBER = 2
def of(
defaultValidationContext: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext],
validationContextSdsSecretConfig: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]
): _root_.io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext = _root_.io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext(
defaultValidationContext,
validationContextSdsSecretConfig
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext])
}
implicit class CommonTlsContextLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext](_l) {
def tlsParams: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.TlsParameters] = field(_.getTlsParams)((c_, f_) => c_.copy(tlsParams = Option(f_)))
def optionalTlsParams: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.TlsParameters]] = field(_.tlsParams)((c_, f_) => c_.copy(tlsParams = f_))
def tlsCertificates: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.TlsCertificate]] = field(_.tlsCertificates)((c_, f_) => c_.copy(tlsCertificates = f_))
def tlsCertificateSdsSecretConfigs: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig]] = field(_.tlsCertificateSdsSecretConfigs)((c_, f_) => c_.copy(tlsCertificateSdsSecretConfigs = f_))
def validationContext: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CertificateValidationContext] = field(_.getValidationContext)((c_, f_) => c_.copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContext(f_)))
def validationContextSdsSecretConfig: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig] = field(_.getValidationContextSdsSecretConfig)((c_, f_) => c_.copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.ValidationContextSdsSecretConfig(f_)))
def combinedValidationContext: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.CombinedCertificateValidationContext] = field(_.getCombinedValidationContext)((c_, f_) => c_.copy(validationContextType = io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType.CombinedValidationContext(f_)))
def alpnProtocols: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Predef.String]] = field(_.alpnProtocols)((c_, f_) => c_.copy(alpnProtocols = f_))
def validationContextType: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType] = field(_.validationContextType)((c_, f_) => c_.copy(validationContextType = f_))
}
final val TLS_PARAMS_FIELD_NUMBER = 1
final val TLS_CERTIFICATES_FIELD_NUMBER = 2
final val TLS_CERTIFICATE_SDS_SECRET_CONFIGS_FIELD_NUMBER = 6
final val VALIDATION_CONTEXT_FIELD_NUMBER = 3
final val VALIDATION_CONTEXT_SDS_SECRET_CONFIG_FIELD_NUMBER = 7
final val COMBINED_VALIDATION_CONTEXT_FIELD_NUMBER = 8
final val ALPN_PROTOCOLS_FIELD_NUMBER = 4
def of(
tlsParams: _root_.scala.Option[io.envoyproxy.envoy.api.v2.auth.TlsParameters],
tlsCertificates: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.TlsCertificate],
tlsCertificateSdsSecretConfigs: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.auth.SdsSecretConfig],
validationContextType: io.envoyproxy.envoy.api.v2.auth.CommonTlsContext.ValidationContextType,
alpnProtocols: _root_.scala.Seq[_root_.scala.Predef.String]
): _root_.io.envoyproxy.envoy.api.v2.auth.CommonTlsContext = _root_.io.envoyproxy.envoy.api.v2.auth.CommonTlsContext(
tlsParams,
tlsCertificates,
tlsCertificateSdsSecretConfigs,
validationContextType,
alpnProtocols
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.auth.CommonTlsContext])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy